STM324xG_EVAL BSP User Manual
|
stm324xg_eval_io.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm324xg_eval_io.c 00004 * @author MCD Application Team 00005 * @version V2.2.1 00006 * @date 15-January-2016 00007 * @brief This file provides a set of functions needed to manage the IO pins 00008 * on STM324xG-EVAL evaluation board. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* File Info : ----------------------------------------------------------------- 00040 User NOTES 00041 1. How To use this driver: 00042 -------------------------- 00043 - This driver is used to drive the IO module of the STM324xG-EVAL evaluation 00044 board. 00045 - The STMPE811 IO expander device component driver must be included with this 00046 driver in order to run the IO functionalities commanded by the IO expander 00047 device mounted on the evaluation board. 00048 00049 2. Driver description: 00050 --------------------- 00051 + Initialization steps: 00052 o Initialize the IO module using the BSP_IO_Init() function. This 00053 function includes the MSP layer hardware resources initialization and the 00054 communication layer configuration to start the IO functionalities use. 00055 00056 + IO functionalities use 00057 o The IO pin mode is configured when calling the function BSP_IO_ConfigPin(), you 00058 must specify the desired IO mode by choosing the "IO_ModeTypedef" parameter 00059 predefined value. 00060 o If an IO pin is used in interrupt mode, the function BSP_IO_ITGetStatus() is 00061 needed to get the interrupt status. To clear the IT pending bits, you should 00062 call the function BSP_IO_ITClear() with specifying the IO pending bit to clear. 00063 o The IT is handled using the corresponding external interrupt IRQ handler, 00064 the user IT callback treatment is implemented on the same external interrupt 00065 callback. 00066 o To get/set an IO pin combination state you can use the functions 00067 BSP_IO_ReadPin()/BSP_IO_WritePin() or the function BSP_IO_TogglePin() to toggle the pin 00068 state. 00069 00070 ------------------------------------------------------------------------------*/ 00071 00072 /* Includes ------------------------------------------------------------------*/ 00073 #include "stm324xg_eval_io.h" 00074 00075 /** @addtogroup BSP 00076 * @{ 00077 */ 00078 00079 /** @addtogroup STM324xG_EVAL 00080 * @{ 00081 */ 00082 00083 /** @defgroup STM324xG_EVAL_IO STM324xG EVAL IO 00084 * @{ 00085 */ 00086 00087 /** @defgroup STM324xG_EVAL_IO_Private_Types_Definitions STM324xG EVAL IO Private Types Definitions 00088 * @{ 00089 */ 00090 /** 00091 * @} 00092 */ 00093 00094 /** @defgroup STM324xG_EVAL_IO_Private_Defines STM324xG EVAL IO Private Defines 00095 * @{ 00096 */ 00097 /** 00098 * @} 00099 */ 00100 00101 /** @defgroup STM324xG_EVAL_IO_Private_Macros STM324xG EVAL IO Private Macros 00102 * @{ 00103 */ 00104 /** 00105 * @} 00106 */ 00107 00108 /** @defgroup STM324xG_EVAL_IO_Private_Variables STM324xG EVAL IO Private Variables 00109 * @{ 00110 */ 00111 static IO_DrvTypeDef *io_driver; 00112 /** 00113 * @} 00114 */ 00115 00116 /** @defgroup STM324xG_EVAL_IO_Private_Function_Prototypes STM324xG EVAL IO Private Function Prototypes 00117 * @{ 00118 */ 00119 /** 00120 * @} 00121 */ 00122 00123 /** @defgroup STM324xG_EVAL_IO_Private_Functions STM324xG EVAL IO Private Functions 00124 * @{ 00125 */ 00126 00127 /** 00128 * @brief Initializes and configures the IO functionalities and configures all 00129 * necessary hardware resources (GPIOs, clocks..). 00130 * @note BSP_IO_Init() is using HAL_Delay() function to ensure that stmpe811 00131 * IO Expander is correctly reset. HAL_Delay() function provides accurate 00132 * delay (in milliseconds) based on variable incremented in SysTick ISR. 00133 * This implies that if BSP_IO_Init() is called from a peripheral ISR process, 00134 * then the SysTick interrupt must have higher priority (numerically lower) 00135 * than the peripheral interrupt. Otherwise the caller ISR process will be blocked. 