STM324x9I_EVAL BSP User Manual
|
stm324x9i_eval_camera.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm324x9i_eval_camera.c 00004 * @author MCD Application Team 00005 * @version V2.2.2 00006 * @date 13-January-2016 00007 * @brief This file includes the driver for Camera modules mounted on 00008 * STM324x9I-EVAL evaluation board. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* File Info: ------------------------------------------------------------------ 00040 User NOTES 00041 1. How to use this driver: 00042 -------------------------- 00043 - This driver is used to drive the camera. 00044 - The OV2640 component driver MUST be included with this driver. 00045 00046 2. Driver description: 00047 --------------------- 00048 + Initialization steps: 00049 o Initialize the camera using the BSP_CAMERA_Init() function. 00050 o Start the camera capture/snapshot using the CAMERA_Start() function. 00051 o Suspend, resume or stop the camera capture using the following functions: 00052 - BSP_CAMERA_Suspend() 00053 - BSP_CAMERA_Resume() 00054 - BSP_CAMERA_Stop() 00055 00056 + Options 00057 o Increase or decrease on the fly the brightness and/or contrast 00058 using the following function: 00059 - BSP_CAMERA_ContrastBrightnessConfig 00060 o Add a special effect on the fly using the following functions: 00061 - BSP_CAMERA_BlackWhiteConfig() 00062 - BSP_CAMERA_ColorEffectConfig() 00063 00064 ------------------------------------------------------------------------------*/ 00065 00066 /* Includes ------------------------------------------------------------------*/ 00067 #include "stm324x9i_eval_camera.h" 00068 00069 /** @addtogroup BSP 00070 * @{ 00071 */ 00072 00073 /** @addtogroup STM324x9I_EVAL 00074 * @{ 00075 */ 00076 00077 /** @defgroup STM324x9I_EVAL_CAMERA STM324x9I EVAL CAMERA 00078 * @{ 00079 */ 00080 00081 /** @defgroup STM324x9I_EVAL_CAMERA_Private_TypesDefinitions STM324x9I EVAL CAMERA Private TypesDefinitions 00082 * @{ 00083 */ 00084 /** 00085 * @} 00086 */ 00087 00088 /** @defgroup STM324x9I_EVAL_CAMERA_Private_Defines STM324x9I EVAL CAMERA Private Defines 00089 * @{ 00090 */ 00091 /** 00092 * @} 00093 */ 00094 00095 /** @defgroup STM324x9I_EVAL_CAMERA_Private_Macros STM324x9I EVAL CAMERA Private Macros 00096 * @{ 00097 */ 00098 /** 00099 * @} 00100 */ 00101 00102 /** @defgroup STM324x9I_EVAL_CAMERA_Private_Variables STM324x9I EVAL CAMERA Private Variables 00103 * @{ 00104 */ 00105 static DCMI_HandleTypeDef hdcmi_eval; 00106 CAMERA_DrvTypeDef *camera_drv; 00107 uint32_t current_resolution; 00108 /** 00109 * @} 00110 */ 00111 00112 /** @defgroup STM324x9I_EVAL_CAMERA_Private_FunctionPrototypes STM324x9I EVAL CAMERA Private FunctionPrototypes 00113 * @{ 00114 */ 00115 static void DCMI_MspInit(void); 00116 static uint32_t GetSize(uint32_t resolution); 00117 /** 00118 * @} 00119 */ 00120 00121 /** @defgroup STM324x9I_EVAL_CAMERA_Private_Functions STM324x9I EVAL CAMERA Private Functions 00122 * @{ 00123 */ 00124 00125 /** 00126 * @brief Initializes the camera. 