STM32072B_EVAL BSP User Manual
|
stm32072b_eval.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32072b_eval.c 00004 * @author MCD Application Team 00005 * @brief This file provides: a set of firmware functions to manage Leds, 00006 * push-button and COM ports 00007 ****************************************************************************** 00008 * @attention 00009 * 00010 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00011 * 00012 * Redistribution and use in source and binary forms, with or without modification, 00013 * are permitted provided that the following conditions are met: 00014 * 1. Redistributions of source code must retain the above copyright notice, 00015 * this list of conditions and the following disclaimer. 00016 * 2. Redistributions in binary form must reproduce the above copyright notice, 00017 * this list of conditions and the following disclaimer in the documentation 00018 * and/or other materials provided with the distribution. 00019 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00020 * may be used to endorse or promote products derived from this software 00021 * without specific prior written permission. 00022 * 00023 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00024 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00025 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00026 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00027 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00028 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00029 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00030 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00031 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00032 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00033 * 00034 ****************************************************************************** 00035 */ 00036 00037 /* Includes ------------------------------------------------------------------*/ 00038 #include "stm32072b_eval.h" 00039 00040 /** @addtogroup BSP 00041 * @{ 00042 */ 00043 00044 /** @addtogroup STM32072B_EVAL 00045 * @{ 00046 */ 00047 00048 /** @addtogroup STM32072B_EVAL_Common 00049 * @brief This file provides firmware functions to manage Leds, push-buttons, 00050 * COM ports, SD card on SPI and temperature sensor (LM75) available on 00051 * STM32072B-EVAL evaluation board from STMicroelectronics. 00052 * @{ 00053 */ 00054 00055 /** @defgroup STM32072B_EVAL_Private_Constants Private Constants 00056 * @{ 00057 */ 00058 /* LINK LCD */ 00059 #define START_BYTE 0x70 00060 #define SET_INDEX 0x00 00061 #define READ_STATUS 0x01 00062 #define LCD_WRITE_REG 0x02 00063 #define LCD_READ_REG 0x03 00064 00065 /* LINK SD Card */ 00066 #define SD_DUMMY_BYTE 0xFF 00067 #define SD_NO_RESPONSE_EXPECTED 0x80 00068 00069 /** 00070 * @brief STM32072B EVAL BSP Driver version number V2.1.8 00071 */ 00072 #define __STM32072B_EVAL_BSP_VERSION_MAIN (0x02) /*!< [31:24] main version */ 00073 #define __STM32072B_EVAL_BSP_VERSION_SUB1 (0x01) /*!< [23:16] sub1 version */ 00074 #define __STM32072B_EVAL_BSP_VERSION_SUB2 (0x08) /*!< [15:8] sub2 version */ 00075 #define __STM32072B_EVAL_BSP_VERSION_RC (0x00) /*!< [7:0] release candidate */ 00076 #define __STM32072B_EVAL_BSP_VERSION ((__STM32072B_EVAL_BSP_VERSION_MAIN << 24)\ 00077 |(__STM32072B_EVAL_BSP_VERSION_SUB1 << 16)\ 00078 |(__STM32072B_EVAL_BSP_VERSION_SUB2 << 8 )\ 00079 |(__STM32072B_EVAL_BSP_VERSION_RC)) 00080 /** 00081 * @} 00082 */ 00083 00084 /** @defgroup STM32072B_EVAL_Private_Variables Private Variables 00085 * @{ 00086 */ 00087 /** 00088 * @brief LED variables 00089 */ 00090 GPIO_TypeDef* LED_PORT[LEDn] = {LED1_GPIO_PORT, 00091 LED2_GPIO_PORT, 00092 LED3_GPIO_PORT, 00093 LED4_GPIO_PORT}; 00094 00095 const uint16_t LED_PIN[LEDn] = {LED1_PIN, 00096 LED2_PIN, 00097 LED3_PIN, 00098 LED4_PIN}; 00099 /** 00100 * @brief BUTTON variables 00101 */ 00102 GPIO_TypeDef* BUTTON_PORT[BUTTONn] = {TAMPER_BUTTON_GPIO_PORT}; 00103 const uint16_t BUTTON_PIN[BUTTONn] = {TAMPER_BUTTON_PIN}; 00104 const uint8_t BUTTON_IRQn[BUTTONn] = {TAMPER_BUTTON_EXTI_IRQn}; 00105 00106 /** 00107 * @brief JOYSTICK variables 00108 */ 00109 GPIO_TypeDef* JOY_PORT[JOYn] = {SEL_JOY_GPIO_PORT, 00110 DOWN_JOY_GPIO_PORT, 00111 LEFT_JOY_GPIO_PORT, 00112 RIGHT_JOY_GPIO_PORT, 00113 UP_JOY_GPIO_PORT}; 00114 00115 const uint16_t JOY_PIN[JOYn] = {SEL_JOY_PIN, 00116 DOWN_JOY_PIN, 00117 