STM32469I_EVAL BSP User Manual
|
stm32469i_eval.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32469i_eval.c 00004 * @author MCD Application Team 00005 * @version V1.0.2 00006 * @date 12-January-2016 00007 * @brief This file provides a set of firmware functions to manage LEDs, 00008 * push-buttons and COM ports available on STM32469I-EVAL evaluation 00009 * board(MB1165) RevA/B from STMicroelectronics. 00010 ****************************************************************************** 00011 * @attention 00012 * 00013 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00014 * 00015 * Redistribution and use in source and binary forms, with or without modification, 00016 * are permitted provided that the following conditions are met: 00017 * 1. Redistributions of source code must retain the above copyright notice, 00018 * this list of conditions and the following disclaimer. 00019 * 2. Redistributions in binary form must reproduce the above copyright notice, 00020 * this list of conditions and the following disclaimer in the documentation 00021 * and/or other materials provided with the distribution. 00022 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00023 * may be used to endorse or promote products derived from this software 00024 * without specific prior written permission. 00025 * 00026 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00027 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00028 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00029 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00030 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00031 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00032 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00033 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00034 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00035 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00036 * 00037 ****************************************************************************** 00038 */ 00039 00040 /* File Info: ------------------------------------------------------------------ 00041 User NOTE 00042 00043 This driver requires the stm32469i_eval_io to manage the joystick 00044 00045 ------------------------------------------------------------------------------*/ 00046 00047 /* Includes ------------------------------------------------------------------*/ 00048 #include "stm32469i_eval.h" 00049 #if defined(USE_IOEXPANDER) 00050 #include "stm32469i_eval_io.h" 00051 #endif /* USE_IOEXPANDER */ 00052 00053 /** @defgroup BSP BSP 00054 * @{ 00055 */ 00056 00057 /** @defgroup STM32469I_EVAL STM32469I EVAL 00058 * @{ 00059 */ 00060 00061 /** @defgroup STM32469I_EVAL_LOW_LEVEL STM32469I EVAL LOW LEVEL 00062 * @{ 00063 */ 00064 00065 /** @defgroup STM32469I_EVAL_LOW_LEVEL_Private_TypesDefinitions STM32469I EVAL LOW LEVEL Private TypesDefinitions 00066 * @{ 00067 */ 00068 /** 00069 * @} 00070 */ 00071 00072 /** @defgroup STM32469I_EVAL_LOW_LEVEL_Private_Defines STM32469I EVAL LOW LEVEL Private Defines 00073 * @{ 00074 */ 00075 /** 00076 * @brief STM32469I EVAL BSP Driver version number V1.0.2 00077 */ 00078 #define __STM32469I_EVAL_BSP_VERSION_MAIN (0x01) /*!< [31:24] main version */ 00079 #define __STM32469I_EVAL_BSP_VERSION_SUB1 (0x00) /*!< [23:16] sub1 version */ 00080 #define __STM32469I_EVAL_BSP_VERSION_SUB2 (0x02) /*!< [15:8] sub2 version */ 00081 #define __STM32469I_EVAL_BSP_VERSION_RC (0x00) /*!< [7:0] release candidate */ 00082 #define __STM32469I_EVAL_BSP_VERSION ((__STM32469I_EVAL_BSP_VERSION_MAIN << 24)\ 00083 |(__STM32469I_EVAL_BSP_VERSION_SUB1 << 16)\ 00084 |(__STM32469I_EVAL_BSP_VERSION_SUB2 << 8 )\ 00085 |(__STM32469I_EVAL_BSP_VERSION_RC)) 00086 /** 00087 * @} 00088 */ 00089 00090 /** @defgroup STM32469I_EVAL_LOW_LEVEL_Private_Macros STM32469I EVAL LOW LEVEL Private Macros 00091 * @{ 00092 */ 00093 /** 00094 * @} 00095 */ 00096 00097 /** @defgroup STM32469I_EVAL_LOW_LEVEL_Private_Variables STM32469I EVAL LOW LEVEL Private Variables 00098 * @{ 00099 */ 00100 const uint32_t GPIO_PIN[LEDn] = {LED1_PIN, 00101 LED2_PIN, 00102 LED3_PIN, 00103 LED4_PIN}; 00104 00105 GPIO_TypeDef* BUTTON_PORT[BUTTONn] = {WAKEUP_BUTTON_GPIO_PORT, 00106 TAMPER_BUTTON_GPIO_PORT, 00107 KEY_BUTTON_GPIO_PORT}; 00108 00109 const uint16_t BUTTON_PIN[BUTTONn] = {WAKEUP_BUTTON_PIN, 00110 TAMPER_BUTTON_PIN, 00111 KEY_BUTTON_PIN}; 00112 00113 const uint16_t BUTTON_IRQn[BUTTONn] = {WAKEUP_BUTTON_EXTI_IRQn, 00114 TAMPER_BUTTON_EXTI_IRQn, 00115 KEY_BUTTON_EXTI_IRQn}; 00116 00117 USART_TypeDef* COM_USART[COMn] = {EVAL_COM1}; 00118 00119 GPIO_TypeDef* COM_TX_PORT[COMn] = {EVAL_COM1_TX_GPIO_PORT}; 00120 00121 GPIO_TypeDef* COM_RX_PORT[COMn] = {EVAL_COM1_RX_GPIO_PORT}; 00122 00123 const uint16_t COM_TX_PIN[COMn] = {EVAL_COM1_TX_PIN}; 00124 00125 const uint16_t COM_RX_PIN[COMn] = {EVAL_COM1_RX_PIN}; 00126 00127 const uint16_t COM_TX_AF[COMn] = {EVAL_COM1_TX_AF}; 00128 00129 const uint16_t COM_RX_AF[COMn] = {EVAL_COM1_RX_AF}; 00130 00131 static I2C_HandleTypeDef heval_I2c; 00132 00133 /** 00134 * @} 00135 */ 00136 00137 /** @defgroup