STM324x9I_EVAL BSP User Manual
|
stm324x9i_eval_sram.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm324x9i_eval_sram.c 00004 * @author MCD Application Team 00005 * @version V2.2.2 00006 * @date 13-January-2016 00007 * @brief This file includes the SRAM driver for the IS61WV102416BLL-10M memory 00008 * device mounted on STM324x9I-EVAL evaluation board. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* File Info : ----------------------------------------------------------------- 00040 User NOTES 00041 1. How To use this driver: 00042 -------------------------- 00043 - This driver is used to drive the IS61WV102416BLL-10M SRAM external memory mounted 00044 on STM324x9I-EVAL evaluation board. 00045 - This driver does not need a specific component driver for the SRAM device 00046 to be included with. 00047 00048 2. Driver description: 00049 --------------------- 00050 + Initialization steps: 00051 o Initialize the SRAM external memory using the BSP_SRAM_Init() function. This 00052 function includes the MSP layer hardware resources initialization and the 00053 FMC controller configuration to interface with the external SRAM memory. 00054 00055 + SRAM read/write operations 00056 o SRAM external memory can be accessed with read/write operations once it is 00057 initialized. 00058 Read/write operation can be performed with AHB access using the functions 00059 BSP_SRAM_ReadData()/BSP_SRAM_WriteData(), or by DMA transfer using the functions 00060 BSP_SRAM_ReadData_DMA()/BSP_SRAM_WriteData_DMA(). 00061 o The AHB access is performed with 16-bit width transaction, the DMA transfer 00062 configuration is fixed at single (no burst) halfword transfer 00063 (see the SRAM_MspInit() static function). 00064 o User can implement his own functions for read/write access with his desired 00065 configurations. 00066 o If interrupt mode is used for DMA transfer, the function BSP_SRAM_DMA_IRQHandler() 00067 is called in IRQ handler file, to serve the generated interrupt once the DMA 00068 transfer is complete. 00069 00070 ------------------------------------------------------------------------------*/ 00071 00072 /* Includes ------------------------------------------------------------------*/ 00073 #include "stm324x9i_eval_sram.h" 00074 00075 /** @addtogroup BSP 00076 * @{ 00077 */ 00078 00079 /** @addtogroup STM324x9I_EVAL 00080 * @{ 00081 */ 00082 00083 /** @defgroup STM324x9I_EVAL_SRAM STM324x9I EVAL SRAM 00084 * @{ 00085 */ 00086 00087 /** @defgroup STM324x9I_EVAL_SRAM_Private_Types_Definitions STM324x9I EVAL SRAM Private Types Definitions 00088 * @{ 00089 */ 00090 /** 00091 * @} 00092 */ 00093 00094 /** @defgroup STM324x9I_EVAL_SRAM_Private_Defines STM324x9I EVAL SRAM Private Defines 00095 * @{ 00096 */ 00097 /** 00098 * @} 00099 */ 00100 00101 /** @defgroup STM324x9I_EVAL_SRAM_Private_Macros STM324x9I EVAL SRAM Private Macros 00102 * @{ 00103 */ 00104 /** 00105 * @} 00106 */ 00107 00108 /** @defgroup STM324x9I_EVAL_SRAM_Private_Variables STM324x9I EVAL SRAM Private Variables 00109 * @{ 00110 */ 00111 static SRAM_HandleTypeDef sramHandle; 00112 static FMC_NORSRAM_TimingTypeDef Timing; 00113 /** 00114 * @} 00115 */ 00116 00117 /** @defgroup STM324x9I_EVAL_SRAM_Private_Function_Prototypes STM324x9I EVAL SRAM Private Function Prototypes 00118 * @{ 00119 */ 00120 static void SRAM_MspInit(void); 00121 /** 00122 * @} 00123 */ 00124 00125 /** @defgroup STM324x9I_EVAL_SRAM_Private_Functions STM324x9I EVAL SRAM Private Functions 00126 * @{ 00127 */ 00128 00129 /** 00130 * @brief Initializes the SRAM device. 