STM324xG_EVAL BSP User Manual
|
stm324xg_eval_eeprom.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm324xg_eval_eeprom.c 00004 * @author MCD Application Team 00005 * @version V2.2.1 00006 * @date 15-January-2016 00007 * @brief This file provides a set of functions needed to manage an I2C M24C64 00008 * EEPROM memory. 00009 * 00010 * =================================================================== 00011 * Notes: 00012 * - This driver is intended for STM32F4xx families devices only. 00013 * - The I2C EEPROM memory (M24C64) is available on STM324xG-EVAL 00014 * - To use this driver you have to connect the eeprom jumper (JP24). 00015 * =================================================================== 00016 * 00017 * It implements a high level communication layer for read and write 00018 * from/to this memory. The needed STM32F4xx hardware resources (I2C and 00019 * GPIO) are defined in stm32f4xg_eval.h file, and the initialization is 00020 * performed in EEPROM_IO_Init() function declared in stm32f4xg_eval.c 00021 * file. 00022 * You can easily tailor this driver to any other development board, 00023 * by just adapting the defines for hardware resources and 00024 * EEPROM_IO_Init() function. 00025 * 00026 * @note In this driver, basic read and write functions (BSP_EEPROM_ReadBuffer() 00027 * and EEPROM_WritePage()) use Polling mode to perform the data transfer 00028 * to/from EEPROM memory. 00029 * 00030 * +-----------------------------------------------------------------+ 00031 * | Pin assignment for M24C64 EEPROM | 00032 * +---------------------------------------+-----------+-------------+ 00033 * | STM32F4xx I2C Pins | EEPROM | Pin | 00034 * +---------------------------------------+-----------+-------------+ 00035 * | . | E0 | 1 (0V) | 00036 * | . | E1 | 2 (0V) | 00037 * | . | E2 | 3 (0V) | 00038 * | . | VSS(GND)| 4 (0V) | 00039 * | SDA | SDA | 5 | 00040 * | SCL | SCL | 6 | 00041 * | JP24 | /WS | 7 | 00042 * | . | VDD | 8 (3.3V) | 00043 * +---------------------------------------+-----------+-------------+ 00044 * 00045 ****************************************************************************** 00046 * @attention 00047 * 00048 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00049 * 00050 * Redistribution and use in source and binary forms, with or without modification, 00051 * are permitted provided that the following conditions are met: 00052 * 1. Redistributions of source code must retain the above copyright notice, 00053 * this list of conditions and the following disclaimer. 00054 * 2. Redistributions in binary form must reproduce the above copyright notice, 00055 * this list of conditions and the following disclaimer in the documentation 00056 * and/or other materials provided with the distribution. 00057 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00058 * may be used to endorse or promote products derived from this software 00059 * without specific prior written permission. 00060 * 00061 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00062 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00063 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00064 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00065 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00066 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00067 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00068 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00069 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00070 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00071 * 00072 ****************************************************************************** 00073 */ 00074 /* Includes ------------------------------------------------------------------*/ 00075 #include "stm324xg_eval_eeprom.h" 00076 00077 /** @addtogroup BSP 00078 * @{ 00079 */ 00080 00081 /** @addtogroup STM324xG_EVAL 00082 * @{ 00083 */ 00084 00085 /** @defgroup STM324xG_EVAL_EEPROM STM324xG EVAL EEPROM 00086 * @brief This file includes the I2C EEPROM driver of STM32F4xG-EVAL evaluation board. 