_BSP_User_Manual
|
stm3210e_eval_nor.h
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm3210e_eval_nor.h 00004 * @author MCD Application Team 00005 * @version $VERSION$ 00006 * @date $DATE$ 00007 * @brief This file contains the common defines and functions prototypes for 00008 * the stm3210e_eval_nor.c driver. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2014 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* Define to prevent recursive inclusion -------------------------------------*/ 00040 #ifndef __STM3210E_EVAL_NOR_H 00041 #define __STM3210E_EVAL_NOR_H 00042 00043 #ifdef __cplusplus 00044 extern "C" { 00045 #endif 00046 00047 /* Includes ------------------------------------------------------------------*/ 00048 #include "stm32f1xx_hal.h" 00049 00050 /** @addtogroup BSP 00051 * @{ 00052 */ 00053 00054 /** @addtogroup STM3210E_EVAL 00055 * @{ 00056 */ 00057 00058 /** @addtogroup STM3210E_EVAL_NOR 00059 * @{ 00060 */ 00061 00062 /* Exported types ------------------------------------------------------------*/ 00063 00064 /** @defgroup STM3210E_EVAL_NOR_Exported_Types Exported_Types 00065 * @{ 00066 */ 00067 00068 /** 00069 * @} 00070 */ 00071 00072 /* Exported constants --------------------------------------------------------*/ 00073 /** @defgroup STM3210E_EVAL_NOR_Exported_Constants Exported_Constants 00074 * @{ 00075 */ 00076 00077 /** 00078 * @brief NOR status structure definition 00079 */ 00080 #define NOR_STATUS_OK 0x00 00081 #define NOR_STATUS_ERROR 0x01 00082 00083 #define NOR_DEVICE_ADDR ((uint32_t)0x64000000) 00084 00085 /* #define NOR_MEMORY_WIDTH FSMC_NORSRAM_MEM_BUS_WIDTH_8 */ 00086 #define NOR_MEMORY_WIDTH FSMC_NORSRAM_MEM_BUS_WIDTH_16 00087 00088 #define NOR_BURSTACCESS FSMC_BURST_ACCESS_MODE_DISABLE 00089 /* #define NOR_BURSTACCESS FSMC_BURST_ACCESS_MODE_ENABLE*/ 00090 00091 #define NOR_WRITEBURST FSMC_WRITE_BURST_DISABLE 00092 /* #define NOR_WRITEBURST FSMC_WRITE_BURST_ENABLE */ 00093 00094 /* NOR operations Timeout definitions */ 00095 #define BLOCKERASE_TIMEOUT ((uint32_t)0x00A00000) /* NOR block erase timeout */ 00096 #define CHIPERASE_TIMEOUT ((uint32_t)0x30000000) /* NOR chip erase timeout */ 00097 #define PROGRAM_TIMEOUT ((uint32_t)0x00004400) /* NOR program timeout */ 00098 00099 /* NOR Ready/Busy signal GPIO definitions */ 00100 #define NOR_READY_BUSY_PIN GPIO_PIN_6 00101 #define NOR_READY_BUSY_GPIO GPIOD 00102 #define NOR_READY_STATE GPIO_PIN_SET 00103 #define NOR_BUSY_STATE GPIO_PIN_RESET 00104 00105 /** 00106 * @} 00107 */ 00108 00109 /* Exported macro ------------------------------------------------------------*/ 00110 00111 /** @defgroup STM3210E_EVAL_NOR_Exported_Macro Exported_Macro 00112 * @{ 00113 */ 00114 00115 /** 00116 * @} 00117 */ 00118 00119 /* Exported functions --------------------------------------------------------*/ 00120 00121 /** @addtogroup STM3210E_EVAL_NOR_Exported_Functions 00122 * @{ 00123 */ 00124 uint8_t BSP_NOR_Init(void); 00125 uint8_t BSP_NOR_ReadData(uint32_t uwStartAddress, uint16_t *pData, uint32_t uwDataSize); 00126 uint8_t BSP_NOR_WriteData(uint32_t uwStartAddress, uint16_t *pData, uint32_t uwDataSize); 00127 uint8_t BSP_NOR_ProgramData(uint32_t uwStartAddress, uint16_t *pData, uint32_t uwDataSize); 00128 uint8_t BSP_NOR_Erase_Block(uint32_t BlockAddress); 00129 uint8_t BSP_NOR_Erase_Chip(void); 00130 uint8_t BSP_NOR_Read_ID(NOR_IDTypeDef *pNOR_ID); 00131 void BSP_NOR_ReturnToReadMode(void); 00132 00133 /** 00134 * @} 00135 */ 00136 00137 /** 00138 * @} 00139 */ 00140 00141 /** 00142 * @} 00143 */ 00144 00145 /** 00146 * @} 00147 */ 00148 00149 #ifdef __cplusplus 00150 } 00151 #endif 00152 00153 #endif /* __STM3210E_EVAL_NOR_H */ 00154 00155 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Thu Dec 11 2014 16:16:37 for _BSP_User_Manual by
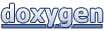