STM32469I_EVAL BSP User Manual
|
stm32469i_eval_lcd.h
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32469i_eval_lcd.h 00004 * @author MCD Application Team 00005 * @version V1.0.2 00006 * @date 12-January-2016 00007 * @brief This file contains the common defines and functions prototypes for 00008 * the stm32469i_eval_lcd.c driver. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* Define to prevent recursive inclusion -------------------------------------*/ 00040 #ifndef __STM32469I_EVAL_LCD_H 00041 #define __STM32469I_EVAL_LCD_H 00042 00043 #ifdef __cplusplus 00044 extern "C" { 00045 #endif 00046 00047 /* Includes ------------------------------------------------------------------*/ 00048 /* Include LCD component Driver */ 00049 00050 /* Include OTM8009A LCD Driver IC driver code */ 00051 #include "../Components/otm8009a/otm8009a.h" 00052 00053 /* Include SDRAM Driver */ 00054 #include "stm32469i_eval_sdram.h" 00055 #include "stm32469i_eval.h" 00056 00057 #include "../../../Utilities/Fonts/fonts.h" 00058 00059 #include <string.h> /* use of memset() */ 00060 00061 /** @addtogroup BSP 00062 * @{ 00063 */ 00064 00065 /** @addtogroup STM32469I_EVAL 00066 * @{ 00067 */ 00068 00069 /** @addtogroup STM32469I_EVAL_LCD STM32469I EVAL LCD 00070 * @{ 00071 */ 00072 00073 /** @defgroup STM32469I_EVAL_LCD_Exported_Constants STM32469I EVAL LCD Exported Constants 00074 * @{ 00075 */ 00076 00077 #define LCD_LayerCfgTypeDef LTDC_LayerCfgTypeDef 00078 /** 00079 * @brief LCD FB_StartAddress 00080 */ 00081 #define LCD_FB_START_ADDRESS ((uint32_t)0xC0000000) 00082 00083 /** @brief Maximum number of LTDC layers 00084 */ 00085 #define LTDC_MAX_LAYER_NUMBER ((uint32_t) 2) 00086 00087 /** @brief LTDC Background layer index 00088 */ 00089 #define LTDC_ACTIVE_LAYER_BACKGROUND ((uint32_t) 0) 00090 00091 /** @brief LTDC Foreground layer index 00092 */ 00093 #define LTDC_ACTIVE_LAYER_FOREGROUND ((uint32_t) 1) 00094 00095 /** @brief Number of LTDC layers 00096 */ 00097 #define LTDC_NB_OF_LAYERS ((uint32_t) 2) 00098 00099 /** @brief LTDC Default used layer index 00100 */ 00101 #define LTDC_DEFAULT_ACTIVE_LAYER LTDC_ACTIVE_LAYER_FOREGROUND 00102 00103 /** 00104 * @brief LCD status structure definition 00105 */ 00106 #define LCD_OK 0x00 00107 #define LCD_ERROR 0x01 00108 #define LCD_TIMEOUT 0x02 00109 00110 /** 00111 * @brief LCD Display OTM8009A ID 00112 */ 00113 #define LCD_OTM8009A_ID ((uint32_t) 0) 00114 00115 /** 00116 * @brief LCD color definitions values 00117 * in ARGB8888 format. 