STM32469I_EVAL_LOW_LEVEL_Private_FunctionPrototypes STM32469I EVAL LOW LEVEL Private FunctionPrototypes 00138 * @{ 00139 */ 00140 static void I2Cx_MspInit(void); 00141 static void I2Cx_Init(void); 00142 static void I2Cx_Write(uint8_t Addr, uint8_t Reg, uint8_t Value); 00143 static uint8_t I2Cx_Read(uint8_t Addr, uint8_t Reg); 00144 static HAL_StatusTypeDef I2Cx_ReadMultiple(uint8_t Addr, uint16_t Reg, uint16_t MemAddSize, uint8_t *Buffer, uint16_t Length); 00145 static HAL_StatusTypeDef I2Cx_WriteMultiple(uint8_t Addr, uint16_t Reg, uint16_t MemAddSize, uint8_t *Buffer, uint16_t Length); 00146 static HAL_StatusTypeDef I2Cx_IsDeviceReady(uint16_t DevAddress, uint32_t Trials); 00147 static void I2Cx_Error(uint8_t Addr); 00148 00149 /* IOExpander IO functions */ 00150 void IOE_Init(void); 00151 void IOE_ITConfig(void); 00152 void IOE_Delay(uint32_t Delay); 00153 void IOE_Write(uint8_t Addr, uint8_t Reg, uint8_t Value); 00154 uint8_t IOE_Read(uint8_t Addr, uint8_t Reg); 00155 uint16_t IOE_ReadMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length); 00156 void IOE_WriteMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length); 00157 00158 #if defined(USE_IOEXPANDER) 00159 /* MFX IO functions */ 00160 void MFX_IO_Init(void); 00161 void MFX_IO_DeInit(void); 00162 void MFX_IO_ITConfig(void); 00163 void MFX_IO_Delay(uint32_t Delay); 00164 void MFX_IO_Write(uint16_t Addr, uint8_t Reg, uint8_t Value); 00165 uint8_t MFX_IO_Read(uint16_t Addr, uint8_t Reg); 00166 uint16_t MFX_IO_ReadMultiple(uint16_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length); 00167 void MFX_IO_Wakeup(void); 00168 void MFX_IO_EnableWakeupPin(void); 00169 #endif /* USE_IOEXPANDER */ 00170 00171 /* AUDIO IO functions */ 00172 void AUDIO_IO_Init(void); 00173 void AUDIO_IO_DeInit(void); 00174 void AUDIO_IO_Write(uint8_t Addr, uint16_t Reg, uint16_t Value); 00175 uint16_t AUDIO_IO_Read(uint8_t Addr, uint16_t Reg); 00176 void AUDIO_IO_Delay(uint32_t Delay); 00177 00178 /* CAMERA IO functions */ 00179 void CAMERA_IO_Init(void); 00180 void CAMERA_Delay(uint32_t Delay); 00181 void CAMERA_IO_Write(uint8_t Addr, uint16_t Reg, uint16_t Value); 00182 uint16_t CAMERA_IO_Read(uint8_t Addr, uint16_t Reg); 00183 00184 /* I2C EEPROM IO function */ 00185 void EEPROM_IO_Init(void); 00186 HAL_StatusTypeDef EEPROM_IO_WriteData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize); 00187 HAL_StatusTypeDef EEPROM_IO_ReadData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize); 00188 HAL_StatusTypeDef EEPROM_IO_IsDeviceReady(uint16_t DevAddress, uint32_t Trials); 00189 00190 /* TouchScreen (TS) IO functions */ 00191 void TS_IO_Init(void); 00192 void TS_IO_Write(uint8_t Addr, uint8_t Reg, uint8_t Value); 00193 uint8_t TS_IO_Read(uint8_t Addr, uint8_t Reg); 00194 uint16_t TS_IO_ReadMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length); 00195 void TS_IO_WriteMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length); 00196 void TS_IO_Delay(uint32_t Delay); 00197 00198 void OTM8009A_IO_Delay(uint32_t Delay); 00199 /** 00200 * @} 00201 */ 00202 00203 /** @defgroup STM32469I_EVAL_BSP_Public_Functions STM32469I EVAL BSP Public Functions 00204 * @{ 00205 */ 00206 00207 /** 00208 * @brief This method returns the STM32469I EVAL BSP Driver revision 00209 * @retval version: 0xXYZR (8bits for each decimal, R for RC) 00210 */ 00211 uint32_t BSP_GetVersion(void) 00212 { 00213 return __STM32469I_EVAL_BSP_VERSION; 00214 } 00215 00216 /** 00217 * @brief Configures LED GPIO. 00218 * @param Led: LED to be configured. 00219 * This parameter can be one of the following values: 00220 * @arg LED1 00221 * @arg LED2 00222 * @arg LED3 00223 * @arg LED4 00224 */ 00225 void BSP_LED_Init(Led_TypeDef Led) 00226 { 00227 #if !defined(USE_STM32469I_EVAL_REVA) 00228 /* Eval Rev B and beyond : leds are directly connected to MCU o PK3, 4, 5, 6 */ 00229 GPIO_InitTypeDef gpio_init_structure; 00230 00231 /* On RevB LED1, LED2, LED3 and LED4 are on GPIO */ 00232 if (Led <= LED4) 00233 { 00234 /* Enable the GPIO_LED clock */ 00235 LEDx_GPIO_CLK_ENABLE(); 00236 00237 /* Configure the GPIO_LED pin */ 00238 gpio_init_structure.Pin = GPIO_PIN[Led]; 00239 gpio_init_structure.Mode = GPIO_MODE_OUTPUT_PP; 00240 gpio_init_structure.Pull = GPIO_PULLUP; 00241 gpio_init_structure.Speed = GPIO_SPEED_HIGH; 00242 00243 HAL_GPIO_Init(LEDx_GPIO_PORT, &gpio_init_structure); 00244 00245 /* By default, turn off LED by setting a high level on corresponding GPIO */ 00246 HAL_GPIO_WritePin(LEDx_GPIO_PORT, GPIO_PIN[Led], GPIO_PIN_SET); 00247 } 00248 #else 00249 #if defined(USE_IOEXPANDER) /* (USE_IOEXPANDER always defined for RevA) */ 00250 /* Initialize the IO functionalities */ 00251 BSP_IO_Init(); 00252 00253 /* the output mode choices shall be extended to PushPull / PullUp*/ 00254 BSP_IO_ConfigPin(GPIO_PIN[Led], IO_MODE_OUTPUT_PP_PU); 00255 00256 BSP_IO_WritePin(GPIO_PIN[Led], BSP_IO_PIN_SET); 00257 #endif /* USE_IOEXPANDER */ 00258 #endif /* USE_STM32469I_EVAL_REVA */ 00259 } 00260 00261 00262 /** 00263 * @brief DeInit LEDs. 00264 * @param Led: LED to be configured. 