STM32303E_EVAL BSP User Manual
|
stm32303e_eval.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32303e_eval.c 00004 * @author MCD Application Team 00005 * @brief This file provides a set of firmware functions to manage Leds, 00006 * push-button and COM ports 00007 ****************************************************************************** 00008 * @attention 00009 * 00010 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00011 * 00012 * Redistribution and use in source and binary forms, with or without modification, 00013 * are permitted provided that the following conditions are met: 00014 * 1. Redistributions of source code must retain the above copyright notice, 00015 * this list of conditions and the following disclaimer. 00016 * 2. Redistributions in binary form must reproduce the above copyright notice, 00017 * this list of conditions and the following disclaimer in the documentation 00018 * and/or other materials provided with the distribution. 00019 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00020 * may be used to endorse or promote products derived from this software 00021 * without specific prior written permission. 00022 * 00023 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00024 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00025 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00026 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00027 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00028 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00029 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00030 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00031 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00032 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00033 * 00034 ****************************************************************************** 00035 */ 00036 00037 /* Includes ------------------------------------------------------------------*/ 00038 #include "stm32303e_eval.h" 00039 00040 /** @addtogroup BSP 00041 * @{ 00042 */ 00043 00044 /** @addtogroup STM32303E_EVAL 00045 * @brief This file provides firmware functions to manage Leds, push-buttons, 00046 * COM ports, SD card on SPI and temperature sensor (TS751) available on 00047 * STM32303E-EVAL evaluation board from STMicroelectronics. 00048 * @{ 00049 */ 00050 00051 /** @addtogroup STM32303E_EVAL_Common 00052 * @{ 00053 */ 00054 00055 /** @addtogroup STM32303E_EVAL_Private_Constants 00056 * @{ 00057 */ 00058 /* LINK LCD */ 00059 #define START_BYTE 0x70 00060 #define SET_INDEX 0x00 00061 #define READ_STATUS 0x01 00062 #define LCD_WRITE_REG 0x02 00063 #define LCD_READ_REG 0x03 00064 00065 /* LINK SD Card */ 00066 #define SD_DUMMY_BYTE 0xFF 00067 #define SD_NO_RESPONSE_EXPECTED 0x80 00068 00069 /* LINK EEPROM SPI */ 00070 #define EEPROM_CMD_WREN 0x06 /*!< Write enable instruction */ 00071 #define EEPROM_CMD_WRDI 0x04 /*!< Write disable instruction */ 00072 #define EEPROM_CMD_RDSR 0x05 /*!< Read Status Register instruction */ 00073 #define EEPROM_CMD_WRSR 0x01 /*!< Write Status Register instruction */ 00074 #define EEPROM_CMD_WRITE 0x02 /*!< Write to Memory instruction */ 00075 #define EEPROM_CMD_READ 0x03 /*!< Read from Memory instruction */ 00076 00077 #define EEPROM_WIP_FLAG 0x01 /*!< Write In Progress (WIP) flag */ 00078 00079 /** 00080 * @brief STM32303E EVAL BSP Driver version number V2.1.2 00081 */ 00082 #define __STM32303E_EVAL_BSP_VERSION_MAIN (0x02) /*!< [31:24] main version */ 00083 #define __STM32303E_EVAL_BSP_VERSION_SUB1 (0x01) /*!< [23:16] sub1 version */ 00084 #define __STM32303E_EVAL_BSP_VERSION_SUB2 (0x02) /*!< [15:8] sub2 version */ 00085 #define __STM32303E_EVAL_BSP_VERSION_RC (0x00) /*!< [7:0] release candidate */ 00086 #define __STM32303E_EVAL_BSP_VERSION ((__STM32303E_EVAL_BSP_VERSION_MAIN << 24)\ 00087 |(__STM32303E_EVAL_BSP_VERSION_SUB1 << 16)\ 00088 |(__STM32303E_EVAL_BSP_VERSION_SUB2 << 8 )\ 00089 |(__STM32303E_EVAL_BSP_VERSION_RC)) 00090 /** 00091 * @} 00092 */ 00093 00094 /** @addtogroup STM32303E_EVAL_Private_Variables 00095 * @{ 00096 */ 00097 /** 00098 * @brief LED variables 00099 */ 00100 GPIO_TypeDef* LED_PORT[LEDn] = {LED1_GPIO_PORT, 00101 LED2_GPIO_PORT, 00102 LED3_GPIO_PORT, 00103 LED4_GPIO_PORT}; 00104 00105 const uint16_t LED_PIN[LEDn] = {LED1_PIN, 00106 LED2_PIN, 00107 LED3_PIN, 00108 LED4_PIN}; 00109 00110 /** 00111 * @brief BUTTON variables 00112 */ 00113 GPIO_TypeDef* BUTTON_PORT[BUTTONn] = {KEY_BUTTON_GPIO_PORT, 00114 SEL_JOY_GPIO_PORT, 00115 LEFT_JOY_GPIO_PORT, 00116 RIGHT_JOY_GPIO_PORT, 00117 DOWN_JOY_GPIO_PORT, 00118 UP_JOY_GPIO_PORT}; 00119 00120 const uint16_t BUTTON_PIN[BUTTONn] = {KEY_BUTTON_PIN, 00121 SEL_JOY_PIN, 00122 LEFT_JOY_PIN, 00123 RIGHT_JOY_PIN, 00124 DOWN_JOY_PIN, 00125 UP_JOY_PIN}; 00126 00127 const uint8_t BUTTON_IRQn[BUTTONn] = {KEY_BUTTON_EXTI_IRQn, 00128 SEL_JOY_EXTI_IRQn, 00129 LEFT_JOY_EXTI_IRQn, 00130 RIGHT_JOY_EXTI_IRQn, 00131 DOWN_JOY_EXTI_IRQn, 00132 UP_JOY_EXTI_IRQn}; 00133 00134 /** 00135 * @brief JOYSTICK variables 00136 */ 00137 GPIO_TypeDef* JOY_PORT[JOYn] = {SEL_JOY_GPIO_PORT, 00138 LEFT_JOY_GPIO_PORT, 00139 RIGHT_JOY_GPIO_PORT, 00140 DOWN_JOY_GPIO_PORT, 00141 UP_JOY_GPIO_PORT}; 00142 00143 const uint16_t JOY_PIN[JOYn] = {SEL_JOY_PIN, 00144 LEFT_JOY_PIN, 00145 RIGHT_JOY_PIN, 00146 DOWN_JOY_PIN, 00147 UP_JOY_PIN}; 00148 00149 const uint8_t JOY_IRQn[JOYn] = {SEL_JOY_EXTI_IRQn, 00150 LEFT_JOY_EXTI_IRQn, 00151 RIGHT_JOY_EXTI_IRQn, 00152 DOWN_JOY_EXTI_IRQn, 00153 UP_JOY_EXTI_IRQn}; 00154 00155 /** 00156 * @brief COM variables 00157 */ 00158 USART_TypeDef* COM_USART[COMn] = {EVAL_COM1}; 00159 00160 GPIO_TypeDef* COM_TX_PORT[COMn] = {EVAL_COM1_TX_GPIO_PORT}; 00161 00162 GPIO_TypeDef* COM_RX_PORT[COMn] = {EVAL_COM1_RX_GPIO_PORT}; 00163 00164 const uint16_t COM_TX_PIN[COMn] = {EVAL_COM1_TX_PIN}; 00165 00166 const uint16_t COM_RX_PIN[COMn] = {EVAL_COM1_RX_PIN}; 00167 00168 const uint16_t COM_TX_AF[COMn] = {EVAL_COM1_TX_AF}; 00169 00170 const uint16_t COM_RX_AF[COMn] = {EVAL_COM1_RX_AF}; 00171 00172 /** 00173 * @brief BUS variables 00174 */ 00175 #ifdef HAL_SPI_MODULE_ENABLED 00176 uint32_t