STM324xG_EVAL BSP User Manual
|
stm324xg_eval_audio.h
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm324xg_eval_audio.h 00004 * @author MCD Application Team 00005 * @version V2.2.1 00006 * @date 15-January-2016 00007 * @brief This file contains the common defines and functions prototypes for 00008 * the stm324xg_eval_audio.c driver. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* Define to prevent recursive inclusion -------------------------------------*/ 00040 #ifndef __STM324xG_EVAL_AUDIO_H 00041 #define __STM324xG_EVAL_AUDIO_H 00042 00043 #ifdef __cplusplus 00044 extern "C" { 00045 #endif 00046 00047 /* Includes ------------------------------------------------------------------*/ 00048 #include "../Components/cs43l22/cs43l22.h" 00049 #include "stm324xg_eval.h" 00050 00051 /** @addtogroup BSP 00052 * @{ 00053 */ 00054 00055 /** @addtogroup STM324xG_EVAL 00056 * @{ 00057 */ 00058 00059 /** @addtogroup STM324xG_EVAL_AUDIO 00060 * @{ 00061 */ 00062 00063 /** @defgroup STM324xG_EVAL_AUDIO_Exported_Types STM324xG EVAL AUDIO Exported Types 00064 * @{ 00065 */ 00066 /** 00067 * @} 00068 */ 00069 00070 /** @defgroup STM324xG_EVAL_AUDIO_Exported_Constants STM324xG EVAL AUDIO Exported Constants 00071 * @{ 00072 */ 00073 /* Audio Reset Pin definition */ 00074 #define AUDIO_RESET_PIN IO_PIN_2 00075 00076 /* I2S peripheral configuration defines */ 00077 #define AUDIO_I2Sx SPI2 00078 #define AUDIO_I2Sx_CLK_ENABLE() __SPI2_CLK_ENABLE() 00079 #define AUDIO_I2Sx_SCK_SD_WS_AF GPIO_AF5_SPI2 00080 #define AUDIO_I2Sx_SCK_SD_WS_CLK_ENABLE() __GPIOI_CLK_ENABLE() 00081 #define AUDIO_I2Sx_MCK_CLK_ENABLE() __GPIOC_CLK_ENABLE() 00082 #define AUDIO_I2Sx_WS_PIN GPIO_PIN_0 00083 #define AUDIO_I2Sx_SCK_PIN GPIO_PIN_1 00084 #define AUDIO_I2Sx_SD_PIN GPIO_PIN_3 00085 #define AUDIO_I2Sx_MCK_PIN GPIO_PIN_6 00086 #define AUDIO_I2Sx_SCK_SD_WS_GPIO_PORT GPIOI 00087 #define AUDIO_I2Sx_MCK_GPIO_PORT GPIOC 00088 00089 /* I2S DMA Stream definitions */ 00090 #define AUDIO_I2Sx_DMAx_CLK_ENABLE() __DMA1_CLK_ENABLE() 00091 #define AUDIO_I2Sx_DMAx_STREAM DMA1_Stream4 00092 #define AUDIO_I2Sx_DMAx_CHANNEL DMA_CHANNEL_0 00093 #define AUDIO_I2Sx_DMAx_IRQ DMA1_Stream4_IRQn 00094 #define AUDIO_I2Sx_DMAx_PERIPH_DATA_SIZE DMA_PDATAALIGN_HALFWORD 00095 #define AUDIO_I2Sx_DMAx_MEM_DATA_SIZE DMA_MDATAALIGN_HALFWORD 00096 #define DMA_MAX_SZE 0xFFFF 00097 00098 #define AUDIO_I2Sx_DMAx_IRQHandler DMA1_Stream4_IRQHandler 00099 00100 /*------------------------------------------------------------------------------ 00101 CONFIGURATION: Audio Driver Configuration parameters 00102 ------------------------------------------------------------------------------*/ 00103 /* Select the interrupt preemption priority for the DMA interrupt */ 00104 #define AUDIO_IRQ_PREPRIO 5 /* Select the preemption priority level(0 is the highest) */ 00105 00106 #define AUDIODATA_SIZE 2 /* 16-bits audio data size */ 00107 00108 /* Audio status definition */ 00109 #define AUDIO_OK 0x00 00110 #define AUDIO_ERROR 0x01 00111 #define AUDIO_TIMEOUT 0x02 00112 00113 /*------------------------------------------------------------------------------ 00114 OPTIONAL Configuration defines parameters 00115 ------------------------------------------------------------------------------*/ 00116 /* Delay for the Codec to be correctly reset */ 00117 #define CODEC_RESET_DELAY 5 00118 00119 /** 00120 * @} 00121 */ 00122 00123 /** @defgroup STM324xG_EVAL_AUDIO_Exported_Macros STM324xG EVAL AUDIO Exported Macros 00124 * @{ 00125 */ 00126 #define DMA_MAX(x) (((x) <= DMA_MAX_SZE)? (x):DMA_MAX_SZE) 00127 /** 00128 * @} 00129 */ 00130 00131 /** @defgroup STM324xG_EVAL_AUDIO_Exported_Functions STM324xG EVAL AUDIO Exported Functions 00132 * @{ 00133 */ 00134 uint8_t BSP_AUDIO_OUT_Init(uint16_t OutputDevice, uint8_t Volume, uint32_t AudioFreq); 00135 uint8_t BSP_AUDIO_OUT_Play(uint16_t *pBuffer, uint32_t Size); 00136 void BSP_AUDIO_OUT_ChangeBuffer(uint16_t *pData, uint16_t Size); 00137 uint8_t BSP_AUDIO_OUT_Pause(void); 00138 uint8_t BSP_AUDIO_OUT_Resume(void); 00139 uint8_t BSP_AUDIO_OUT_Stop(uint32_t Option); 00140 uint8_t BSP_AUDIO_OUT_SetVolume(uint8_t Volume); 00141 void BSP_AUDIO_OUT_SetFrequency(uint32_t AudioFreq); 00142 uint8_t BSP_AUDIO_OUT_SetMute(uint32_t Cmd); 00143 uint8_t BSP_AUDIO_OUT_SetOutputMode(uint8_t Output); 00144 00145 /* User Callbacks: user has to implement these functions in his code if they are needed. */ 00146 /* This function is called when the requested data has been completely transferred.*/ 00147 void BSP_AUDIO_OUT_TransferComplete_CallBack(void); 00148 00149 /* This function is called when half of the requested buffer has been transferred. */ 00150 void BSP_AUDIO_OUT_HalfTransfer_CallBack(void); 00151 00152 /* This function is called when an Interrupt due to transfer error on or peripheral 00153 error occurs. */ 00154 void BSP_AUDIO_OUT_Error_CallBack(void); 00155 00156 /** 00157 * @} 00158 */ 00159 00160 /** 00161 * @} 00162 */ 00163 00164 /** 00165 * @} 00166 */ 00167 00168 /** 00169 * @} 00170 */ 00171 00172 #ifdef __cplusplus 00173 } 00174 #endif 00175 00176 #endif /* __STM324xG_EVAL_AUDIO_H */ 00177 00178 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Fri Jan 15 2016 14:22:29 for STM324xG_EVAL BSP User Manual by
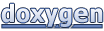