STM32469I_EVAL BSP User Manual
|
stm32469i_eval.h
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32469i_eval.h 00004 * @author MCD Application Team 00005 * @version V1.0.2 00006 * @date 12-January-2016 00007 * @brief This file contains definitions for STM32469I-EVAL's LEDs, 00008 * push-buttons and COM ports hardware resources. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* Define to prevent recursive inclusion -------------------------------------*/ 00040 #ifndef __STM32469I_EVAL_H 00041 #define __STM32469I_EVAL_H 00042 00043 #ifdef __cplusplus 00044 extern "C" { 00045 #endif 00046 00047 /* USE_STM32469I_EVAL_REVA must USE USE_IOEXPANDER */ 00048 #if defined(USE_STM32469I_EVAL_REVA) 00049 #ifndef USE_IOEXPANDER 00050 #define USE_IOEXPANDER 00051 #endif /* USE_IOEXPANDER */ 00052 #endif /* USE_STM32469I_EVAL_REVA */ 00053 00054 /* Includes ------------------------------------------------------------------*/ 00055 #include "stm32f4xx_hal.h" 00056 00057 /** @addtogroup BSP 00058 * @{ 00059 */ 00060 00061 00062 /** @addtogroup STM32469I_EVAL 00063 * @{ 00064 */ 00065 00066 /** @defgroup STM32469I_EVAL_LOW_LEVEL STM32469I EVAL LOW LEVEL 00067 * @{ 00068 */ 00069 00070 /** @defgroup STM32469I_EVAL_LOW_LEVEL_Exported_Types STM32469I EVAL LOW LEVEL Exported Types 00071 * @{ 00072 */ 00073 00074 /** @brief Led_TypeDef 00075 * STM32469I_EVAL board leds definitions. 00076 */ 00077 typedef enum 00078 { 00079 LED1 = 0, 00080 LED_GREEN = LED1, 00081 LED2 = 1, 00082 LED_ORANGE = LED2, 00083 LED3 = 2, 00084 LED_RED = LED3, 00085 LED4 = 3, 00086 LED_BLUE = LED4 00087 00088 } Led_TypeDef; 00089 00090 /** @brief Button_TypeDef 00091 * STM32469I_EVAL board Buttons definitions. 00092 */ 00093 typedef enum 00094 { 00095 BUTTON_WAKEUP = 0, 00096 BUTTON_TAMPER = 1, 00097 BUTTON_KEY = 2 00098 00099 } Button_TypeDef; 00100 00101 /** @brief ButtonMode_TypeDef 00102 * STM32469I_EVAL board Buttons Modes definitions. 00103 */ 00104 typedef enum 00105 { 00106 BUTTON_MODE_GPIO = 0, 00107 BUTTON_MODE_EXTI = 1 00108 00109 } ButtonMode_TypeDef; 00110 00111 #if defined(USE_IOEXPANDER) 00112 /** @brief JOYMode_TypeDef 00113 * STM32469I_EVAL board Joystick Mode definitions. 00114 */ 00115 typedef enum 00116 { 00117 JOY_MODE_GPIO = 0, 00118 JOY_MODE_EXTI = 1 00119 00120 } JOYMode_TypeDef; 00121 00122 /** @brief JOYState_TypeDef 00123 * STM32469I_EVAL board Joystick State definitions. 