STM3210C_EVAL BSP User Manual
|
This file provides the Audio driver for the STM3210C-Eval board. More...
#include "stm3210c_eval_audio.h"
Go to the source code of this file.
Functions | |
static void | I2SOUT_MspInit (void) |
AUDIO OUT I2S MSP Init. | |
static void | I2SOUT_Init (uint32_t AudioFreq) |
Initializes the Audio Codec audio interface (I2S) | |
uint8_t | BSP_AUDIO_OUT_Init (uint16_t OutputDevice, uint8_t Volume, uint32_t AudioFreq) |
Configure the audio peripherals. | |
uint8_t | BSP_AUDIO_OUT_Play (uint16_t *pBuffer, uint32_t Size) |
Starts playing audio stream from a data buffer for a determined size. | |
void | BSP_AUDIO_OUT_ChangeBuffer (uint16_t *pData, uint16_t Size) |
Sends n-Bytes on the I2S interface. | |
uint8_t | BSP_AUDIO_OUT_Pause (void) |
This function Pauses the audio file stream. | |
uint8_t | BSP_AUDIO_OUT_Resume (void) |
This function Resumes the audio file stream. | |
uint8_t | BSP_AUDIO_OUT_Stop (uint32_t Option) |
Stops audio playing and Power down the Audio Codec. | |
uint8_t | BSP_AUDIO_OUT_SetVolume (uint8_t Volume) |
Controls the current audio volume level. | |
uint8_t | BSP_AUDIO_OUT_SetMute (uint32_t Cmd) |
Enables or disables the MUTE mode by software. | |
uint8_t | BSP_AUDIO_OUT_SetOutputMode (uint8_t Output) |
Switch dynamically (while audio file is played) the output target (speaker or headphone). | |
void | BSP_AUDIO_OUT_SetFrequency (uint32_t AudioFreq) |
Update the audio frequency. | |
void | HAL_I2S_TxCpltCallback (I2S_HandleTypeDef *hi2s) |
Tx Transfer completed callbacks. | |
void | HAL_I2S_TxHalfCpltCallback (I2S_HandleTypeDef *hi2s) |
Tx Transfer Half completed callbacks. | |
void | HAL_I2S_ErrorCallback (I2S_HandleTypeDef *hi2s) |
I2S error callbacks. | |
__weak void | BSP_AUDIO_OUT_TransferComplete_CallBack (void) |
Manages the DMA full Transfer complete event. | |
__weak void | BSP_AUDIO_OUT_HalfTransfer_CallBack (void) |
Manages the DMA Half Transfer complete event. | |
__weak void | BSP_AUDIO_OUT_Error_CallBack (void) |
Manages the DMA FIFO error event. | |
Variables | |
static AUDIO_DrvTypeDef * | pAudioDrv |
I2S_HandleTypeDef | hAudioOutI2s |
Detailed Description
This file provides the Audio driver for the STM3210C-Eval board.
- Attention:
© COPYRIGHT(c) 2015 STMicroelectronics
Redistribution and use in source and binary forms, with or without modification, are permitted provided that the following conditions are met: 1. Redistributions of source code must retain the above copyright notice, this list of conditions and the following disclaimer. 2. Redistributions in binary form must reproduce the above copyright notice, this list of conditions and the following disclaimer in the documentation and/or other materials provided with the distribution. 3. Neither the name of STMicroelectronics nor the names of its contributors may be used to endorse or promote products derived from this software without specific prior written permission.
THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
Definition in file stm3210c_eval_audio.c.
Generated on Thu Dec 10 2015 17:13:52 for STM3210C_EVAL BSP User Manual by
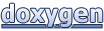