SpixTimeout = EVAL_SPIx_TIMEOUT_MAX; /*<! Value of Timeout when SPI communication fails */ 00177 static SPI_HandleTypeDef heval_Spi; 00178 #endif /* HAL_SPI_MODULE_ENABLED */ 00179 00180 #ifdef HAL_I2C_MODULE_ENABLED 00181 uint32_t I2cxTimeout = EVAL_I2Cx_TIMEOUT_MAX; /*<! Value of Timeout when I2C communication fails */ 00182 I2C_HandleTypeDef heval_I2c; 00183 #endif /* HAL_I2C_MODULE_ENABLED */ 00184 00185 /** 00186 * @} 00187 */ 00188 00189 /** @defgroup STM32303E_EVAL_BUS Bus Operation functions 00190 * @{ 00191 */ 00192 00193 /* I2Cx bus function */ 00194 #ifdef HAL_I2C_MODULE_ENABLED 00195 /* Link function for I2C EEPROM peripheral */ 00196 static void I2Cx_Init(void); 00197 static void I2Cx_WriteData(uint16_t Addr, uint8_t Reg, uint16_t RegSize, uint8_t Value); 00198 static HAL_StatusTypeDef I2Cx_WriteBuffer(uint16_t Addr, uint8_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length); 00199 static uint8_t I2Cx_ReadData(uint16_t Addr, uint8_t Reg, uint16_t RegSize); 00200 static HAL_StatusTypeDef I2Cx_ReadBuffer(uint16_t Addr, uint8_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length); 00201 static HAL_StatusTypeDef I2Cx_IsDeviceReady(uint16_t DevAddress, uint32_t Trials); 00202 static void I2Cx_Error (void); 00203 static void I2Cx_MspInit(I2C_HandleTypeDef *hi2c); 00204 00205 /* Link function for EEPROM peripheral over I2C */ 00206 void EEPROM_I2C_IO_Init(void); 00207 HAL_StatusTypeDef EEPROM_I2C_IO_WriteData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize); 00208 HAL_StatusTypeDef EEPROM_I2C_IO_ReadData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize); 00209 HAL_StatusTypeDef EEPROM_I2C_IO_IsDeviceReady(uint16_t DevAddress, uint32_t Trials); 00210 00211 /* Link function for Temperature Sensor peripheral */ 00212 void TSENSOR_IO_Init(void); 00213 void TSENSOR_IO_Write(uint16_t DevAddress, uint8_t* pBuffer, uint8_t WriteAddr, uint16_t Length); 00214 void TSENSOR_IO_Read(uint16_t DevAddress, uint8_t* pBuffer, uint8_t ReadAddr, uint16_t Length); 00215 uint16_t TSENSOR_IO_IsDeviceReady(uint16_t DevAddress, uint32_t Trials); 00216 00217 /* Link function for Audio Codec peripheral */ 00218 void AUDIO_IO_Init(void); 00219 void AUDIO_IO_DeInit(void); 00220 void AUDIO_IO_Write(uint16_t DevAddress, uint8_t Reg, uint8_t Value); 00221 uint8_t AUDIO_IO_Read(uint16_t DevAddress, uint8_t Reg); 00222 void AUDIO_IO_Delay(uint32_t delay); 00223 #endif /* HAL_I2C_MODULE_ENABLED */ 00224 00225 /* SPIx bus function */ 00226 #ifdef HAL_SPI_MODULE_ENABLED 00227 static void SPIx_Init(void); 00228 static void SPIx_Write(uint8_t Value); 00229 static uint32_t SPIx_Read(void); 00230 static void SPIx_Error (void); 00231 static void SPIx_MspInit(SPI_HandleTypeDef *hspi); 00232 00233 /* Link function for LCD peripheral over SPI */ 00234 void LCD_IO_Init(void); 00235 void LCD_IO_WriteMultipleData(uint8_t *pData, uint32_t Size); 00236 void LCD_IO_WriteReg(uint8_t Reg); 00237 uint16_t LCD_IO_ReadData(uint16_t Reg); 00238 void LCD_Delay (uint32_t delay); 00239 00240 /* Link function for EEPROM peripheral over SPI */ 00241 void EEPROM_SPI_IO_Init(void); 00242 void EEPROM_SPI_IO_WriteByte(uint8_t Data); 00243 uint8_t EEPROM_SPI_IO_ReadByte(void); 00244 HAL_StatusTypeDef EEPROM_SPI_IO_WriteData(uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize); 00245 HAL_StatusTypeDef EEPROM_SPI_IO_ReadData(uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize); 00246 HAL_StatusTypeDef EEPROM_SPI_IO_WaitEepromStandbyState(void); 00247 00248 /* Link functions for SD Card peripheral over SPI */ 00249 void SD_IO_Init(void); 00250 HAL_StatusTypeDef SD_IO_WriteCmd(uint8_t Cmd, uint32_t Arg, uint8_t Crc, uint8_t Response); 00251 HAL_StatusTypeDef SD_IO_WaitResponse(uint8_t Response); 00252 void SD_IO_WriteDummy(void); 00253 void SD_IO_WriteByte(uint8_t Data); 00254 uint8_t SD_IO_ReadByte(void); 00255 #endif /* HAL_SPI_MODULE_ENABLED */ 00256 00257 /** 00258 * @} 00259 */ 00260 00261 /** @addtogroup STM32303E_EVAL_Exported_Functions 00262 * @{ 00263 */ 00264 00265 /** 00266 * @brief This method returns the STM32303E EVAL BSP Driver revision 00267 * @retval version : 0xXYZR (8bits for each decimal, R for RC) 00268 */ 00269 uint32_t BSP_GetVersion(void) 00270 { 00271 return __STM32303E_EVAL_BSP_VERSION; 00272 } 00273 00274 /** 00275 * @brief Configures LED GPIO. 00276 * @param Led Specifies the Led to be configured. 00277 * This parameter can be one of following parameters: 00278 * @arg LED1 00279 * @arg LED2 00280 * @arg LED3 00281 * @arg LED4 00282 * @retval None 00283 */ 00284 void BSP_LED_Init(Led_TypeDef Led) 00285 { 00286 GPIO_InitTypeDef GPIO_InitStructure; 00287 00288 /* Enable the GPIO_LED clock */ 00289 LEDx_GPIO_CLK_ENABLE(Led); 00290 00291 /* Configure the GPIO_LED pin */ 00292 GPIO_InitStructure.Pin = LED_PIN[Led]; 00293 GPIO_InitStructure.Mode = GPIO_MODE_OUTPUT_PP; 00294 GPIO_InitStructure.Pull = GPIO_PULLUP; 00295 GPIO_InitStructure.Speed = GPIO_SPEED_FREQ_HIGH; 00296 00297 HAL_GPIO_Init(LED_PORT[Led], &GPIO_InitStructure); 00298 00299 HAL_GPIO_WritePin(LED_PORT[Led], LED_PIN[Led], GPIO_PIN_SET); 00300 } 00301 00302 /** 00303 * @brief Turns selected LED On. 00304 * @param Led Specifies the Led to be set on. 00305 * This parameter can be one of following parameters: 00306 * @arg LED1 00307 * @arg LED2 00308 * @arg LED3 00309 * @arg LED4 00310 * @retval None 00311 */ 00312 void BSP_LED_On(Led_TypeDef Led) 00313 { 00314 HAL_GPIO_WritePin(LED_PORT[Led], LED_PIN[Led], GPIO_PIN_RESET); 00315 } 00316 00317 /** 00318 * @brief Turns selected LED Off. 00319 * @param Led Specifies the Led to be set off. 00320 * This parameter can be one of following parameters: 00321 * @arg LED1 00322 * @arg LED2 00323 * @arg LED3 00324 * @arg LED4 00325 * @retval None 00326 */ 00327 void BSP_LED_Off(Led_TypeDef Led) 00328 { 00329 HAL_GPIO_WritePin(LED_PORT[Led], LED_PIN[Led], GPIO_PIN_SET); 00330 } 00331 00332 /** 00333 * @brief Toggles the selected LED. 00334 * @param Led Specifies the Led to be toggled. 