STM324xG_EVAL BSP User Manual
|
stm324xg_eval_audio.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm324xg_eval_audio.c 00004 * @author MCD Application Team 00005 * @version V2.2.1 00006 * @date 15-January-2016 00007 * @brief This file provides the Audio driver for the STM324xG-EVAL evaluation 00008 * board. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /*============================================================================== 00040 User NOTES 00041 How To use this driver: 00042 ----------------------- 00043 + This driver supports STM32F4xx devices on STM324xG-EVAL Evaluation board. 00044 + Call the function BSP_AUDIO_OUT_Init( 00045 OutputDevice: physical output mode (OUTPUT_DEVICE_SPEAKER, 00046 OUTPUT_DEVICE_HEADPHONE, OUTPUT_DEVICE_AUTO or 00047 OUTPUT_DEVICE_BOTH) 00048 Volume: initial volume to be set (0 is min (mute), 100 is max (100%) 00049 AudioFreq: Audio frequency in Hz (8000, 16000, 22500, 32000 ...) 00050 this parameter is relative to the audio file/stream type. 00051 ) 00052 This function configures all the hardware required for the audio application (codec, I2C, I2S, 00053 GPIOs, DMA and interrupt if needed). This function returns 0 if configuration is OK. 00054 If the returned value is different from 0 or the function is stuck then the communication with 00055 the codec or the IOExpander has failed (try to un-plug the power or reset device in this case). 00056 - OUTPUT_DEVICE_SPEAKER: only speaker will be set as output for the audio stream. 00057 - OUTPUT_DEVICE_HEADPHONE: only headphones will be set as output for the audio stream. 00058 - OUTPUT_DEVICE_AUTO: Selection of output device is made through external switch (implemented 00059 into the audio jack on the evaluation board). When the Headphone is connected it is used 00060 as output. When the headphone is disconnected from the audio jack, the output is 00061 automatically switched to Speaker. 00062 - OUTPUT_DEVICE_BOTH: both Speaker and Headphone are used as outputs for the audio stream 00063 at the same time. 00064 + Call the function BSP_AUDIO_OUT_Play( 00065 pBuffer: pointer to the audio data file address 00066 Size: size of the buffer to be sent in Bytes 00067 ) 00068 to start playing (for the first time) from the audio file/stream. 00069 + Call the function BSP_AUDIO_OUT_Pause() to pause playing 00070 + Call the function BSP_AUDIO_OUT_Resume() to resume playing. 00071 Note. After calling BSP_AUDIO_OUT_Pause() function for pause, only BSP_AUDIO_OUT_Resume() should be called 00072 for resume (it is not allowed to call BSP_AUDIO_OUT_Play() in this case). 00073 Note. This function should be called only when the audio file is played or paused (not stopped). 00074 + For each mode, you may need to implement the relative callback functions into your code. 00075 The Callback functions are named AUDIO_OUT_XXX_CallBack() and only their prototypes are declared in 00076 the stm324xg_eval_audio.h file. (refer to the example for more details on the callbacks implementations) 00077 + To Stop playing, to modify the volume level or to mute, use the functions 00078 BSP_AUDIO_OUT_Stop(), BSP_AUDIO_OUT_SetVolume(), AUDIO_OUT_SetFrequency() BSP_AUDIO_OUT_SetOutputMode and BSP_AUDIO_OUT_SetMute(). 