00265 * This parameter can be one of the following values: 00266 * @arg LED1 00267 * @arg LED2 00268 * @arg LED3 00269 * @arg LED4 00270 * @note Led DeInit does not disable the GPIO clock nor disable the Mfx 00271 */ 00272 void BSP_LED_DeInit(Led_TypeDef Led) 00273 { 00274 #if !defined(USE_STM32469I_EVAL_REVA) 00275 /* Eval Rev B and beyond */ 00276 GPIO_InitTypeDef gpio_init_structure; 00277 00278 /* On RevB LED1 LED2 LED3 and LED4 are on GPIO PK3, 4, 5, 6 */ 00279 if (Led <= LED4) 00280 { 00281 /* Turn off LED */ 00282 HAL_GPIO_WritePin(LEDx_GPIO_PORT, GPIO_PIN[Led], GPIO_PIN_RESET); 00283 /* DeInit the GPIO_LED pin */ 00284 gpio_init_structure.Pin = GPIO_PIN[Led]; 00285 HAL_GPIO_DeInit(LEDx_GPIO_PORT, gpio_init_structure.Pin); 00286 } 00287 #else 00288 #if defined(USE_IOEXPANDER) /* (USE_IOEXPANDER always defined for RevA) */ 00289 /* GPIO_PIN[Led] depends on the board revision: */ 00290 /* - in case of RevA all leds are on IOEXPANDER (Mfx) */ 00291 BSP_IO_ConfigPin(GPIO_PIN[Led], IO_MODE_OFF); 00292 #endif /* USE_IOEXPANDER */ 00293 #endif /* USE_STM32469I_EVAL_REVA */ 00294 } 00295 /** 00296 * @brief Turns selected LED On. 00297 * @param Led: LED to be set on 00298 * This parameter can be one of the following values: 00299 * @arg LED1 00300 * @arg LED2 00301 * @arg LED3 00302 * @arg LED4 00303 */ 00304 void BSP_LED_On(Led_TypeDef Led) 00305 { 00306 #if !defined(USE_STM32469I_EVAL_REVA) 00307 /* Eval Rev B and beyond */ 00308 /* On RevB LED1 LED2 LED3 and LED4 are on GPIO PK3, 4, 5, 6 */ 00309 if (Led <= LED4) 00310 { 00311 HAL_GPIO_WritePin(LEDx_GPIO_PORT, GPIO_PIN[Led], GPIO_PIN_RESET); 00312 } 00313 #else 00314 #if defined(USE_IOEXPANDER) /* (USE_IOEXPANDER always defined for RevA) */ 00315 /* GPIO_PIN[Led] depends on the board revision: */ 00316 /* - in case of RevA all leds are on IOEXPANDER (Mfx) */ 00317 BSP_IO_WritePin(GPIO_PIN[Led], BSP_IO_PIN_RESET); 00318 #endif /* USE_IOEXPANDER */ 00319 #endif /* USE_STM32469I_EVAL_REVA */ 00320 00321 } 00322 00323 /** 00324 * @brief Turns selected LED Off. 00325 * @param Led: LED to be set off 00326 * This parameter can be one of the following values: 00327 * @arg LED1 00328 * @arg LED2 00329 * @arg LED3 00330 * @arg LED4 00331 */ 00332 void BSP_LED_Off(Led_TypeDef Led) 00333 { 00334 #if !defined(USE_STM32469I_EVAL_REVA) 00335 /* Eval Rev B and beyond */ 00336 /* On RevB LED1 LED2 LED3 and LED4 are on GPIO PK3, 4, 5, 6 */ 00337 if (Led <= LED4) 00338 { 00339 HAL_GPIO_WritePin(LEDx_GPIO_PORT, GPIO_PIN[Led], GPIO_PIN_SET); 00340 } 00341 #else 00342 #if defined(USE_IOEXPANDER) /* (USE_IOEXPANDER always defined for RevA) */ 00343 /* GPIO_PIN[Led] depends on the board revision: */ 00344 /* - in case of RevA all leds are on IOEXPANDER (Mfx) */ 00345 BSP_IO_WritePin(GPIO_PIN[Led], BSP_IO_PIN_SET); 00346 #endif /* USE_IOEXPANDER */ 00347 #endif /* USE_STM32469I_EVAL_REVA */ 00348 } 00349 00350 /** 00351 * @brief Toggles the selected LED. 00352 * @param Led: LED to be toggled 00353 * This parameter can be one of the following values: 00354 * @arg LED1 00355 * @arg LED2 00356 * @arg LED3 00357 * @arg LED4 00358 */ 00359 void BSP_LED_Toggle(Led_TypeDef Led) 00360 { 00361 #if !defined(USE_STM32469I_EVAL_REVA) 00362 /* Eval Rev B and beyond */ 00363 /* On RevB LED1 LED2 LED3 and LED4 are on GPIO PK3, 4, 5, 6 */ 00364 if (Led <= LED4) 00365 { 00366 HAL_GPIO_TogglePin(LEDx_GPIO_PORT, GPIO_PIN[Led]); 00367 } 00368 #else 00369 #if defined(USE_IOEXPANDER) /* (USE_IOEXPANDER always defined for RevA) */ 00370 /* GPIO_PIN[Led] depends on the board revision: */ 00371 /* - in case of RevA all leds are on IOEXPANDER (Mfx) */ 00372 BSP_IO_TogglePin(GPIO_PIN[Led]); 00373 #endif /* USE_IOEXPANDER */ 00374 #endif /* USE_STM32469I_EVAL_REVA */ 00375 } 00376 00377 /** 00378 * @brief Configures button GPIO and EXTI Line. 00379 * @param Button: Button to be configured 00380 * This parameter can be one of the following values: 00381 * @arg BUTTON_WAKEUP: Wakeup Push Button 00382 * @arg BUTTON_TAMPER: Tamper Push Button 00383 * @param Button_Mode: Button mode 00384 * This parameter can be one of the following values: 00385 * @arg BUTTON_MODE_GPIO: Button will be used as simple IO 00386 * @arg BUTTON_MODE_EXTI: Button will be connected to EXTI line 00387 * with interrupt generation capability 00388 */ 00389 void BSP_PB_Init(Button_TypeDef Button, ButtonMode_TypeDef Button_Mode) 00390 { 00391 GPIO_InitTypeDef gpio_init_structure; 00392 00393 /* Enable the BUTTON clock */ 00394 BUTTONx_GPIO_CLK_ENABLE(Button); 00395 00396 if(Button_Mode == BUTTON_MODE_GPIO) 00397 { 00398 /* Configure Button pin as input */ 00399 gpio_init_structure.Pin = BUTTON_PIN[Button]; 00400 gpio_init_structure.Mode = GPIO_MODE_INPUT; 00401 gpio_init_structure.Pull = GPIO_NOPULL; 00402 gpio_init_structure.Speed = GPIO_SPEED_FAST; 00403 HAL_GPIO_Init(BUTTON_PORT[Button], &gpio_init_structure); 00404 } 00405 00406 if(Button_Mode == BUTTON_MODE_EXTI) 00407 { 00408 /* Configure Button pin as input with External interrupt */ 00409 gpio_init_structure.Pin = BUTTON_PIN[Button]; 00410 gpio_init_structure.Pull = GPIO_NOPULL; 00411 gpio_init_structure.Speed = GPIO_SPEED_FAST; 00412 00413 if(Button != BUTTON_WAKEUP) 00414 { 00415 gpio_init_structure.Mode = GPIO_MODE_IT_FALLING; 00416 } 00417 else 00418 { 00419 gpio_init_structure.Mode = GPIO_MODE_IT_RISING; 00420 } 00421 00422 HAL_GPIO_Init(BUTTON_PORT[Button], &gpio_init_structure); 00423 00424 /* Enable and set Button EXTI Interrupt to the lowest priority */ 00425 HAL_NVIC_SetPriority((IRQn_Type)(BUTTON_IRQn[Button]), 0x0F, 0x00); 00426 HAL_NVIC_EnableIRQ((IRQn_Type)(BUTTON_IRQn[Button])); 00427 } 00428 } 00429 00430 /** 00431 * @brief Push Button DeInit. 