00335 * This parameter can be one of following parameters: 00336 * @arg LED1 00337 * @arg LED2 00338 * @arg LED3 00339 * @arg LED4 00340 * @retval None 00341 */ 00342 void BSP_LED_Toggle(Led_TypeDef Led) 00343 { 00344 HAL_GPIO_TogglePin(LED_PORT[Led], LED_PIN[Led]); 00345 } 00346 00347 /** 00348 * @brief Configures push button GPIO and EXTI Line. 00349 * @param Button Button to be configured. 00350 * This parameter can be one of the following values: 00351 * @arg BUTTON_KEY: Key Push Button 00352 * @arg BUTTON_SEL : Sel Push Button on Joystick 00353 * @arg BUTTON_LEFT : Left Push Button on Joystick 00354 * @arg BUTTON_RIGHT : Right Push Button on Joystick 00355 * @arg BUTTON_DOWN : Down Push Button on Joystick 00356 * @arg BUTTON_UP : Up Push Button on Joystick 00357 * @param Button_Mode Button mode requested. 00358 * This parameter can be one of the following values: 00359 * @arg BUTTON_MODE_GPIO: Button will be used as simple IO 00360 * @arg BUTTON_MODE_EXTI: Button will be connected to EXTI line 00361 * with interrupt generation capability 00362 * @retval None 00363 */ 00364 void BSP_PB_Init(Button_TypeDef Button, ButtonMode_TypeDef Button_Mode) 00365 { 00366 GPIO_InitTypeDef GPIO_InitStructure; 00367 00368 /* Enable the corresponding Push Button clock */ 00369 BUTTONx_GPIO_CLK_ENABLE(Button); 00370 00371 /* Configure Push Button pin as input */ 00372 GPIO_InitStructure.Pin = BUTTON_PIN[Button]; 00373 GPIO_InitStructure.Pull = GPIO_PULLDOWN; 00374 GPIO_InitStructure.Speed = GPIO_SPEED_FREQ_HIGH; 00375 00376 if (Button_Mode == BUTTON_MODE_GPIO) 00377 { 00378 /* Configure Button pin as input */ 00379 GPIO_InitStructure.Mode = GPIO_MODE_INPUT; 00380 HAL_GPIO_Init(BUTTON_PORT[Button], &GPIO_InitStructure); 00381 } 00382 00383 if (Button_Mode == BUTTON_MODE_EXTI) 00384 { 00385 if(Button == BUTTON_KEY) 00386 { 00387 /* Configure Key Push Button pin as input with External interrupt, falling edge */ 00388 GPIO_InitStructure.Mode = GPIO_MODE_IT_FALLING; 00389 } 00390 else 00391 { 00392 /* Configure Joystick Push Button pin as input with External interrupt, rising edge */ 00393 GPIO_InitStructure.Mode = GPIO_MODE_IT_RISING; 00394 } 00395 HAL_GPIO_Init(BUTTON_PORT[Button], &GPIO_InitStructure); 00396 00397 /* Enable and set Button EXTI Interrupt to the lowest priority */ 00398 HAL_NVIC_SetPriority((IRQn_Type)(BUTTON_IRQn[Button]), 0x0F, 0); 00399 HAL_NVIC_EnableIRQ((IRQn_Type)(BUTTON_IRQn[Button])); 00400 } 00401 } 00402 00403 /** 00404 * @brief Returns the selected button state. 00405 * @param Button Button to be checked. 00406 * This parameter can be one of the following values: 00407 * @arg BUTTON_KEY: Key Push Button 00408 * @retval The Button GPIO pin value 00409 */ 00410 uint32_t BSP_PB_GetState(Button_TypeDef Button) 00411 { 00412 return HAL_GPIO_ReadPin(BUTTON_PORT[Button], BUTTON_PIN[Button]); 00413 } 00414 00415 /** 00416 * @brief Configures all button of the joystick in GPIO or EXTI modes. 00417 * @param Joy_Mode Joystick mode. 00418 * This parameter can be one of the following values: 00419 * @arg JOY_MODE_GPIO: Joystick pins will be used as simple IOs 00420 * @arg JOY_MODE_EXTI: Joystick pins will be connected to EXTI line 00421 * with interrupt generation capability 00422 * @retval HAL_OK: if all initializations are OK. Other value if error. 00423 */ 00424 uint8_t BSP_JOY_Init(JOYMode_TypeDef Joy_Mode) 00425 { 00426 JOYState_TypeDef JoyKey; 00427 GPIO_InitTypeDef GPIO_InitStructure; 00428 00429 /* Initialized the Joystick. */ 00430 for(JoyKey = JOY_SEL; JoyKey < (JOY_SEL+JOYn) ; JoyKey++) 00431 { 00432 /* Enable the JOY clock */ 00433 JOYx_GPIO_CLK_ENABLE(JoyKey); 00434 00435 GPIO_InitStructure.Pin = JOY_PIN[JoyKey]; 00436 GPIO_InitStructure.Pull = GPIO_PULLDOWN; 00437 GPIO_InitStructure.Speed = GPIO_SPEED_FREQ_HIGH; 00438 00439 if (Joy_Mode == JOY_MODE_GPIO) 00440 { 00441 /* Configure Joy pin as input */ 00442 GPIO_InitStructure.Mode = GPIO_MODE_INPUT; 00443 HAL_GPIO_Init(JOY_PORT[JoyKey], &GPIO_InitStructure); 00444 } 00445 00446 if (Joy_Mode == JOY_MODE_EXTI) 00447 { 00448 /* Configure Joy pin as input with External interrupt */ 00449 GPIO_InitStructure.Mode = GPIO_MODE_IT_RISING; 00450 HAL_GPIO_Init(JOY_PORT[JoyKey], &GPIO_InitStructure); 00451 00452 /* Enable and set Joy EXTI Interrupt to the lowest priority */ 00453 HAL_NVIC_SetPriority((IRQn_Type)(JOY_IRQn[JoyKey]), 0x0F, 0); 00454 HAL_NVIC_EnableIRQ((IRQn_Type)(JOY_IRQn[JoyKey])); 00455 } 00456 } 00457 00458 return HAL_OK; 00459 } 00460 00461 /** 00462 * @brief Returns the current joystick status. 00463 * @retval Code of the joystick key pressed 00464 * This code can be one of the following values: 00465 * @arg JOY_NONE 00466 * @arg JOY_SEL 00467 * @arg JOY_DOWN 00468 * @arg JOY_LEFT 00469 * @arg JOY_RIGHT 00470 * @arg JOY_UP 00471 * @arg JOY_NONE 00472 */ 00473 JOYState_TypeDef BSP_JOY_GetState(void) 00474 { 00475 JOYState_TypeDef JoyKey; 00476 00477 for(JoyKey = JOY_SEL; JoyKey < (JOY_SEL+JOYn) ; JoyKey++) 00478 { 00479 if(HAL_GPIO_ReadPin(JOY_PORT[JoyKey], JOY_PIN[JoyKey]) == GPIO_PIN_SET) 00480 { 00481 /* Return Code Joystick key pressed */ 00482 return JoyKey; 00483 } 00484 } 00485 00486 /* No Joystick key pressed */ 00487 return JOY_NONE; 00488 } 00489 00490 #if defined(HAL_UART_MODULE_ENABLED) 00491 /** 00492 * @brief Configures COM port. 00493 * @param COM Specifies the COM port to be configured. 00494 * This parameter can be one of following parameters: 00495 * @arg COM1 00496 * @param huart pointer to a UART_HandleTypeDef structure that 00497 * contains the configuration information for the specified UART peripheral. 