LEFT_JOY_PIN, 00118 RIGHT_JOY_PIN, 00119 UP_JOY_PIN}; 00120 00121 const uint8_t JOY_IRQn[JOYn] = {SEL_JOY_EXTI_IRQn, 00122 DOWN_JOY_EXTI_IRQn, 00123 LEFT_JOY_EXTI_IRQn, 00124 RIGHT_JOY_EXTI_IRQn, 00125 UP_JOY_EXTI_IRQn}; 00126 00127 /** 00128 * @brief COM variables 00129 */ 00130 #ifdef HAL_UART_MODULE_ENABLED 00131 USART_TypeDef* COM_USART[COMn] = {EVAL_COM1}; 00132 00133 GPIO_TypeDef* COM_TX_PORT[COMn] = {EVAL_COM1_TX_GPIO_PORT}; 00134 00135 GPIO_TypeDef* COM_RX_PORT[COMn] = {EVAL_COM1_RX_GPIO_PORT}; 00136 00137 const uint16_t COM_TX_PIN[COMn] = {EVAL_COM1_TX_PIN}; 00138 00139 const uint16_t COM_RX_PIN[COMn] = {EVAL_COM1_RX_PIN}; 00140 00141 const uint16_t COM_TX_AF[COMn] = {EVAL_COM1_TX_AF}; 00142 00143 const uint16_t COM_RX_AF[COMn] = {EVAL_COM1_RX_AF}; 00144 00145 #endif /*HAL_UART_MODULE_ENABLED*/ 00146 00147 /** 00148 * @brief BUS variables 00149 */ 00150 #if defined(HAL_I2C_MODULE_ENABLED) 00151 uint32_t I2c1Timeout = EVAL_I2C1_TIMEOUT_MAX; /*<! Value of Timeout when I2C1 communication fails */ 00152 uint32_t I2c2Timeout = EVAL_I2C2_TIMEOUT_MAX; /*<! Value of Timeout when I2C2 communication fails */ 00153 I2C_HandleTypeDef heval_I2c1; 00154 I2C_HandleTypeDef heval_I2c2; 00155 #endif /* HAL_I2C_MODULE_ENABLED */ 00156 00157 #if defined(HAL_SPI_MODULE_ENABLED) 00158 uint32_t SpixTimeout = EVAL_SPIx_TIMEOUT_MAX; /*<! Value of Timeout when SPI communication fails */ 00159 static SPI_HandleTypeDef heval_Spi; 00160 #endif /* HAL_SPI_MODULE_ENABLED */ 00161 00162 /** 00163 * @} 00164 */ 00165 00166 /** @defgroup STM32072B_EVAL_BUS_Operations_Functions BUS Operations Functions 00167 * @{ 00168 */ 00169 #if defined(HAL_I2C_MODULE_ENABLED) 00170 /* I2Cx bus function */ 00171 /* Link function for I2C EEPROM, TSENSOR & HDMI_SOURCE peripherals */ 00172 static void I2C1_Init(void); 00173 static void I2C1_Error (void); 00174 static void I2C1_MspInit(I2C_HandleTypeDef *hi2c); 00175 /* Link function for I2C EEPROM & TSENSOR peripherals */ 00176 static HAL_StatusTypeDef I2C1_WriteBuffer(uint16_t Addr, uint8_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length); 00177 static HAL_StatusTypeDef I2C1_ReadBuffer(uint16_t Addr, uint8_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length); 00178 static HAL_StatusTypeDef I2C1_IsDeviceReady(uint16_t DevAddress, uint32_t Trials); 00179 /* Link function for HDMI_SOURCE peripheral */ 00180 static HAL_StatusTypeDef I2C1_TransmitData(uint8_t *pBuffer, uint16_t Length); 00181 00182 /* Link function for I2C HDMI_SINK peripherals */ 00183 static void I2C2_Init(void); 00184 static void I2C2_Error(void); 00185 static void I2C2_MspInit(I2C_HandleTypeDef *hi2c); 00186 /* Link function for HDMI_SINK peripheral */ 00187 static HAL_StatusTypeDef I2C2_ReceiveData(uint16_t Addr, uint8_t * pBuffer, uint16_t Length); 00188 00189 /** 00190 * @} 00191 */ 00192 00193 /** @defgroup STM32072B_EVAL_LINK_Operations_Functions LINK Operations Functions 00194 * @{ 00195 */ 00196 00197 /* Link functions for EEPROM peripheral */ 00198 void EEPROM_IO_Init(void); 00199 HAL_StatusTypeDef EEPROM_IO_WriteData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize); 00200 HAL_StatusTypeDef EEPROM_IO_ReadData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize); 00201 HAL_StatusTypeDef EEPROM_IO_IsDeviceReady(uint16_t DevAddress, uint32_t Trials); 00202 00203 /* Link functions for Temperature Sensor peripheral */ 00204 void TSENSOR_IO_Init(void); 00205 void TSENSOR_IO_Write(uint16_t DevAddress, uint8_t* pBuffer, uint8_t WriteAddr, uint16_t Length); 00206 void TSENSOR_IO_Read(uint16_t DevAddress, uint8_t* pBuffer, uint8_t ReadAddr, uint16_t Length); 00207 uint16_t TSENSOR_IO_IsDeviceReady(uint16_t DevAddress, uint32_t Trials); 00208 00209 /* Link functions for CEC peripheral */ 00210 void HDMI_CEC_IO_Init(void); 00211 HAL_StatusTypeDef HDMI_CEC_IO_WriteData(uint8_t * pBuffer, uint16_t BufferSize); 00212 HAL_StatusTypeDef HDMI_CEC_IO_ReadData(uint16_t DevAddress, uint8_t * pBuffer, uint16_t BufferSize); 00213 #endif /* HAL_I2C_MODULE_ENABLED */ 00214 /** 00215 * @} 00216 */ 00217 00218 /** @addtogroup STM32072B_EVAL_BUS_Operations_Functions 00219 * @{ 00220 */ 00221 00222 #if defined(HAL_SPI_MODULE_ENABLED) 00223 /* SPIx bus function */ 00224 static void SPIx_Init(void); 00225 static void SPIx_Write(uint8_t Value); 00226 static void SPIx_WriteReadData(const uint8_t *DataIn, uint8_t *DataOut, uint16_t DataLegnth); 