00118 */ 00119 00120 /** @brief Blue value in ARGB8888 format 00121 */ 00122 #define LCD_COLOR_BLUE ((uint32_t) 0xFF0000FF) 00123 00124 /** @brief Green value in ARGB8888 format 00125 */ 00126 #define LCD_COLOR_GREEN ((uint32_t) 0xFF00FF00) 00127 00128 /** @brief Red value in ARGB8888 format 00129 */ 00130 #define LCD_COLOR_RED ((uint32_t) 0xFFFF0000) 00131 00132 /** @brief Cyan value in ARGB8888 format 00133 */ 00134 #define LCD_COLOR_CYAN ((uint32_t) 0xFF00FFFF) 00135 00136 /** @brief Magenta value in ARGB8888 format 00137 */ 00138 #define LCD_COLOR_MAGENTA ((uint32_t) 0xFFFF00FF) 00139 00140 /** @brief Yellow value in ARGB8888 format 00141 */ 00142 #define LCD_COLOR_YELLOW ((uint32_t) 0xFFFFFF00) 00143 00144 /** @brief Light Blue value in ARGB8888 format 00145 */ 00146 #define LCD_COLOR_LIGHTBLUE ((uint32_t) 0xFF8080FF) 00147 00148 /** @brief Light Green value in ARGB8888 format 00149 */ 00150 #define LCD_COLOR_LIGHTGREEN ((uint32_t) 0xFF80FF80) 00151 00152 /** @brief Light Red value in ARGB8888 format 00153 */ 00154 #define LCD_COLOR_LIGHTRED ((uint32_t) 0xFFFF8080) 00155 00156 /** @brief Light Cyan value in ARGB8888 format 00157 */ 00158 #define LCD_COLOR_LIGHTCYAN ((uint32_t) 0xFF80FFFF) 00159 00160 /** @brief Light Magenta value in ARGB8888 format 00161 */ 00162 #define LCD_COLOR_LIGHTMAGENTA ((uint32_t) 0xFFFF80FF) 00163 00164 /** @brief Light Yellow value in ARGB8888 format 00165 */ 00166 #define LCD_COLOR_LIGHTYELLOW ((uint32_t) 0xFFFFFF80) 00167 00168 /** @brief Dark Blue value in ARGB8888 format 00169 */ 00170 #define LCD_COLOR_DARKBLUE ((uint32_t) 0xFF000080) 00171 00172 /** @brief Light Dark Green value in ARGB8888 format 00173 */ 00174 #define LCD_COLOR_DARKGREEN ((uint32_t) 0xFF008000) 00175 00176 /** @brief Light Dark Red value in ARGB8888 format 00177 */ 00178 #define LCD_COLOR_DARKRED ((uint32_t) 0xFF800000) 00179 00180 /** @brief Dark Cyan value in ARGB8888 format 00181 */ 00182 #define LCD_COLOR_DARKCYAN ((uint32_t) 0xFF008080) 00183 00184 /** @brief Dark Magenta value in ARGB8888 format 00185 */ 00186 #define LCD_COLOR_DARKMAGENTA ((uint32_t) 0xFF800080) 00187 00188 /** @brief Dark Yellow value in ARGB8888 format 00189 */ 00190 #define LCD_COLOR_DARKYELLOW ((uint32_t) 0xFF808000) 00191 00192 /** @brief White value in ARGB8888 format 00193 */ 00194 #define LCD_COLOR_WHITE ((uint32_t) 0xFFFFFFFF) 00195 00196 /** @brief Light Gray value in ARGB8888 format 00197 */ 00198 #define LCD_COLOR_LIGHTGRAY ((uint32_t) 0xFFD3D3D3) 00199 00200 /** @brief Gray value in ARGB8888 format 00201 */ 00202 #define LCD_COLOR_GRAY ((uint32_t) 0xFF808080) 00203 00204 /** @brief Dark Gray value in ARGB8888 format 00205 */ 00206 #define LCD_COLOR_DARKGRAY ((uint32_t) 0xFF404040) 00207 00208 /** @brief Black value in ARGB8888 format 00209 */ 00210 #define LCD_COLOR_BLACK ((uint32_t) 0xFF000000) 00211 00212 /** @brief Brown value in ARGB8888 format 00213 */ 00214 #define LCD_COLOR_BROWN ((uint32_t) 0xFFA52A2A) 00215 00216 /** @brief Orange value in ARGB8888 format 00217 */ 00218 #define LCD_COLOR_ORANGE ((uint32_t) 0xFFFFA500) 00219 00220 /** @brief Transparent value in ARGB8888 format 00221 */ 00222 #define LCD_COLOR_TRANSPARENT ((uint32_t) 0xFF000000) 00223 00224 /** 00225 * @brief LCD default font 00226 */ 00227 #define LCD_DEFAULT_FONT Font24 00228 /** 00229 * @} 00230 */ 00231 00232 /** @defgroup STM32469I-EVAL_LCD_Exported_Types STM32469I EVAL LCD Exported Types 00233 * @{ 00234 */ 00235 00236 /** 00237 * @brief LCD Drawing main properties 00238 */ 00239 typedef struct 00240 { 00241 uint32_t TextColor; /*!