00131 * @retval SRAM status 00132 */ 00133 uint8_t BSP_SRAM_Init(void) 00134 { 00135 sramHandle.Instance = FMC_NORSRAM_DEVICE; 00136 sramHandle.Extended = FMC_NORSRAM_EXTENDED_DEVICE; 00137 00138 /* SRAM device configuration */ 00139 Timing.AddressSetupTime = 2; 00140 Timing.AddressHoldTime = 1; 00141 Timing.DataSetupTime = 2; 00142 Timing.BusTurnAroundDuration = 1; 00143 Timing.CLKDivision = 2; 00144 Timing.DataLatency = 2; 00145 Timing.AccessMode = FMC_ACCESS_MODE_A; 00146 00147 sramHandle.Init.NSBank = FMC_NORSRAM_BANK2; 00148 sramHandle.Init.DataAddressMux = FMC_DATA_ADDRESS_MUX_DISABLE; 00149 sramHandle.Init.MemoryType = FMC_MEMORY_TYPE_SRAM; 00150 sramHandle.Init.MemoryDataWidth = SRAM_MEMORY_WIDTH; 00151 sramHandle.Init.BurstAccessMode = SRAM_BURSTACCESS; 00152 sramHandle.Init.WaitSignalPolarity = FMC_WAIT_SIGNAL_POLARITY_LOW; 00153 sramHandle.Init.WrapMode = FMC_WRAP_MODE_DISABLE; 00154 sramHandle.Init.WaitSignalActive = FMC_WAIT_TIMING_BEFORE_WS; 00155 sramHandle.Init.WriteOperation = FMC_WRITE_OPERATION_ENABLE; 00156 sramHandle.Init.WaitSignal = FMC_WAIT_SIGNAL_DISABLE; 00157 sramHandle.Init.ExtendedMode = FMC_EXTENDED_MODE_DISABLE; 00158 sramHandle.Init.AsynchronousWait = FMC_ASYNCHRONOUS_WAIT_DISABLE; 00159 sramHandle.Init.WriteBurst = SRAM_WRITEBURST; 00160 sramHandle.Init.ContinuousClock = CONTINUOUSCLOCK_FEATURE; 00161 00162 /* SRAM controller initialization */ 00163 SRAM_MspInit(); 00164 if(HAL_SRAM_Init(&sramHandle, &Timing, &Timing) != HAL_OK) 00165 { 00166 return SRAM_ERROR; 00167 } 00168 else 00169 { 00170 return SRAM_OK; 00171 } 00172 } 00173 00174 /** 00175 * @brief Reads an amount of data from the SRAM device in polling mode. 00176 * @param uwStartAddress: Read start address 00177 * @param pData: Pointer to data to be read 00178 * @param uwDataSize: Size of read data from the memory 00179 * @retval SRAM status 00180 */ 00181 uint8_t BSP_SRAM_ReadData(uint32_t uwStartAddress, uint16_t *pData, uint32_t uwDataSize) 00182 { 00183 if(HAL_SRAM_Read_16b(&sramHandle, (uint32_t *)uwStartAddress, pData, uwDataSize) != HAL_OK) 00184 { 00185 return SRAM_ERROR; 00186 } 00187 else 00188 { 00189 return SRAM_OK; 00190 } 00191 } 00192 00193 /** 00194 * @brief Reads an amount of data from the SRAM device in DMA mode. 00195 * @param uwStartAddress: Read start address 00196 * @param pData: Pointer to data to be read 00197 * @param uwDataSize: Size of read data from the memory 00198 * @retval SRAM status 00199 */ 00200 uint8_t BSP_SRAM_ReadData_DMA(uint32_t uwStartAddress, uint16_t *pData, uint32_t uwDataSize) 00201 { 00202 if(HAL_SRAM_Read_DMA(&sramHandle, (uint32_t *)uwStartAddress, (uint32_t *)pData, uwDataSize) != HAL_OK) 00203 { 00204 return SRAM_ERROR; 00205 } 00206 else 00207 { 00208 return SRAM_OK; 00209 } 00210 } 00211 00212 /** 00213 * @brief Writes an amount of data from the SRAM device in polling mode. 00214 * @param uwStartAddress: Write start address 00215 * @param pData: Pointer to data to be written 00216 * @param uwDataSize: Size of written data from the memory 00217 * @retval SRAM status 00218 */ 00219 uint8_t BSP_SRAM_WriteData(uint32_t uwStartAddress, uint16_t *pData, uint32_t uwDataSize) 00220 { 00221 if(HAL_SRAM_Write_16b(&sramHandle, (uint32_t *)uwStartAddress, pData, uwDataSize) != HAL_OK) 00222 { 00223 return SRAM_ERROR; 00224 } 00225 else 00226 { 00227 return SRAM_OK; 00228 } 00229 } 00230 00231 /** 00232 * @brief Writes an amount of data from the SRAM device in DMA mode. 00233 * @param uwStartAddress: Write start address 00234 * @param pData: Pointer to data to be written 00235 * @param uwDataSize: Size of written data from the memory 00236 * @retval SRAM status 00237 */ 00238 uint8_t BSP_SRAM_WriteData_DMA(uint32_t uwStartAddress, uint16_t *pData, uint32_t uwDataSize) 00239 { 00240 if(HAL_SRAM_Write_DMA(&sramHandle, (uint32_t *)uwStartAddress, (uint32_t *)pData, uwDataSize) != HAL_OK) 00241 { 00242 return SRAM_ERROR; 00243 } 00244 else 00245 { 00246 return SRAM_OK; 00247 } 00248 } 00249 00250 /** 00251 * @brief Handles SRAM DMA transfer interrupt request. 