STM3210C_EVAL BSP User Manual
|
stm3210c_eval_io.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm3210c_eval_io.c 00004 * @author MCD Application Team 00005 * @version V6.0.1 00006 * @date 18-December-2015 00007 * @brief This file provides a set of functions needed to manage the IO pins 00008 * on STM3210C-EVAL evaluation board. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* File Info : ----------------------------------------------------------------- 00040 User NOTES 00041 1. How To use this driver: 00042 -------------------------- 00043 - This driver is used to drive the IO module of the STM3210C-EVAL evaluation 00044 board. 00045 - The STMPE811 IO expander device component driver must be included with this 00046 driver in order to run the IO functionalities commanded by the IO expander 00047 device mounted on the evaluation board. 00048 00049 2. Driver description: 00050 --------------------- 00051 + Initialization steps: 00052 o Initialize the IO module using the BSP_IO_Init() function. This 00053 function includes the MSP layer hardware resources initialization and the 00054 communication layer configuration to start the IO functionalities use. 00055 00056 + IO functionalities use 00057 o The IO pin mode is configured when calling the function BSP_IO_ConfigPin(), you 00058 must specify the desired IO mode by choosing the "IO_ModeTypedef" parameter 00059 predefined value. 00060 o If an IO pin is used in interrupt mode, the function BSP_IO_ITGetStatus() is 00061 needed to get the interrupt status. To clear the IT pending bits, you should 00062 call the function BSP_IO_ITClear() with specifying the IO pending bit to clear. 00063 o The IT is handled using the corresponding external interrupt IRQ handler, 00064 the user IT callback treatment is implemented on the same external interrupt 00065 callback. 00066 o To get/set an IO pin combination state you can use the functions 00067 BSP_IO_ReadPin()/BSP_IO_WritePin() or the function BSP_IO_TogglePin() to toggle the pin 00068 state. 00069 00070 ------------------------------------------------------------------------------*/ 00071 00072 /* Includes ------------------------------------------------------------------*/ 00073 #include "stm3210c_eval_io.h" 00074 00075 /** @addtogroup BSP 00076 * @{ 00077 */ 00078 00079 /** @addtogroup STM3210C_EVAL 00080 * @{ 00081 */ 00082 00083 /** @defgroup STM3210C_EVAL_IO STM3210C_EVAL IO Expander 00084 * @{ 00085 */ 00086 00087 /* Private typedef -----------------------------------------------------------*/ 00088 00089 /** @defgroup STM3210C_EVAL_IO_Private_Types_Definitions Private Types Definitions 00090 * @{ 00091 */ 00092 00093 /** 00094 * @} 00095 */ 00096 00097 /* Private define ------------------------------------------------------------*/ 00098 00099 /** @defgroup STM3210C_EVAL_IO_Private_Defines Private_Defines 00100 * @{ 00101 */ 00102 00103 /** 00104 * @} 00105 */ 00106 00107 /* Private macro -------------------------------------------------------------*/ 00108 00109 /** @defgroup STM3210C_EVAL_IO_Private_Macros Private_Macros 00110 * @{ 00111 */ 00112 00113 /** 00114 * @} 00115 */ 00116 00117 /* Private variables ---------------------------------------------------------*/ 00118 00119 /** @defgroup STM3210C_EVAL_IO_Private_Variables Private_Variables 00120 * @{ 00121 */ 00122 static IO_DrvTypeDef *io1_driver; 00123 static IO_DrvTypeDef *io2_driver; 00124 00125 00126 /** 00127 * @} 00128 */ 00129 00130 /* Private function prototypes -----------------------------------------------*/ 00131 00132 /** @defgroup STM3210C_EVAL_IO_Private_Function_Prototypes Private_Function_Prototypes 00133 * @{ 00134 */ 00135 00136 /** 00137 * @} 00138 */ 00139 00140 /* Private functions ---------------------------------------------------------*/ 00141 00142 /** @defgroup STM3210C_EVAL_IO_Exported_Functions Exported_Functions 00143 * @{ 00144 */ 00145 00146 /** 00147 * @brief Initializes and configures the IO functionalities and configures all 00148 * necessary hardware resources (GPIOs, clocks..). 