00227 static void SPIx_FlushFifo(void); 00228 static uint32_t SPIx_Read(void); 00229 static void SPIx_Error (void); 00230 static void SPIx_MspInit(SPI_HandleTypeDef *hspi); 00231 /** 00232 * @} 00233 */ 00234 00235 /** @addtogroup STM32072B_EVAL_LINK_Operations_Functions 00236 * @{ 00237 */ 00238 /* Link functions for LCD peripheral */ 00239 void LCD_IO_Init(void); 00240 void LCD_IO_WriteMultipleData(uint8_t *pData, uint32_t Size); 00241 void LCD_IO_WriteReg(uint8_t Reg); 00242 uint16_t LCD_IO_ReadData(uint16_t RegValue); 00243 void LCD_Delay(uint32_t delay); 00244 00245 /* Link functions for SD Card peripheral */ 00246 void SD_IO_Init(void); 00247 void SD_IO_CSState(uint8_t state); 00248 void SD_IO_WriteReadData(const uint8_t *DataIn, uint8_t *DataOut, uint16_t DataLength); 00249 uint8_t SD_IO_WriteByte(uint8_t Data); 00250 #endif /* HAL_SPI_MODULE_ENABLED */ 00251 00252 /** 00253 * @} 00254 */ 00255 00256 /** @addtogroup STM32072B_EVAL_Exported_Functions 00257 * @{ 00258 */ 00259 00260 /** 00261 * @brief This method returns the STM32F072B EVAL BSP Driver revision 00262 * @retval version : 0xXYZR (8bits for each decimal, R for RC) 00263 */ 00264 uint32_t BSP_GetVersion(void) 00265 { 00266 return __STM32072B_EVAL_BSP_VERSION; 00267 } 00268 00269 /** 00270 * @brief Configures LED GPIO. 00271 * @param Led Specifies the Led to be configured. 00272 * This parameter can be one of following parameters: 00273 * @arg LED1 00274 * @arg LED2 00275 * @arg LED3 00276 * @arg LED4 00277 * @retval None 00278 */ 00279 void BSP_LED_Init(Led_TypeDef Led) 00280 { 00281 GPIO_InitTypeDef GPIO_InitStruct; 00282 00283 /* Enable the GPIO_LED clock */ 00284 LEDx_GPIO_CLK_ENABLE(Led); 00285 00286 /* Configure the GPIO_LED pin */ 00287 GPIO_InitStruct.Pin = LED_PIN[Led]; 00288 GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP; 00289 GPIO_InitStruct.Pull = GPIO_PULLUP; 00290 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_HIGH; 00291 00292 HAL_GPIO_Init(LED_PORT[Led], &GPIO_InitStruct); 00293 00294 HAL_GPIO_WritePin(LED_PORT[Led], LED_PIN[Led], GPIO_PIN_SET); 00295 } 00296 00297 /** 00298 * @brief Turns selected LED On. 00299 * @param Led Specifies the Led to be set on. 00300 * This parameter can be one of following parameters: 00301 * @arg LED1 00302 * @arg LED2 00303 * @arg LED3 00304 * @arg LED4 00305 * @retval None 00306 */ 00307 void BSP_LED_On(Led_TypeDef Led) 00308 { 00309 HAL_GPIO_WritePin(LED_PORT[Led], LED_PIN[Led], GPIO_PIN_RESET); 00310 } 00311 00312 /** 00313 * @brief Turns selected LED Off. 00314 * @param Led Specifies the Led to be set off. 00315 * This parameter can be one of following parameters: 00316 * @arg LED1 00317 * @arg LED2 00318 * @arg LED3 00319 * @arg LED4 00320 * @retval None 00321 */ 00322 void BSP_LED_Off(Led_TypeDef Led) 00323 { 00324 HAL_GPIO_WritePin(LED_PORT[Led], LED_PIN[Led], GPIO_PIN_SET); 00325 } 00326 00327 /** 00328 * @brief Toggles the selected LED. 00329 * @param Led Specifies the Led to be toggled. 00330 * This parameter can be one of following parameters: 00331 * @arg LED1 00332 * @arg LED2 00333 * @arg LED3 00334 * @arg LED4 00335 * @retval None 00336 */ 00337 void BSP_LED_Toggle(Led_TypeDef Led) 00338 { 00339 HAL_GPIO_TogglePin(LED_PORT[Led], LED_PIN[Led]); 00340 } 00341 00342 /** 00343 * @brief Configures Tamper Button GPIO or EXTI Line. 00344 * @param Button Button to be configured 00345 * This parameter can be one of the following values: 00346 * @arg BUTTON_TAMPER: Tamper Push Button 00347 * @param Mode Button mode 00348 * This parameter can be one of the following values: 00349 * @arg BUTTON_MODE_GPIO: Button will be used as simple IO 00350 * @arg BUTTON_MODE_EXTI: Button will be connected to EXTI line 00351 * with interrupt generation capability 00352 * @retval None 00353 */ 00354 void BSP_PB_Init(Button_TypeDef Button, ButtonMode_TypeDef Mode) 00355 { 00356 GPIO_InitTypeDef GPIO_InitStruct; 00357 00358 /* Enable the Tamper Clock */ 00359 TAMPERx_GPIO_CLK_ENABLE(Button); 00360 00361 GPIO_InitStruct.Pin = BUTTON_PIN[Button]; 00362 GPIO_InitStruct.Pull = GPIO_PULLDOWN; 00363 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_HIGH; 00364 00365 if (Mode == BUTTON_MODE_GPIO) 00366 { 00367 /* Configure Button pin as input */ 00368 GPIO_InitStruct.Mode = GPIO_MODE_INPUT; 00369 00370 HAL_GPIO_Init(BUTTON_PORT[Button], &GPIO_InitStruct); 00371 } 00372 00373 if (Mode == BUTTON_MODE_EXTI) 00374 { 00375 /* Configure Button pin as input with External interrupt */ 00376 GPIO_InitStruct.Mode = GPIO_MODE_IT_FALLING; 00377 HAL_GPIO_Init(BUTTON_PORT[Button], &GPIO_InitStruct); 00378 00379 /* Enable and set Button EXTI Interrupt to the lowest priority */ 00380 HAL_NVIC_SetPriority((IRQn_Type)(BUTTON_IRQn[Button]), 0x03, 0x00); 00381 HAL_NVIC_EnableIRQ((IRQn_Type)(BUTTON_IRQn[Button])); 00382 } 00383 } 00384 00385 /** 00386 * @brief Returns the selected button state. 