_BSP_User_Manual
|
stm3210e_eval_nand.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm3210e_eval_nand.c 00004 * @author MCD Application Team 00005 * @version $VERSION$ 00006 * @date $DATE$ 00007 * @brief This file includes a standard driver for the NAND512W3A2CN6E NAND flash memory 00008 * device mounted on STM32410E-EVAL evaluation board. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2014 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* File Info : ----------------------------------------------------------------- 00040 User NOTES 00041 1. How To use this driver: 00042 -------------------------- 00043 - This driver is used to drive the NAND512W3A2CN6E NAND flash external memory mounted 00044 on STM3210E-EVAL evaluation board. 00045 - This driver does not need a specific component driver for the NAND device 00046 to be included with. 00047 00048 2. Driver description: 00049 --------------------- 00050 + Initialization steps: 00051 o Initialize the NAND external memory using the BSP_NAND_Init() function. This 00052 function includes the MSP layer hardware resources initialization and the 00053 FMC controller configuration to interface with the external NAND memory. 00054 00055 + NAND flash operations 00056 o NAND external memory can be accessed with read/write operations once it is 00057 initialized. 00058 Read/write operation can be performed with AHB access using the functions 00059 BSP_NAND_ReadData()/BSP_NAND_WriteData(). The BSP_NAND_WriteData() performs write operation 00060 of an amount of data by unit (halfword). 00061 o The function BSP_NAND_Read_ID() returns the chip IDs stored in the structure 00062 "NAND_IDTypeDef". (see the NAND IDs in the memory data sheet) 00063 o Perform erase block operation using the function BSP_NAND_Erase_Block() and by 00064 specifying the block address. 00065 00066 ------------------------------------------------------------------------------*/ 00067 00068 /* Includes ------------------------------------------------------------------*/ 00069 #include "stm3210e_eval_nand.h" 00070 00071 /** @addtogroup BSP 00072 * @{ 00073 */ 00074 00075 /** @addtogroup STM3210E_EVAL 00076 * @{ 00077 */ 00078 00079 /** @defgroup STM3210E_EVAL_NAND STM3210E_EVAL NAND 00080 * @{ 00081 */ 00082 00083 /* Private typedef -----------------------------------------------------------*/ 00084 00085 /** @defgroup STM3210E_EVAL_NAND_Private_Types_Definitions Private_Types_Definitions 00086 * @{ 00087 */ 00088 00089 /** 00090 * @} 00091 */ 00092 00093 /* Private define ------------------------------------------------------------*/ 00094 00095 /** @defgroup STM3210E_EVAL_NAND_Private_Defines Private_Defines 00096 * @{ 00097 */ 00098 00099 /** 00100 * @} 00101 */ 00102 00103 /* Private macro -------------------------------------------------------------*/ 00104 00105 /** @defgroup STM3210E_EVAL_NAND_Private_Macros Private_Macros 00106 * @{ 00107 */ 00108 00109 /** 00110 * @} 00111 */ 00112 00113 /* Private variables ---------------------------------------------------------*/ 00114 00115 /** @defgroup STM3210E_EVAL_NAND_Private_Variables Private_Variables 00116 * @{ 00117 */ 00118 static NAND_HandleTypeDef nandHandle; 00119 static FSMC_NAND_PCC_TimingTypeDef Timing; 00120 00121 /** 00122 * @} 00123 */ 00124 00125 /* Private function prototypes -----------------------------------------------*/ 00126 00127 /** @defgroup STM3210E_EVAL_NAND_Private_Function_Prototypes Private_Function_Prototypes 00128 * @{ 00129 */ 00130 static void NAND_MspInit(void); 00131 00132 /** 00133 * @} 00134 */ 00135 00136 /* Private functions ---------------------------------------------------------*/ 00137 00138 /** @defgroup STM3210E_EVAL_NAND_Exported_Functions Exported_Functions 00139 * @{ 00140 */ 00141 00142 /** 00143 * @brief Initializes the NAND device. 