00252 */ 00253 void BSP_SRAM_DMA_IRQHandler(void) 00254 { 00255 HAL_DMA_IRQHandler(sramHandle.hdma); 00256 } 00257 00258 /** 00259 * @brief Initializes SRAM MSP. 00260 */ 00261 static void SRAM_MspInit(void) 00262 { 00263 static DMA_HandleTypeDef dmaHandle; 00264 GPIO_InitTypeDef GPIO_Init_Structure; 00265 SRAM_HandleTypeDef *hsram = &sramHandle; 00266 00267 /* Enable FMC clock */ 00268 __FMC_CLK_ENABLE(); 00269 00270 /* Enable chosen DMAx clock */ 00271 __SRAM_DMAx_CLK_ENABLE(); 00272 00273 /* Enable GPIOs clock */ 00274 __GPIOD_CLK_ENABLE(); 00275 __GPIOE_CLK_ENABLE(); 00276 __GPIOF_CLK_ENABLE(); 00277 __GPIOG_CLK_ENABLE(); 00278 00279 /* Common GPIO configuration */ 00280 GPIO_Init_Structure.Mode = GPIO_MODE_AF_PP; 00281 GPIO_Init_Structure.Pull = GPIO_PULLUP; 00282 GPIO_Init_Structure.Speed = GPIO_SPEED_HIGH; 00283 GPIO_Init_Structure.Alternate = GPIO_AF12_FMC; 00284 00285 /* GPIOD configuration */ 00286 GPIO_Init_Structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_4 | GPIO_PIN_5 | GPIO_PIN_8 |\ 00287 GPIO_PIN_9 | GPIO_PIN_10 | GPIO_PIN_11 | GPIO_PIN_12 | GPIO_PIN_13 |\ 00288 GPIO_PIN_14 | GPIO_PIN_15; 00289 HAL_GPIO_Init(GPIOD, &GPIO_Init_Structure); 00290 00291 /* GPIOE configuration */ 00292 GPIO_Init_Structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_3| GPIO_PIN_4 | GPIO_PIN_7 |\ 00293 GPIO_PIN_8 | GPIO_PIN_9 | GPIO_PIN_10 | GPIO_PIN_11 | GPIO_PIN_12 |\ 00294 GPIO_PIN_13 | GPIO_PIN_14 | GPIO_PIN_15; 00295 HAL_GPIO_Init(GPIOE, &GPIO_Init_Structure); 00296 00297 /* GPIOF configuration */ 00298 GPIO_Init_Structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_2| GPIO_PIN_3 | GPIO_PIN_4 |\ 00299 GPIO_PIN_5 | GPIO_PIN_12 | GPIO_PIN_13 | GPIO_PIN_14 | GPIO_PIN_15; 00300 HAL_GPIO_Init(GPIOF, &GPIO_Init_Structure); 00301 00302 /* GPIOG configuration */ 00303 GPIO_Init_Structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_2| GPIO_PIN_3 | GPIO_PIN_4 |\ 00304 GPIO_PIN_5 | GPIO_PIN_9; 00305 HAL_GPIO_Init(GPIOG, &GPIO_Init_Structure); 00306 00307 /* Configure common DMA parameters */ 00308 dmaHandle.Init.Channel = SRAM_DMAx_CHANNEL; 00309 dmaHandle.Init.Direction = DMA_MEMORY_TO_MEMORY; 00310 dmaHandle.Init.PeriphInc = DMA_PINC_ENABLE; 00311 dmaHandle.Init.MemInc = DMA_MINC_ENABLE; 00312 dmaHandle.Init.PeriphDataAlignment = DMA_PDATAALIGN_HALFWORD; 00313 dmaHandle.Init.MemDataAlignment = DMA_MDATAALIGN_HALFWORD; 00314 dmaHandle.Init.Mode = DMA_NORMAL; 00315 dmaHandle.Init.Priority = DMA_PRIORITY_HIGH; 00316 dmaHandle.Init.FIFOMode = DMA_FIFOMODE_DISABLE; 00317 dmaHandle.Init.FIFOThreshold = DMA_FIFO_THRESHOLD_FULL; 00318 dmaHandle.Init.MemBurst = DMA_MBURST_SINGLE; 00319 dmaHandle.Init.PeriphBurst = DMA_PBURST_SINGLE; 00320 00321 dmaHandle.Instance = SRAM_DMAx_STREAM; 00322 00323 /* Associate the DMA handle */ 00324 __HAL_LINKDMA(hsram, hdma, dmaHandle); 00325 00326 /* Deinitialize the Stream for new transfer */ 00327 HAL_DMA_DeInit(&dmaHandle); 00328 00329 /* Configure the DMA Stream */ 00330 HAL_DMA_Init(&dmaHandle); 00331 00332 /* NVIC configuration for DMA transfer complete interrupt */ 00333 HAL_NVIC_SetPriority(SRAM_DMAx_IRQn, 5, 0); 00334 HAL_NVIC_EnableIRQ(SRAM_DMAx_IRQn); 00335 } 00336 00337 /** 00338 * @} 00339 */ 00340 00341 /** 00342 * @} 00343 */ 00344 00345 /** 00346 * @} 00347 */ 00348 00349 /** 00350 * @} 00351 */ 00352 00353 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Wed Jan 13 2016 15:52:54 for STM324x9I_EVAL BSP User Manual by
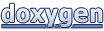