00387 * @param Button Button to be checked. 00388 * This parameter can be one of the following values: 00389 * @arg BUTTON_TAMPER: Tamper Push Button 00390 * @retval The Button GPIO pin value 00391 */ 00392 uint32_t BSP_PB_GetState(Button_TypeDef Button) 00393 { 00394 return HAL_GPIO_ReadPin(BUTTON_PORT[Button], BUTTON_PIN[Button]); 00395 } 00396 00397 /** 00398 * @brief Configures joystick GPIO and EXTI modes. 00399 * @param Joy_Mode Button mode. 00400 * This parameter can be one of the following values: 00401 * @arg JOY_MODE_GPIO: Joystick pins will be used as simple IOs 00402 * @arg JOY_MODE_EXTI: Joystick pins will be connected to EXTI line 00403 * with interrupt generation capability 00404 * @retval HAL_OK: if all initializations are OK. Other value if error. 00405 */ 00406 uint8_t BSP_JOY_Init(JOYMode_TypeDef Joy_Mode) 00407 { 00408 JOYState_TypeDef joykey; 00409 GPIO_InitTypeDef GPIO_InitStruct; 00410 00411 /* Initialized the Joystick. */ 00412 for(joykey = JOY_SEL; joykey < (JOY_SEL+JOYn) ; joykey++) 00413 { 00414 /* Enable the JOY clock */ 00415 JOYx_GPIO_CLK_ENABLE(joykey); 00416 00417 GPIO_InitStruct.Pin = JOY_PIN[joykey]; 00418 GPIO_InitStruct.Pull = GPIO_PULLDOWN; 00419 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_HIGH; 00420 00421 if (Joy_Mode == JOY_MODE_GPIO) 00422 { 00423 /* Configure Joy pin as input */ 00424 GPIO_InitStruct.Mode = GPIO_MODE_INPUT; 00425 HAL_GPIO_Init(JOY_PORT[joykey], &GPIO_InitStruct); 00426 } 00427 00428 if (Joy_Mode == JOY_MODE_EXTI) 00429 { 00430 /* Configure Joy pin as input with External interrupt */ 00431 GPIO_InitStruct.Mode = GPIO_MODE_IT_RISING; 00432 HAL_GPIO_Init(JOY_PORT[joykey], &GPIO_InitStruct); 00433 00434 /* Enable and set Joy EXTI Interrupt to the lowest priority */ 00435 HAL_NVIC_SetPriority((IRQn_Type)(JOY_IRQn[joykey]), 0x03, 0x00); 00436 HAL_NVIC_EnableIRQ((IRQn_Type)(JOY_IRQn[joykey])); 00437 } 00438 } 00439 00440 return HAL_OK; 00441 } 00442 00443 /** 00444 * @brief Returns the current joystick status. 00445 * @retval Code of the joystick key pressed 00446 * This code can be one of the following values: 00447 * @arg JOY_NONE 00448 * @arg JOY_SEL 00449 * @arg JOY_DOWN 00450 * @arg JOY_LEFT 00451 * @arg JOY_RIGHT 00452 * @arg JOY_UP 00453 */ 00454 JOYState_TypeDef BSP_JOY_GetState(void) 00455 { 00456 JOYState_TypeDef joykey; 00457 00458 for (joykey = JOY_SEL; joykey < (JOY_SEL + JOYn) ; joykey++) 00459 { 00460 if (HAL_GPIO_ReadPin(JOY_PORT[joykey], JOY_PIN[joykey]) == GPIO_PIN_SET) 00461 { 00462 /* Return Code Joystick key pressed */ 00463 return joykey; 00464 } 00465 } 00466 00467 /* No Joystick key pressed */ 00468 return JOY_NONE; 00469 } 00470 00471 #if defined(HAL_UART_MODULE_ENABLED) 00472 /** 00473 * @brief Configures COM port. 00474 * @param COM Specifies the COM port to be configured. 00475 * This parameter can be one of following parameters: 00476 * @arg COM1 00477 * @param huart pointer to a UART_HandleTypeDef structure that 00478 * contains the configuration information for the specified UART peripheral. 00479 * @retval None 00480 */ 00481 void BSP_COM_Init(COM_TypeDef COM, UART_HandleTypeDef* huart) 00482 { 00483 GPIO_InitTypeDef GPIO_InitStruct; 00484 00485 /* Enable GPIO clock */ 00486 COMx_TX_GPIO_CLK_ENABLE(COM); 00487 COMx_RX_GPIO_CLK_ENABLE(COM); 00488 00489 /* Enable USART clock */ 00490 COMx_CLK_ENABLE(COM); 00491 00492 /* Configure USART Tx as alternate function push-pull */ 00493 GPIO_InitStruct.Pin = COM_TX_PIN[COM]; 00494 GPIO_InitStruct.Mode = GPIO_MODE_AF_PP; 00495 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_HIGH; 00496 GPIO_InitStruct.Pull = GPIO_PULLUP; 00497 GPIO_InitStruct.Alternate = COM_TX_AF[COM]; 00498 HAL_GPIO_Init(COM_TX_PORT[COM], &GPIO_InitStruct); 00499 00500 /* Configure USART Rx as alternate function push-pull */ 00501 GPIO_InitStruct.Pin = COM_RX_PIN[COM]; 00502 GPIO_InitStruct.Alternate = COM_RX_AF[COM]; 00503 