< Specifies the color of text */ 00242 uint32_t BackColor; /*!< Specifies the background color below the text */ 00243 sFONT *pFont; /*!< Specifies the font used for the text */ 00244 00245 } LCD_DrawPropTypeDef; 00246 00247 /** 00248 * @brief LCD Drawing point (pixel) geometric definition 00249 */ 00250 typedef struct 00251 { 00252 int16_t X; /*!< geometric X position of drawing */ 00253 int16_t Y; /*!< geometric Y position of drawing */ 00254 00255 } Point; 00256 00257 /** 00258 * @brief Pointer on LCD Drawing point (pixel) geometric definition 00259 */ 00260 typedef Point * pPoint; 00261 00262 /** 00263 * @brief LCD drawing Line alignment mode definitions 00264 */ 00265 typedef enum 00266 { 00267 CENTER_MODE = 0x01, /*!< Center mode */ 00268 RIGHT_MODE = 0x02, /*!< Right mode */ 00269 LEFT_MODE = 0x03 /*!< Left mode */ 00270 00271 } Text_AlignModeTypdef; 00272 00273 00274 /** 00275 * @brief LCD_OrientationTypeDef 00276 * Possible values of Display Orientation 00277 */ 00278 typedef enum 00279 { 00280 LCD_ORIENTATION_PORTRAIT = 0x00, /*!< Portrait orientation choice of LCD screen */ 00281 LCD_ORIENTATION_LANDSCAPE = 0x01, /*!< Landscape orientation choice of LCD screen */ 00282 LCD_ORIENTATION_INVALID = 0x02 /*!< Invalid orientation choice of LCD screen */ 00283 } LCD_OrientationTypeDef; 00284 00285 /** 00286 * @brief Possible values of 00287 * pixel data format (ie color coding) transmitted on DSI Data lane in DSI packets 00288 */ 00289 typedef enum 00290 { 00291 LCD_DSI_PIXEL_DATA_FMT_RBG888 = 0x00, /*!< DSI packet pixel format chosen is RGB888 : 24 bpp */ 00292 LCD_DSI_PIXEL_DATA_FMT_RBG565 = 0x02, /*!< DSI packet pixel format chosen is RGB565 : 16 bpp */ 00293 LCD_DSI_PIXEL_DATA_FMT_INVALID = 0x03 /*!< Invalid DSI packet pixel format */ 00294 00295 } LCD_DsiPixelDataFmtTypeDef; 00296 00297 /** 00298 * @} 00299 */ 00300 00301 /** @defgroup STM32469I-EVAL_LCD_Exported_Macro STM32469I EVAL LCD Exported Macro 00302 * @{ 00303 */ 00304 00305 /** 00306 * @} 00307 */ 00308 00309 /** @addtogroup STM32469I-EVAL_LCD_Exported_Functions 00310 * @{ 00311 */ 00312 00313 void BSP_LCD_DMA2D_IRQHandler(void); 00314 void BSP_LCD_DSI_IRQHandler(void); 00315 void BSP_LCD_LTDC_IRQHandler(void); 00316 void BSP_LCD_LTDC_ER_IRQHandler(void); 00317 00318 uint8_t BSP_LCD_Init(void); 00319 uint8_t BSP_LCD_InitEx(LCD_OrientationTypeDef orientation); 00320 00321 void BSP_LCD_MspDeInit(void); 00322 void BSP_LCD_MspInit(void); 00323 void BSP_LCD_Reset(void); 00324 00325 uint32_t BSP_LCD_GetXSize(void); 00326 uint32_t BSP_LCD_GetYSize(void); 00327 void BSP_LCD_SetXSize(uint32_t imageWidthPixels); 00328 void BSP_LCD_SetYSize(uint32_t imageHeightPixels); 00329 00330 void BSP_LCD_LayerDefaultInit(uint16_t LayerIndex, uint32_t FB_Address); 00331 void