00079 + The driver API and the callback functions are at the end of the stm324xg_eval_audio.h file. 00080 00081 Driver architecture: 00082 ------------------- 00083 + This driver provide the High Audio Layer: consists of the function API exported in the stm324xg_eval_audio.h file 00084 (BSP_AUDIO_OUT_Init(), BSP_AUDIO_OUT_Play() ...) 00085 + This driver provide also the Media Access Layer (MAL): which consists of functions allowing to access the media containing/ 00086 providing the audio file/stream. These functions are also included as local functions into 00087 the stm324xg_eval_audio_codec.c file (I2Sx_MspInit() and I2Sx_Init()) 00088 00089 Known Limitations: 00090 ------------------- 00091 1- When using the Speaker, if the audio file quality is not high enough, the speaker output 00092 may produce high and uncomfortable noise level. To avoid this issue, to use speaker 00093 output properly, try to increase audio file sampling rate (typically higher than 48KHz). 00094 This operation will lead to larger file size. 00095 2- Communication with the audio codec (through I2C) may be corrupted if it is interrupted by some 00096 user interrupt routines (in this case, interrupts could be disabled just before the start of 00097 communication then re-enabled when it is over). Note that this communication is only done at 00098 the configuration phase (BSP_AUDIO_OUT_Init() or BSP_AUDIO_OUT_Stop()) and when Volume control modification is 00099 performed (BSP_AUDIO_OUT_SetVolume() or AUDIO_OUT_Mute() or BSP_AUDIO_OUT_SetOutputMode()). 00100 When the audio data is played, no communication is required with the audio codec. 00101 3- Parsing of audio file is not implemented (in order to determine audio file properties: Mono/Stereo, Data size, 00102 File size, Audio Frequency, Audio Data header size ...). The configuration is fixed for the given audio file. 00103 4- Supports only Stereo audio streaming. To play mono audio streams, each data should be sent twice 00104 on the I2S or should be duplicated on the source buffer. Or convert the stream in stereo before playing. 00105 5- Supports only 16-bits audio data size. 00106 ==============================================================================*/ 00107 00108 /* Includes ------------------------------------------------------------------*/ 00109 #include "stm324xg_eval_audio.h" 00110 #include "stm324xg_eval_io.h" /* IOExpander driver is included in order to allow 00111 CS43L22 codec reset pin managment on the evaluation board */ 00112 /** @addtogroup BSP 00113 * @{ 00114 */ 00115 00116 /** @addtogroup STM324xG_EVAL 00117 * @{ 00118 */ 00119 00120 /** @defgroup STM324xG_EVAL_AUDIO STM324xG EVAL AUDIO 00121 * @brief This file includes the low layer audio driver available on STM324xG-EVAL 00122 * evaluation board. 