00432 * @param Button: Button to be configured 00433 * This parameter can be one of the following values: 00434 * @arg BUTTON_WAKEUP: Wakeup Push Button 00435 * @arg BUTTON_TAMPER: Tamper Push Button 00436 * @note PB DeInit does not disable the GPIO clock 00437 */ 00438 void BSP_PB_DeInit(Button_TypeDef Button) 00439 { 00440 GPIO_InitTypeDef gpio_init_structure; 00441 00442 gpio_init_structure.Pin = BUTTON_PIN[Button]; 00443 HAL_NVIC_DisableIRQ((IRQn_Type)(BUTTON_IRQn[Button])); 00444 HAL_GPIO_DeInit(BUTTON_PORT[Button], gpio_init_structure.Pin); 00445 } 00446 00447 00448 /** 00449 * @brief Returns the selected button state. 00450 * @param Button: Button to be checked 00451 * This parameter can be one of the following values: 00452 * @arg BUTTON_WAKEUP: Wakeup Push Button 00453 * @arg BUTTON_TAMPER: Tamper Push Button 00454 * @retval The Button GPIO pin value 00455 */ 00456 uint32_t BSP_PB_GetState(Button_TypeDef Button) 00457 { 00458 return HAL_GPIO_ReadPin(BUTTON_PORT[Button], BUTTON_PIN[Button]); 00459 } 00460 00461 /** 00462 * @brief Configures COM port. 00463 * @param COM: COM port to be configured. 00464 * This parameter can be one of the following values: 00465 * @arg COM1 00466 * @arg COM2 00467 * @param huart: Pointer to a UART_HandleTypeDef structure that contains the 00468 * configuration information for the specified USART peripheral. 00469 */ 00470 void BSP_COM_Init(COM_TypeDef COM, UART_HandleTypeDef *huart) 00471 { 00472 GPIO_InitTypeDef gpio_init_structure; 00473 00474 /* Enable GPIO clock of GPIO port supporting COM_TX and COM_RX pins */ 00475 EVAL_COMx_TX_RX_GPIO_CLK_ENABLE(COM); 00476 00477 /* Enable USART clock */ 00478 EVAL_COMx_CLK_ENABLE(COM); 00479 00480 /* Configure USART Tx as alternate function */ 00481 gpio_init_structure.Pin = COM_TX_PIN[COM]; 00482 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00483 gpio_init_structure.Pull = GPIO_PULLUP; 00484 gpio_init_structure.Speed = GPIO_SPEED_HIGH; 00485 gpio_init_structure.Alternate = COM_TX_AF[COM]; 00486 HAL_GPIO_Init(COM_TX_PORT[COM], &gpio_init_structure); 00487 00488 /* Configure USART Rx as alternate function */ 00489 gpio_init_structure.Pin = COM_RX_PIN[COM]; 00490 HAL_GPIO_Init(COM_RX_PORT[COM], &gpio_init_structure); 00491 00492 /* USART configuration */ 00493 huart->Instance = COM_USART[COM]; 00494 HAL_UART_Init(huart); 00495 } 00496 00497 /** 00498 * @brief DeInit COM port. 00499 * @param COM: COM port to be configured. 00500 * This parameter can be one of the following values: 00501 * @arg COM1 00502 * @arg COM2 00503 * @param huart: Pointer to a UART_HandleTypeDef structure that contains the 00504 * configuration information for the specified USART peripheral. 00505 */ 00506 void BSP_COM_DeInit(COM_TypeDef COM, UART_HandleTypeDef *huart) 00507 { 00508 /* USART configuration */ 00509 huart->Instance = COM_USART[COM]; 00510 HAL_UART_DeInit(huart); 00511 00512 /* Enable USART clock */ 00513 EVAL_COMx_CLK_DISABLE(COM); 00514 00515 /* DeInit GPIO pins can be done in the application 00516 (by surcharging this __weak function) */ 00517 00518 /* GPOI pins clock, FMC clock and DMA clock can be shut down in the applic 00519 by surcgarging this __weak function */ 00520 } 00521 #if defined(USE_IOEXPANDER) 00522 00523 /** 00524 * @brief Configures joystick GPIO and EXTI modes. 00525 * @param Joy_Mode: Button mode. 00526 * This parameter can be one of the following values: 00527 * @arg JOY_MODE_GPIO: Joystick pins will be used as simple IOs 00528 * @arg JOY_MODE_EXTI: Joystick pins will be connected to EXTI line 00529 * with interrupt generation capability 00530 * @retval IO_OK: if all initializations are OK. Other value if error. 00531 */ 00532 uint8_t BSP_JOY_Init(JOYMode_TypeDef Joy_Mode) 00533 { 00534 uint8_t ret = 0; 00535 00536 /* Initialize the IO functionalities */ 00537 ret = BSP_IO_Init(); 00538 00539 /* Configure joystick pins in IT mode */ 00540 if(Joy_Mode == JOY_MODE_EXTI) 00541 { 00542 /* Configure IO interrupt acquisition mode */ 00543 BSP_IO_ConfigPin(JOY_ALL_PINS, IO_MODE_IT_LOW_LEVEL_PU); 00544 } 00545 else 00546 { 00547 BSP_IO_ConfigPin(JOY_ALL_PINS, IO_MODE_INPUT_PU); 00548 } 00549 00550 return ret; 00551 } 00552 00553 00554 /** 00555 * @brief DeInit joystick GPIOs. 00556 * @note JOY DeInit does not disable the Mfx, just set the Mfx pins in Off mode 00557 */ 00558 void BSP_JOY_DeInit() 00559 { 00560 BSP_IO_ConfigPin(JOY_ALL_PINS, IO_MODE_OFF); 00561 } 00562 00563 /** 00564 * @brief Returns the current joystick status. 