HAL_GPIO_Init(COM_RX_PORT[COM], &GPIO_InitStruct); 00504 00505 /* USART configuration */ 00506 huart->Instance = COM_USART[COM]; 00507 HAL_UART_Init(huart); 00508 } 00509 #endif /* HAL_UART_MODULE_ENABLED */ 00510 00511 /** 00512 * @} 00513 */ 00514 00515 /** @addtogroup STM32072B_EVAL_BUS_Operations_Functions 00516 * @{ 00517 */ 00518 00519 /******************************************************************************* 00520 BUS OPERATIONS 00521 *******************************************************************************/ 00522 #if defined(HAL_I2C_MODULE_ENABLED) 00523 /******************************* I2C Routines *********************************/ 00524 00525 /** 00526 * @brief I2C Bus initialization 00527 * @retval None 00528 */ 00529 static void I2C1_Init(void) 00530 { 00531 if(HAL_I2C_GetState(&heval_I2c1) == HAL_I2C_STATE_RESET) 00532 { 00533 heval_I2c1.Instance = EVAL_I2C1; 00534 heval_I2c1.Init.Timing = I2C1_TIMING; 00535 heval_I2c1.Init.OwnAddress1 = 0; 00536 heval_I2c1.Init.AddressingMode = I2C_ADDRESSINGMODE_7BIT; 00537 heval_I2c1.Init.DualAddressMode = I2C_DUALADDRESS_DISABLE; 00538 heval_I2c1.Init.OwnAddress2 = 0; 00539 heval_I2c1.Init.OwnAddress2Masks = I2C_OA2_NOMASK; 00540 heval_I2c1.Init.GeneralCallMode = I2C_GENERALCALL_DISABLE; 00541 heval_I2c1.Init.NoStretchMode = I2C_NOSTRETCH_DISABLE; 00542 00543 /* Init the I2C */ 00544 I2C1_MspInit(&heval_I2c1); 00545 HAL_I2C_Init(&heval_I2c1); 00546 } 00547 } 00548 00549 /** 00550 * @brief Reads multiple data on the BUS. 00551 * @param Addr I2C Address 00552 * @param Reg Reg Address 00553 * @param RegSize The target register size (can be 8BIT or 16BIT) 00554 * @param pBuffer pointer to read data buffer 00555 * @param Length length of the data 00556 * @retval 0 if no problems to read multiple data 00557 */ 00558 static HAL_StatusTypeDef I2C1_ReadBuffer(uint16_t Addr, uint8_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length) 00559 { 00560 HAL_StatusTypeDef status = HAL_OK; 00561 00562 status = HAL_I2C_Mem_Read(&heval_I2c1, Addr, Reg, RegSize, pBuffer, Length, I2c1Timeout); 00563 00564 /* Check the communication status */ 00565 if(status != HAL_OK) 00566 { 00567 /* Re-Initiaize the BUS */ 00568 I2C1_Error(); 00569 } 00570 return status; 00571 } 00572 00573 /** 00574 * @brief Checks if target device is ready for communication. 00575 * @note This function is used with Memory devices 00576 * @param DevAddress Target device address 00577 * @param Trials Number of trials 00578 * @retval HAL status 00579 */ 00580 static HAL_StatusTypeDef I2C1_IsDeviceReady(uint16_t DevAddress, uint32_t Trials) 00581 { 00582 return (HAL_I2C_IsDeviceReady(&heval_I2c1, DevAddress, Trials, I2c1Timeout)); 00583 } 00584 00585 /** 00586 * @brief Write a value in a register of the device through BUS. 00587 * @param Addr Device address on BUS Bus. 00588 * @param Reg The target register address to write 00589 * @param RegSize The target register size (can be 8BIT or 16BIT) 00590 * @param pBuffer The target register value to be written 00591 * @param Length buffer size to be written 00592 * @retval None 00593 */ 00594 static HAL_StatusTypeDef I2C1_WriteBuffer(uint16_t Addr, uint8_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length) 00595 { 00596 HAL_StatusTypeDef status = HAL_OK; 00597 00598 status = HAL_I2C_Mem_Write(&heval_I2c1, Addr, Reg, RegSize, pBuffer, Length, I2c1Timeout); 00599 00600 /* Check the communication status */ 00601 if(status != HAL_OK) 00602 { 00603 /* Re-Initiaize the BUS */ 00604 I2C1_Error(); 00605 } 00606 return status; 00607 } 00608 00609 /** 00610 * @brief Write buffer through I2C. 00611 * @param pBuffer The address of the data to be written 00612 * @param Length buffer size to be written 00613 * @retval None 00614 */ 00615 static HAL_StatusTypeDef I2C1_TransmitData(uint8_t *pBuffer, uint16_t Length) 00616 { 00617 HAL_StatusTypeDef status = HAL_OK; 00618 00619 status = HAL_I2C_Slave_Transmit(&heval_I2c1, pBuffer, Length, I2c1Timeout); 00620 00621 /* Check the communication status */ 00622 if(status != HAL_OK) 00623 { 00624 /* Execute user timeout callback */ 00625 I2C1_Error(); 00626 return HAL_ERROR; 00627 } 00628 return HAL_OK; 00629 } 00630 00631 /** 00632 * @brief Manages error callback by re-initializing I2C. 00633 * @retval None 00634 */ 00635 static void I2C1_Error(void) 00636 { 00637 /* De-initialize the I2C communication BUS */ 00638 