STM32F072B-Discovery BSP User Manual
|
stm32f072b_discovery_gyroscope.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32f072b_discovery_gyroscope.c 00004 * @author MCD Application Team 00005 * @brief This file provides a set of functions needed to manage the l3gd20 00006 * MEMS accelerometer available on STM32F072B-Discovery Kit. 00007 ****************************************************************************** 00008 * @attention 00009 * 00010 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00011 * 00012 * Redistribution and use in source and binary forms, with or without modification, 00013 * are permitted provided that the following conditions are met: 00014 * 1. Redistributions of source code must retain the above copyright notice, 00015 * this list of conditions and the following disclaimer. 00016 * 2. Redistributions in binary form must reproduce the above copyright notice, 00017 * this list of conditions and the following disclaimer in the documentation 00018 * and/or other materials provided with the distribution. 00019 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00020 * may be used to endorse or promote products derived from this software 00021 * without specific prior written permission. 00022 * 00023 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00024 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00025 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00026 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00027 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00028 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00029 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00030 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00031 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00032 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00033 * 00034 ****************************************************************************** 00035 */ 00036 /* Includes ------------------------------------------------------------------*/ 00037 #include "stm32f072b_discovery_gyroscope.h" 00038 00039 /** @addtogroup BSP 00040 * @{ 00041 */ 00042 00043 /** @addtogroup STM32F072B_DISCOVERY 00044 * @{ 00045 */ 00046 00047 /** @addtogroup STM32F072B_DISCOVERY_GYRO 00048 * @{ 00049 */ 00050 00051 /** @defgroup STM32F072B_DISCOVERY_GYRO_Private_Variables Private Variables 00052 * @{ 00053 */ 00054 static GYRO_DrvTypeDef *GyroscopeDrv; 00055 00056 /** 00057 * @} 00058 */ 00059 00060 /** @addtogroup STM32F072B_DISCOVERY_GYRO_Exported_Functions 00061 * @{ 00062 */ 00063 00064 /** 00065 * @brief Set GYRO Initialization. 00066 * @retval GYRO_OK if no problem during initialization 00067 */ 00068 uint8_t BSP_GYRO_Init(void) 00069 { 00070 uint8_t ret = GYRO_ERROR; 00071 uint16_t ctrl = 0x0000; 00072 GYRO_InitTypeDef L3GD20_InitStructure; 00073 GYRO_FilterConfigTypeDef L3GD20_FilterStructure={0,0}; 00074 00075 if((L3gd20Drv.ReadID() == I_AM_L3GD20) || (L3gd20Drv.ReadID() == I_AM_L3GD20_TR)) 00076 { 00077 /* Initialize the gyroscope driver structure */ 00078 GyroscopeDrv = &L3gd20Drv; 00079 00080 /* Configure Mems : data rate, power mode, full scale and axes */ 00081 L3GD20_InitStructure.Power_Mode = L3GD20_MODE_ACTIVE; 00082 L3GD20_InitStructure.Output_DataRate = L3GD20_OUTPUT_DATARATE_1; 00083 L3GD20_InitStructure.Axes_Enable = L3GD20_AXES_ENABLE; 00084 L3GD20_InitStructure.Band_Width = L3GD20_BANDWIDTH_4; 00085 L3GD20_InitStructure.BlockData_Update = L3GD20_BlockDataUpdate_Continous; 00086 L3GD20_InitStructure.Endianness = L3GD20_BLE_LSB; 00087 L3GD20_InitStructure.Full_Scale = L3GD20_FULLSCALE_500; 