00565 * @retval Code of the joystick key pressed 00566 * This code can be one of the following values: 00567 * @arg JOY_NONE 00568 * @arg JOY_SEL 00569 * @arg JOY_DOWN 00570 * @arg JOY_LEFT 00571 * @arg JOY_RIGHT 00572 * @arg JOY_UP 00573 */ 00574 JOYState_TypeDef BSP_JOY_GetState(void) 00575 { 00576 uint16_t pin_status = 0; 00577 00578 /* Read the status joystick pins */ 00579 pin_status = BSP_IO_ReadPin(JOY_ALL_PINS); 00580 00581 /* Check the pressed keys */ 00582 if((pin_status & JOY_NONE_PIN) == JOY_NONE) 00583 { 00584 return(JOYState_TypeDef) JOY_NONE; 00585 } 00586 else if(!(pin_status & JOY_SEL_PIN)) 00587 { 00588 return(JOYState_TypeDef) JOY_SEL; 00589 } 00590 else if(!(pin_status & JOY_DOWN_PIN)) 00591 { 00592 return(JOYState_TypeDef) JOY_DOWN; 00593 } 00594 else if(!(pin_status & JOY_LEFT_PIN)) 00595 { 00596 return(JOYState_TypeDef) JOY_LEFT; 00597 } 00598 else if(!(pin_status & JOY_RIGHT_PIN)) 00599 { 00600 return(JOYState_TypeDef) JOY_RIGHT; 00601 } 00602 else if(!(pin_status & JOY_UP_PIN)) 00603 { 00604 return(JOYState_TypeDef) JOY_UP; 00605 } 00606 else 00607 { 00608 return(JOYState_TypeDef) JOY_NONE; 00609 } 00610 } 00611 #endif /* USE_IOEXPANDER */ 00612 00613 /** 00614 * @} 00615 */ 00616 00617 /** @defgroup STM32469I_EVAL_LOW_LEVEL_Private_Functions STM32469I EVAL LOW LEVEL Private Functions 00618 * @{ 00619 */ 00620 00621 00622 /******************************************************************************* 00623 BUS OPERATIONS 00624 *******************************************************************************/ 00625 00626 /******************************* I2C Routines *********************************/ 00627 /** 00628 * @brief Initializes I2C MSP. 00629 */ 00630 static void I2Cx_MspInit(void) 00631 { 00632 GPIO_InitTypeDef gpio_init_structure; 00633 00634 /*** Configure the GPIOs ***/ 00635 /* Enable GPIO clock */ 00636 EVAL_I2Cx_SCL_SDA_GPIO_CLK_ENABLE(); 00637 00638 /* Configure I2C Tx as alternate function */ 00639 gpio_init_structure.Pin = EVAL_I2Cx_SCL_PIN; 00640 gpio_init_structure.Mode = GPIO_MODE_AF_OD; 00641 gpio_init_structure.Pull = GPIO_NOPULL; 00642 gpio_init_structure.Speed = GPIO_SPEED_FAST; 00643 gpio_init_structure.Alternate = EVAL_I2Cx_SCL_SDA_AF; 00644 HAL_GPIO_Init(EVAL_I2Cx_SCL_SDA_GPIO_PORT, &gpio_init_structure); 00645 00646 /* Configure I2C Rx as alternate function */ 00647 gpio_init_structure.Pin = EVAL_I2Cx_SDA_PIN; 00648 HAL_GPIO_Init(EVAL_I2Cx_SCL_SDA_GPIO_PORT, &gpio_init_structure); 00649 00650 /*** Configure the I2C peripheral ***/ 00651 /* Enable I2C clock */ 00652 EVAL_I2Cx_CLK_ENABLE(); 00653 00654 /* Force the I2C peripheral clock reset */ 00655 EVAL_I2Cx_FORCE_RESET(); 00656 00657 /* Release the I2C peripheral clock reset */ 00658 EVAL_I2Cx_RELEASE_RESET(); 00659 00660 /* Enable and set I2Cx Interrupt to a lower priority */ 00661 HAL_NVIC_SetPriority(EVAL_I2Cx_EV_IRQn, 0x05, 0); 00662 HAL_NVIC_EnableIRQ(EVAL_I2Cx_EV_IRQn); 00663 00664 /* Enable and set I2Cx Interrupt to a lower priority */ 00665 HAL_NVIC_SetPriority(EVAL_I2Cx_ER_IRQn, 0x05, 0); 00666 HAL_NVIC_EnableIRQ(EVAL_I2Cx_ER_IRQn); 00667 } 00668 00669 /** 00670 * @brief Initializes I2C HAL. 00671 */ 00672 static void I2Cx_Init(void) 00673 { 00674 if(HAL_I2C_GetState(&heval_I2c) == HAL_I2C_STATE_RESET) 00675 { 00676 heval_I2c.Instance = I2C1; 00677 heval_I2c.Init.ClockSpeed = I2C_SCL_FREQ_KHZ; 00678 heval_I2c.Init.DutyCycle = I2C_DUTYCYCLE_2; 00679 heval_I2c.Init.OwnAddress1 = 0; 00680 heval_I2c.Init.AddressingMode = I2C_ADDRESSINGMODE_7BIT; 00681 heval_I2c.Init.DualAddressMode = I2C_DUALADDRESS_DISABLE; 00682 heval_I2c.Init.OwnAddress2 = 0; 00683 heval_I2c.Init.GeneralCallMode = I2C_GENERALCALL_DISABLE; 00684 heval_I2c.Init.NoStretchMode = I2C_NOSTRETCH_DISABLE; 00685 00686 /* Init the I2C */ 00687 I2Cx_MspInit(); 00688 HAL_I2C_Init(&heval_I2c); 00689 } 00690 } 00691 00692 /** 00693 * @brief Writes a single data. 00694 * @param Addr: I2C address 00695 * @param Reg: Register address 00696 * @param Value: Data to be written 00697 */ 00698 static void I2Cx_Write(uint8_t Addr, uint8_t Reg, uint8_t Value) 00699 { 00700 HAL_StatusTypeDef status = HAL_OK; 00701 00702 status = HAL_I2C_Mem_Write(&heval_I2c, 00703 Addr, 00704 (uint16_t)Reg, 00705 I2C_MEMADD_SIZE_8BIT, 00706 &Value, 00707 1, 00708 100); 00709 00710 /* Check the communication status */ 00711 if(status != HAL_OK) 00712 { 00713 /* Execute user timeout callback */ 00714 I2Cx_Error(Addr); 00715 } 00716 } 00717 00718 /** 00719 * @brief Reads a single data. 00720 * @param Addr: I2C address 00721 * @param Reg: Register address 00722 * @retval Read data 00723 */ 00724 static uint8_t I2Cx_Read(uint8_t Addr, uint8_t Reg) 00725 { 00726 HAL_StatusTypeDef status = HAL_OK; 00727 uint8_t Value = 0; 00728 00729 status = HAL_I2C_Mem_Read(&heval_I2c, 00730 Addr, 00731 Reg, 00732 I2C_MEMADD_SIZE_8BIT, 00733 &Value, 00734 1, 00735 1000); 00736 00737 /* Check the communication status */ 00738 if(status != HAL_OK) 00739 { 00740 /* Execute user timeout callback */ 00741 I2Cx_Error(Addr); 00742 } 00743 return Value; 00744 } 00745 00746 /** 00747 * @brief Reads multiple data. 00748 * @param Addr: I2C address 00749 * @param Reg: Reg address 00750 * @param MemAddress: memory address 00751 * @param Buffer: Pointer to data buffer 00752 * @param Length: Length of the data 00753 * @retval HAL status 00754 */ 00755 static HAL_StatusTypeDef I2Cx_ReadMultiple(uint8_t Addr, 00756 uint16_t Reg, 00757 uint16_t MemAddress, 00758 uint8_t *Buffer, 00759 uint16_t Length) 00760 { 00761 HAL_StatusTypeDef status = HAL_OK; 00762 00763 status = HAL_I2C_Mem_Read(&heval_I2c, 00764 Addr, 00765 (uint16_t)Reg, 00766 MemAddress, 00767 Buffer, 00768 Length, 00769 1000); 00770 00771 /* Check the communication status */ 00772 if(status != HAL_OK) 00773 { 00774 /* I2C error occured */ 00775 I2Cx_Error(Addr); 00776 } 00777 return status; 00778 } 00779 00780 /** 00781 * @brief Writes a value in a register of the device through BUS in using DMA mode. 00782 * @param Addr: Device address on BUS Bus. 00783 * @param Reg: The target register address to write 00784 * @param MemAddress: memory address 00785 * @param Buffer: The target register value to be written 00786 * @param Length: buffer size to be written 00787 * @retval HAL status 00788 */ 00789 static HAL_StatusTypeDef I2Cx_WriteMultiple(uint8_t Addr, 00790 uint16_t Reg, 00791 uint16_t MemAddress, 00792 uint8_t *Buffer, 00793 uint16_t Length) 00794 { 00795 HAL_StatusTypeDef status = HAL_OK; 00796 00797 status = HAL_I2C_Mem_Write(&heval_I2c, 00798 Addr, 00799 (uint16_t)Reg, 00800 MemAddress, 00801 Buffer, 00802 Length, 00803 1000); 00804 00805 /* Check the communication status */ 00806 if(status != HAL_OK) 00807 { 00808 /* Re-Initiaize the I2C Bus */ 00809 I2Cx_Error(Addr); 00810 } 00811 return status; 00812 } 00813 00814 /** 00815 * @brief Checks if target device is ready for communication. 00816 * @note This function is used with Memory devices 00817 * @param DevAddress: Target device address 00818 * @param Trials: Number of trials 00819 * @retval HAL status 00820 */ 00821 static HAL_StatusTypeDef I2Cx_IsDeviceReady(uint16_t DevAddress, uint32_t Trials) 00822 { 00823 return (HAL_I2C_IsDeviceReady(&heval_I2c, DevAddress, Trials, 1000)); 00824 } 00825 00826 /** 00827 * @brief Manages error callback by re-initializing I2C. 00828 * @param Addr: I2C Address 00829 */ 00830 static void I2Cx_Error(uint8_t Addr) 00831 { 00832 /* De-initialize the I2C comunication bus */ 00833 HAL_I2C_DeInit(&heval_I2c); 00834 00835 /* Re-Initiaize the I2C comunication bus */ 00836 I2Cx_Init(); 00837 } 00838 00839 /** 00840 * @} 00841 */ 00842 00843 /******************************************************************************* 00844 LINK OPERATIONS 00845 *******************************************************************************/ 00846 00847 /********************************* LINK IOE ***********************************/ 00848 00849 /** 00850 * @brief Initializes IOE low level. 00851 */ 00852 void IOE_Init(void) 00853 { 00854 I2Cx_Init(); 00855 } 00856 00857 /** 00858 * @brief Configures IOE low level interrupt. 00859 */ 00860 void IOE_ITConfig(void) 00861 { 00862 static uint8_t IOE_IT_Enabled = 0; 00863 /* GPIO_InitTypeDef GPIO_InitStruct; */ 00864 00865 if(IOE_IT_Enabled == 0) 00866 { 00867 IOE_IT_Enabled = 1; 00868 00869 /* to be implemented */ 00870 } 00871 } 00872 00873 /** 00874 * @brief IOE writes single data. 00875 * @param Addr: I2C address 00876 * @param Reg: Register address 00877 * @param Value: Data to be written 00878 */ 00879 void IOE_Write(uint8_t Addr, uint8_t Reg, uint8_t Value) 00880 { 00881 I2Cx_Write(Addr, Reg, Value); 00882 } 00883 00884 /** 00885 * @brief IOE reads single data. 00886 * @param Addr: I2C address 00887 * @param Reg: Register address 00888 * @retval Read data 00889 */ 00890 uint8_t IOE_Read(uint8_t Addr, uint8_t Reg) 00891 { 00892 return I2Cx_Read(Addr, Reg); 00893 } 00894 00895 /** 00896 * @brief IOE reads multiple data. 00897 * @param Addr: I2C address 00898 * @param Reg: Register address 00899 * @param Buffer: Pointer to data buffer 00900 * @param Length: Length of the data 00901 * @retval Number of read data 00902 */ 00903 uint16_t IOE_ReadMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length) 00904 { 00905 return I2Cx_ReadMultiple(Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT, Buffer, Length); 00906 } 00907 00908 /** 00909 * @brief IOE writes multiple data. 00910 * @param Addr: I2C address 00911 * @param Reg: Register address 00912 * @param Buffer: Pointer to data buffer 00913 * @param Length: Length of the data 00914 */ 00915 void IOE_WriteMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length) 00916 { 00917 I2Cx_WriteMultiple(Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT, Buffer, Length); 00918 } 00919 00920 /** 00921 * @brief IOE delay 00922 * @param Delay: Delay in ms 00923 */ 00924 void IOE_Delay(uint32_t Delay) 00925 { 00926 HAL_Delay(Delay); 00927 } 00928 00929 #if defined(USE_IOEXPANDER) 00930 00931 /********************************* LINK MFX ***********************************/ 00932 00933 /** 00934 * @brief Initializes MFX low level. 