00498 * @retval None 00499 */ 00500 void BSP_COM_Init(COM_TypeDef COM, UART_HandleTypeDef* huart) 00501 { 00502 GPIO_InitTypeDef GPIO_InitStructure; 00503 00504 /* Enable GPIO clock */ 00505 COMx_TX_GPIO_CLK_ENABLE(COM); 00506 COMx_RX_GPIO_CLK_ENABLE(COM); 00507 00508 /* Enable USART clock */ 00509 COMx_CLK_ENABLE(COM); 00510 00511 /* Configure USART Tx as alternate function push-pull */ 00512 GPIO_InitStructure.Pin = COM_TX_PIN[COM]; 00513 GPIO_InitStructure.Mode = GPIO_MODE_AF_PP; 00514 GPIO_InitStructure.Speed = GPIO_SPEED_FREQ_HIGH; 00515 GPIO_InitStructure.Pull = GPIO_PULLUP; 00516 GPIO_InitStructure.Alternate = COM_TX_AF[COM]; 00517 HAL_GPIO_Init(COM_TX_PORT[COM], &GPIO_InitStructure); 00518 00519 /* Configure USART Rx as alternate function push-pull */ 00520 GPIO_InitStructure.Pin = COM_RX_PIN[COM]; 00521 GPIO_InitStructure.Alternate = COM_RX_AF[COM]; 00522 HAL_GPIO_Init(COM_RX_PORT[COM], &GPIO_InitStructure); 00523 00524 /* USART configuration */ 00525 huart->Instance = COM_USART[COM]; 00526 HAL_UART_Init(huart); 00527 } 00528 #endif /* HAL_UART_MODULE_ENABLED) */ 00529 /** 00530 * @} 00531 */ 00532 00533 /** @addtogroup STM32303E_EVAL_BUS 00534 * @{ 00535 */ 00536 /******************************************************************************* 00537 BUS OPERATIONS 00538 *******************************************************************************/ 00539 #ifdef HAL_I2C_MODULE_ENABLED 00540 /******************************* I2C Routines**********************************/ 00541 00542 /** 00543 * @brief Eval I2Cx MSP Initialization 00544 * @param hi2c I2C handle 00545 * @retval None 00546 */ 00547 static void I2Cx_MspInit(I2C_HandleTypeDef *hi2c) 00548 { 00549 GPIO_InitTypeDef GPIO_InitStructure; 00550 RCC_PeriphCLKInitTypeDef RCC_PeriphCLKInitStruct; 00551 00552 if (hi2c->Instance == EVAL_I2Cx) 00553 { 00554 /*##-1- Configure the Eval I2C clock source. The clock is derived from the SYSCLK #*/ 00555 RCC_PeriphCLKInitStruct.PeriphClockSelection = RCC_PERIPHCLK_I2C2; 00556 RCC_PeriphCLKInitStruct.I2c2ClockSelection = RCC_I2C2CLKSOURCE_SYSCLK; 00557 HAL_RCCEx_PeriphCLKConfig(&RCC_PeriphCLKInitStruct); 00558 00559 /*##-2- Configure the GPIOs ################################################*/ 00560 00561 /* Enable GPIO clock */ 00562 EVAL_I2Cx_SDA_GPIO_CLK_ENABLE(); 00563 EVAL_I2Cx_SCL_GPIO_CLK_ENABLE(); 00564 00565 /* Configure I2C Tx as alternate function */ 00566 GPIO_InitStructure.Pin = EVAL_I2Cx_SCL_PIN; 00567 GPIO_InitStructure.Mode = GPIO_MODE_AF_OD; 00568 GPIO_InitStructure.Pull = GPIO_NOPULL; 00569 GPIO_InitStructure.Speed = GPIO_SPEED_FREQ_HIGH; 00570 GPIO_InitStructure.Alternate = EVAL_I2Cx_SCL_SDA_AF; 00571 HAL_GPIO_Init(EVAL_I2Cx_SCL_GPIO_PORT, &GPIO_InitStructure); 00572 00573 /* Configure I2C Rx as alternate function */ 00574 GPIO_InitStructure.Pin = EVAL_I2Cx_SDA_PIN; 00575 HAL_GPIO_Init(EVAL_I2Cx_SDA_GPIO_PORT, &GPIO_InitStructure); 00576 00577 00578 /*##-3- Configure the Eval I2Cx peripheral #######################################*/ 00579 /* Enable Eval_I2Cx clock */ 00580 EVAL_I2Cx_CLK_ENABLE(); 00581 00582 /* Force the I2C Periheral Clock Reset */ 00583 EVAL_I2Cx_FORCE_RESET(); 00584 00585 /* Release the I2C Periheral Clock Reset */ 00586 EVAL_I2Cx_RELEASE_RESET(); 00587 00588 /* Enable and set Eval I2Cx Interrupt to the highest priority */ 00589 HAL_NVIC_SetPriority(EVAL_I2Cx_EV_IRQn, 0x0F, 0); 00590 HAL_NVIC_EnableIRQ(EVAL_I2Cx_EV_IRQn); 00591 00592 /* Enable and set Eval I2Cx Interrupt to the highest priority */ 00593 HAL_NVIC_SetPriority(EVAL_I2Cx_ER_IRQn, 0x0F, 0); 00594 HAL_NVIC_EnableIRQ(EVAL_I2Cx_ER_IRQn); 00595 } 00596 } 00597 00598 /** 00599 * @brief Eval I2Cx Bus initialization 00600 * @retval None 00601 */ 00602 static void I2Cx_Init(void) 00603 { 00604 if(HAL_I2C_GetState(&heval_I2c) == HAL_I2C_STATE_RESET) 00605 { 00606 heval_I2c.Instance = EVAL_I2Cx; 00607 heval_I2c.Init.Timing = EVAL_I2Cx_TIMING; 00608 heval_I2c.Init.OwnAddress1 = 0; 00609 heval_I2c.Init.AddressingMode = I2C_ADDRESSINGMODE_7BIT; 00610 heval_I2c.Init.DualAddressMode = I2C_DUALADDRESS_DISABLE; 00611 heval_I2c.Init.OwnAddress2 = 0; 00612 heval_I2c.Init.GeneralCallMode = I2C_GENERALCALL_DISABLE; 00613 heval_I2c.Init.NoStretchMode = I2C_NOSTRETCH_DISABLE; 00614 00615 /* Init the I2C */ 00616 I2Cx_MspInit(&heval_I2c); 00617 HAL_I2C_Init(&heval_I2c); 00618 } 00619 } 00620 00621 /** 00622 * @brief Write a value in a register of the device through BUS. 00623 * @param Addr Device address on BUS Bus. 00624 * @param Reg The target register address to write 00625 * @param RegSize The target register size (can be 8BIT or 16BIT) 00626 * @param Value The target register value to be written 00627 * @retval None 00628 */ 00629 static void I2Cx_WriteData(uint16_t Addr, uint8_t Reg, uint16_t RegSize, uint8_t Value) 00630 { 00631 HAL_StatusTypeDef status = HAL_OK; 00632 00633 status = HAL_I2C_Mem_Write(&heval_I2c, Addr, (uint16_t)Reg, RegSize, &Value, 1, I2cxTimeout); 00634 00635 /* Check the communication status */ 00636 if(status != HAL_OK) 00637 { 00638 /* Re-Initiaize the BUS */ 00639 I2Cx_Error(); 00640 } 00641 } 00642 00643 /** 00644 * @brief Write a value in a register of the device through BUS. 00645 * @param Addr Device address on BUS Bus. 00646 * @param Reg The target register address to write 00647 * @param RegSize The target register size (can be 8BIT or 16BIT) 00648 * @param pBuffer The target register value to be written 00649 * @param Length buffer size to be written 00650 * @retval None 00651 */ 00652 static HAL_StatusTypeDef I2Cx_WriteBuffer(uint16_t Addr, uint8_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length) 00653 { 00654 HAL_StatusTypeDef status = HAL_OK; 00655 00656 status = HAL_I2C_Mem_Write(&heval_I2c, Addr, (uint16_t)Reg, RegSize, pBuffer, Length, I2cxTimeout); 00657 00658 /* Check the communication status */ 00659 if(status != HAL_OK) 00660 { 00661 /* Re-Initiaize the BUS */ 00662 I2Cx_Error(); 00663 } 00664 return status; 00665 } 00666 00667 /** 00668 * @brief Read a register of the device through BUS 00669 * @param Addr Device address on BUS 00670 * @param Reg The target register address to read 00671 * @param RegSize The target register size (can be 8BIT or 16BIT) 00672 * @retval read register value 00673 */ 00674 static uint8_t I2Cx_ReadData(uint16_t Addr, uint8_t Reg, uint16_t RegSize) 00675 { 00676 HAL_StatusTypeDef status = HAL_OK; 00677 uint8_t value = 0; 00678 00679 status = HAL_I2C_Mem_Read(&heval_I2c, Addr, Reg, RegSize, &value, 1, I2cxTimeout); 00680 00681 /* Check the communication status */ 00682 if(status != HAL_OK) 00683 { 00684 /* Re-Initiaize the BUS */ 00685 I2Cx_Error(); 00686 00687 } 00688 return value; 00689 } 00690 00691 /** 00692 * @brief Reads multiple data on the BUS. 00693 * @param Addr I2C Address 00694 * @param Reg Reg Address 00695 * @param RegSize The target register size (can be 8BIT or 16BIT) 00696 * @param pBuffer pointer to read data buffer 00697 * @param Length length of the data 00698 * @retval 0 if no problems to read multiple data 00699 */ 00700 static HAL_StatusTypeDef I2Cx_ReadBuffer(uint16_t Addr, uint8_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length) 00701 { 00702 HAL_StatusTypeDef status = HAL_OK; 00703 00704 status = HAL_I2C_Mem_Read(&heval_I2c, Addr, (uint16_t)Reg, RegSize, pBuffer, Length, I2cxTimeout); 00705 00706 /* Check the communication status */ 00707 if(status != HAL_OK) 00708 { 00709 /* Re-Initiaize the BUS */ 00710 I2Cx_Error(); 00711 } 00712 return status; 00713 } 00714 00715 /** 00716 * @brief Checks if target device is ready for communication. 