STM32303E_EVAL BSP User Manual
|
Functions | |
static void | I2Cx_Init (void) |
Eval I2Cx Bus initialization. | |
static void | I2Cx_WriteData (uint16_t Addr, uint8_t Reg, uint16_t RegSize, uint8_t Value) |
Write a value in a register of the device through BUS. | |
static HAL_StatusTypeDef | I2Cx_WriteBuffer (uint16_t Addr, uint8_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length) |
Write a value in a register of the device through BUS. | |
static uint8_t | I2Cx_ReadData (uint16_t Addr, uint8_t Reg, uint16_t RegSize) |
Read a register of the device through BUS. | |
static HAL_StatusTypeDef | I2Cx_ReadBuffer (uint16_t Addr, uint8_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length) |
Reads multiple data on the BUS. | |
static HAL_StatusTypeDef | I2Cx_IsDeviceReady (uint16_t DevAddress, uint32_t Trials) |
Checks if target device is ready for communication. | |
static void | I2Cx_Error (void) |
Eval I2Cx error treatment function. | |
static void | I2Cx_MspInit (I2C_HandleTypeDef *hi2c) |
Eval I2Cx MSP Initialization. | |
static void | SPIx_Init (void) |
Initializes SPI HAL. | |
static void | SPIx_Write (uint8_t Value) |
SPI Write a byte to device. | |
static uint32_t | SPIx_Read (void) |
SPI Read 4 bytes from device. | |
static void | SPIx_Error (void) |
SPI error treatment function. | |
static void | SPIx_MspInit (SPI_HandleTypeDef *hspi) |
Initializes SPI MSP. |
Function Documentation
static void I2Cx_Error | ( | void | ) | [static] |
Eval I2Cx error treatment function.
- Return values:
-
None
Definition at line 732 of file stm32303e_eval.c.
References heval_I2c, and I2Cx_Init().
Referenced by I2Cx_ReadBuffer(), I2Cx_ReadData(), I2Cx_WriteBuffer(), and I2Cx_WriteData().
static void I2Cx_Init | ( | void | ) | [static] |
Eval I2Cx Bus initialization.
- Return values:
-
None
Definition at line 602 of file stm32303e_eval.c.
References EVAL_I2Cx, heval_I2c, and I2Cx_MspInit().
Referenced by AUDIO_IO_Init(), EEPROM_I2C_IO_Init(), I2Cx_Error(), and TSENSOR_IO_Init().
static HAL_StatusTypeDef I2Cx_IsDeviceReady | ( | uint16_t | DevAddress, |
uint32_t | Trials | ||
) | [static] |
Checks if target device is ready for communication.
- Note:
- This function is used with Memory devices
- Parameters:
-
DevAddress Target device address Trials Number of trials
- Return values:
-
HAL status
Definition at line 722 of file stm32303e_eval.c.
References heval_I2c, and I2cxTimeout.
Referenced by EEPROM_I2C_IO_IsDeviceReady(), and TSENSOR_IO_IsDeviceReady().
static void I2Cx_MspInit | ( | I2C_HandleTypeDef * | hi2c | ) | [static] |
Eval I2Cx MSP Initialization.
- Parameters:
-
hi2c I2C handle
- Return values:
-
None
Definition at line 547 of file stm32303e_eval.c.
References EVAL_I2Cx, EVAL_I2Cx_CLK_ENABLE, EVAL_I2Cx_ER_IRQn, EVAL_I2Cx_EV_IRQn, EVAL_I2Cx_FORCE_RESET, EVAL_I2Cx_RELEASE_RESET, EVAL_I2Cx_SCL_GPIO_CLK_ENABLE, EVAL_I2Cx_SCL_GPIO_PORT, EVAL_I2Cx_SCL_PIN, EVAL_I2Cx_SCL_SDA_AF, EVAL_I2Cx_SDA_GPIO_CLK_ENABLE, EVAL_I2Cx_SDA_GPIO_PORT, and EVAL_I2Cx_SDA_PIN.
Referenced by I2Cx_Init().
static HAL_StatusTypeDef I2Cx_ReadBuffer | ( | uint16_t | Addr, |
uint8_t | Reg, | ||
uint16_t | RegSize, | ||
uint8_t * | pBuffer, | ||
uint16_t | Length | ||
) | [static] |
Reads multiple data on the BUS.
- Parameters:
-
Addr I2C Address Reg Reg Address RegSize The target register size (can be 8BIT or 16BIT) pBuffer pointer to read data buffer Length length of the data
- Return values:
-
0 if no problems to read multiple data
Definition at line 700 of file stm32303e_eval.c.
References heval_I2c, I2Cx_Error(), and I2cxTimeout.
