_BSP_User_Manual
|
stm3210e_eval_tsensor.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm3210e_eval_tsensor.c 00004 * @author MCD Application Team 00005 * @version $VERSION$ 00006 * @date $DATE$ 00007 * @brief This file provides a set of functions needed to manage the I2C STLM75 00008 * temperature sensor mounted on STM3210E_EVAL board . 00009 * It implements a high level communication layer for read and write 00010 * from/to this sensor. The needed STM32L152xD hardware resources (I2C and 00011 * GPIO) are defined in stm3210e_eval.h file, and the initialization is 00012 * performed in TSENSOR_IO_Init() function declared in stm3210e_eval.c 00013 * file. 00014 * You can easily tailor this driver to any other development board, 00015 * by just adapting the defines for hardware resources and 00016 * TSENSOR_IO_Init() function. 00017 * 00018 * +--------------------------------------------------------------------+ 00019 * | Pin assignment | 00020 * +----------------------------------------+--------------+------------+ 00021 * | STM3210E I2C Pins | STLM75 | Pin | 00022 * +----------------------------------------+--------------+------------+ 00023 * | EVAL_I2C2_SDA_PIN | SDA | 1 | 00024 * | EVAL_I2C2_SCL_PIN | SCL | 2 | 00025 * | EVAL_I2C2_SMBUS_PIN | OS/INT | 3 | 00026 * | . | GND | 4 (0V) | 00027 * | . | A2 | 5 | 00028 * | . | A1 | 6 | 00029 * | . | A0 | 7 | 00030 * | . | VDD | 8 (3.3V)| 00031 * +----------------------------------------+--------------+------------+ 00032 ****************************************************************************** 00033 * @attention 00034 * 00035 * <h2><center>© COPYRIGHT(c) 2014 STMicroelectronics</center></h2> 00036 * 00037 * Redistribution and use in source and binary forms, with or without modification, 00038 * are permitted provided that the following conditions are met: 00039 * 1. Redistributions of source code must retain the above copyright notice, 00040 * this list of conditions and the following disclaimer. 00041 * 2. Redistributions in binary form must reproduce the above copyright notice, 00042 * this list of conditions and the following disclaimer in the documentation 00043 * and/or other materials provided with the distribution. 00044 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00045 * may be used to endorse or promote products derived from this software 00046 * without specific prior written permission. 00047 * 00048 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00049 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00050 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00051 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00052 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00053 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00054 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00055 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00056 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00057 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00058 * 00059 ****************************************************************************** 00060 */ 00061 00062 /* Includes ------------------------------------------------------------------*/ 00063 #include "stm3210e_eval_tsensor.h" 00064 00065 /** @addtogroup BSP 00066 * @{ 00067 */ 00068 00069 /** @addtogroup STM3210E_EVAL 00070 * @{ 00071 */ 00072 00073 /** @addtogroup STM3210E_EVAL_TSENSOR STM3210E_EVAL TSENSOR 00074 * @brief This file includes the STLM75 Temperature Sensor driver of 00075 * STM3210E_EVAL board. 00076 * @{ 00077 */ 00078 00079 00080 00081 /** @defgroup STM3210E_EVAL_EVAL_TSENSOR_Private_Variables Private Variables 00082 * @{ 00083 */ 00084 static TSENSOR_DrvTypeDef *tsensor_drv; 00085 __IO uint16_t TSENSORAddress = 0; 00086 /** 00087 * @} 00088 */ 00089 00090 00091 /** @defgroup STM3210E_EVAL_EVAL_I2C_TSENSOR_Exported_Functions Exported Functions 00092 * @{ 00093 */ 00094 00095 /** 00096 * @brief Initializes peripherals used by the I2C Temperature Sensor driver. 00097 * @retval TSENSOR status 00098 */ 00099 uint32_t BSP_TSENSOR_Init(void) 00100 { 00101 uint8_t ret = TSENSOR_ERROR; 00102 TSENSOR_InitTypeDef tsensor_initstruct = {0}; 00103 00104 /* Temperature Sensor Initialization */ 00105 if(Stlm75Drv.IsReady(TSENSOR_I2C_ADDRESS_A01, TSENSOR_MAX_TRIALS) == HAL_OK) 00106 { 00107 /* Initialize the temperature sensor driver structure */ 00108 TSENSORAddress = TSENSOR_I2C_ADDRESS_A01; 00109 tsensor_drv = &Stlm75Drv; 00110 00111 ret = TSENSOR_OK; 00112 } 00113 else 00114 { 00115 if(Stlm75Drv.IsReady(TSENSOR_I2C_ADDRESS_A02, TSENSOR_MAX_TRIALS) == HAL_OK) 00116 { 00117 /* Initialize the temperature sensor driver structure */ 00118 TSENSORAddress = TSENSOR_I2C_ADDRESS_A02; 00119 tsensor_drv = &Stlm75Drv; 00120 00121 ret = TSENSOR_OK; 00122 } 00123 else 00124 { 00125 ret = TSENSOR_ERROR; 00126 } 00127 } 00128 00129 if (ret == TSENSOR_OK) 00130 { 00131 /* Configure Temperature Sensor : Conversion 9 bits in continuous mode */ 00132 /* Alert outside range Limit Temperature 12� <-> 24�c */ 00133 tsensor_initstruct.AlertMode = STLM75_INTERRUPT_MODE; 00134 tsensor_initstruct.ConversionMode = STLM75_CONTINUOUS_MODE; 00135 tsensor_initstruct.TemperatureLimitHigh = 24; 00136 tsensor_initstruct.TemperatureLimitLow = 12; 00137 00138 /* TSENSOR Init */ 00139 tsensor_drv->Init(TSENSORAddress, &tsensor_initstruct); 00140 00141 ret = TSENSOR_OK; 00142 } 00143 00144 return ret; 00145 } 00146 00147 /** 00148 * @brief Returns the Temperature Sensor status. 00149 * @retval The Temperature Sensor status. 00150 */ 00151 uint8_t BSP_TSENSOR_ReadStatus(void) 00152 { 00153 return (tsensor_drv->ReadStatus(TSENSORAddress)); 00154 } 00155 00156 /** 00157 * @brief Read Temperature register of STLM75. 00158 * @retval STLM75 measured temperature value. 00159 */ 00160 uint16_t BSP_TSENSOR_ReadTemp(void) 00161 { 00162 return tsensor_drv->ReadTemp(TSENSORAddress); 00163 00164 } 00165 00166 /** 00167 * @} 00168 */ 00169 00170 00171 /** 00172 * @} 00173 */ 00174 00175 00176 /** 00177 * @} 00178 */ 00179 00180 /** 00181 * @} 00182 */ 00183 00184 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Thu Dec 11 2014 16:16:37 for _BSP_User_Manual by
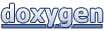