00127 * @param Resolution: Camera Resolution 00128 * @retval Camera status 00129 */ 00130 uint8_t BSP_CAMERA_Init(uint32_t Resolution) 00131 { 00132 DCMI_HandleTypeDef *phdcmi; 00133 00134 uint8_t ret = CAMERA_ERROR; 00135 00136 /* Get the DCMI handle structure */ 00137 phdcmi = &hdcmi_eval; 00138 00139 /*** Configures the DCMI to interface with the camera module ***/ 00140 /* DCMI configuration */ 00141 phdcmi->Init.CaptureRate = DCMI_CR_ALL_FRAME; 00142 phdcmi->Init.HSPolarity = DCMI_HSPOLARITY_LOW; 00143 phdcmi->Init.SynchroMode = DCMI_SYNCHRO_HARDWARE; 00144 phdcmi->Init.VSPolarity = DCMI_VSPOLARITY_LOW; 00145 phdcmi->Init.ExtendedDataMode = DCMI_EXTEND_DATA_8B; 00146 phdcmi->Init.PCKPolarity = DCMI_PCKPOLARITY_RISING; 00147 phdcmi->Instance = DCMI; 00148 00149 /* Configure IO functionalities for camera detect pin */ 00150 BSP_IO_Init(); 00151 00152 /* Set the camera STANDBY pin */ 00153 BSP_IO_ConfigPin(XSDN_PIN, IO_MODE_OUTPUT); 00154 BSP_IO_WritePin(XSDN_PIN, SET); 00155 00156 /* Check if the camera is plugged */ 00157 if(BSP_IO_ReadPin(CAM_PLUG_PIN)) 00158 { 00159 return CAMERA_ERROR; 00160 } 00161 00162 /* DCMI Initialization */ 00163 DCMI_MspInit(); 00164 HAL_DCMI_Init(phdcmi); 00165 00166 if(ov2640_ReadID(CAMERA_I2C_ADDRESS) == OV2640_ID) 00167 { 00168 /* Initialize the camera driver structure */ 00169 camera_drv = &ov2640_drv; 00170 00171 /* Camera Init */ 00172 camera_drv->Init(CAMERA_I2C_ADDRESS, Resolution); 00173 00174 /* Return CAMERA_OK status */ 00175 ret = CAMERA_OK; 00176 } 00177 00178 current_resolution = Resolution; 00179 00180 return ret; 00181 } 00182 00183 /** 00184 * @brief Starts the camera capture in continuous mode. 00185 * @param buff: pointer to the camera output buffer 00186 */ 00187 void BSP_CAMERA_ContinuousStart(uint8_t *buff) 00188 { 00189 /* Start the camera capture */ 00190 HAL_DCMI_Start_DMA(&hdcmi_eval, DCMI_MODE_CONTINUOUS, (uint32_t)buff, GetSize(current_resolution)); 00191 } 00192 00193 /** 00194 * @brief Starts the camera capture in snapshot mode. 00195 * @param buff: pointer to the camera output buffer 00196 */ 00197 void BSP_CAMERA_SnapshotStart(uint8_t *buff) 00198 { 00199 /* Start the camera capture */ 00200 HAL_DCMI_Start_DMA(&hdcmi_eval, DCMI_MODE_SNAPSHOT, (uint32_t)buff, GetSize(current_resolution)); 00201 } 00202 00203 /** 00204 * @brief Suspend the CAMERA capture 00205 */ 00206 void BSP_CAMERA_Suspend(void) 00207 { 00208 /* Disable the DMA */ 00209 __HAL_DMA_DISABLE(hdcmi_eval.DMA_Handle); 00210 /* Disable the DCMI */ 00211 __HAL_DCMI_DISABLE(&hdcmi_eval); 00212 00213 } 00214 00215 /** 00216 * @brief Resume the CAMERA capture 00217 */ 00218 void BSP_CAMERA_Resume(void) 00219 { 00220 /* Enable the DCMI */ 00221 __HAL_DCMI_ENABLE(&hdcmi_eval); 00222 /* Enable the DMA */ 00223 __HAL_DMA_ENABLE(hdcmi_eval.DMA_Handle); 00224 } 00225 00226 /** 00227 * @brief Stop the CAMERA capture 00228 * @retval Camera status 00229 */ 00230 uint8_t BSP_CAMERA_Stop(void) 00231 { 00232 DCMI_HandleTypeDef *phdcmi; 00233 00234 uint8_t ret = CAMERA_ERROR; 00235 00236 /* Get the DCMI handle structure */ 00237 phdcmi = &hdcmi_eval; 00238 00239 if(HAL_DCMI_Stop(phdcmi) == HAL_OK) 00240 { 00241 ret = CAMERA_OK; 00242 } 00243 00244 /* Initialize IO */ 00245 BSP_IO_Init(); 00246 00247 /* Reset the camera STANDBY pin */ 00248 BSP_IO_ConfigPin(XSDN_PIN, IO_MODE_OUTPUT); 00249 BSP_IO_WritePin(XSDN_PIN, RESET); 00250 00251 return ret; 00252 } 00253 00254 /** 00255 * @brief Configures the camera contrast and brightness. 