00149 * @note BSP_IO_Init() is using HAL_Delay() function to ensure that stmpe811 00150 * IO Expander is correctly reset. HAL_Delay() function provides accurate 00151 * delay (in milliseconds) based on variable incremented in SysTick ISR. 00152 * This implies that if BSP_IO_Init() is called from a peripheral ISR process, 00153 * then the SysTick interrupt must have higher priority (numerically lower) 00154 * than the peripheral interrupt. Otherwise the caller ISR process will be blocked. 00155 * @retval IO_OK: if all initializations are OK. Other value if error. 00156 */ 00157 uint8_t BSP_IO_Init(void) 00158 { 00159 uint8_t ret = IO_ERROR; 00160 00161 /* Initialize IO Expander 1*/ 00162 if(stmpe811_io_drv.ReadID(IO1_I2C_ADDRESS) == STMPE811_ID) 00163 { 00164 /* Initialize the IO Expander 1 driver structure */ 00165 io1_driver = &stmpe811_io_drv; 00166 00167 io1_driver->Init(IO1_I2C_ADDRESS); 00168 io1_driver->Start(IO1_I2C_ADDRESS, IO1_PIN_ALL >> IO1_PIN_OFFSET); 00169 00170 ret = IO_OK; 00171 } 00172 00173 /* Initialize IO Expander 2*/ 00174 if(stmpe811_io_drv.ReadID(IO2_I2C_ADDRESS) == STMPE811_ID) 00175 { 00176 /* Initialize the IO Expander 2 driver structure */ 00177 io2_driver = &stmpe811_io_drv; 00178 00179 io2_driver->Init(IO2_I2C_ADDRESS); 00180 io2_driver->Start(IO2_I2C_ADDRESS, IO2_PIN_ALL >> IO2_PIN_OFFSET); 00181 00182 ret = IO_OK; 00183 } 00184 00185 return ret; 00186 } 00187 00188 /** 00189 * @brief Gets the selected pins IT status. 00190 * @param IO_Pin: Selected pins to check the status. 00191 * This parameter can be any combination of the IO pins. 00192 * @retval Status of the checked IO pin(s). 00193 */ 00194 uint32_t BSP_IO_ITGetStatus(uint32_t IO_Pin) 00195 { 00196 uint32_t status = 0; 00197 uint32_t io1_pin = 0; 00198 uint32_t io2_pin = 0; 00199 00200 io1_pin = (IO_Pin & IO1_PIN_ALL) >> IO1_PIN_OFFSET; 00201 io2_pin = (IO_Pin & IO2_PIN_ALL) >> IO2_PIN_OFFSET; 00202 00203 if (io1_pin != 0) 00204 { 00205 /* Return the IO Expander 1 Pin IT status */ 00206 status |= (io1_driver->ITStatus(IO1_I2C_ADDRESS, io1_pin)) << IO1_PIN_OFFSET; 00207 } 00208 00209 if (io2_pin != 0) 00210 { 00211 /* Return the IO Expander 2 Pin IT status */ 00212 status |= (io2_driver->ITStatus(IO2_I2C_ADDRESS, io2_pin)) << IO2_PIN_OFFSET; 00213 } 00214 00215 return status; 00216 } 00217 00218 /** 00219 * @brief Clears the selected IO IT pending bit. 00220 * @param IO_Pin: Selected pins to check the status. 00221 * This parameter can be any combination of the IO pins. 00222 * @retval None 00223 */ 00224 void BSP_IO_ITClear(uint32_t IO_Pin) 00225 { 00226 uint32_t io1_pin = 0; 00227 uint32_t io2_pin = 0; 00228 00229 io1_pin = (IO_Pin & IO1_PIN_ALL) >> IO1_PIN_OFFSET; 00230 io2_pin = (IO_Pin & IO2_PIN_ALL) >> IO2_PIN_OFFSET; 00231 00232 if (io1_pin != 0) 00233 { 00234 /* Clears the selected IO Expander 1 pin(s) mode */ 00235 io1_driver->ClearIT(IO1_I2C_ADDRESS, io1_pin); 00236 } 00237 00238 if (io2_pin != 0) 00239 { 00240 /* Clears the selected IO Expander 2 pin(s) mode */ 00241 io2_driver->ClearIT(IO2_I2C_ADDRESS, io2_pin); 00242 } 00243 } 00244 00245 /** 00246 * @brief Configures the IO pin(s) according to IO mode structure value. 00247 * @param IO_Pin: Output pin to be set or reset. 00248 * This parameter can be any combination of the IO pins. 00249 * @param IO_Mode: IO pin mode to configure 00250 * This parameter can be one of the following values: 00251 * @arg IO_MODE_INPUT 00252 * @arg IO_MODE_OUTPUT 00253 * @arg IO_MODE_IT_RISING_EDGE 00254 * @arg IO_MODE_IT_FALLING_EDGE 00255 * @arg IO_MODE_IT_LOW_LEVEL 00256 * @arg IO_MODE_IT_HIGH_LEVEL 00257 * @retval IO_OK: if all initializations are OK. Other value if error. 