00123 * @{ 00124 */ 00125 00126 /** @defgroup STM324xG_EVAL_AUDIO_Private_Types STM324xG EVAL AUDIO Private Types 00127 * @{ 00128 */ 00129 /** 00130 * @} 00131 */ 00132 00133 /** @defgroup STM324xG_EVAL_AUDIO_Private_Defines STM324xG EVAL AUDIO Private Defines 00134 * @{ 00135 */ 00136 /* These PLL parameters are valide when the f(VCO clock) = 1Mhz */ 00137 const uint32_t I2SFreq[8] = {8000, 11025, 16000, 22050, 32000, 44100, 48000, 96000}; 00138 const uint32_t I2SPLLN[8] = {256, 429, 213, 429, 426, 271, 258, 344}; 00139 const uint32_t I2SPLLR[8] = {5, 4, 4, 4, 4, 6, 3, 1}; 00140 /** 00141 * @} 00142 */ 00143 00144 /** @defgroup STM324xG_EVAL_AUDIO_Private_Macros STM324xG EVAL AUDIO Private Macros 00145 * @{ 00146 */ 00147 /** 00148 * @} 00149 */ 00150 00151 /** @defgroup STM324xG_EVAL_AUDIO_Private_Variables STM324xG EVAL AUDIO Private Variables 00152 * @{ 00153 */ 00154 AUDIO_DrvTypeDef *audio_drv; 00155 I2S_HandleTypeDef haudio_i2s; 00156 /** 00157 * @} 00158 */ 00159 00160 /** @defgroup STM324xG_EVAL_AUDIO_Private_Function_Prototypes STM324xG EVAL AUDIO Private Function Prototypes 00161 * @{ 00162 */ 00163 static void CODEC_Reset(void); 00164 static void I2Sx_MspInit(void); 00165 static void I2Sx_Init(uint32_t AudioFreq); 00166 /** 00167 * @} 00168 */ 00169 00170 /** @defgroup STM324xG_EVAL_AUDIO_Private_Functions STM324xG EVAL AUDIO Private Functions 00171 * @{ 00172 */ 00173 00174 /** 00175 * @brief Configures the audio peripherals. 00176 * @param OutputDevice: OUTPUT_DEVICE_SPEAKER, OUTPUT_DEVICE_HEADPHONE, 00177 * OUTPUT_DEVICE_BOTH or OUTPUT_DEVICE_AUTO . 00178 * @param Volume: Initial volume level (from 0 (Mute) to 100 (Max)) 00179 * @param AudioFreq: Audio frequency used to play the audio stream. 00180 * @note This function configure also that the I2S PLL input clock. 00181 * @retval 0 if correct communication, else wrong communication 00182 */ 00183 uint8_t BSP_AUDIO_OUT_Init(uint16_t OutputDevice, uint8_t Volume, uint32_t AudioFreq) 00184 { 00185 uint8_t ret = AUDIO_ERROR; 00186 uint32_t deviceid = 0x00; 00187 RCC_PeriphCLKInitTypeDef RCC_ExCLKInitStruct; 00188 uint8_t index = 0, freqindex = 0xFF; 00189 00190 for(index = 0; index < 8; index++) 00191 { 00192 if(I2SFreq[index] == AudioFreq) 00193 { 00194 freqindex = index; 00195 } 00196 } 00197 HAL_RCCEx_GetPeriphCLKConfig(&RCC_ExCLKInitStruct); 00198 if(freqindex != 0xFF) 00199 { 00200 /* I2S clock config 00201 PLLI2S_VCO = f(VCO clock) = f(PLLI2S clock input) � (PLLI2SN/PLLM) 00202 I2SCLK = f(PLLI2S clock output) = f(VCO clock) / PLLI2SR */ 00203 RCC_ExCLKInitStruct.PeriphClockSelection = RCC_PERIPHCLK_I2S; 00204 RCC_ExCLKInitStruct.PLLI2S.PLLI2SN = I2SPLLN[freqindex]; 00205 RCC_ExCLKInitStruct.PLLI2S.PLLI2SR = I2SPLLR[freqindex]; 00206 HAL_RCCEx_PeriphCLKConfig(&RCC_ExCLKInitStruct); 00207 } 00208 else /* Default PLL I2S configuration */ 00209 { 00210 /* I2S clock config 00211 PLLI2S_VCO = f(VCO clock) = f(PLLI2S clock input) � (PLLI2SN/PLLM) 00212 I2SCLK = f(PLLI2S clock output) = f(VCO clock) / PLLI2SR */ 00213 RCC_ExCLKInitStruct.PeriphClockSelection = RCC_PERIPHCLK_I2S; 00214 RCC_ExCLKInitStruct.PLLI2S.PLLI2SN = 258; 00215 RCC_ExCLKInitStruct.PLLI2S.PLLI2SR = 3; 00216 HAL_RCCEx_PeriphCLKConfig(&RCC_ExCLKInitStruct); 00217 } 00218 00219 /* Reset the Codec Registers */ 00220 CODEC_Reset(); 00221 00222 deviceid = cs43l22_drv.ReadID(AUDIO_I2C_ADDRESS); 00223 00224 if((deviceid & CS43L22_ID_MASK) == CS43L22_ID) 00225 { 00226 /* Initialize the audio driver structure */ 00227 audio_drv = &cs43l22_drv; 00228 ret = AUDIO_OK; 00229 } 00230 else 00231 { 00232 ret = AUDIO_ERROR; 00233 } 00234 00235 if(ret == AUDIO_OK) 00236 { 00237 audio_drv->Init(AUDIO_I2C_ADDRESS, OutputDevice, Volume, AudioFreq); 00238 /* I2S data transfer preparation: 00239 Prepare the Media to be used for the audio transfer from memory to I2S peripheral */ 00240 /* Configure the I2S peripheral */ 00241 I2Sx_Init(AudioFreq); 00242 } 00243 00244 return ret; 00245 } 00246 00247 /** 00248 * @brief Starts playing audio stream from a data buffer for a determined size. 00249 * @param pBuffer: Pointer to the buffer 00250 * @param Size: Number of audio data BYTES. 00251 * @retval AUDIO_OK if correct communication, else wrong communication 00252 */ 00253 uint8_t BSP_AUDIO_OUT_Play(uint16_t* pBuffer, uint32_t Size) 00254 { 00255 /* Call the audio Codec Play function */ 00256 if(audio_drv->Play(AUDIO_I2C_ADDRESS, pBuffer, Size) != 0) 00257 { 00258 return AUDIO_ERROR; 00259 } 00260 else 00261 { 00262 /* Update the Media layer and enable it for play */ 00263 HAL_I2S_Transmit_DMA(&haudio_i2s, pBuffer, DMA_MAX(Size/AUDIODATA_SIZE)); 00264 return AUDIO_OK; 00265 } 00266 } 00267 00268 /** 00269 * @brief Sends n-Bytes on the I2S interface. 00270 * @param pData: Pointer to data address 00271 * @param Size: Number of data to be written. 00272 */ 00273 void BSP_AUDIO_OUT_ChangeBuffer(uint16_t *pData, uint16_t Size) 00274 { 00275 HAL_I2S_Transmit_DMA(&haudio_i2s, pData, Size); 00276 } 00277 00278 /** 00279 * @brief Pauses the audio file stream. 00280 * In case of using DMA, the DMA Pause feature is used. 00281 * WARNING: When calling BSP_AUDIO_OUT_Pause() function for pause, only 00282 * BSP_AUDIO_OUT_Resume() function should be called for resume (use of BSP_AUDIO_OUT_Play() 00283 * function for resume could lead to unexpected behavior). 00284 * @retval AUDIO_OK if correct communication, else wrong communication 00285 */ 00286 uint8_t BSP_AUDIO_OUT_Pause(void) 00287 { 00288 /* Call the Audio Codec Pause/Resume function */ 00289 if(audio_drv->Pause(AUDIO_I2C_ADDRESS) != 0) 00290 { 00291 return AUDIO_ERROR; 00292 } 00293 else 00294 { 00295 /* Call the Media layer pause function */ 00296 HAL_I2S_DMAPause(&haudio_i2s); 00297 00298 /* Return AUDIO_OK when all operations are correctly done */ 00299 return AUDIO_OK; 00300 } 00301 } 00302 00303 /** 00304 * @brief Resumes the audio file stream. 