00256 * @param contrast_level: Contrast level 00257 * This parameter can be one of the following values: 00258 * @arg CAMERA_CONTRAST_LEVEL4: for contrast +2 00259 * @arg CAMERA_CONTRAST_LEVEL3: for contrast +1 00260 * @arg CAMERA_CONTRAST_LEVEL2: for contrast 0 00261 * @arg CAMERA_CONTRAST_LEVEL1: for contrast -1 00262 * @arg CAMERA_CONTRAST_LEVEL0: for contrast -2 00263 * @param brightness_level: Contrast level 00264 * This parameter can be one of the following values: 00265 * @arg CAMERA_BRIGHTNESS_LEVEL4: for brightness +2 00266 * @arg CAMERA_BRIGHTNESS_LEVEL3: for brightness +1 00267 * @arg CAMERA_BRIGHTNESS_LEVEL2: for brightness 0 00268 * @arg CAMERA_BRIGHTNESS_LEVEL1: for brightness -1 00269 * @arg CAMERA_BRIGHTNESS_LEVEL0: for brightness -2 00270 */ 00271 void BSP_CAMERA_ContrastBrightnessConfig(uint32_t contrast_level, uint32_t brightness_level) 00272 { 00273 if(camera_drv->Config != NULL) 00274 { 00275 camera_drv->Config(CAMERA_I2C_ADDRESS, CAMERA_CONTRAST_BRIGHTNESS, contrast_level, brightness_level); 00276 } 00277 } 00278 00279 /** 00280 * @brief Configures the camera white balance. 00281 * @param Mode: black_white mode 00282 * This parameter can be one of the following values: 00283 * @arg CAMERA_BLACK_WHITE_BW 00284 * @arg CAMERA_BLACK_WHITE_NEGATIVE 00285 * @arg CAMERA_BLACK_WHITE_BW_NEGATIVE 00286 * @arg CAMERA_BLACK_WHITE_NORMAL 00287 */ 00288 void BSP_CAMERA_BlackWhiteConfig(uint32_t Mode) 00289 { 00290 if(camera_drv->Config != NULL) 00291 { 00292 camera_drv->Config(CAMERA_I2C_ADDRESS, CAMERA_BLACK_WHITE, Mode, 0); 00293 } 00294 } 00295 00296 /** 00297 * @brief Configures the camera color effect. 00298 * @param Effect: Color effect 00299 * This parameter can be one of the following values: 00300 * @arg CAMERA_COLOR_EFFECT_ANTIQUE 00301 * @arg CAMERA_COLOR_EFFECT_BLUE 00302 * @arg CAMERA_COLOR_EFFECT_GREEN 00303 * @arg CAMERA_COLOR_EFFECT_RED 00304 */ 00305 void BSP_CAMERA_ColorEffectConfig(uint32_t Effect) 00306 { 00307 if(camera_drv->Config != NULL) 00308 { 00309 camera_drv->Config(CAMERA_I2C_ADDRESS, CAMERA_COLOR_EFFECT, Effect, 0); 00310 } 00311 } 00312 00313 /** 00314 * @brief Handles DCMI interrupt request. 00315 */ 00316 void BSP_CAMERA_IRQHandler(void) 00317 { 00318 HAL_DCMI_IRQHandler(&hdcmi_eval); 00319 } 00320 00321 /** 00322 * @brief Handles DMA interrupt request. 00323 */ 00324 void BSP_CAMERA_DMA_IRQHandler(void) 00325 { 00326 HAL_DMA_IRQHandler(hdcmi_eval.DMA_Handle); 00327 } 00328 00329 /** 00330 * @brief Get the capture size. 00331 * @param resolution: the current resolution. 00332 * @retval capture size. 00333 */ 00334 static uint32_t GetSize(uint32_t resolution) 00335 { 00336 uint32_t size = 0; 00337 00338 /* Get capture size */ 00339 switch (resolution) 00340 { 00341 case CAMERA_R160x120: 00342 { 00343 size = 0x2580; 00344 } 00345 break; 00346 case CAMERA_R320x240: 00347 { 00348 size = 0x9600; 00349 } 00350 break; 00351 case CAMERA_R480x272: 00352 { 00353 size = 0xFF00; 00354 } 00355 break; 00356 case CAMERA_R640x480: 00357 { 00358 size = 0x25800; 00359 } 00360 break; 00361 default: 00362 { 00363 break; 00364 } 00365 } 00366 