00087 * @{ 00088 */ 00089 00090 /** @defgroup STM324xG_EVAL_EEPROM_Private_Types STM324xG EVAL EEPROM Private Types 00091 * @{ 00092 */ 00093 /** 00094 * @} 00095 */ 00096 00097 /** @defgroup STM324xG_EVAL_EEPROM_Private_Defines STM324xG EVAL EEPROM Private Defines 00098 * @{ 00099 */ 00100 /** 00101 * @} 00102 */ 00103 00104 /** @defgroup STM324xG_EVAL_EEPROM_Private_Macros STM324xG EVAL EEPROM Private Macros 00105 * @{ 00106 */ 00107 /** 00108 * @} 00109 */ 00110 00111 /** @defgroup STM324xG_EVAL_EEPROM_Private_Variables STM324xG EVAL EEPROM Private Variables 00112 * @{ 00113 */ 00114 __IO uint32_t EEPROMTimeout = EEPROM_READ_TIMEOUT; 00115 __IO uint16_t EEPROMDataRead; 00116 __IO uint8_t EEPROMDataWrite; 00117 /** 00118 * @} 00119 */ 00120 00121 /** @defgroup STM324xG_EVAL_EEPROM_Private_Function_Prototypes STM324xG EVAL EEPROM Private Function Prototypes 00122 * @{ 00123 */ 00124 static uint32_t EEPROM_WritePage(uint8_t* pBuffer, uint16_t WriteAddr, uint8_t* NumByteToWrite); 00125 static uint32_t EEPROM_WaitEepromStandbyState(void); 00126 /** 00127 * @} 00128 */ 00129 00130 /** @defgroup STM324xG_EVAL_EEPROM_Private_Functions STM324xG EVAL EEPROM Private Functions 00131 * @{ 00132 */ 00133 00134 /** 00135 * @brief Initializes peripherals used by the I2C EEPROM driver. 00136 * @retval EEPROM_OK (0) if operation is correctly performed, else return value 00137 * different from EEPROM_OK (0) 00138 */ 00139 uint32_t BSP_EEPROM_Init(void) 00140 { 00141 /* I2C Initialization */ 00142 EEPROM_IO_Init(); 00143 00144 /* Select the EEPROM address and check if OK */ 00145 if(EEPROM_IO_IsDeviceReady(EEPROM_I2C_ADDRESS, EEPROM_MAX_TRIALS) != HAL_OK) 00146 { 00147 return EEPROM_FAIL; 00148 } 00149 return EEPROM_OK; 00150 } 00151 00152 /** 00153 * @brief Reads a block of data from the EEPROM. 00154 * @param pBuffer: pointer to the buffer that receives the data read from 00155 * the EEPROM. 00156 * @param ReadAddr: EEPROM's internal address to start reading from. 00157 * @param NumByteToRead: pointer to the variable holding number of bytes to 00158 * be read from the EEPROM. 00159 * 00160 * @note The variable pointed by NumByteToRead is reset to 0 when all the 00161 * data are read from the EEPROM. Application should monitor this 00162 * variable in order know when the transfer is complete. 00163 * 00164 * @retval EEPROM_OK (0) if operation is correctly performed, else return value 00165 * different from EEPROM_OK (0) or the timeout user callback. 00166 */ 00167 uint32_t BSP_EEPROM_ReadBuffer(uint8_t* pBuffer, uint16_t ReadAddr, uint16_t* NumByteToRead) 00168 { 00169 uint32_t buffersize = *NumByteToRead; 00170 00171 /* Set the pointer to the Number of data to be read */ 00172 EEPROMDataRead = *NumByteToRead; 00173 00174 if(EEPROM_IO_ReadData(EEPROM_I2C_ADDRESS, ReadAddr, pBuffer, buffersize) != HAL_OK) 00175 { 00176 BSP_EEPROM_TIMEOUT_UserCallback(); 00177 return EEPROM_FAIL; 00178 } 00179 00180 /* If all operations OK, return EEPROM_OK (0) */ 00181 return EEPROM_OK; 00182 } 00183 00184 /** 00185 * @brief Writes buffer of data to the I2C EEPROM. 00186 * @param pBuffer: pointer to the buffer containing the data to be written 00187 * to the EEPROM. 00188 * @param WriteAddr: EEPROM's internal address to write to. 00189 * @param NumByteToWrite: number of bytes to write to the EEPROM. 00190 * @retval EEPROM_OK (0) if operation is correctly performed, else return value 00191 * different from EEPROM_OK (0) or the timeout user callback. 