00144 * @retval NAND memory status 00145 */ 00146 uint8_t BSP_NAND_Init(void) 00147 { 00148 nandHandle.Instance = FSMC_NAND_DEVICE; 00149 00150 /*NAND Configuration */ 00151 Timing.SetupTime = 0; 00152 Timing.WaitSetupTime = 2; 00153 Timing.HoldSetupTime = 1; 00154 Timing.HiZSetupTime = 0; 00155 00156 nandHandle.Init.NandBank = FSMC_NAND_BANK2; 00157 nandHandle.Init.Waitfeature = FSMC_NAND_PCC_WAIT_FEATURE_ENABLE; 00158 nandHandle.Init.MemoryDataWidth = FSMC_NAND_PCC_MEM_BUS_WIDTH_8; 00159 nandHandle.Init.EccComputation = FSMC_NAND_ECC_ENABLE; 00160 nandHandle.Init.ECCPageSize = FSMC_NAND_ECC_PAGE_SIZE_512BYTE; 00161 nandHandle.Init.TCLRSetupTime = 0; 00162 nandHandle.Init.TARSetupTime = 0; 00163 00164 nandHandle.Info.BlockNbr = NAND_MAX_ZONE; 00165 nandHandle.Info.BlockSize = NAND_BLOCK_SIZE; 00166 nandHandle.Info.ZoneSize = NAND_ZONE_SIZE; 00167 nandHandle.Info.PageSize = NAND_PAGE_SIZE; 00168 nandHandle.Info.SpareAreaSize = NAND_SPARE_AREA_SIZE; 00169 00170 /* NAND controller initialization */ 00171 NAND_MspInit(); 00172 00173 if(HAL_NAND_Init(&nandHandle, &Timing, &Timing) != HAL_OK) 00174 { 00175 return NAND_ERROR; 00176 } 00177 else 00178 { 00179 return NAND_OK; 00180 } 00181 } 00182 00183 /** 00184 * @brief Reads an amount of data from the NAND device. 00185 * @param BlockAddress: Block address to Read 00186 * @param pData: Pointer to data to be read 00187 * @param uwNumPage: Number of Pages to read to Block 00188 * @retval NAND memory status 00189 */ 00190 uint8_t BSP_NAND_ReadData(NAND_AddressTypeDef BlockAddress, uint8_t* pData, uint32_t uwNumPage) 00191 { 00192 /* Read data from NAND */ 00193 if (HAL_NAND_Read_Page(&nandHandle, &BlockAddress, pData, uwNumPage) != HAL_OK) 00194 { 00195 return NAND_ERROR; 00196 } 00197 00198 return NAND_OK; 00199 } 00200 00201 /** 00202 * @brief Writes an amount of data to the NAND device. 00203 * @param BlockAddress: Block address to Write 00204 * @param pData: Pointer to data to be written 00205 * @param uwNumPage: Number of Pages to write to Block 00206 * @retval NAND memory status 00207 */ 00208 uint8_t BSP_NAND_WriteData(NAND_AddressTypeDef BlockAddress, uint8_t* pData, uint32_t uwNumPage) 00209 { 00210 /* Write data to NAND */ 00211 if (HAL_NAND_Write_Page(&nandHandle, &BlockAddress, pData, uwNumPage) != HAL_OK) 00212 { 00213 return NAND_ERROR; 00214 } 00215 00216 return NAND_OK; 00217 } 00218 00219 /** 00220 * @brief Erases the specified block of the NAND device. 00221 * @param BlockAddress: Block address to Erase 00222 * @retval NAND memory status 00223 */ 00224 uint8_t BSP_NAND_Erase_Block(NAND_AddressTypeDef BlockAddress) 00225 { 00226 /* Send NAND erase block operation */ 00227 if (HAL_NAND_Erase_Block(&nandHandle, &BlockAddress) != HAL_OK) 00228 { 00229 return NAND_ERROR; 00230 } 00231 00232 return NAND_OK; 00233 } 00234 00235 /** 00236 * @brief Reads NAND flash IDs. 00237 * @param pNAND_ID : Pointer to NAND ID structure 00238 * @retval NAND memory status 00239 */ 00240 uint8_t BSP_NAND_Read_ID(NAND_IDTypeDef *pNAND_ID) 00241 { 00242 if(HAL_NAND_Read_ID(&nandHandle, pNAND_ID) != HAL_OK) 00243 { 00244 return NAND_ERROR; 00245 } 00246 else 00247 { 00248 return NAND_OK; 00249 } 00250 } 00251 00252 /** 00253 * @brief Initializes the NAND MSP. 00254 * @retval None 00255 */ 00256 static void NAND_MspInit(void) 00257 { 00258 GPIO_InitTypeDef gpioinitstruct = {0}; 00259 00260 /* Enable FSMC clock */ 00261 __HAL_RCC_FSMC_CLK_ENABLE(); 00262 00263 /* Enable GPIOs clock */ 00264 __HAL_RCC_GPIOD_CLK_ENABLE(); 00265 __HAL_RCC_GPIOE_CLK_ENABLE(); 00266 __HAL_RCC_GPIOF_CLK_ENABLE(); 00267 __HAL_RCC_GPIOG_CLK_ENABLE(); 00268 00269 /* Common GPIO configuration */ 00270 gpioinitstruct.Mode = GPIO_MODE_AF_PP; 00271 gpioinitstruct.Pull = GPIO_PULLUP; 00272 gpioinitstruct.Speed = GPIO_SPEED_HIGH; 00273 00274 /*-- GPIO Configuration ------------------------------------------------------*/ 00275 /*!< CLE, ALE, D0->D3, NOE, NWE and NCE2 NAND pin configuration */ 00276 gpioinitstruct.Pin = GPIO_PIN_11 | GPIO_PIN_12 | GPIO_PIN_14 | GPIO_PIN_15 | 00277 GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_4 | GPIO_PIN_5 | 00278 GPIO_PIN_7; 00279 HAL_GPIO_Init(GPIOD, &gpioinitstruct); 00280 00281 /*!< D4->D7 NAND pin configuration */ 00282 gpioinitstruct.Pin = GPIO_PIN_7 | GPIO_PIN_8 | GPIO_PIN_9 | GPIO_PIN_10; 00283 00284 HAL_GPIO_Init(GPIOE, &gpioinitstruct); 00285 00286 00287 /*!< NWAIT NAND pin configuration */ 00288 gpioinitstruct.Pin = GPIO_PIN_6; 00289 gpioinitstruct.Mode = GPIO_MODE_INPUT; 00290 00291 HAL_GPIO_Init(GPIOD, &gpioinitstruct); 00292 00293 /*!< INT2 NAND pin configuration */ 00294 gpioinitstruct.Pin = GPIO_PIN_6; 00295 HAL_GPIO_Init(GPIOG, &gpioinitstruct); 00296 } 00297 00298 /** 00299 * @} 00300 */ 00301 00302 /** 00303 * @} 00304 */ 00305 00306 /** 00307 * @} 00308 */ 00309 00310 /** 00311 * @} 00312 */ 00313 00314 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Thu Dec 11 2014 16:16:37 for _BSP_User_Manual by
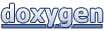