00367 return size; 00368 } 00369 00370 /** 00371 * @brief Initializes the DCMI MSP. 00372 */ 00373 static void DCMI_MspInit(void) 00374 { 00375 static DMA_HandleTypeDef hdma_eval; 00376 GPIO_InitTypeDef GPIO_Init_Structure; 00377 DCMI_HandleTypeDef *hdcmi = &hdcmi_eval; 00378 00379 /*** Enable peripherals and GPIO clocks ***/ 00380 /* Enable DCMI clock */ 00381 __DCMI_CLK_ENABLE(); 00382 00383 /* Enable DMA2 clock */ 00384 __DMA2_CLK_ENABLE(); 00385 00386 /* Enable GPIO clocks */ 00387 __GPIOA_CLK_ENABLE(); 00388 __GPIOB_CLK_ENABLE(); 00389 __GPIOC_CLK_ENABLE(); 00390 __GPIOD_CLK_ENABLE(); 00391 __GPIOE_CLK_ENABLE(); 00392 00393 /*** Configure the GPIO ***/ 00394 /* Configure DCMI GPIO as alternate function */ 00395 GPIO_Init_Structure.Pin = GPIO_PIN_4; 00396 GPIO_Init_Structure.Mode = GPIO_MODE_AF_PP; 00397 GPIO_Init_Structure.Pull = GPIO_PULLUP; 00398 GPIO_Init_Structure.Speed = GPIO_SPEED_HIGH; 00399 GPIO_Init_Structure.Alternate = GPIO_AF13_DCMI; 00400 HAL_GPIO_Init(GPIOA, &GPIO_Init_Structure); 00401 00402 GPIO_Init_Structure.Pin = GPIO_PIN_7 | GPIO_PIN_8; 00403 GPIO_Init_Structure.Mode = GPIO_MODE_AF_PP; 00404 GPIO_Init_Structure.Pull = GPIO_PULLUP; 00405 GPIO_Init_Structure.Speed = GPIO_SPEED_HIGH; 00406 GPIO_Init_Structure.Alternate = GPIO_AF13_DCMI; 00407 HAL_GPIO_Init(GPIOB, &GPIO_Init_Structure); 00408 00409 GPIO_Init_Structure.Pin = GPIO_PIN_6 | GPIO_PIN_7 | GPIO_PIN_8 |\ 00410 GPIO_PIN_9 | GPIO_PIN_10 | GPIO_PIN_11 |\ 00411 GPIO_PIN_12; 00412 GPIO_Init_Structure.Mode = GPIO_MODE_AF_PP; 00413 GPIO_Init_Structure.Pull = GPIO_PULLUP; 00414 GPIO_Init_Structure.Speed = GPIO_SPEED_HIGH; 00415 GPIO_Init_Structure.Alternate = GPIO_AF13_DCMI; 00416 HAL_GPIO_Init(GPIOC, &GPIO_Init_Structure); 00417 00418 GPIO_Init_Structure.Pin = GPIO_PIN_2 | GPIO_PIN_3 | GPIO_PIN_6; 00419 GPIO_Init_Structure.Mode = GPIO_MODE_AF_PP; 00420 GPIO_Init_Structure.Pull = GPIO_PULLUP; 00421 GPIO_Init_Structure.Speed = GPIO_SPEED_HIGH; 00422 GPIO_Init_Structure.Alternate = GPIO_AF13_DCMI; 00423 HAL_GPIO_Init(GPIOD, &GPIO_Init_Structure); 00424 00425 GPIO_Init_Structure.Pin = GPIO_PIN_6; 00426 GPIO_Init_Structure.Mode = GPIO_MODE_AF_PP; 00427 GPIO_Init_Structure.Pull = GPIO_PULLUP; 00428 GPIO_Init_Structure.Speed = GPIO_SPEED_HIGH; 00429 GPIO_Init_Structure.Alternate = GPIO_AF13_DCMI; 00430 HAL_GPIO_Init(GPIOE, &GPIO_Init_Structure); 00431 00432 GPIO_Init_Structure.Pin = GPIO_PIN_6; 00433 GPIO_Init_Structure.Mode = GPIO_MODE_AF_PP; 00434 GPIO_Init_Structure.Pull = GPIO_PULLUP; 00435 GPIO_Init_Structure.Speed = GPIO_SPEED_HIGH; 00436 GPIO_Init_Structure.Alternate = GPIO_AF13_DCMI; 00437 HAL_GPIO_Init(GPIOA, &GPIO_Init_Structure); 00438 00439 /*** Configure the DMA ***/ 00440 /* Set the parameters to be configured */ 00441 hdma_eval.Init.Channel = DMA_CHANNEL_1; 00442 hdma_eval.Init.Direction = DMA_PERIPH_TO_MEMORY; 00443 hdma_eval.Init.PeriphInc = DMA_PINC_DISABLE; 00444 hdma_eval.Init.MemInc = DMA_MINC_ENABLE; 00445 hdma_eval.Init.PeriphDataAlignment = DMA_PDATAALIGN_WORD; 00446 hdma_eval.Init.MemDataAlignment = DMA_MDATAALIGN_WORD; 00447 hdma_eval.Init.Mode = DMA_CIRCULAR; 00448 hdma_eval.Init.Priority = DMA_PRIORITY_HIGH; 00449 hdma_eval.Init.FIFOMode = DMA_FIFOMODE_DISABLE; 