00192 */ 00193 uint32_t BSP_EEPROM_WriteBuffer(uint8_t* pBuffer, uint16_t WriteAddr, uint16_t NumByteToWrite) 00194 { 00195 uint8_t numofpage = 0, numofsingle = 0, count = 0; 00196 uint16_t addr = 0; 00197 uint8_t dataindex = 0; 00198 uint32_t status = EEPROM_OK; 00199 00200 addr = WriteAddr % EEPROM_PAGESIZE; 00201 count = EEPROM_PAGESIZE - addr; 00202 numofpage = NumByteToWrite / EEPROM_PAGESIZE; 00203 numofsingle = NumByteToWrite % EEPROM_PAGESIZE; 00204 00205 /* If WriteAddr is EEPROM_PAGESIZE aligned */ 00206 if(addr == 0) 00207 { 00208 /* If NumByteToWrite < EEPROM_PAGESIZE */ 00209 if(numofpage == 0) 00210 { 00211 /* Store the number of data to be written */ 00212 dataindex = numofsingle; 00213 /* Start writing data */ 00214 status = EEPROM_WritePage(pBuffer, WriteAddr, (uint8_t*)(&dataindex)); 00215 if(status != EEPROM_OK) 00216 { 00217 return status; 00218 } 00219 } 00220 /* If NumByteToWrite > EEPROM_PAGESIZE */ 00221 else 00222 { 00223 while(numofpage--) 00224 { 00225 /* Store the number of data to be written */ 00226 dataindex = EEPROM_PAGESIZE; 00227 status = EEPROM_WritePage(pBuffer, WriteAddr, (uint8_t*)(&dataindex)); 00228 if(status != EEPROM_OK) 00229 { 00230 return status; 00231 } 00232 00233 WriteAddr += EEPROM_PAGESIZE; 00234 pBuffer += EEPROM_PAGESIZE; 00235 } 00236 00237 if(numofsingle!=0) 00238 { 00239 /* Store the number of data to be written */ 00240 dataindex = numofsingle; 00241 status = EEPROM_WritePage(pBuffer, WriteAddr, (uint8_t*)(&dataindex)); 00242 if(status != EEPROM_OK) 00243 { 00244 return status; 00245 } 00246 } 00247 } 00248 } 00249 /* If WriteAddr is not EEPROM_PAGESIZE aligned */ 00250 else 00251 { 00252 /* If NumByteToWrite < EEPROM_PAGESIZE */ 00253 if(numofpage == 0) 00254 { 00255 /* If the number of data to be written is more than the remaining space 00256 in the current page: */ 00257 if(NumByteToWrite > count) 00258 { 00259 /* Store the number of data to be written */ 00260 dataindex = count; 00261 /* Write the data contained in same page */ 00262 status = EEPROM_WritePage(pBuffer, WriteAddr, (uint8_t*)(&dataindex)); 00263 if(status != EEPROM_OK) 00264 { 00265 return status; 00266 } 00267 00268 /* Store the number of data to be written */ 00269 dataindex = (NumByteToWrite - count); 00270 /* Write the remaining data in the following page */ 00271 status = EEPROM_WritePage((uint8_t*)(pBuffer + count), (WriteAddr + count), (uint8_t*)(&dataindex)); 00272 if(status != EEPROM_OK) 00273 { 00274 return status; 00275 } 00276 } 00277 else 00278 { 00279 /* Store the number of data to be written */ 00280 dataindex = numofsingle; 00281 status = EEPROM_WritePage(pBuffer, WriteAddr, (uint8_t*)(&dataindex)); 00282 if(status != EEPROM_OK) 00283 { 00284 return status; 00285 } 00286 } 00287 } 00288 /* If NumByteToWrite > EEPROM_PAGESIZE */ 00289 else 00290 { 00291 NumByteToWrite -= count; 00292 numofpage = NumByteToWrite / EEPROM_PAGESIZE; 00293 numofsingle = NumByteToWrite % EEPROM_PAGESIZE; 00294 00295 if(count != 0) 00296 { 00297 /* Store the number of data to be written */ 00298 dataindex = count; 00299 status = EEPROM_WritePage(pBuffer, WriteAddr, (uint8_t*)(&dataindex)); 00300 if(status != EEPROM_OK) 00301 { 00302 return status; 00303 } 00304 WriteAddr += count; 00305 pBuffer += count; 00306 } 00307 00308 while(numofpage--) 00309 { 00310 /* Store the number of data to be written */ 00311 dataindex = EEPROM_PAGESIZE; 00312 status = EEPROM_WritePage(pBuffer, WriteAddr, (uint8_t*)(&dataindex)); 00313 if(status != EEPROM_OK) 00314 { 00315 return status; 00316 } 00317 WriteAddr += EEPROM_PAGESIZE; 00318 pBuffer += EEPROM_PAGESIZE; 00319 } 00320 if(numofsingle != 0) 00321 { 00322 /* Store the number of data to be written */ 00323 dataindex = numofsingle; 00324 status = EEPROM_WritePage(pBuffer, WriteAddr, (uint8_t*)(&dataindex)); 00325 if(status != EEPROM_OK) 00326 { 00327 return status; 00328 } 00329 } 00330 } 00331 } 00332 00333 /* If all operations OK, return EEPROM_OK (0) */ 00334 return EEPROM_OK; 00335 } 00336 00337 /** 00338 * @brief Writes more than one byte to the EEPROM with a single WRITE cycle. 