00124 */ 00125 typedef enum 00126 { 00127 JOY_NONE = 0, 00128 JOY_SEL = 1, 00129 JOY_DOWN = 2, 00130 JOY_LEFT = 3, 00131 JOY_RIGHT = 4, 00132 JOY_UP = 5 00133 00134 } JOYState_TypeDef; 00135 #endif /* USE_IOEXPANDER */ 00136 00137 /** @brief COM_TypeDef 00138 * STM32469I_EVAL board COM ports. 00139 */ 00140 typedef enum 00141 { 00142 COM1 = 0, 00143 COM2 = 1 00144 00145 } COM_TypeDef; 00146 00147 /** @brief EVAL_Status_TypeDef 00148 * STM32469I_EVAL board Eval Status return possible values. 00149 */ 00150 typedef enum 00151 { 00152 EVAL_OK = 0, 00153 EVAL_ERROR = 1 00154 00155 } EVAL_Status_TypeDef; 00156 00157 /** 00158 * @} 00159 */ 00160 00161 /** @defgroup STM32469I_EVAL_LOW_LEVEL_Exported_Constants STM32469I EVAL LOW LEVEL Exported Constants 00162 * @{ 00163 */ 00164 00165 /** 00166 * @brief Define for STM32469I_EVAL board 00167 */ 00168 #if !defined (USE_STM32469I_EVAL) 00169 #define USE_STM32469I_EVAL 00170 #endif 00171 00172 /** @defgroup STM32469I_EVAL_LOW_LEVEL_LED STM32469I EVAL LOW LEVEL LED 00173 * @{ 00174 */ 00175 /* Always four leds for all revisions of Eval boards */ 00176 #define LEDn ((uint8_t)4) 00177 00178 #if !defined(USE_STM32469I_EVAL_REVA) 00179 /* Rev B board and beyond : 4 Leds are connected to MCU directly and not via MFX */ 00180 /* PK3, PK4, PK5, PK6 are used */ 00181 #define LEDx_GPIO_PORT GPIOK 00182 #define LEDx_GPIO_CLK_ENABLE() __HAL_RCC_GPIOK_CLK_ENABLE() 00183 #define LEDx_GPIO_CLK_DISABLE() __HAL_RCC_GPIOK_CLK_DISABLE() 00184 #define LED1_PIN GPIO_PIN_3 00185 #define LED2_PIN GPIO_PIN_4 00186 #define LED3_PIN GPIO_PIN_5 00187 #define LED4_PIN GPIO_PIN_6 00188 #else 00189 /* Eval Rev A board */ 00190 #define LED1_PIN IO_PIN_16 00191 #define LED2_PIN IO_PIN_17 00192 #define LED3_PIN IO_PIN_18 00193 #define LED4_PIN IO_PIN_19 00194 #endif /* USE_STM32469I_EVAL_REVA */ 00195 /** 00196 * @} 00197 */ 00198 00199 /** 00200 * @brief MFX_IRQOUt pin 00201 */ 00202 #define MFX_IRQOUT_PIN GPIO_PIN_8 00203 #define MFX_IRQOUT_GPIO_PORT GPIOI 00204 #define MFX_IRQOUT_GPIO_CLK_ENABLE() __HAL_RCC_GPIOI_CLK_ENABLE() 00205 #define MFX_IRQOUT_GPIO_CLK_DISABLE() __HAL_RCC_GPIOI_CLK_DISABLE() 00206 #define MFX_IRQOUT_EXTI_IRQn EXTI9_5_IRQn 00207 #define MFX_IRQOUT_EXTI_IRQnHandler EXTI9_5_IRQHandler 00208 00209 /** @defgroup STM32469I_EVAL_LOW_LEVEL_BUTTON STM32469I EVAL LOW LEVEL BUTTON 00210 * @{ 00211 */ 00212 /* Joystick pins are connected to IO Expander (accessible through I2C1 interface) */ 00213 #define BUTTONn ((uint8_t)3) 00214 00215 /** 00216 * @brief Wakeup push-button 00217 */ 00218 #define WAKEUP_BUTTON_PIN