00717 * @note This function is used with Memory devices 00718 * @param DevAddress Target device address 00719 * @param Trials Number of trials 00720 * @retval HAL status 00721 */ 00722 static HAL_StatusTypeDef I2Cx_IsDeviceReady(uint16_t DevAddress, uint32_t Trials) 00723 { 00724 return (HAL_I2C_IsDeviceReady(&heval_I2c, DevAddress, Trials, I2cxTimeout)); 00725 } 00726 00727 00728 /** 00729 * @brief Eval I2Cx error treatment function 00730 * @retval None 00731 */ 00732 static void I2Cx_Error (void) 00733 { 00734 /* De-initialize the I2C communication BUS */ 00735 HAL_I2C_DeInit(&heval_I2c); 00736 00737 /* Re- Initiaize the I2C communication BUS */ 00738 I2Cx_Init(); 00739 } 00740 #endif /*HAL_I2C_MODULE_ENABLED*/ 00741 00742 /******************************* SPI Routines**********************************/ 00743 #ifdef HAL_SPI_MODULE_ENABLED 00744 /** 00745 * @brief Initializes SPI MSP. 00746 * @retval None 00747 */ 00748 static void SPIx_MspInit(SPI_HandleTypeDef *hspi) 00749 { 00750 GPIO_InitTypeDef GPIO_InitStructure; 00751 00752 /*** Configure the GPIOs ***/ 00753 /* Enable GPIO clock */ 00754 EVAL_SPIx_SCK_GPIO_CLK_ENABLE(); 00755 EVAL_SPIx_MISO_MOSI_GPIO_CLK_ENABLE(); 00756 00757 /* configure SPI SCK */ 00758 GPIO_InitStructure.Pin = EVAL_SPIx_SCK_PIN; 00759 GPIO_InitStructure.Mode = GPIO_MODE_AF_PP; 00760 GPIO_InitStructure.Pull = GPIO_PULLDOWN; 00761 GPIO_InitStructure.Speed = GPIO_SPEED_FREQ_HIGH; 00762 GPIO_InitStructure.Alternate = EVAL_SPIx_SCK_AF; 00763 HAL_GPIO_Init(EVAL_SPIx_SCK_GPIO_PORT, &GPIO_InitStructure); 00764 00765 /* configure SPI MISO and MOSI */ 00766 GPIO_InitStructure.Pin = (EVAL_SPIx_MISO_PIN | EVAL_SPIx_MOSI_PIN); 00767 GPIO_InitStructure.Mode = GPIO_MODE_AF_PP; 00768 GPIO_InitStructure.Pull = GPIO_NOPULL; 00769 GPIO_InitStructure.Speed = GPIO_SPEED_FREQ_HIGH; 00770 GPIO_InitStructure.Alternate = EVAL_SPIx_MISO_MOSI_AF; 00771 HAL_GPIO_Init(EVAL_SPIx_MISO_MOSI_GPIO_PORT, &GPIO_InitStructure); 00772 00773 /*** Configure the SPI peripheral ***/ 00774 /* Enable SPI clock */ 00775 EVAL_SPIx_CLK_ENABLE(); 00776 } 00777 00778 /** 00779 * @brief Initializes SPI HAL. 00780 * @retval None 00781 */ 00782 static void SPIx_Init(void) 00783 { 00784 if(HAL_SPI_GetState(&heval_Spi) == HAL_SPI_STATE_RESET) 00785 { 00786 /* SPI Config */ 00787 heval_Spi.Instance = EVAL_SPIx; 00788 /* SPI baudrate is set to 18 MHz (PCLK2/SPI_BaudRatePrescaler = 36/2 = 18 MHz) */ 00789 heval_Spi.Init.BaudRatePrescaler = SPI_BAUDRATEPRESCALER_2; 00790 heval_Spi.Init.Direction = SPI_DIRECTION_2LINES; 00791 heval_Spi.Init.CLKPhase = SPI_PHASE_1EDGE; 00792 heval_Spi.Init.CLKPolarity = SPI_POLARITY_LOW; 00793 heval_Spi.Init.CRCCalculation = SPI_CRCCALCULATION_DISABLE; 00794 heval_Spi.Init.CRCPolynomial = 7; 00795 heval_Spi.Init.DataSize = SPI_DATASIZE_8BIT; 00796 heval_Spi.Init.FirstBit = SPI_FIRSTBIT_MSB; 00797 heval_Spi.Init.NSS = SPI_NSS_SOFT; 00798 heval_Spi.Init.TIMode = SPI_TIMODE_DISABLE; 00799 heval_Spi.Init.Mode = SPI_MODE_MASTER; 00800 00801 SPIx_MspInit(&heval_Spi); 00802 HAL_SPI_Init(&heval_Spi); 00803 } 00804 } 00805 00806 /** 00807 * @brief SPI Read 4 bytes from device 00808 * @retval Read data 00809 */ 00810 static uint32_t SPIx_Read(void) 00811 { 00812 HAL_StatusTypeDef status = HAL_OK; 00813 uint32_t readvalue = 0; 00814 uint32_t writevalue = 0xFFFFFFFF; 00815 00816 status = HAL_SPI_TransmitReceive(&heval_Spi, (uint8_t*) &writevalue, (uint8_t*) &readvalue, 1, SpixTimeout); 00817 00818 /* Check the communication status */ 00819 if(status != HAL_OK) 00820 { 00821 /* Execute user timeout callback */ 00822 SPIx_Error(); 00823 } 00824 00825 return readvalue; 00826 } 00827 00828 /** 00829 * @brief SPI Write a byte to device 00830 * @param Value value to be written 00831 * @retval None 00832 */ 00833 static void SPIx_Write(uint8_t Value) 00834 { 00835 HAL_StatusTypeDef status = HAL_OK; 00836 00837 status = HAL_SPI_Transmit(&heval_Spi, (uint8_t*) &Value, 1, SpixTimeout); 00838 00839 /* Check the communication status */ 00840 if(status != HAL_OK) 00841 { 00842 /* Execute user timeout callback */ 00843 SPIx_Error(); 00844 } 00845 } 00846 00847 /** 00848 * @brief SPI error treatment function 00849 * @retval None 00850 */ 00851 static void SPIx_Error (void) 00852 { 00853 /* De-initialize the SPI communication BUS */ 00854 HAL_SPI_DeInit(&heval_Spi); 00855 00856 /* Re- Initiaize the SPI communication BUS */ 00857 SPIx_Init(); 00858 } 00859 /** 00860 * @} 00861 */ 00862 00863 /** @defgroup STM32303E_EVAL_LINK_OPERATIONS Link Operation functions 00864 * @{ 00865 */ 00866 00867 /********************************* LINK LCD ***********************************/ 00868 00869 /** 00870 * @brief Configures the LCD_SPI interface. 00871 * @retval None 00872 */ 00873 void LCD_IO_Init(void) 00874 { 00875 GPIO_InitTypeDef GPIO_InitStructureure; 00876 00877 /* Configure the LCD Control pins ------------------------------------------*/ 00878 LCD_NCS_GPIO_CLK_ENABLE(); 00879 00880 /* Configure NCS in Output Push-Pull mode */ 00881 GPIO_InitStructureure.Pin = LCD_NCS_PIN; 00882 GPIO_InitStructureure.Mode = GPIO_MODE_OUTPUT_PP; 00883 GPIO_InitStructureure.Pull = GPIO_NOPULL; 00884 GPIO_InitStructureure.Speed = GPIO_SPEED_FREQ_HIGH; 00885 HAL_GPIO_Init(LCD_NCS_GPIO_PORT, &GPIO_InitStructureure); 00886 00887 /* Set or Reset the control line */ 00888 LCD_CS_LOW(); 00889 LCD_CS_HIGH(); 00890 00891 SPIx_Init(); 00892 } 00893 00894 /** 00895 * @brief Write register value. 