BSP_LCD_SetTransparency(uint32_t LayerIndex, uint8_t Transparency); 00332 void BSP_LCD_SetLayerAddress(uint32_t LayerIndex, uint32_t Address); 00333 void BSP_LCD_SetColorKeying(uint32_t LayerIndex, uint32_t RGBValue); 00334 void BSP_LCD_ResetColorKeying(uint32_t LayerIndex); 00335 void BSP_LCD_SetLayerWindow(uint16_t LayerIndex, uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height); 00336 00337 void BSP_LCD_SelectLayer(uint32_t LayerIndex); 00338 void BSP_LCD_SetLayerVisible(uint32_t LayerIndex, FunctionalState State); 00339 00340 void BSP_LCD_SetTextColor(uint32_t Color); 00341 uint32_t BSP_LCD_GetTextColor(void); 00342 void BSP_LCD_SetBackColor(uint32_t Color); 00343 uint32_t BSP_LCD_GetBackColor(void); 00344 void BSP_LCD_SetFont(sFONT *fonts); 00345 sFONT *BSP_LCD_GetFont(void); 00346 00347 uint32_t BSP_LCD_ReadPixel(uint16_t Xpos, uint16_t Ypos); 00348 void BSP_LCD_DrawPixel(uint16_t Xpos, uint16_t Ypos, uint32_t pixel); 00349 void BSP_LCD_Clear(uint32_t Color); 00350 void BSP_LCD_ClearStringLine(uint32_t Line); 00351 void BSP_LCD_DisplayStringAtLine(uint16_t Line, uint8_t *ptr); 00352 void BSP_LCD_DisplayStringAt(uint16_t Xpos, uint16_t Ypos, uint8_t *Text, Text_AlignModeTypdef Mode); 00353 void BSP_LCD_DisplayChar(uint16_t Xpos, uint16_t Ypos, uint8_t Ascii); 00354 00355 void BSP_LCD_DrawHLine(uint16_t Xpos, uint16_t Ypos, uint16_t Length); 00356 void BSP_LCD_DrawVLine(uint16_t Xpos, uint16_t Ypos, uint16_t Length); 00357 void BSP_LCD_DrawLine(uint16_t x1, uint16_t y1, uint16_t x2, uint16_t y2); 00358 void BSP_LCD_DrawRect(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height); 00359 void BSP_LCD_DrawCircle(uint16_t Xpos, uint16_t Ypos, uint16_t Radius); 00360 void BSP_LCD_DrawPolygon(pPoint Points, uint16_t PointCount); 00361 void BSP_LCD_DrawEllipse(int Xpos, int Ypos, int XRadius, int YRadius); 00362 void BSP_LCD_DrawBitmap(uint32_t Xpos, uint32_t Ypos, uint8_t *pbmp); 00363 00364 void BSP_LCD_FillRect(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height); 00365 void BSP_LCD_FillCircle(uint16_t Xpos, uint16_t Ypos, uint16_t Radius); 00366 void BSP_LCD_FillPolygon(pPoint Points, uint16_t PointCount); 00367 void BSP_LCD_FillEllipse(int Xpos, int Ypos, int XRadius, int YRadius); 00368 00369 void BSP_LCD_DisplayOff(void); 00370 void BSP_LCD_DisplayOn(void); 00371 00372 /** 00373 * @} 00374 */ 00375 00376 /** @addtogroup STM32469I-EVAL_LCD_Exported_Variables 00377 * @{ 00378 */ 00379 00380 /* @brief DMA2D handle variable */ 00381 extern DMA2D_HandleTypeDef hdma2d_eval; 00382 00383 /** 00384 * @} 00385 */ 00386 00387 /** 00388 * @} 00389 */ 00390 00391 /** 00392 * @} 00393 */ 00394 00395 /** 00396 * @} 00397 */ 00398 00399 #ifdef __cplusplus 00400 } 00401 #endif 00402 00403 #endif /* __STM32469I_EVAL_LCD_H */ 00404 00405 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Tue Jan 12 2016 17:51:25 for STM32469I_EVAL BSP User Manual by
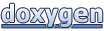