00339 * 00340 * @note The number of bytes (combined to write start address) must not 00341 * cross the EEPROM page boundary. This function can only write into 00342 * the boundaries of an EEPROM page. 00343 * This function doesn't check on boundaries condition (in this driver 00344 * the function BSP_EEPROM_WriteBuffer() which calls EEPROM_WritePage() is 00345 * responsible of checking on Page boundaries). 00346 * 00347 * @param pBuffer: pointer to the buffer containing the data to be written to 00348 * the EEPROM. 00349 * @param WriteAddr: EEPROM's internal address to write to. 00350 * @param NumByteToWrite: pointer to the variable holding number of bytes to 00351 * be written into the EEPROM. 00352 * 00353 * @note The variable pointed by NumByteToWrite is reset to 0 when all the 00354 * data are written to the EEPROM. Application should monitor this 00355 * variable in order know when the transfer is complete. 00356 * 00357 * @retval EEPROM_OK (0) if operation is correctly performed, else return value 00358 * different from EEPROM_OK (0) or the timeout user callback. 00359 */ 00360 static uint32_t EEPROM_WritePage(uint8_t* pBuffer, uint16_t WriteAddr, uint8_t* NumByteToWrite) 00361 { 00362 uint32_t buffersize = *NumByteToWrite; 00363 uint32_t status = EEPROM_OK; 00364 00365 /* Set the pointer to the Number of data to be written */ 00366 EEPROMDataWrite = *NumByteToWrite; 00367 if(EEPROM_IO_WriteData(EEPROM_I2C_ADDRESS, WriteAddr, pBuffer, buffersize) != HAL_OK) 00368 { 00369 BSP_EEPROM_TIMEOUT_UserCallback(); 00370 status = EEPROM_FAIL; 00371 } 00372 00373 while(EEPROM_WaitEepromStandbyState() != EEPROM_OK) 00374 { 00375 return EEPROM_FAIL; 00376 } 00377 00378 /* If all operations OK, return EEPROM_OK (0) */ 00379 return status; 00380 } 00381 00382 /** 00383 * @brief Waits for EEPROM Standby state. 00384 * 00385 * @note This function allows to wait and check that EEPROM has finished the 00386 * last operation. It is mostly used after Write operation: after receiving 00387 * the buffer to be written, the EEPROM may need additional time to actually 00388 * perform the write operation. During this time, it doesn't answer to 00389 * I2C packets addressed to it. Once the write operation is complete 00390 * the EEPROM responds to its address. 00391 * 00392 * @retval EEPROM_OK (0) if operation is correctly performed, else return value 00393 * different from EEPROM_OK (0) or the timeout user callback. 00394 */ 00395 static uint32_t EEPROM_WaitEepromStandbyState(void) 00396 { 00397 /* Check if the maximum allowed number of trials has bee reached */ 00398 if(EEPROM_IO_IsDeviceReady(EEPROM_I2C_ADDRESS, EEPROM_MAX_TRIALS) != HAL_OK) 00399 { 00400 /* If the maximum number of trials has been reached, exit the function */ 00401 BSP_EEPROM_TIMEOUT_UserCallback(); 00402 return EEPROM_TIMEOUT; 00403 } 00404 return EEPROM_OK; 00405 } 00406 00407 /** 00408 * @brief Basic management of the timeout situation. 00409 */ 00410 __weak void BSP_EEPROM_TIMEOUT_UserCallback(void) 00411 { 00412 } 00413 00414 /** 00415 * @} 00416 */ 00417 00418 /** 00419 * @} 00420 */ 00421 00422 /** 00423 * @} 00424 */ 00425 00426 /** 00427 * @} 00428 */ 00429 00430 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Fri Jan 15 2016 14:22:29 for STM324xG_EVAL BSP User Manual by
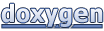