00136 * @retval IO_OK: if all initializations are OK. Other value if error. 00137 */ 00138 uint8_t BSP_IO_Init(void) 00139 { 00140 uint8_t ret = IO_ERROR; 00141 00142 if(stmpe811_io_drv.ReadID(IO_I2C_ADDRESS) == STMPE811_ID) 00143 { 00144 /* Initialize the IO driver structure */ 00145 io_driver = &stmpe811_io_drv; 00146 00147 io_driver->Init(IO_I2C_ADDRESS); 00148 io_driver->Start(IO_I2C_ADDRESS, IO_PIN_ALL); 00149 00150 ret = IO_OK; 00151 } 00152 00153 return ret; 00154 } 00155 00156 /** 00157 * @brief Gets the selected pins IT status. 00158 * @param IO_Pin: Selected pins to check the status. 00159 * This parameter can be any combination of the IO pins. 00160 * @retval IO_OK: if read status OK. Other value if error. 00161 */ 00162 uint8_t BSP_IO_ITGetStatus(uint16_t IO_Pin) 00163 { 00164 /* Return the IO Pin IT status */ 00165 return (io_driver->ITStatus(IO_I2C_ADDRESS, IO_Pin)); 00166 } 00167 00168 /** 00169 * @brief Clears the selected IO IT pending bit. 00170 * @param IO_Pin: Selected pins to check the status. 00171 * This parameter can be any combination of the IO pins. 00172 */ 00173 void BSP_IO_ITClear(uint16_t IO_Pin) 00174 { 00175 io_driver->ClearIT(IO_I2C_ADDRESS, IO_Pin); 00176 } 00177 00178 /** 00179 * @brief Configures the IO pin(s) according to IO mode structure value. 00180 * @param IO_Pin: Output pin to be set or reset. 00181 * This parameter can be one of the following values: 00182 * @arg STMPE811_PIN_x: where x can be from 0 to 7 00183 * @param IO_Mode: IO pin mode to configure 00184 * This parameter can be one of the following values: 00185 * @arg IO_MODE_INPUT 00186 * @arg IO_MODE_OUTPUT 00187 * @arg IO_MODE_IT_RISING_EDGE 00188 * @arg IO_MODE_IT_FALLING_EDGE 00189 * @arg IO_MODE_IT_LOW_LEVEL 00190 * @arg IO_MODE_IT_HIGH_LEVEL 00191 * @retval IO_OK: if all initializations are OK. Other value if error. 00192 */ 00193 uint8_t BSP_IO_ConfigPin(uint16_t IO_Pin, IO_ModeTypedef IO_Mode) 00194 { 00195 /* Configure the selected IO pin(s) mode */ 00196 io_driver->Config(IO_I2C_ADDRESS, IO_Pin, IO_Mode); 00197 00198 return IO_OK; 00199 } 00200 00201 /** 00202 * @brief Sets the selected pins state. 00203 * @param IO_Pin: Selected pins to write. 00204 * This parameter can be any combination of the IO pins. 00205 * @param PinState: New pins state to write 00206 */ 00207 void BSP_IO_WritePin(uint16_t IO_Pin, uint8_t PinState) 00208 { 00209 io_driver->WritePin(IO_I2C_ADDRESS, IO_Pin, PinState); 00210 } 00211 00212 /** 00213 * @brief Gets the selected pins current state. 00214 * @param IO_Pin: Selected pins to read. 00215 * This parameter can be any combination of the IO pins. 00216 * @retval The current pins state 00217 */ 00218 uint16_t BSP_IO_ReadPin(uint16_t IO_Pin) 00219 { 00220 return(io_driver->ReadPin(IO_I2C_ADDRESS, IO_Pin)); 00221 } 00222 00223 /** 00224 * @brief Toggles the selected pins state 00225 * @param IO_Pin: Selected pins to toggle. 00226 * This parameter can be any combination of the IO pins. 00227 */ 00228 void BSP_IO_TogglePin(uint16_t IO_Pin) 00229 { 00230 if(io_driver->ReadPin(IO_I2C_ADDRESS, IO_Pin) == 1) /* Set */ 00231 { 00232 io_driver->WritePin(IO_I2C_ADDRESS, IO_Pin, 0); /* Reset */ 00233 } 00234 else 00235 { 00236 io_driver->WritePin(IO_I2C_ADDRESS, IO_Pin, 1); /* Set */ 00237 } 00238 } 00239 00240 /** 00241 * @} 00242 */ 00243 00244 /** 00245 * @} 00246 */ 00247 00248 /** 00249 * @} 00250 */ 00251 00252 /** 00253 * @} 00254 */ 00255 00256 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Fri Jan 15 2016 14:22:29 for STM324xG_EVAL BSP User Manual by
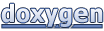