GPIO_PIN_0 00219 #define WAKEUP_BUTTON_GPIO_PORT GPIOA 00220 #define WAKEUP_BUTTON_GPIO_CLK_ENABLE() __HAL_RCC_GPIOA_CLK_ENABLE() 00221 #define WAKEUP_BUTTON_GPIO_CLK_DISABLE() __HAL_RCC_GPIOA_CLK_DISABLE() 00222 #define WAKEUP_BUTTON_EXTI_IRQn EXTI0_IRQn 00223 00224 /** 00225 * @brief Tamper push-button 00226 */ 00227 #define TAMPER_BUTTON_PIN GPIO_PIN_13 00228 #define TAMPER_BUTTON_GPIO_PORT GPIOC 00229 #define TAMPER_BUTTON_GPIO_CLK_ENABLE() __HAL_RCC_GPIOC_CLK_ENABLE() 00230 #define TAMPER_BUTTON_GPIO_CLK_DISABLE() __HAL_RCC_GPIOC_CLK_DISABLE() 00231 #define TAMPER_BUTTON_EXTI_IRQn EXTI15_10_IRQn 00232 00233 /** 00234 * @brief Key push-button 00235 */ 00236 #define KEY_BUTTON_PIN GPIO_PIN_13 00237 #define KEY_BUTTON_GPIO_PORT GPIOC 00238 #define KEY_BUTTON_GPIO_CLK_ENABLE() __HAL_RCC_GPIOC_CLK_ENABLE() 00239 #define KEY_BUTTON_GPIO_CLK_DISABLE() __HAL_RCC_GPIOC_CLK_DISABLE() 00240 #define KEY_BUTTON_EXTI_IRQn EXTI15_10_IRQn 00241 00242 #define BUTTONx_GPIO_CLK_ENABLE(__INDEX__) do { if((__INDEX__) == 0) {WAKEUP_BUTTON_GPIO_CLK_ENABLE();} else\ 00243 if((__INDEX__) == 1) {TAMPER_BUTTON_GPIO_CLK_ENABLE();} else\ 00244 {KEY_BUTTON_GPIO_CLK_ENABLE(); }} while(0) 00245 00246 #define BUTTONx_GPIO_CLK_DISABLE(__INDEX__) (((__INDEX__) == 0) ? WAKEUP_BUTTON_GPIO_CLK_DISABLE() :\ 00247 ((__INDEX__) == 1) ? TAMPER_BUTTON_GPIO_CLK_DISABLE() : KEY_BUTTON_GPIO_CLK_DISABLE()) 00248 /** 00249 * @} 00250 */ 00251 00252 /** @defgroup STM32469I_EVAL_LOW_LEVEL_COM STM32469I EVAL LOW LEVEL COM 00253 * @{ 00254 */ 00255 #define COMn ((uint8_t)1) 00256 00257 /** 00258 * @brief Definition for COM port1, connected to USART1 00259 */ 00260 #define EVAL_COM1 USART1 00261 #define EVAL_COM1_CLK_ENABLE() __HAL_RCC_USART1_CLK_ENABLE() 00262 #define EVAL_COM1_CLK_DISABLE() __HAL_RCC_USART1_CLK_DISABLE() 00263 00264 #define EVAL_COM1_TX_PIN GPIO_PIN_9 00265 #define EVAL_COM1_RX_PIN GPIO_PIN_10 00266 #define EVAL_COM1_TX_GPIO_PORT GPIOA 00267 #define EVAL_COM1_RX_GPIO_PORT EVAL_COM1_TX_GPIO_PORT 00268 00269 #define EVAL_COM1_TX_AF GPIO_AF7_USART1 00270 #define EVAL_COM1_RX_AF EVAL_COM1_TX_AF 00271 00272 #define EVAL_COM1_TX_RX_GPIO_CLK_ENABLE() __HAL_RCC_GPIOA_CLK_ENABLE() 00273 #define EVAL_COM1_TX_RX_GPIO_CLK_DISABLE() __HAL_RCC_GPIOA_CLK_DISABLE() 00274 00275 #define EVAL_COM1_IRQn USART1_IRQn 00276 00277 #define EVAL_COMx_CLK_ENABLE(__INDEX__) do { if((__INDEX__) == 0) {EVAL_COM1_CLK_ENABLE();} } while(0) 00278 #define EVAL_COMx_CLK_DISABLE(__INDEX__) (((__INDEX__) == 