00305 * WARNING: When calling BSP_AUDIO_OUT_Pause() function for pause, only 00306 * BSP_AUDIO_OUT_Resume() function should be called for resume (use of BSP_AUDIO_OUT_Play() 00307 * function for resume could lead to unexpected behavior). 00308 * @retval AUDIO_OK if correct communication, else wrong communication 00309 */ 00310 uint8_t BSP_AUDIO_OUT_Resume(void) 00311 { 00312 /* Call the Audio Codec Pause/Resume function */ 00313 if(audio_drv->Resume(AUDIO_I2C_ADDRESS) != 0) 00314 { 00315 return AUDIO_ERROR; 00316 } 00317 else 00318 { 00319 /* Call the Media layer pause/resume function */ 00320 HAL_I2S_DMAResume(&haudio_i2s); 00321 00322 /* Return AUDIO_OK when all operations are correctly done */ 00323 return AUDIO_OK; 00324 } 00325 } 00326 00327 /** 00328 * @brief Stops audio playing and Power down the Audio Codec. 00329 * @param Option: could be one of the following parameters 00330 * - CODEC_PDWN_SW: for software power off (by writing registers). 00331 * Then no need to reconfigure the Codec after power on. 00332 * - CODEC_PDWN_HW: completely shut down the codec (physically). 00333 * Then need to reconfigure the Codec after power on. 00334 * @retval AUDIO_OK if correct communication, else wrong communication 00335 */ 00336 uint8_t BSP_AUDIO_OUT_Stop(uint32_t Option) 00337 { 00338 /* Call the Media layer stop function */ 00339 HAL_I2S_DMAStop(&haudio_i2s); 00340 00341 /* Call Audio Codec Stop function */ 00342 if(audio_drv->Stop(AUDIO_I2C_ADDRESS, Option) != 0) 00343 { 00344 return AUDIO_ERROR; 00345 } 00346 else 00347 { 00348 if(Option == CODEC_PDWN_HW) 00349 { 00350 /* Wait at least 1ms */ 00351 HAL_Delay(1); 00352 00353 /* Reset the pin */ 00354 BSP_IO_WritePin(AUDIO_RESET_PIN, RESET); 00355 } 00356 00357 /* Return AUDIO_OK when all operations are correctly done */ 00358 return AUDIO_OK; 00359 } 00360 } 00361 00362 /** 00363 * @brief Controls the current audio volume level. 00364 * @param Volume: Volume level to be set in percentage from 0% to 100% (0 for 00365 * Mute and 100 for Max volume level). 00366 * @retval AUDIO_OK if correct communication, else wrong communication 00367 */ 00368 uint8_t BSP_AUDIO_OUT_SetVolume(uint8_t Volume) 00369 { 00370 /* Call the codec volume control function with converted volume value */ 00371 if(audio_drv->SetVolume(AUDIO_I2C_ADDRESS, Volume) != 0) 00372 { 00373 return AUDIO_ERROR; 00374 } 00375 else 00376 { 00377 /* Return AUDIO_OK when all operations are correctly done */ 00378 return AUDIO_OK; 00379 } 00380 } 00381 00382 /** 00383 * @brief Enables or disables the MUTE mode by software 00384 * @param Cmd: could be AUDIO_MUTE_ON to mute sound or AUDIO_MUTE_OFF to 00385 * unmute the codec and restore previous volume level. 