Referenced by EEPROM_I2C_IO_ReadData(), and TSENSOR_IO_Read().
static uint8_t I2Cx_ReadData | ( | uint16_t | Addr, |
uint8_t | Reg, | ||
uint16_t | RegSize | ||
) | [static] |
Read a register of the device through BUS.
- Parameters:
-
Addr Device address on BUS Reg The target register address to read RegSize The target register size (can be 8BIT or 16BIT)
- Return values:
-
read register value
Definition at line 674 of file stm32303e_eval.c.
References heval_I2c, I2Cx_Error(), and I2cxTimeout.
Referenced by AUDIO_IO_Read().
static HAL_StatusTypeDef I2Cx_WriteBuffer | ( | uint16_t | Addr, |
uint8_t | Reg, | ||
uint16_t | RegSize, | ||
uint8_t * | pBuffer, | ||
uint16_t | Length | ||
) | [static] |
Write a value in a register of the device through BUS.
- Parameters:
-
Addr Device address on BUS Bus. Reg The target register address to write RegSize The target register size (can be 8BIT or 16BIT) pBuffer The target register value to be written Length buffer size to be written
- Return values:
-
None
Definition at line 652 of file stm32303e_eval.c.
References heval_I2c, I2Cx_Error(), and I2cxTimeout.
Referenced by EEPROM_I2C_IO_WriteData(), and TSENSOR_IO_Write().
static void I2Cx_WriteData | ( | uint16_t | Addr, |
uint8_t | Reg, | ||
uint16_t | RegSize, | ||
uint8_t | Value | ||
) | [static] |
Write a value in a register of the device through BUS.
- Parameters:
-
Addr Device address on BUS Bus. Reg The target register address to write RegSize The target register size (can be 8BIT or 16BIT) Value The target register value to be written
- Return values:
-
None
Definition at line 629 of file stm32303e_eval.c.
References heval_I2c, I2Cx_Error(), and I2cxTimeout.
Referenced by AUDIO_IO_Write().
static void SPIx_Error | ( | void | ) | [static] |
SPI error treatment function.
- Return values:
-
None
Definition at line 851 of file stm32303e_eval.c.
References heval_Spi, and SPIx_Init().
Referenced by SPIx_Read(), and SPIx_Write().
static void SPIx_Init | ( | void | ) | [static] |
Initializes SPI HAL.
- Return values:
-
None
Definition at line 782 of file stm32303e_eval.c.
References EVAL_SPIx, heval_Spi, and SPIx_MspInit().
Referenced by EEPROM_SPI_IO_Init(), LCD_IO_Init(), SD_IO_Init(), and SPIx_Error().
static void SPIx_MspInit | ( | SPI_HandleTypeDef * | hspi | ) | [static] |
Initializes SPI MSP.
- Return values:
-
None
Definition at line 748 of file stm32303e_eval.c.
References EVAL_SPIx_CLK_ENABLE, EVAL_SPIx_MISO_MOSI_AF, EVAL_SPIx_MISO_MOSI_GPIO_CLK_ENABLE, EVAL_SPIx_MISO_MOSI_GPIO_PORT, EVAL_SPIx_MISO_PIN, EVAL_SPIx_MOSI_PIN, EVAL_SPIx_SCK_AF, EVAL_SPIx_SCK_GPIO_CLK_ENABLE, EVAL_SPIx_SCK_GPIO_PORT, and EVAL_SPIx_SCK_PIN.
Referenced by SPIx_Init().
static uint32_t SPIx_Read | ( | void | ) | [static] |
SPI Read 4 bytes from device.
- Return values:
-
Read data
Definition at line 810 of file stm32303e_eval.c.
References heval_Spi, SPIx_Error(), and SpixTimeout.
Referenced by EEPROM_SPI_IO_ReadByte(), EEPROM_SPI_IO_ReadData(), EEPROM_SPI_IO_WaitEepromStandbyState(), LCD_IO_ReadData(), and SD_IO_ReadByte().
static void SPIx_Write | ( | uint8_t | Value | ) | [static] |
SPI Write a byte to device.
- Parameters:
-
Value value to be written
- Return values:
-
None
Definition at line 833 of file stm32303e_eval.c.
References heval_Spi, SPIx_Error(), and SpixTimeout.
Referenced by EEPROM_SPI_IO_ReadData(), EEPROM_SPI_IO_WaitEepromStandbyState(), EEPROM_SPI_IO_WriteByte(), EEPROM_SPI_IO_WriteData(), LCD_IO_ReadData(), LCD_IO_WriteMultipleData(), LCD_IO_WriteReg(), and SD_IO_WriteByte().
Generated on Wed May 31 2017 11:17:17 for STM32303E_EVAL BSP User Manual by
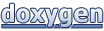