00896 * @param pData Pointer on the register value 00897 * @param Size Size of byte to transmit to the register 00898 * @retval None 00899 */ 00900 void LCD_IO_WriteMultipleData(uint8_t *pData, uint32_t Size) 00901 { 00902 uint32_t counter = 0; 00903 00904 /* Reset LCD control line CS */ 00905 LCD_CS_LOW(); 00906 00907 /* Send Start Byte */ 00908 SPIx_Write(START_BYTE | LCD_WRITE_REG); 00909 00910 for (counter = Size; counter != 0; counter--) 00911 { 00912 /* Need to invert bytes for LCD*/ 00913 SPIx_Write(*(pData+1)); 00914 SPIx_Write(*pData); 00915 counter--; 00916 pData += 2; 00917 } 00918 00919 /* Deselect : Chip Select high */ 00920 LCD_CS_HIGH(); 00921 } 00922 00923 /** 00924 * @brief register address. 00925 * @param Reg 00926 * @retval None 00927 */ 00928 void LCD_IO_WriteReg(uint8_t Reg) 00929 { 00930 /* Reset LCD control line(/CS) and Send command */ 00931 LCD_CS_LOW(); 00932 00933 /* Send Start Byte */ 00934 SPIx_Write(START_BYTE | SET_INDEX); 00935 00936 /* Write 16-bit Reg Index (High Byte is 0) */ 00937 SPIx_Write(0x00); 00938 SPIx_Write(Reg); 00939 00940 /* Deselect : Chip Select high */ 00941 LCD_CS_HIGH(); 00942 } 00943 00944 /** 00945 * @brief Read register value. 00946 * @param Reg 00947 * @retval None 00948 */ 00949 uint16_t LCD_IO_ReadData(uint16_t Reg) 00950 { 00951 uint32_t readvalue = 0; 00952 00953 /* Change BaudRate Prescaler 8 for Read */ 00954 /* Mean SPI baudrate is set to 72/8 = 9 MHz */ 00955 heval_Spi.Instance->CR1 &= 0xFFC7; 00956 heval_Spi.Instance->CR1 |= SPI_BAUDRATEPRESCALER_8; 00957 00958 /* Send Reg value to Read */ 00959 LCD_IO_WriteReg(Reg); 00960 00961 /* Reset LCD control line(/CS) and Send command */ 00962 LCD_CS_LOW(); 00963 00964 /* Send Start Byte */ 00965 SPIx_Write(START_BYTE | LCD_READ_REG); 00966 00967 /* Read Upper Byte */ 00968 SPIx_Write(0xFF); 00969 readvalue = SPIx_Read(); 00970 readvalue = readvalue << 8; 00971 readvalue |= SPIx_Read(); 00972 00973 /* Recover Baud Rate initial value */ 00974 heval_Spi.Instance->CR1 &= 0xFFC7; 00975 heval_Spi.Instance->CR1 |= heval_Spi.Init.BaudRatePrescaler; 00976 00977 HAL_Delay(10); 00978 00979 /* Deselect : Chip Select high */ 00980 LCD_CS_HIGH(); 00981 return readvalue; 00982 } 00983 00984 /** 00985 * @brief Wait for loop in ms. 00986 * @param Delay in ms. 00987 * @retval None 00988 */ 00989 void LCD_Delay (uint32_t Delay) 00990 { 00991 HAL_Delay(Delay); 00992 } 00993 00994 /******************************** LINK SD Card ********************************/ 00995 00996 /** 00997 * @brief Initializes the SD Card and put it into StandBy State (Ready for 00998 * data transfer). 00999 * @retval None 01000 */ 01001 void SD_IO_Init(void) 01002 { 01003 GPIO_InitTypeDef GPIO_InitStructureure; 01004 uint8_t counter; 01005 01006 /* SD_CS_GPIO and SD_DETECT_GPIO Periph clock enable */ 01007 SD_CS_GPIO_CLK_ENABLE(); 01008 SD_DETECT_GPIO_CLK_ENABLE(); 01009 01010 /* Configure SD_CS_PIN pin: SD Card CS pin */ 01011 GPIO_InitStructureure.Pin = SD_CS_PIN; 01012 GPIO_InitStructureure.Mode = GPIO_MODE_OUTPUT_PP; 01013 GPIO_InitStructureure.Pull = GPIO_PULLUP; 01014 GPIO_InitStructureure.Speed = GPIO_SPEED_FREQ_HIGH; 01015 HAL_GPIO_Init(SD_CS_GPIO_PORT, &GPIO_InitStructureure); 01016 01017 /* Configure SD_DETECT_PIN pin: SD Card detect pin */ 01018 GPIO_InitStructureure.Pin = SD_DETECT_PIN; 01019 GPIO_InitStructureure.Mode = GPIO_MODE_IT_RISING_FALLING; 01020 GPIO_InitStructureure.Pull = GPIO_PULLUP; 01021 HAL_GPIO_Init(SD_DETECT_GPIO_PORT, &GPIO_InitStructureure); 01022 01023 /* Enable and set SD EXTI Interrupt to the lowest priority */ 01024 HAL_NVIC_SetPriority(SD_DETECT_EXTI_IRQn, 0x0F, 0); 01025 HAL_NVIC_EnableIRQ(SD_DETECT_EXTI_IRQn); 01026 01027 /*------------Put SD in SPI mode--------------*/ 01028 /* SD SPI Config */ 01029 SPIx_Init(); 01030 01031 /* SD chip select high */ 01032 SD_CS_HIGH(); 01033 01034 /* Send dummy byte 0xFF, 10 times with CS high */ 01035 /* Rise CS and MOSI for 80 clocks cycles */ 01036 for (counter = 0; counter <= 9; counter++) 01037 { 01038 /* Send dummy byte 0xFF */ 01039 SD_IO_WriteByte(SD_DUMMY_BYTE); 01040 } 01041 } 01042 01043 /** 01044 * @brief Writes a byte on the SD. 01045 * @param Data byte to send. 01046 * @retval None 01047 */ 01048 void SD_IO_WriteByte(uint8_t Data) 01049 { 01050 /* Send the byte */ 01051 SPIx_Write(Data); 01052 } 01053 01054 /** 01055 * @brief Reads a byte from the SD. 01056 * @retval The received byte. 01057 */ 01058 uint8_t SD_IO_ReadByte(void) 01059 { 01060 uint8_t data = 0; 01061 01062 /* Change BaudRate Prescaler 4 for Read */ 01063 /* Mean SPI baudrate is set to 72/4 = 18 MHz */ 01064 heval_Spi.Instance->CR1 &= 0xFFC7; 01065 heval_Spi.Instance->CR1 |= SPI_BAUDRATEPRESCALER_4; 01066 01067 /* Get the received data */ 01068 data = SPIx_Read(); 01069 01070 /* Return the shifted data */ 01071 return data; 01072 } 01073 01074 /** 01075 * @brief Sends 5 bytes command to the SD card and get response 01076 * @param Cmd The user expected command to send to SD card. 01077 * @param Arg The command argument. 