0) ? EVAL_COM1_CLK_DISABLE() : 0) 00279 00280 #define EVAL_COMx_TX_RX_GPIO_CLK_ENABLE(__INDEX__) do { if((__INDEX__) == 0) {EVAL_COM1_TX_RX_GPIO_CLK_ENABLE();} } while(0) 00281 #define EVAL_COMx_TX_RX_GPIO_CLK_DISABLE(__INDEX__) (((__INDEX__) == 0) ? EVAL_COM1_TX_RX_GPIO_CLK_DISABLE() : 0) 00282 00283 /** 00284 * @brief Joystick Pins definition 00285 */ 00286 #if defined(USE_IOEXPANDER) 00287 #define JOY_SEL_PIN IO_PIN_0 00288 #define JOY_DOWN_PIN IO_PIN_1 00289 #define JOY_LEFT_PIN IO_PIN_2 00290 #define JOY_RIGHT_PIN IO_PIN_3 00291 #define JOY_UP_PIN IO_PIN_4 00292 #define JOY_NONE_PIN JOY_ALL_PINS 00293 #define JOY_ALL_PINS (IO_PIN_0 | IO_PIN_1 | IO_PIN_2 | IO_PIN_3 | IO_PIN_4) 00294 #endif /* USE_IOEXPANDER */ 00295 00296 /** 00297 * @brief Eval Pins definition 00298 */ 00299 #if defined(USE_IOEXPANDER) 00300 #define XSDN_PIN IO_PIN_10 00301 #define MII_INT_PIN IO_PIN_13 00302 #define RSTI_PIN IO_PIN_11 00303 #define CAM_PLUG_PIN IO_PIN_12 00304 #define TS_INT_PIN IO_PIN_14 00305 #define AUDIO_INT_PIN IO_PIN_5 00306 #define OTG_FS1_OVER_CURRENT_PIN IO_PIN_6 00307 #define OTG_FS1_POWER_SWITCH_PIN IO_PIN_7 00308 #define OTG_FS2_OVER_CURRENT_PIN IO_PIN_8 00309 #define OTG_FS2_POWER_SWITCH_PIN IO_PIN_9 00310 #define SD_DETECT_PIN IO_PIN_15 00311 #endif /* USE_IOEXPANDER */ 00312 00313 /* Exported constant IO ------------------------------------------------------*/ 00314 00315 /** 00316 * @brief TouchScreen FT6206 Slave I2C address 00317 */ 00318 #define TS_I2C_ADDRESS ((uint16_t)0x54) 00319 00320 /** 00321 * @brief MFX_I2C_ADDR 0 00322 */ 00323 #define IO_I2C_ADDRESS ((uint16_t)0x84) 00324 00325 /** 00326 * @brief Camera I2C Slave address 00327 */ 00328 #define CAMERA_I2C_ADDRESS ((uint16_t)0x5A) 00329 00330 /** 00331 * @brief Audio I2C Slave address 00332 */ 00333 #define AUDIO_I2C_ADDRESS ((uint16_t)0x34) 00334 00335 /** 00336 * @brief EEPROM I2C Slave address 1 00337 */ 00338 #define EEPROM_I2C_ADDRESS_A01 ((uint16_t)0xA0) 00339 00340 /** 00341 * @brief EEPROM I2C Slave address 2 00342 */ 00343 #define EEPROM_I2C_ADDRESS_A02 ((uint16_t)0xA6) 00344 00345 /** 00346 * @brief I2C clock speed configuration (in Hz) 00347 * WARNING: 00348 * Make sure that this define is not already declared in other files 00349 * It can be used in parallel by other modules. 00350 */ 00351 #ifndef I2C_SCL_FREQ_KHZ 00352 #define I2C_SCL_FREQ_KHZ 400000 /*!< f(I2C_SCL) = 400 kHz */ 00353 #endif /* I2C_SCL_FREQ_KHZ */ 00354 00355 /** 00356 * @brief User can use this section to tailor I2Cx/I2Cx instance used and associated 00357 * resources. 