00386 * @retval AUDIO_OK if correct communication, else wrong communication 00387 */ 00388 uint8_t BSP_AUDIO_OUT_SetMute(uint32_t Cmd) 00389 { 00390 /* Call the Codec Mute function */ 00391 if(audio_drv->SetMute(AUDIO_I2C_ADDRESS, Cmd) != 0) 00392 { 00393 return AUDIO_ERROR; 00394 } 00395 else 00396 { 00397 /* Return AUDIO_OK when all operations are correctly done */ 00398 return AUDIO_OK; 00399 } 00400 } 00401 00402 /** 00403 * @brief Switch dynamically (while audio file is played) the output target 00404 * (speaker or headphone). 00405 * @note This function modifies a global variable of the audio codec driver: OutputDev. 00406 * @param Output: specifies the audio output target: OUTPUT_DEVICE_SPEAKER, 00407 * OUTPUT_DEVICE_HEADPHONE, OUTPUT_DEVICE_BOTH or OUTPUT_DEVICE_AUTO 00408 * @retval AUDIO_OK if correct communication, else wrong communication 00409 */ 00410 uint8_t BSP_AUDIO_OUT_SetOutputMode(uint8_t Output) 00411 { 00412 /* Call the Codec output Device function */ 00413 if(audio_drv->SetOutputMode(AUDIO_I2C_ADDRESS, Output) != 0) 00414 { 00415 return AUDIO_ERROR; 00416 } 00417 else 00418 { 00419 /* Return AUDIO_OK when all operations are correctly done */ 00420 return AUDIO_OK; 00421 } 00422 } 00423 00424 /** 00425 * @brief Updates the audio frequency. 00426 * @param AudioFreq: Audio frequency used to play the audio stream. 00427 * @retval AUDIO_OK if correct communication, else wrong communication 00428 */ 00429 void BSP_AUDIO_OUT_SetFrequency(uint32_t AudioFreq) 00430 { 00431 RCC_PeriphCLKInitTypeDef RCC_ExCLKInitStruct; 00432 uint8_t index = 0, freqindex = 0xFF; 00433 00434 for(index = 0; index < 8; index++) 00435 { 00436 if(I2SFreq[index] == AudioFreq) 00437 { 00438 freqindex = index; 00439 } 00440 } 00441 HAL_RCCEx_GetPeriphCLKConfig(&RCC_ExCLKInitStruct); 00442 if(freqindex != 0xFF) 00443 { 00444 /* I2S clock config 00445 PLLI2S_VCO = f(VCO clock) = f(PLLI2S clock input) � (PLLI2SN/PLLM) 00446 I2SCLK = f(PLLI2S clock output) = f(VCO clock) / PLLI2SR */ 00447 RCC_ExCLKInitStruct.PeriphClockSelection = RCC_PERIPHCLK_I2S; 00448 RCC_ExCLKInitStruct.PLLI2S.PLLI2SN = I2SPLLN[freqindex]; 00449 RCC_ExCLKInitStruct.PLLI2S.PLLI2SR = I2SPLLR[freqindex]; 00450 HAL_RCCEx_PeriphCLKConfig(&RCC_ExCLKInitStruct); 00451 } 00452 else /* Default PLL I2S configuration */ 00453 { 00454 /* I2S clock config 00455 PLLI2S_VCO = f(VCO clock) = f(PLLI2S clock input) � (PLLI2SN/PLLM) 00456 I2SCLK = f(PLLI2S clock output) = f(VCO clock) / PLLI2SR */ 00457 RCC_ExCLKInitStruct.PeriphClockSelection = RCC_PERIPHCLK_I2S; 00458 RCC_ExCLKInitStruct.PLLI2S.PLLI2SN = 258; 00459 RCC_ExCLKInitStruct.PLLI2S.PLLI2SR = 3; 00460 HAL_RCCEx_PeriphCLKConfig(&RCC_ExCLKInitStruct); 00461 } 00462 /* Update the I2S audio frequency configuration */ 00463 I2Sx_Init(AudioFreq); 00464 } 00465 00466 /** 00467 * @brief Tx Transfer completed callbacks 00468 * @param hi2s: I2S handle 00469 */ 00470 void HAL_I2S_TxCpltCallback(I2S_HandleTypeDef *hi2s) 00471 { 00472 /* Manage the remaining file size and new address offset: This function 00473 should be coded by user (its prototype is already declared in stm324xg_eval_audio.h) */ 00474 BSP_AUDIO_OUT_TransferComplete_CallBack(); 00475 } 00476 00477 /** 00478 * @brief Tx Transfer Half completed callbacks 00479 * @param hi2s: I2S handle 00480 */ 00481 void HAL_I2S_TxHalfCpltCallback(I2S_HandleTypeDef *hi2s) 00482 { 00483 /* Manage the remaining file size and new address offset: This function 00484 should be coded by user (its prototype is already declared in stm324xg_eval_audio.h) */ 00485 BSP_AUDIO_OUT_HalfTransfer_CallBack(); 00486 } 00487 00488 /** 00489 * @brief I2S error callbacks. 