HAL_I2C_DeInit(&heval_I2c1); 00639 00640 /* Re-Initiaize the I2C communication BUS */ 00641 I2C1_Init(); 00642 } 00643 00644 /** 00645 * @brief I2C MSP Initialization 00646 * @param hi2c I2C handle 00647 * @retval None 00648 */ 00649 static void I2C1_MspInit(I2C_HandleTypeDef *hi2c) 00650 { 00651 GPIO_InitTypeDef GPIO_InitStruct; 00652 RCC_PeriphCLKInitTypeDef RCC_PeriphCLKInitStruct; 00653 00654 /*##-1- Set source clock to SYSCLK for I2C1 ################################################*/ 00655 RCC_PeriphCLKInitStruct.PeriphClockSelection = RCC_PERIPHCLK_I2C1; 00656 RCC_PeriphCLKInitStruct.I2c1ClockSelection = RCC_I2C1CLKSOURCE_SYSCLK; 00657 HAL_RCCEx_PeriphCLKConfig(&RCC_PeriphCLKInitStruct); 00658 00659 /*##-2- Configure the GPIOs ################################################*/ 00660 00661 /* Enable GPIO clock */ 00662 EVAL_I2C1_GPIO_CLK_ENABLE(); 00663 00664 /* Configure I2C SCL & SDA as alternate function */ 00665 GPIO_InitStruct.Pin = (EVAL_I2C1_SCL_PIN| EVAL_I2C1_SDA_PIN); 00666 GPIO_InitStruct.Mode = GPIO_MODE_AF_OD; 00667 GPIO_InitStruct.Pull = GPIO_NOPULL; 00668 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_HIGH; 00669 GPIO_InitStruct.Alternate = EVAL_I2C1_SCL_SDA_AF; 00670 HAL_GPIO_Init(EVAL_I2C1_GPIO_PORT, &GPIO_InitStruct); 00671 00672 /*##-3- Configure the Eval I2C peripheral #######################################*/ 00673 /* Enable I2C clock */ 00674 EVAL_I2C1_CLK_ENABLE(); 00675 00676 /* Force the I2C peripheral clock reset */ 00677 EVAL_I2C1_FORCE_RESET(); 00678 00679 /* Release the I2C peripheral clock reset */ 00680 EVAL_I2C1_RELEASE_RESET(); 00681 } 00682 00683 /** 00684 * @brief I2C Bus initialization 00685 * @retval None 00686 */ 00687 static void I2C2_Init(void) 00688 { 00689 if(HAL_I2C_GetState(&heval_I2c2) == HAL_I2C_STATE_RESET) 00690 { 00691 heval_I2c2.Instance = EVAL_I2C2; 00692 heval_I2c2.Init.Timing = I2C2_TIMING; 00693 heval_I2c2.Init.OwnAddress1 = 0; 00694 heval_I2c2.Init.AddressingMode = I2C_ADDRESSINGMODE_7BIT; 00695 heval_I2c2.Init.DualAddressMode = I2C_DUALADDRESS_DISABLE; 00696 heval_I2c2.Init.OwnAddress2 = 0; 00697 heval_I2c2.Init.OwnAddress2Masks = I2C_OA2_NOMASK; 00698 heval_I2c2.Init.GeneralCallMode = I2C_GENERALCALL_DISABLE; 00699 heval_I2c2.Init.NoStretchMode = I2C_NOSTRETCH_DISABLE; 00700 00701 /* Init the I2C */ 00702 I2C2_MspInit(&heval_I2c2); 00703 HAL_I2C_Init(&heval_I2c2); 00704 } 00705 } 00706 00707 /** 00708 * @brief Read a register of the device through I2C. 00709 * @param Addr Device address on I2C Bus. 00710 * @param pBuffer The address to store the read data 00711 * @param Length buffer size to be read 00712 * @retval None 00713 */ 00714 static HAL_StatusTypeDef I2C2_ReceiveData(uint16_t Addr, uint8_t * pBuffer, uint16_t Length) 00715 { 00716 HAL_StatusTypeDef status = HAL_OK; 00717 00718 status = HAL_I2C_Master_Receive(&heval_I2c2, Addr, pBuffer, Length, I2c2Timeout); 00719 00720 /* Check the communication status */ 00721 if(status != HAL_OK) 00722 { 00723 /* Execute user timeout callback */ 00724 I2C2_Error(); 00725 } 00726 return status; 00727 } 00728 00729 /** 00730 * @brief Discovery I2C2 error treatment function 00731 * @retval None 00732 */ 00733 static void I2C2_Error(void) 00734 { 00735 /* De-initialize the I2C communication BUS */ 00736 HAL_I2C_DeInit(&heval_I2c2); 00737 00738 /* Re-Initiaize the I2C communication BUS */ 00739 I2C2_Init(); 00740 } 00741 00742 /** 00743 * @brief I2C MSP Initialization 00744 * @param hi2c I2C handle 00745 * @retval None 00746 */ 00747 static void I2C2_MspInit(I2C_HandleTypeDef *hi2c) 00748 { 00749 GPIO_InitTypeDef GPIO_InitStruct; 00750 /* Enable GPIO clock */ 00751 EVAL_I2C2_GPIO_CLK_ENABLE(); 00752 00753 /* Configure I2C SCL and SDA as alternate function */ 00754 GPIO_InitStruct.Pin = (EVAL_I2C2_SCL_PIN | EVAL_I2C2_SDA_PIN); 00755 GPIO_InitStruct.Mode = GPIO_MODE_AF_OD; 00756 GPIO_InitStruct.Pull = GPIO_NOPULL; 00757 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_HIGH; 00758 GPIO_InitStruct.Alternate = EVAL_I2C2_AF; 00759 HAL_GPIO_Init(EVAL_I2C2_GPIO_PORT, &GPIO_InitStruct); 00760 00761 /* Enable I2C clock */ 00762 EVAL_I2C2_CLK_ENABLE(); 00763 00764 /* Force the I2C peripheral clock reset */ 00765 EVAL_I2C2_FORCE_RESET(); 00766 00767 /* Release the I2C peripheral clock reset */ 00768 EVAL_I2C2_RELEASE_RESET(); 00769 } 00770 #endif /*HAL_I2C_MODULE_ENABLED*/ 00771 00772 #if defined(HAL_SPI_MODULE_ENABLED) 00773 /******************************* SPI Routines *********************************/ 00774 00775 /** 00776 * @brief SPIx Bus initialization 00777 * @retval None 00778 */ 00779 static void SPIx_Init(void) 00780 { 00781 if(HAL_SPI_GetState(&heval_Spi) == HAL_SPI_STATE_RESET) 00782 { 00783 /* SPI Config */ 00784 heval_Spi.Instance = EVAL_SPIx; 00785 /* SPI baudrate is set to 12 MHz (PCLK1/SPI_BaudRatePrescaler = 48/4 = 12 MHz) 00786 to verify these constraints: 00787 HX8347D LCD SPI interface max baudrate is 50MHz for write and 6.66MHz for read 00788 PCLK1 frequency is set to 48 MHz 00789 - SD card SPI interface max baudrate is 25MHz for write/read 00790 */ 00791 heval_Spi.Init.BaudRatePrescaler = SPI_BAUDRATEPRESCALER_4; 00792 heval_Spi.Init.Direction = SPI_DIRECTION_2LINES; 00793 heval_Spi.Init.CLKPhase = SPI_PHASE_2EDGE; 00794 heval_Spi.Init.CLKPolarity = SPI_POLARITY_HIGH; 00795 heval_Spi.Init.CRCCalculation = SPI_CRCCALCULATION_DISABLE; 00796 heval_Spi.Init.CRCPolynomial = 7; 00797 heval_Spi.Init.DataSize = SPI_DATASIZE_8BIT; 00798 heval_Spi.Init.FirstBit = SPI_FIRSTBIT_MSB; 00799 heval_Spi.Init.NSS = SPI_NSS_SOFT; 00800 heval_Spi.Init.TIMode = SPI_TIMODE_DISABLE; 00801 heval_Spi.Init.Mode = SPI_MODE_MASTER; 00802 00803 SPIx_MspInit(&heval_Spi); 00804 HAL_SPI_Init(&heval_Spi); 00805 } 00806 } 00807 00808 /** 00809 * @brief SPI Read 4 bytes from device 00810 * @retval Read data 00811 */ 00812 static uint32_t SPIx_Read(void) 00813 { 00814 HAL_StatusTypeDef status = HAL_OK; 00815 uint32_t readvalue = 0x0; 00816 uint32_t writevalue = 0xFFFFFFFF; 00817 00818 status = HAL_SPI_TransmitReceive(&heval_Spi, (uint8_t*) &writevalue, (uint8_t*) &readvalue, 1, SpixTimeout); 00819 00820 /* Check the communication status */ 00821 if(status != HAL_OK) 00822 { 00823 /* Execute user timeout callback */ 00824 SPIx_Error(); 00825 } 00826 00827 return readvalue; 00828 } 00829 00830 /** 00831 * @brief SPI Write a byte to device 00832 * @param DataIn value to be written 00833 * @param DataOut read value 00834 * @param DataLegnth data length 00835 * @retval None 00836 */ 00837 static void SPIx_WriteReadData(const uint8_t *DataIn, uint8_t *DataOut, uint16_t DataLegnth) 00838 { 00839 HAL_StatusTypeDef status = HAL_OK; 00840 00841 status = HAL_SPI_TransmitReceive(&heval_Spi, (uint8_t*) DataIn, DataOut, DataLegnth, SpixTimeout); 00842 00843 /* Check the communication status */ 00844 if(status != HAL_OK) 00845 { 00846 /* Execute user timeout callback */ 00847 SPIx_Error(); 00848 } 00849 } 00850 00851 /** 00852 * @brief SPI Write a byte to device 00853 * @param Value value to be written 00854 * @retval None 00855 */ 00856 static void SPIx_Write(uint8_t Value) 00857 { 00858 HAL_StatusTypeDef status = HAL_OK; 00859 uint8_t data; 00860 00861 status = HAL_SPI_TransmitReceive(&heval_Spi, (uint8_t*) &Value, &data, 1, SpixTimeout); 00862 00863 /* Check the communication status */ 00864 if(status != HAL_OK) 00865 { 00866 /* Execute user timeout callback */ 00867 SPIx_Error(); 00868 } 00869 } 00870 00871 /** 00872 * @brief SPIx_FlushFifo 00873 * @retval None 00874 */ 00875 static void SPIx_FlushFifo(void) 00876 { 00877 HAL_SPIEx_FlushRxFifo(&heval_Spi); 00878 } 00879 00880 /** 00881 * @brief SPI error treatment function 00882 * @retval None 00883 */ 00884 static void SPIx_Error (void) 00885 { 00886 /* De-initialize the SPI communication BUS */ 00887 HAL_SPI_DeInit(&heval_Spi); 00888 00889 /* Re- Initiaize the SPI communication BUS */ 00890 SPIx_Init(); 00891 } 00892 00893 /** 00894 * @brief SPI MSP Init 00895 * @param hspi SPI handle 00896 * @retval None 00897 */ 00898 static void SPIx_MspInit(SPI_HandleTypeDef *hspi) 00899 { 00900 GPIO_InitTypeDef GPIO_InitStruct; 00901 00902 /* Enable SPI clock */ 00903 EVAL_SPIx_CLK_ENABLE(); 00904 00905 /* enable EVAL_SPI gpio clocks */ 00906 EVAL_SPIx_SCK_GPIO_CLK_ENABLE(); 00907 EVAL_SPIx_MISO_GPIO_CLK_ENABLE(); 00908 EVAL_SPIx_MOSI_GPIO_CLK_ENABLE(); 00909 EVAL_SPIx_MOSI_DIR_GPIO_CLK_ENABLE(); 00910 00911 /* configure SPI SCK */ 00912 GPIO_InitStruct.Pin = EVAL_SPIx_SCK_PIN; 00913 GPIO_InitStruct.Mode = GPIO_MODE_AF_PP; 00914 GPIO_InitStruct.Pull = GPIO_NOPULL; 00915 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_HIGH; 00916 GPIO_InitStruct.Alternate = EVAL_SPIx_SCK_AF; 00917 HAL_GPIO_Init(EVAL_SPIx_SCK_GPIO_PORT, &GPIO_InitStruct); 00918 00919 /* configure SPI MOSI */ 00920 GPIO_InitStruct.Pin = EVAL_SPIx_MOSI_PIN; 