00935 */ 00936 void MFX_IO_Init(void) 00937 { 00938 I2Cx_Init(); 00939 } 00940 00941 /** 00942 * @brief DeInitializes MFX low level. 00943 */ 00944 void MFX_IO_DeInit(void) 00945 { 00946 } 00947 00948 /** 00949 * @brief Configures MFX low level interrupt. 00950 */ 00951 void MFX_IO_ITConfig(void) 00952 { 00953 static uint8_t MFX_IO_IT_Enabled = 0; 00954 GPIO_InitTypeDef gpio_init_structure; 00955 00956 if(MFX_IO_IT_Enabled == 0) 00957 { 00958 MFX_IO_IT_Enabled = 1; 00959 /* Enable the GPIO EXTI clock */ 00960 __HAL_RCC_GPIOI_CLK_ENABLE(); 00961 __HAL_RCC_SYSCFG_CLK_ENABLE(); 00962 00963 gpio_init_structure.Pin = GPIO_PIN_8; 00964 gpio_init_structure.Pull = GPIO_NOPULL; 00965 gpio_init_structure.Speed = GPIO_SPEED_LOW; 00966 gpio_init_structure.Mode = GPIO_MODE_IT_RISING; 00967 HAL_GPIO_Init(GPIOI, &gpio_init_structure); 00968 00969 /* Enable and set GPIO EXTI Interrupt to the lowest priority */ 00970 HAL_NVIC_SetPriority((IRQn_Type)(EXTI9_5_IRQn), 0x0F, 0x0F); 00971 HAL_NVIC_EnableIRQ((IRQn_Type)(EXTI9_5_IRQn)); 00972 } 00973 } 00974 00975 /** 00976 * @brief MFX writes single data. 00977 * @param Addr: I2C address 00978 * @param Reg: Register address 00979 * @param Value: Data to be written 00980 */ 00981 void MFX_IO_Write(uint16_t Addr, uint8_t Reg, uint8_t Value) 00982 { 00983 I2Cx_Write((uint8_t) Addr, Reg, Value); 00984 } 00985 00986 /** 00987 * @brief MFX reads single data. 00988 * @param Addr: I2C address 00989 * @param Reg: Register address 00990 * @retval Read data 00991 */ 00992 uint8_t MFX_IO_Read(uint16_t Addr, uint8_t Reg) 00993 { 00994 return I2Cx_Read((uint8_t) Addr, Reg); 00995 } 00996 00997 /** 00998 * @brief MFX reads multiple data. 00999 * @param Addr: I2C address 01000 * @param Reg: Register address 01001 * @param Buffer: Pointer to data buffer 01002 * @param Length: Length of the data 01003 * @retval Number of read data 01004 */ 01005 uint16_t MFX_IO_ReadMultiple(uint16_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length) 01006 { 01007 return I2Cx_ReadMultiple((uint8_t)Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT, Buffer, Length); 01008 } 01009 01010 /** 01011 * @brief MFX delay 01012 * @param Delay: Delay in ms 01013 */ 01014 void MFX_IO_Delay(uint32_t Delay) 01015 { 01016 HAL_Delay(Delay); 01017 } 01018 01019 /** 01020 * @brief Used by Lx family but requested for MXF component compatibility. 01021 */ 01022 void MFX_IO_Wakeup(void) 01023 { 01024 } 01025 01026 /** 01027 * @brief Used by Lx family but requested for MXF component compatibility. 01028 */ 01029 void MFX_IO_EnableWakeupPin(void) 01030 { 01031 } 01032 01033 #endif /* USE_IOEXPANDER */ 01034 01035 /********************************* LINK AUDIO *********************************/ 01036 01037 /** 01038 * @brief Initializes Audio low level. 01039 */ 01040 void AUDIO_IO_Init(void) 01041 { 01042 I2Cx_Init(); 01043 } 01044 01045 /** 01046 * @brief DeInitializes Audio low level. 01047 */ 01048 void AUDIO_IO_DeInit(void) 01049 { 01050 01051 } 01052 01053 /** 01054 * @brief Writes a single data. 01055 * @param Addr: I2C address 01056 * @param Reg: Reg address 01057 * @param Value: Data to be written 01058 */ 01059 void AUDIO_IO_Write(uint8_t Addr, uint16_t Reg, uint16_t Value) 01060 { 01061 uint16_t tmp = Value; 01062 01063 Value = ((uint16_t)(tmp >> 8) & 0x00FF); 01064 01065 Value |= ((uint16_t)(tmp << 8)& 0xFF00); 01066 01067 I2Cx_WriteMultiple(Addr, Reg, I2C_MEMADD_SIZE_16BIT,(uint8_t*)&Value, 2); 01068 } 01069 01070 /** 01071 * @brief Reads a single data. 01072 * @param Addr: I2C address 01073 * @param Reg: Reg address 01074 * @retval Data to be read 01075 */ 01076 uint16_t AUDIO_IO_Read(uint8_t Addr, uint16_t Reg) 01077 { 01078 uint16_t read_value = 0, tmp = 0; 01079 01080 I2Cx_ReadMultiple(Addr, Reg, I2C_MEMADD_SIZE_16BIT, (uint8_t*)&read_value, 2); 01081 01082 tmp = ((uint16_t)(read_value >> 8) & 0x00FF); 01083 01084 tmp |= ((uint16_t)(read_value << 8)& 0xFF00); 01085 01086 read_value = tmp; 01087 01088 return read_value; 01089 } 01090 01091 /** 01092 * @brief AUDIO Codec delay 01093 * @param Delay: Delay in ms 01094 */ 01095 void AUDIO_IO_Delay(uint32_t Delay) 01096 { 01097 HAL_Delay(Delay); 01098 } 01099 01100 /********************************* LINK CAMERA ********************************/ 01101 01102 /** 01103 * @brief Initializes Camera low level. 