00490 * @param hi2s: I2S handle 00491 */ 00492 void HAL_I2S_ErrorCallback(I2S_HandleTypeDef *hi2s) 00493 { 00494 BSP_AUDIO_OUT_Error_CallBack(); 00495 } 00496 00497 /** 00498 * @brief Manages the DMA full Transfer complete event. 00499 */ 00500 __weak void BSP_AUDIO_OUT_TransferComplete_CallBack(void) 00501 { 00502 } 00503 00504 /** 00505 * @brief Manages the DMA Half Transfer complete event. 00506 */ 00507 __weak void BSP_AUDIO_OUT_HalfTransfer_CallBack(void) 00508 { 00509 } 00510 00511 /** 00512 * @brief Manages the DMA FIFO error event. 00513 */ 00514 __weak void BSP_AUDIO_OUT_Error_CallBack(void) 00515 { 00516 } 00517 00518 /******************************************************************************* 00519 Static Functions 00520 *******************************************************************************/ 00521 00522 /** 00523 * @brief Initializes I2C MSP. 00524 */ 00525 static void I2Sx_MspInit(void) 00526 { 00527 static DMA_HandleTypeDef hdma_i2sTx; 00528 GPIO_InitTypeDef GPIO_InitStruct; 00529 I2S_HandleTypeDef *hi2s = &haudio_i2s; 00530 00531 /* Enable I2S clock */ 00532 AUDIO_I2Sx_CLK_ENABLE(); 00533 00534 /* Enable SCK, SD and WS GPIO clock */ 00535 AUDIO_I2Sx_SCK_SD_WS_CLK_ENABLE(); 00536 00537 /* CODEC_I2S pins configuration: WS, SCK and SD pins */ 00538 GPIO_InitStruct.Pin = AUDIO_I2Sx_SCK_PIN; 00539 GPIO_InitStruct.Mode = GPIO_MODE_AF_PP; 00540 GPIO_InitStruct.Pull = GPIO_NOPULL; 00541 GPIO_InitStruct.Speed = GPIO_SPEED_FAST; 00542 GPIO_InitStruct.Alternate = AUDIO_I2Sx_SCK_SD_WS_AF; 00543 HAL_GPIO_Init(AUDIO_I2Sx_SCK_SD_WS_GPIO_PORT, &GPIO_InitStruct); 00544 00545 GPIO_InitStruct.Pin = AUDIO_I2Sx_SD_PIN; 00546 HAL_GPIO_Init(AUDIO_I2Sx_SCK_SD_WS_GPIO_PORT, &GPIO_InitStruct); 00547 00548 GPIO_InitStruct.Pin = AUDIO_I2Sx_WS_PIN; 00549 HAL_GPIO_Init(AUDIO_I2Sx_SCK_SD_WS_GPIO_PORT, &GPIO_InitStruct); 00550 00551 /* Enable MCK GPIO clock */ 00552 AUDIO_I2Sx_MCK_CLK_ENABLE(); 00553 00554 /* CODEC_I2S pins configuration: MCK pin */ 00555 GPIO_InitStruct.Pin = AUDIO_I2Sx_MCK_PIN; 00556 HAL_GPIO_Init(AUDIO_I2Sx_MCK_GPIO_PORT, &GPIO_InitStruct); 00557 00558 /* Enable the DMA clock */ 00559 AUDIO_I2Sx_DMAx_CLK_ENABLE(); 00560 00561 if(hi2s->Instance == AUDIO_I2Sx) 00562 { 00563 /* Configure the hdma_i2sTx handle parameters */ 00564 hdma_i2sTx.Init.Channel = AUDIO_I2Sx_DMAx_CHANNEL; 00565 hdma_i2sTx.Init.Direction = DMA_MEMORY_TO_PERIPH; 00566 hdma_i2sTx.Init.PeriphInc = DMA_PINC_DISABLE; 00567 hdma_i2sTx.Init.MemInc = DMA_MINC_ENABLE; 00568 hdma_i2sTx.Init.PeriphDataAlignment = AUDIO_I2Sx_DMAx_PERIPH_DATA_SIZE; 00569 hdma_i2sTx.Init.MemDataAlignment = AUDIO_I2Sx_DMAx_MEM_DATA_SIZE; 00570 hdma_i2sTx.Init.Mode = DMA_NORMAL; 00571 hdma_i2sTx.Init.Priority = DMA_PRIORITY_HIGH; 00572 hdma_i2sTx.Init.FIFOMode = DMA_FIFOMODE_ENABLE; 00573 hdma_i2sTx.Init.FIFOThreshold = DMA_FIFO_THRESHOLD_FULL; 00574 hdma_i2sTx.Init.MemBurst = DMA_MBURST_SINGLE; 00575 hdma_i2sTx.Init.PeriphBurst = DMA_PBURST_SINGLE; 00576 00577 hdma_i2sTx.Instance = AUDIO_I2Sx_DMAx_STREAM; 00578 00579 /* Associate the DMA handle */ 00580 __HAL_LINKDMA(hi2s, hdmatx, hdma_i2sTx); 00581 00582 /* Deinitialize the Stream for new transfer */ 00583 HAL_DMA_DeInit(&hdma_i2sTx); 00584 00585 /* Configure the DMA Stream */ 00586 HAL_DMA_Init(&hdma_i2sTx); 00587 } 00588 00589 /* I2S DMA IRQ Channel configuration */ 00590 HAL_NVIC_SetPriority(AUDIO_I2Sx_DMAx_IRQ, AUDIO_IRQ_PREPRIO, 0); 00591 HAL_NVIC_EnableIRQ(AUDIO_I2Sx_DMAx_IRQ); 00592 } 00593 00594 /** 00595 * @brief Initializes the Audio Codec audio interface (I2S). 00596 * @param AudioFreq: Audio frequency to be configured for the I2S peripheral. 00597 */ 00598 static void I2Sx_Init(uint32_t AudioFreq) 00599 { 00600 /* Initialize the haudio_i2s Instance parameter */ 00601 haudio_i2s.Instance = AUDIO_I2Sx; 00602 00603 /* Disable I2S block */ 00604 __HAL_I2S_DISABLE(&haudio_i2s); 00605 00606 haudio_i2s.Init.Mode = I2S_MODE_MASTER_TX; 00607 haudio_i2s.Init.Standard = I2S_STANDARD; 00608 haudio_i2s.Init.DataFormat = I2S_DATAFORMAT_16B; 00609 haudio_i2s.Init.AudioFreq = AudioFreq; 00610 haudio_i2s.Init.CPOL = I2S_CPOL_LOW; 00611 haudio_i2s.Init.MCLKOutput = I2S_MCLKOUTPUT_ENABLE; 00612 00613 if(HAL_I2S_GetState(&haudio_i2s) == HAL_I2S_STATE_RESET) 00614 { 00615 I2Sx_MspInit(); 00616 } 00617 /* Init the I2S */ 00618 HAL_I2S_Init(&haudio_i2s); 00619 } 00620 00621 /** 00622 * @brief Resets the audio codec. It restores the default configuration of the 00623 * codec (this function shall be called before initializing the codec). 00624 * @note This function calls an external driver function: The IO Expander driver. 00625 */ 00626 static void CODEC_Reset(void) 00627 { 00628 /* Configure the IO Expander (to use the Codec Reset pin mapped on the IOExpander) */ 00629 BSP_IO_Init(); 00630 00631 BSP_IO_ConfigPin(AUDIO_RESET_PIN, IO_MODE_OUTPUT); 00632 00633 /* Power Down the codec */ 00634 BSP_IO_WritePin(AUDIO_RESET_PIN, RESET); 00635 00636 /* Wait for a delay to insure registers erasing */ 00637 HAL_Delay(CODEC_RESET_DELAY); 00638 00639 /* Power on the codec */ 00640 BSP_IO_WritePin(AUDIO_RESET_PIN, SET); 00641 00642 /* Wait for a delay to insure registers erasing */ 00643 HAL_Delay(CODEC_RESET_DELAY); 00644 } 00645 00646 /** 00647 * @} 00648 */ 00649 00650 /** 00651 * @} 00652 */ 00653 00654 /** 00655 * @} 00656 */ 00657 00658 /** 00659 * @} 00660 */ 00661 00662 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Fri Jan 15 2016 14:22:29 for STM324xG_EVAL BSP User Manual by
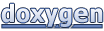