01078 * @param Crc The CRC. 01079 * @param Response Expected response from the SD card 01080 * @retval HAL_StatusTypeDef HAL Status 01081 */ 01082 HAL_StatusTypeDef SD_IO_WriteCmd(uint8_t Cmd, uint32_t Arg, uint8_t Crc, uint8_t Response) 01083 { 01084 uint32_t counter = 0x00; 01085 uint8_t frame[6]; 01086 01087 /* Prepare Frame to send */ 01088 frame[0] = (Cmd | 0x40); /* Construct byte 1 */ 01089 frame[1] = (uint8_t)(Arg >> 24); /* Construct byte 2 */ 01090 frame[2] = (uint8_t)(Arg >> 16); /* Construct byte 3 */ 01091 frame[3] = (uint8_t)(Arg >> 8); /* Construct byte 4 */ 01092 frame[4] = (uint8_t)(Arg); /* Construct byte 5 */ 01093 frame[5] = (Crc); /* Construct CRC: byte 6 */ 01094 01095 /* SD chip select low */ 01096 SD_CS_LOW(); 01097 01098 /* Send Frame */ 01099 for (counter = 0; counter < 6; counter++) 01100 { 01101 SD_IO_WriteByte(frame[counter]); /* Send the Cmd bytes */ 01102 } 01103 01104 if(Response != SD_NO_RESPONSE_EXPECTED) 01105 { 01106 return SD_IO_WaitResponse(Response); 01107 } 01108 01109 return HAL_OK; 01110 } 01111 01112 /** 01113 * @brief Waits response from the SD card 01114 * @param Response Expected response from the SD card 01115 * @retval HAL_StatusTypeDef HAL Status 01116 */ 01117 HAL_StatusTypeDef SD_IO_WaitResponse(uint8_t Response) 01118 { 01119 uint32_t timeout = 0xFFF; 01120 01121 /* Check if response is got or a timeout is happen */ 01122 while ((SD_IO_ReadByte() != Response) && timeout) 01123 { 01124 timeout--; 01125 } 01126 01127 if (timeout == 0) 01128 { 01129 /* After time out */ 01130 return HAL_TIMEOUT; 01131 } 01132 else 01133 { 01134 /* Right response got */ 01135 return HAL_OK; 01136 } 01137 } 01138 01139 /** 01140 * @brief Sends dummy byte with CS High 01141 * @retval None 01142 */ 01143 void SD_IO_WriteDummy(void) 01144 { 01145 /* SD chip select high */ 01146 SD_CS_HIGH(); 01147 01148 /* Send Dummy byte 0xFF */ 01149 SD_IO_WriteByte(SD_DUMMY_BYTE); 01150 } 01151 01152 /******************************** LINK EEPROM SPI ********************************/ 01153 01154 /** 01155 * @brief Initializes the EEPROM SPI and put it into StandBy State (Ready for 01156 * data transfer). 01157 * @retval None 01158 */ 01159 void EEPROM_SPI_IO_Init(void) 01160 { 01161 GPIO_InitTypeDef GPIO_InitStructure; 01162 01163 /* EEPROM_CS_GPIO Periph clock enable */ 01164 EEPROM_CS_GPIO_CLK_ENABLE(); 01165 01166 /* Configure EEPROM_CS_PIN pin: EEPROM SPI CS pin */ 01167 GPIO_InitStructure.Pin = EEPROM_CS_PIN; 01168 GPIO_InitStructure.Mode = GPIO_MODE_OUTPUT_PP; 01169 GPIO_InitStructure.Pull = GPIO_PULLUP; 01170 GPIO_InitStructure.Speed = GPIO_SPEED_FREQ_HIGH; 01171 HAL_GPIO_Init(EEPROM_CS_GPIO_PORT, &GPIO_InitStructure); 01172 01173 /*------------Put EEPROM in SPI mode--------------*/ 01174 /* EEPROM SPI Config */ 01175 SPIx_Init(); 01176 01177 /* EEPROM chip select high */ 01178 EEPROM_CS_HIGH(); 01179 } 01180 01181 /** 01182 * @brief Write a byte on the EEPROM. 01183 * @param Data byte to send. 01184 * @retval None 01185 */ 01186 void EEPROM_SPI_IO_WriteByte(uint8_t Data) 01187 { 01188 /* Send the byte */ 01189 SPIx_Write(Data); 01190 } 01191 01192 /** 01193 * @brief Read a byte from the EEPROM. 01194 * @retval uint8_t (The received byte). 01195 */ 01196 uint8_t EEPROM_SPI_IO_ReadByte(void) 01197 { 01198 uint8_t data = 0; 01199 01200 /* Get the received data */ 01201 data = SPIx_Read(); 01202 01203 /* Return the shifted data */ 01204 return data; 01205 } 01206 01207 /** 01208 * @brief Write data to SPI EEPROM driver 01209 * @param MemAddress Internal memory address 01210 * @param pBuffer Pointer to data buffer 01211 * @param BufferSize Amount of data to be read 01212 * @retval HAL_StatusTypeDef HAL Status 01213 */ 01214 HAL_StatusTypeDef EEPROM_SPI_IO_WriteData(uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize) 01215 { 01216 /*!< Enable the write access to the EEPROM */ 01217 /*!< Select the EEPROM: Chip Select low */ 01218 EEPROM_CS_LOW(); 01219 01220 /*!< Send "Write Enable" instruction */ 01221 SPIx_Write(EEPROM_CMD_WREN); 01222 01223 /*!< Deselect the EEPROM: Chip Select high */ 01224 EEPROM_CS_HIGH(); 01225 01226 /*!< Select the EEPROM: Chip Select low */ 01227 EEPROM_CS_LOW(); 01228 01229 /*!< Send "Write to Memory " instruction */ 01230 /* Send the byte */ 01231 SPIx_Write(EEPROM_CMD_WRITE); 01232 01233 /*!< Send MemAddress high nibble address byte to write to */ 01234 SPIx_Write((MemAddress & 0xFF0000) >> 16); 01235 01236 /*!< Send MemAddress medium nibble address byte to write to */ 01237 SPIx_Write((MemAddress & 0xFF00) >> 8); 01238 01239 /*!< Send MemAddress low nibble address byte to write to */ 01240 SPIx_Write(MemAddress & 0xFF); 01241 01242 /*!< while there is data to be written on the EEPROM */ 01243 while ((BufferSize)--) 01244 { 01245 /*!< Send the current byte */ 01246 SPIx_Write(*pBuffer); 01247 /*!< Point on the next byte to be written */ 01248 pBuffer++; 01249 } 01250 01251 /*!< Deselect the EEPROM: Chip Select high */ 01252 EEPROM_CS_HIGH(); 01253 01254 /*!< Wait the end of EEPROM writing */ 01255 EEPROM_SPI_IO_WaitEepromStandbyState(); 01256 01257 /*!< Disable the write access to the EEROM */ 01258 EEPROM_CS_LOW(); 01259 01260 /*!< Send "Write Disable" instruction */ 01261 SPIx_Write(EEPROM_CMD_WRDI); 01262 01263 /*!< Deselect the EEPROM: Chip Select high */ 01264 EEPROM_CS_HIGH(); 01265 01266 return HAL_OK; 01267 } 01268 01269 /** 01270 * @brief Read data from SPI EEPROM driver 01271 * @param MemAddress Internal memory address 01272 * @param pBuffer Pointer to data buffer 01273 * @param BufferSize Amount of data to be read 01274 * @retval HAL_StatusTypeDef HAL Status 01275 */ 01276 HAL_StatusTypeDef EEPROM_SPI_IO_ReadData(uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize) 01277 { 01278 /*!< Select the EEPROM: Chip Select low */ 01279 EEPROM_CS_LOW(); 01280 01281 /*!< Send "Write to Memory " instruction */ 01282 SPIx_Write(EEPROM_CMD_READ); 01283 01284 /*!< Send MemAddress high nibble address byte to write to */ 01285 SPIx_Write((MemAddress & 0xFF0000) >> 16); 01286 01287 /*!< Send WriteAddr medium nibble address byte to write to */ 01288 SPIx_Write((MemAddress & 0xFF00) >> 8); 01289 01290 /*!< Send WriteAddr low nibble address byte to write to */ 01291 SPIx_Write(MemAddress & 0xFF); 01292 01293 while ((BufferSize)--) /*!< while there is data to be read */ 01294 { 01295 /*!< Read a byte from the EEPROM */ 01296 *pBuffer = SPIx_Read(); 01297 /*!< Point to the next location where the byte read will be saved */ 01298 pBuffer++; 01299 } 01300 01301 /*!< Deselect the EEPROM: Chip Select high */ 01302 EEPROM_CS_HIGH(); 01303 01304 return HAL_OK; 01305 } 01306 01307 /** 01308 * @brief Wait response from the SPI EEPROM 01309 * @retval HAL_StatusTypeDef HAL Status 01310 */ 01311 HAL_StatusTypeDef EEPROM_SPI_IO_WaitEepromStandbyState(void) 01312 { 01313 uint32_t timeout = 0xFFFF; 01314 uint32_t eepromstatus; 01315 01316 /*!< Select the EEPROM: Chip Select low */ 01317 EEPROM_CS_LOW(); 01318 01319 /*!