00088 00089 /* Configure MEMS: data rate, power mode, full scale and axes */ 00090 ctrl = (uint16_t) (L3GD20_InitStructure.Power_Mode | L3GD20_InitStructure.Output_DataRate | \ 00091 L3GD20_InitStructure.Axes_Enable | L3GD20_InitStructure.Band_Width); 00092 00093 ctrl |= (uint16_t) ((L3GD20_InitStructure.BlockData_Update | L3GD20_InitStructure.Endianness | \ 00094 L3GD20_InitStructure.Full_Scale) << 8); 00095 00096 /* L3gd20 Init */ 00097 GyroscopeDrv->Init(ctrl); 00098 00099 L3GD20_FilterStructure.HighPassFilter_Mode_Selection =L3GD20_HPM_NORMAL_MODE_RES; 00100 L3GD20_FilterStructure.HighPassFilter_CutOff_Frequency = L3GD20_HPFCF_0; 00101 00102 ctrl = (uint8_t) ((L3GD20_FilterStructure.HighPassFilter_Mode_Selection |\ 00103 L3GD20_FilterStructure.HighPassFilter_CutOff_Frequency)); 00104 00105 GyroscopeDrv->FilterConfig(ctrl) ; 00106 00107 GyroscopeDrv->FilterCmd(L3GD20_HIGHPASSFILTER_ENABLE); 00108 00109 ret = GYRO_OK; 00110 } 00111 else 00112 { 00113 ret = GYRO_ERROR; 00114 } 00115 00116 return ret; 00117 } 00118 00119 /** 00120 * @brief Read ID of Gyroscope component 00121 * @retval ID 00122 */ 00123 uint8_t BSP_GYRO_ReadID(void) 00124 { 00125 uint8_t id = 0x00; 00126 00127 if(GyroscopeDrv->ReadID != NULL) 00128 { 00129 id = GyroscopeDrv->ReadID(); 00130 } 00131 return id; 00132 } 00133 00134 /** 00135 * @brief Reboot memory content of GYRO 00136 * @retval None 00137 */ 00138 void BSP_GYRO_Reset(void) 00139 { 00140 if(GyroscopeDrv->Reset != NULL) 00141 { 00142 GyroscopeDrv->Reset(); 00143 } 00144 } 00145 00146 /** 00147 * @brief Configure INT1 interrupt 00148 * @param pIntConfig pointer to a L3GD20_InterruptConfig_TypeDef 00149 * structure that contains the configuration setting for the L3GD20 Interrupt. 00150 * @retval None 00151 */ 00152 void BSP_GYRO_ITConfig(GYRO_InterruptConfigTypeDef *pIntConfig) 00153 { 00154 uint16_t interruptconfig = 0x0000; 00155 00156 if(GyroscopeDrv->ConfigIT != NULL) 00157 { 00158 /* Configure latch Interrupt request and axe interrupts */ 00159 interruptconfig |= ((uint8_t)(pIntConfig->Latch_Request| \ 00160 pIntConfig->Interrupt_Axes) << 8); 00161 00162 interruptconfig |= (uint8_t)(pIntConfig->Interrupt_ActiveEdge); 00163 00164 GyroscopeDrv->ConfigIT(interruptconfig); 00165 } 00166 } 00167 00168 /** 00169 * @brief Enable INT1 or INT2 interrupt 00170 * @param IntPin Interrupt pin 00171 * This parameter can be: 00172 * @arg L3GD20_INT1 00173 * @arg L3GD20_INT2 00174 * @retval None 00175 */ 00176 void BSP_GYRO_EnableIT(uint8_t IntPin) 00177 { 00178 if(GyroscopeDrv->EnableIT != NULL) 00179 { 00180 GyroscopeDrv->EnableIT(IntPin); 00181 } 00182 } 00183 00184 /** 00185 * @brief Disable INT1 or INT2 interrupt 00186 * @param IntPin Interrupt pin 00187 * This parameter can be: 00188 * @arg L3GD20_INT1 00189 * @arg L3GD20_INT2 00190 * @retval None 00191 */ 00192 void BSP_GYRO_DisableIT(uint8_t IntPin) 00193 { 00194 if(GyroscopeDrv->DisableIT != NULL) 00195 { 00196 GyroscopeDrv->DisableIT(IntPin); 00197 } 00198 } 00199 00200 /** 00201 * @brief Get XYZ angular acceleration 00202 * @param pfData pointer on floating array 00203 * @retval None 00204 */ 00205 void BSP_GYRO_GetXYZ(float* pfData) 00206 { 00207 if(GyroscopeDrv->GetXYZ!= NULL) 00208 { 00209 GyroscopeDrv->GetXYZ(pfData); 00210 } 00211 } 00212 00213 /** 00214 * @} 00215 */ 00216 00217 /** 00218 * @} 00219 */ 00220 00221 /** 00222 * @} 00223 */ 00224 00225 /** 00226 * @} 00227 */ 00228 00229 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Wed Jul 5 2017 09:43:19 for STM32F072B-Discovery BSP User Manual by
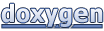