00258 */ 00259 uint8_t BSP_IO_ConfigPin(uint32_t IO_Pin, IO_ModeTypedef IO_Mode) 00260 { 00261 uint32_t io1_pin = 0; 00262 uint32_t io2_pin = 0; 00263 00264 io1_pin = (IO_Pin & IO1_PIN_ALL) >> IO1_PIN_OFFSET; 00265 io2_pin = (IO_Pin & IO2_PIN_ALL) >> IO2_PIN_OFFSET; 00266 00267 if (io1_pin != 0) 00268 { 00269 /* Configure the selected IO Expander 1 pin(s) mode */ 00270 io1_driver->Config(IO1_I2C_ADDRESS, io1_pin, IO_Mode); 00271 } 00272 00273 if (io2_pin != 0) 00274 { 00275 /* Configure the selected IO Expander 2 pin(s) mode */ 00276 io2_driver->Config(IO2_I2C_ADDRESS, io2_pin, IO_Mode); 00277 } 00278 00279 return IO_OK; 00280 } 00281 00282 /** 00283 * @brief Sets the selected pins state. 00284 * @param IO_Pin: Selected pins to write. 00285 * This parameter can be any combination of the IO pins. 00286 * @param PinState: New pins state to write 00287 * @retval None 00288 */ 00289 void BSP_IO_WritePin(uint32_t IO_Pin, uint8_t PinState) 00290 { 00291 uint32_t io1_pin = 0; 00292 uint32_t io2_pin = 0; 00293 00294 io1_pin = (IO_Pin & IO1_PIN_ALL) >> IO1_PIN_OFFSET; 00295 io2_pin = (IO_Pin & IO2_PIN_ALL) >> IO2_PIN_OFFSET; 00296 00297 if (io1_pin != 0) 00298 { 00299 /* Sets the IO Expander 1 selected pins state */ 00300 io1_driver->WritePin(IO1_I2C_ADDRESS, io1_pin, PinState); 00301 } 00302 00303 if (io2_pin != 0) 00304 { 00305 /* Sets the IO Expander 2 selected pins state */ 00306 io2_driver->WritePin(IO2_I2C_ADDRESS, io2_pin, PinState); 00307 } 00308 } 00309 00310 /** 00311 * @brief Gets the selected pins current state. 00312 * @param IO_Pin: Selected pins to read. 00313 * This parameter can be any combination of the IO pins. 00314 * @retval The current pins state 00315 */ 00316 uint32_t BSP_IO_ReadPin(uint32_t IO_Pin) 00317 { 00318 uint32_t pin_state = 0; 00319 uint32_t io1_pin = 0; 00320 uint32_t io2_pin = 0; 00321 00322 io1_pin = (IO_Pin & IO1_PIN_ALL) >> IO1_PIN_OFFSET; 00323 io2_pin = (IO_Pin & IO2_PIN_ALL) >> IO2_PIN_OFFSET; 00324 00325 if (io1_pin != 0) 00326 { 00327 /* Gets the IO Expander 1 selected pins current state */ 00328 pin_state |= (io1_driver->ReadPin(IO1_I2C_ADDRESS, io1_pin)) << IO1_PIN_OFFSET; 00329 } 00330 00331 if (io2_pin != 0) 00332 { 00333 /* Gets the IO Expander 2 selected pins current state */ 00334 pin_state |= (io2_driver->ReadPin(IO2_I2C_ADDRESS, io2_pin)) << IO2_PIN_OFFSET; 00335 } 00336 00337 return pin_state; 00338 } 00339 00340 /** 00341 * @brief Toggles the selected pins state 00342 * @param IO_Pin: Selected pins to toggle. 00343 * This parameter can be any combination of the IO pins. 00344 * @retval None 00345 */ 00346 void BSP_IO_TogglePin(uint32_t IO_Pin) 00347 { 00348 uint32_t io1_pin = 0; 00349 uint32_t io2_pin = 0; 00350 00351 io1_pin = (IO_Pin & IO1_PIN_ALL) >> IO1_PIN_OFFSET; 00352 io2_pin = (IO_Pin & IO2_PIN_ALL) >> IO2_PIN_OFFSET; 00353 00354 if (io1_pin != 0) 00355 { 00356 /* Toggles the IO Expander 1 selected pins state */ 00357 if(io1_driver->ReadPin(IO1_I2C_ADDRESS, io1_pin) == RESET) /* Set */ 00358 { 00359 BSP_IO_WritePin(io1_pin, GPIO_PIN_SET); /* Reset */ 00360 } 00361 else 00362 { 00363 BSP_IO_WritePin(io1_pin, GPIO_PIN_RESET); /* Set */ 00364 } 00365 } 00366 00367 if (io2_pin != 0) 00368 { 00369 /* Toggles the IO Expander 2 selected pins state */ 00370 if(io2_driver->ReadPin(IO2_I2C_ADDRESS, io2_pin) == RESET) /* Set */ 00371 { 00372 BSP_IO_WritePin(io2_pin, GPIO_PIN_SET); /* Reset */ 00373 } 00374 else 00375 { 00376 BSP_IO_WritePin(io2_pin, GPIO_PIN_RESET); /* Set */ 00377 } 00378 } 00379 } 00380 00381 /** 00382 * @} 00383 */ 00384 00385 /** 00386 * @} 00387 */ 00388 00389 /** 00390 * @} 00391 */ 00392 00393 /** 00394 * @} 00395 */ 00396 00397 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Thu Dec 10 2015 17:13:52 for STM3210C_EVAL BSP User Manual by
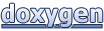