< Send "Read Status Register" instruction */ 01320 SPIx_Write(EEPROM_CMD_RDSR); 01321 01322 /*!< Loop as long as the memory is busy with a write cycle */ 01323 do 01324 { 01325 /*!< Send a dummy byte to generate the clock needed by the EEPROM 01326 and put the value of the status register in EEPROM Status variable */ 01327 eepromstatus = SPIx_Read(); 01328 timeout --; 01329 } 01330 while (((eepromstatus & EEPROM_WIP_FLAG) == SET) && timeout); /* Write in progress */ 01331 01332 /*!< Deselect the EEPROM: Chip Select high */ 01333 EEPROM_CS_HIGH(); 01334 01335 if ((eepromstatus & EEPROM_WIP_FLAG) != SET) 01336 { 01337 /* Right response got */ 01338 return HAL_OK; 01339 } 01340 else 01341 { 01342 /* After time out */ 01343 return HAL_TIMEOUT; 01344 } 01345 } 01346 #endif /* HAL_SPI_MODULE_ENABLED */ 01347 01348 #ifdef HAL_I2C_MODULE_ENABLED 01349 /********************************* LINK I2C EEPROM *****************************/ 01350 /** 01351 * @brief Initializes peripherals used by the I2C EEPROM driver. 01352 * @retval None 01353 */ 01354 void EEPROM_I2C_IO_Init(void) 01355 { 01356 I2Cx_Init(); 01357 } 01358 01359 /** 01360 * @brief Write data to I2C EEPROM driver 01361 * @param DevAddress Target device address 01362 * @param MemAddress Internal memory address 01363 * @param pBuffer Pointer to data buffer 01364 * @param BufferSize Amount of data to be sent 01365 * @retval HAL status 01366 */ 01367 HAL_StatusTypeDef EEPROM_I2C_IO_WriteData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize) 01368 { 01369 return (I2Cx_WriteBuffer(DevAddress, MemAddress, I2C_MEMADD_SIZE_16BIT, pBuffer, BufferSize)); 01370 } 01371 01372 /** 01373 * @brief Read data from I2C EEPROM driver 01374 * @param DevAddress Target device address 01375 * @param MemAddress Internal memory address 01376 * @param pBuffer Pointer to data buffer 01377 * @param BufferSize Amount of data to be read 01378 * @retval HAL status 01379 */ 01380 HAL_StatusTypeDef EEPROM_I2C_IO_ReadData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize) 01381 { 01382 return (I2Cx_ReadBuffer(DevAddress, MemAddress, I2C_MEMADD_SIZE_16BIT, pBuffer, BufferSize)); 01383 } 01384 01385 /** 01386 * @brief Checks if target device is ready for communication. 01387 * @note This function is used with Memory devices 01388 * @param DevAddress Target device address 01389 * @param Trials Number of trials 01390 * @retval HAL status 01391 */ 01392 HAL_StatusTypeDef EEPROM_I2C_IO_IsDeviceReady(uint16_t DevAddress, uint32_t Trials) 01393 { 01394 return (I2Cx_IsDeviceReady(DevAddress, Trials)); 01395 } 01396 01397 /********************************* LINK I2C TEMPERATURE SENSOR *****************************/ 01398 /** 01399 * @brief Initializes peripherals used by the I2C Temperature Sensor driver. 01400 * @retval None 01401 */ 01402 void TSENSOR_IO_Init(void) 01403 { 01404 I2Cx_Init(); 01405 } 01406 01407 /** 01408 * @brief Writes one byte to the TSENSOR. 01409 * @param DevAddress Target device address 01410 * @param pBuffer Pointer to data buffer 01411 * @param WriteAddr TSENSOR's internal address to write to. 01412 * @param Length Number of data to write 01413 * @retval None 01414 */ 01415 void TSENSOR_IO_Write(uint16_t DevAddress, uint8_t* pBuffer, uint8_t WriteAddr, uint16_t Length) 01416 { 01417 I2Cx_WriteBuffer(DevAddress, WriteAddr, I2C_MEMADD_SIZE_8BIT, pBuffer, Length); 01418 } 01419 01420 /** 01421 * @brief Reads one byte from the TSENSOR. 01422 * @param DevAddress Target device address 01423 * @param pBuffer pointer to the buffer that receives the data read from the TSENSOR. 01424 * @param ReadAddr TSENSOR's internal address to read from. 01425 * @param Length Number of data to read 01426 * @retval None 01427 */ 01428 void TSENSOR_IO_Read(uint16_t DevAddress, uint8_t* pBuffer, uint8_t ReadAddr, uint16_t Length) 01429 { 01430 I2Cx_ReadBuffer(DevAddress, ReadAddr, I2C_MEMADD_SIZE_8BIT, pBuffer, Length); 01431 } 01432 01433 /** 01434 * @brief Checks if Temperature Sensor is ready for communication. 01435 * @param DevAddress Target device address 01436 * @param Trials Number of trials 01437 * @retval HAL status 01438 */ 01439 uint16_t TSENSOR_IO_IsDeviceReady(uint16_t DevAddress, uint32_t Trials) 01440 { 01441 return (I2Cx_IsDeviceReady(DevAddress, Trials)); 01442 } 01443 01444 01445 /********************************* LINK AUDIO CODEC ***********************************/ 01446 /** 01447 * @brief Initializes peripherals used by the Audio Codec driver. 01448 * @retval None 01449 */ 01450 void AUDIO_IO_Init(void) 01451 { 01452 I2Cx_Init(); 01453 } 01454 01455 /** 01456 * @brief DeInitializes Audio low level. 01457 * @note This function is intentionally kept empty, user should define it. 01458 */ 01459 void AUDIO_IO_DeInit(void) 01460 { 01461 01462 } 01463 01464 /** 01465 * @brief Writes a single data on the Audio Codec. 01466 * @param DevAddress Target device address 01467 * @param Reg Target Register address 01468 * @param Value Data to be written 01469 * @retval None 01470 */ 01471 void AUDIO_IO_Write(uint16_t DevAddress, uint8_t Reg, uint8_t Value) 01472 { 01473 I2Cx_WriteData(DevAddress, Reg, I2C_MEMADD_SIZE_8BIT, Value); 01474 } 01475 01476 /** 01477 * @brief Reads a single data from the Audio Codec. 01478 * @param DevAddress Target device address 01479 * @param Reg Target Register address 01480 * @retval Data to be read 01481 */ 01482 uint8_t AUDIO_IO_Read(uint16_t DevAddress, uint8_t Reg) 01483 { 01484 uint8_t value; 01485 01486 value = I2Cx_ReadData(DevAddress, Reg, I2C_MEMADD_SIZE_8BIT); 01487 01488 return value; 01489 } 01490 01491 /** 01492 * @brief Wait for loop in ms. 01493 * @param Delay in ms. 01494 * @retval None 01495 */ 01496 void AUDIO_IO_Delay(uint32_t Delay) 01497 { 01498 HAL_Delay(Delay); 01499 } 01500 01501 #endif /* HAL_I2C_MODULE_ENABLED */ 01502 01503 /** 01504 * @} 01505 */ 01506 01507 /** 01508 * @} 01509 */ 01510 01511 /** 01512 * @} 01513 */ 01514 01515 /** 01516 * @} 01517 */ 01518 01519 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Wed May 31 2017 11:17:16 for STM32303E_EVAL BSP User Manual by
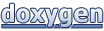