00450 hdma_eval.Init.FIFOThreshold = DMA_FIFO_THRESHOLD_FULL; 00451 hdma_eval.Init.MemBurst = DMA_MBURST_SINGLE; 00452 hdma_eval.Init.PeriphBurst = DMA_PBURST_SINGLE; 00453 00454 hdma_eval.Instance = DMA2_Stream1; 00455 00456 /* Associate the initialized DMA handle to the DCMI handle */ 00457 __HAL_LINKDMA(hdcmi, DMA_Handle, hdma_eval); 00458 00459 /*** Configure the NVIC for DCMI and DMA ***/ 00460 /* NVIC configuration for DCMI transfer complete interrupt */ 00461 HAL_NVIC_SetPriority(DCMI_IRQn, 5, 0); 00462 HAL_NVIC_EnableIRQ(DCMI_IRQn); 00463 00464 /* NVIC configuration for DMA2D transfer complete interrupt */ 00465 HAL_NVIC_SetPriority(DMA2_Stream1_IRQn, 5, 0); 00466 HAL_NVIC_EnableIRQ(DMA2_Stream1_IRQn); 00467 00468 /* Configure the DMA stream */ 00469 HAL_DMA_Init(hdcmi->DMA_Handle); 00470 } 00471 00472 /** 00473 * @brief Line event callback 00474 * @param hdcmi: pointer to the DCMI handle 00475 */ 00476 void HAL_DCMI_LineEventCallback(DCMI_HandleTypeDef *hdcmi) 00477 { 00478 BSP_CAMERA_LineEventCallback(); 00479 } 00480 00481 /** 00482 * @brief Line Event callback. 00483 */ 00484 __weak void BSP_CAMERA_LineEventCallback(void) 00485 { 00486 /* NOTE : This function Should not be modified, when the callback is needed, 00487 the HAL_DCMI_LineEventCallback could be implemented in the user file 00488 */ 00489 } 00490 00491 /** 00492 * @brief VSYNC event callback 00493 * @param hdcmi: pointer to the DCMI handle 00494 */ 00495 void HAL_DCMI_VsyncEventCallback(DCMI_HandleTypeDef *hdcmi) 00496 { 00497 BSP_CAMERA_VsyncEventCallback(); 00498 } 00499 00500 /** 00501 * @brief VSYNC Event callback. 00502 */ 00503 __weak void BSP_CAMERA_VsyncEventCallback(void) 00504 { 00505 /* NOTE : This function Should not be modified, when the callback is needed, 00506 the HAL_DCMI_VsyncEventCallback could be implemented in the user file 00507 */ 00508 } 00509 00510 /** 00511 * @brief Frame event callback 00512 * @param hdcmi: pointer to the DCMI handle 00513 */ 00514 void HAL_DCMI_FrameEventCallback(DCMI_HandleTypeDef *hdcmi) 00515 { 00516 BSP_CAMERA_FrameEventCallback(); 00517 } 00518 00519 /** 00520 * @brief Frame Event callback. 00521 */ 00522 __weak void BSP_CAMERA_FrameEventCallback(void) 00523 { 00524 /* NOTE : This function Should not be modified, when the callback is needed, 00525 the HAL_DCMI_FrameEventCallback could be implemented in the user file 00526 */ 00527 } 00528 00529 /** 00530 * @brief Error callback 00531 * @param hdcmi: pointer to the DCMI handle 00532 */ 00533 void HAL_DCMI_ErrorCallback(DCMI_HandleTypeDef *hdcmi) 00534 { 00535 BSP_CAMERA_ErrorCallback(); 00536 } 00537 00538 /** 00539 * @brief Error callback. 00540 */ 00541 __weak void BSP_CAMERA_ErrorCallback(void) 00542 { 00543 /* NOTE : This function Should not be modified, when the callback is needed, 00544 the HAL_DCMI_ErrorCallback could be implemented in the user file 00545 */ 00546 } 00547 00548 /** 00549 * @} 00550 */ 00551 00552 /** 00553 * @} 00554 */ 00555 00556 /** 00557 * @} 00558 */ 00559 00560 /** 00561 * @} 00562 */ 00563 00564 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Wed Jan 13 2016 15:52:54 for STM324x9I_EVAL BSP User Manual by
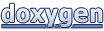