00358 * Definition for I2Cx clock resources 00359 */ 00360 #define EVAL_I2Cx I2C1 00361 #define EVAL_I2Cx_CLK_ENABLE() __HAL_RCC_I2C1_CLK_ENABLE() 00362 #define EVAL_DMAx_CLK_ENABLE() __HAL_RCC_DMA1_CLK_ENABLE() 00363 #define EVAL_I2Cx_SCL_SDA_GPIO_CLK_ENABLE() __HAL_RCC_GPIOB_CLK_ENABLE() 00364 00365 #define EVAL_I2Cx_FORCE_RESET() __HAL_RCC_I2C1_FORCE_RESET() 00366 #define EVAL_I2Cx_RELEASE_RESET() __HAL_RCC_I2C1_RELEASE_RESET() 00367 00368 /** @brief Definition for I2Cx Pins 00369 */ 00370 #define EVAL_I2Cx_SCL_PIN GPIO_PIN_8 /*!< PB8 */ 00371 #define EVAL_I2Cx_SCL_SDA_GPIO_PORT GPIOB 00372 #define EVAL_I2Cx_SCL_SDA_AF GPIO_AF4_I2C1 00373 #define EVAL_I2Cx_SDA_PIN GPIO_PIN_9 /*!< PB9 */ 00374 00375 /** @brief Definition of I2C interrupt requests 00376 */ 00377 #define EVAL_I2Cx_EV_IRQn I2C1_EV_IRQn 00378 #define EVAL_I2Cx_ER_IRQn I2C1_ER_IRQn 00379 00380 /** 00381 * @} 00382 */ 00383 00384 /** 00385 * @} 00386 */ 00387 00388 /** @defgroup STM32469I_EVAL_LOW_LEVEL_Exported_Macros STM32469I EVAL LOW LEVEL Exported Macros 00389 * @{ 00390 */ 00391 /** 00392 * @} 00393 */ 00394 00395 /** @defgroup STM32469I_EVAL_LOW_LEVEL_Exported_Functions STM32469I EVAL LOW LEVEL Exported Functions 00396 * @{ 00397 */ 00398 uint32_t BSP_GetVersion(void); 00399 void BSP_LED_Init(Led_TypeDef Led); 00400 void BSP_LED_DeInit(Led_TypeDef Led); 00401 void BSP_LED_On(Led_TypeDef Led); 00402 void BSP_LED_Off(Led_TypeDef Led); 00403 void BSP_LED_Toggle(Led_TypeDef Led); 00404 void BSP_PB_Init(Button_TypeDef Button, ButtonMode_TypeDef Button_Mode); 00405 void BSP_PB_DeInit(Button_TypeDef Button); 00406 uint32_t BSP_PB_GetState(Button_TypeDef Button); 00407 void BSP_COM_Init(COM_TypeDef COM, UART_HandleTypeDef *husart); 00408 void BSP_COM_DeInit(COM_TypeDef COM, UART_HandleTypeDef *huart); 00409 #if defined(USE_IOEXPANDER) 00410 uint8_t BSP_JOY_Init(JOYMode_TypeDef Joy_Mode); 00411 void BSP_JOY_DeInit(void); 00412 JOYState_TypeDef BSP_JOY_GetState(void); 00413 #endif /* USE_IOEXPANDER */ 00414 00415 /** 00416 * @} 00417 */ 00418 00419 /** 00420 * @} 00421 */ 00422 00423 /** 00424 * @} 00425 */ 00426 00427 /** 00428 * @} 00429 */ 00430 00431 00432 #ifdef __cplusplus 00433 } 00434 #endif 00435 00436 #endif /* __STM32469I_EVAL_H */ 00437 00438 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Tue Jan 12 2016 17:51:25 for STM32469I_EVAL BSP User Manual by
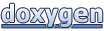