01104 */ 01105 void CAMERA_IO_Init(void) 01106 { 01107 I2Cx_Init(); 01108 } 01109 01110 /** 01111 * @brief Camera writes single data. 01112 * @param Addr: I2C address 01113 * @param Reg: Register address 01114 * @param Value: Data to be written 01115 */ 01116 void CAMERA_IO_Write(uint8_t Addr, uint16_t Reg, uint16_t Value) 01117 { 01118 uint16_t tmp = Value; 01119 /* For S5K5CAG sensor, 16 bits accesses are used */ 01120 Value = ((uint16_t)(tmp >> 8) & 0x00FF); 01121 Value |= ((uint16_t)(tmp << 8)& 0xFF00); 01122 I2Cx_WriteMultiple(Addr, Reg, I2C_MEMADD_SIZE_16BIT,(uint8_t*)&Value, 2); 01123 } 01124 01125 /** 01126 * @brief Camera reads single data. 01127 * @param Addr: I2C address 01128 * @param Reg: Register address 01129 * @retval Read data 01130 */ 01131 uint16_t CAMERA_IO_Read(uint8_t Addr, uint16_t Reg) 01132 { 01133 uint16_t read_value = 0, tmp = 0; 01134 /* For S5K5CAG sensor, 16 bits accesses are used */ 01135 I2Cx_ReadMultiple(Addr, Reg, I2C_MEMADD_SIZE_16BIT, (uint8_t*)&read_value, 2); 01136 tmp = ((uint16_t)(read_value >> 8) & 0x00FF); 01137 tmp |= ((uint16_t)(read_value << 8)& 0xFF00); 01138 read_value = tmp; 01139 01140 return read_value; 01141 } 01142 01143 /** 01144 * @brief Camera delay 01145 * @param Delay: Delay in ms 01146 */ 01147 void CAMERA_Delay(uint32_t Delay) 01148 { 01149 HAL_Delay(Delay); 01150 } 01151 01152 /******************************** LINK I2C EEPROM *****************************/ 01153 01154 /** 01155 * @brief Initializes peripherals used by the I2C EEPROM driver. 01156 */ 01157 void EEPROM_IO_Init(void) 01158 { 01159 I2Cx_Init(); 01160 } 01161 01162 /** 01163 * @brief Write data to I2C EEPROM driver in using DMA channel. 01164 * @param DevAddress: Target device address 01165 * @param MemAddress: Internal memory address 01166 * @param pBuffer: Pointer to data buffer 01167 * @param BufferSize: Amount of data to be sent 01168 * @retval HAL status 01169 */ 01170 HAL_StatusTypeDef EEPROM_IO_WriteData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize) 01171 { 01172 return (I2Cx_WriteMultiple(DevAddress, MemAddress, I2C_MEMADD_SIZE_16BIT, pBuffer, BufferSize)); 01173 } 01174 01175 /** 01176 * @brief Read data from I2C EEPROM driver in using DMA channel. 01177 * @param DevAddress: Target device address 01178 * @param MemAddress: Internal memory address 01179 * @param pBuffer: Pointer to data buffer 01180 * @param BufferSize: Amount of data to be read 01181 * @retval HAL status 01182 */ 01183 HAL_StatusTypeDef EEPROM_IO_ReadData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize) 01184 { 01185 return (I2Cx_ReadMultiple(DevAddress, MemAddress, I2C_MEMADD_SIZE_16BIT, pBuffer, BufferSize)); 01186 } 01187 01188 /** 01189 * @brief Checks if target device is ready for communication. 01190 * @note This function is used with Memory devices 01191 * @param DevAddress: Target device address 01192 * @param Trials: Number of trials 01193 * @retval HAL status 01194 */ 01195 HAL_StatusTypeDef EEPROM_IO_IsDeviceReady(uint16_t DevAddress, uint32_t Trials) 01196 { 01197 return (I2Cx_IsDeviceReady(DevAddress, Trials)); 01198 } 01199 01200 /******************************** LINK TS (TouchScreen) *****************************/ 01201 01202 /** 01203 * @brief Initialize I2C communication 01204 * channel from MCU to TouchScreen (TS). 01205 */ 01206 void TS_IO_Init(void) 01207 { 01208 I2Cx_Init(); 01209 } 01210 01211 /** 01212 * @brief Writes single data with I2C communication 01213 * channel from MCU to TouchScreen. 01214 * @param Addr: I2C address 01215 * @param Reg: Register address 01216 * @param Value: Data to be written 01217 */ 01218 void TS_IO_Write(uint8_t Addr, uint8_t Reg, uint8_t Value) 01219 { 01220 I2Cx_Write(Addr, Reg, Value); 01221 } 01222 01223 /** 01224 * @brief Reads single data with I2C communication 01225 * channel from TouchScreen. 01226 * @param Addr: I2C address 01227 * @param Reg: Register address 01228 * @retval Read data 01229 */ 01230 uint8_t TS_IO_Read(uint8_t Addr, uint8_t Reg) 01231 { 01232 return I2Cx_Read(Addr, Reg); 01233 } 01234 01235 /** 01236 * @brief Reads multiple data with I2C communication 01237 * channel from TouchScreen. 01238 * @param Addr: I2C address 01239 * @param Reg: Register address 01240 * @param Buffer: Pointer to data buffer 01241 * @param Length: Length of the data 01242 * @retval Number of read data 01243 */ 01244 uint16_t TS_IO_ReadMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length) 01245 { 01246 return I2Cx_ReadMultiple(Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT, Buffer, Length); 01247 } 01248 01249 /** 01250 * @brief Writes multiple data with I2C communication 01251 * channel from MCU to TouchScreen. 01252 * @param Addr: I2C address 01253 * @param Reg: Register address 01254 * @param Buffer: Pointer to data buffer 01255 * @param Length: Length of the data 01256 */ 01257 void TS_IO_WriteMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length) 01258 { 01259 I2Cx_WriteMultiple(Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT, Buffer, Length); 01260 } 01261 01262 /** 01263 * @brief Delay function used in TouchScreen low level driver. 01264 * @param Delay: Delay in ms 01265 */ 01266 void TS_IO_Delay(uint32_t Delay) 01267 { 01268 HAL_Delay(Delay); 01269 } 01270 01271 /**************************** LINK OTM8009A (Display driver) ******************/ 01272 /** 01273 * @brief OTM8009A delay 01274 * @param Delay: Delay in ms 01275 */ 01276 void OTM8009A_IO_Delay(uint32_t Delay) 01277 { 01278 HAL_Delay(Delay); 01279 } 01280 01281 /** 01282 * @} 01283 */ 01284 01285 /** 01286 * @} 01287 */ 01288 01289 /** 01290 * @} 01291 */ 01292 01293 /** 01294 * @} 01295 */ 01296 01297 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Tue Jan 12 2016 17:51:25 for STM32469I_EVAL BSP User Manual by
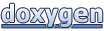