00921 GPIO_InitStruct.Alternate = EVAL_SPIx_MOSI_AF; 00922 HAL_GPIO_Init(EVAL_SPIx_MOSI_GPIO_PORT, &GPIO_InitStruct); 00923 00924 /* configure SPI MISO */ 00925 GPIO_InitStruct.Pin = EVAL_SPIx_MISO_PIN; 00926 GPIO_InitStruct.Alternate = EVAL_SPIx_MISO_AF; 00927 HAL_GPIO_Init(EVAL_SPIx_MISO_GPIO_PORT, &GPIO_InitStruct); 00928 00929 /* Set PB.2 as Out PP, as direction pin for MOSI */ 00930 GPIO_InitStruct.Pin = EVAL_SPIx_MOSI_DIR_PIN; 00931 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_MEDIUM; 00932 GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP; 00933 GPIO_InitStruct.Pull = GPIO_NOPULL; 00934 HAL_GPIO_Init(EVAL_SPIx_MOSI_DIR_GPIO_PORT, &GPIO_InitStruct); 00935 00936 /* MOSI DIRECTION as output */ 00937 HAL_GPIO_WritePin(EVAL_SPIx_MOSI_DIR_GPIO_PORT, EVAL_SPIx_MOSI_DIR_PIN, GPIO_PIN_SET); 00938 00939 /* Force the SPI peripheral clock reset */ 00940 EVAL_SPIx_FORCE_RESET(); 00941 00942 /* Release the SPI peripheral clock reset */ 00943 EVAL_SPIx_RELEASE_RESET(); 00944 } 00945 00946 #endif /*HAL_SPI_MODULE_ENABLED*/ 00947 00948 /** 00949 * @} 00950 */ 00951 00952 /** @addtogroup STM32072B_EVAL_LINK_Operations_Functions 00953 * @{ 00954 */ 00955 00956 /****************************************************************************** 00957 LINK OPERATIONS 00958 *******************************************************************************/ 00959 00960 #if defined(HAL_SPI_MODULE_ENABLED) 00961 /********************************* LINK LCD ***********************************/ 00962 00963 /** 00964 * @brief Configures the LCD_SPI interface. 00965 * @retval None 00966 */ 00967 void LCD_IO_Init(void) 00968 { 00969 GPIO_InitTypeDef GPIO_InitStruct; 00970 00971 /* Configure the LCD Control pins ------------------------------------------*/ 00972 LCD_NCS_GPIO_CLK_ENABLE(); 00973 00974 /* Configure NCS in Output Push-Pull mode */ 00975 GPIO_InitStruct.Pin = LCD_NCS_PIN; 00976 GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_OD; 00977 GPIO_InitStruct.Pull = GPIO_NOPULL; 00978 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_LOW; 00979 HAL_GPIO_Init(LCD_NCS_GPIO_PORT, &GPIO_InitStruct); 00980 00981 /* Set or Reset the control line */ 00982 LCD_CS_LOW(); 00983 LCD_CS_HIGH(); 00984 00985 SPIx_Init(); 00986 } 00987 00988 /** 00989 * @brief Write register value. 00990 * @param pData Pointer on the register value 00991 * @param Size Size of byte to transmit to the register 00992 * @retval None 00993 */ 00994 void LCD_IO_WriteMultipleData(uint8_t *pData, uint32_t Size) 00995 { 00996 uint32_t counter = 0; 00997 00998 /* Reset LCD control line(/CS) and Send data */ 00999 LCD_CS_LOW(); 01000 01001 /* Send Start Byte */ 01002 SPIx_Write(START_BYTE | LCD_WRITE_REG); 01003 01004 if (Size == 1) 01005 { 01006 /* Only 1 byte to be sent to LCD - general interface can be used */ 01007 /* Send Data */ 01008 SPIx_Write(*pData); 01009 } 01010 else 01011 { 01012 for (counter = Size; counter != 0; counter--) 01013 { 01014 while(((heval_Spi.Instance->SR) & SPI_FLAG_TXE) != SPI_FLAG_TXE) 01015 { 01016 } 01017 /* Need to invert bytes for LCD*/ 01018 *((__IO uint8_t*)&heval_Spi.Instance->DR) = *(pData+1); 01019 01020 while(((heval_Spi.Instance->SR) & SPI_FLAG_TXE) != SPI_FLAG_TXE) 01021 { 01022 } 01023 *((__IO uint8_t*)&heval_Spi.Instance->DR) = *pData; 01024 counter--; 01025 pData += 2; 01026 } 01027 01028 /* Wait until the bus is ready before releasing Chip select */ 01029 while(((heval_Spi.Instance->SR) & SPI_FLAG_BSY) != RESET) 01030 { 01031 } 01032 } 01033 01034 /* Empty the Rx fifo */ 01035 SPIx_FlushFifo(); 01036 01037 /* Reset LCD control line(/CS) and Send data */ 01038 LCD_CS_HIGH(); 01039 } 01040 01041 /** 01042 * @brief Writes address on LCD register. 01043 * @param Reg Register to be written 01044 * @retval None 01045 */ 01046 void LCD_IO_WriteReg(uint8_t Reg) 01047 { 01048 /* Reset LCD control line(/CS) and Send command */ 01049 LCD_CS_LOW(); 01050 01051 /* Send Start Byte */ 01052 SPIx_Write(START_BYTE | SET_INDEX); 01053 01054 /* Write 16-bit Reg Index (High Byte is 0) */ 01055 SPIx_Write(0x00); 01056 SPIx_Write(Reg); 01057 01058 /* Deselect : Chip Select high */ 01059 LCD_CS_HIGH(); 01060 } 01061 01062 /** 01063 * @brief Read data from LCD data register. 01064 * @param Reg Regsiter to be read 01065 * @retval readvalue 01066 */ 01067 uint16_t LCD_IO_ReadData(uint16_t Reg) 01068 { 01069 uint32_t readvalue = 0; 01070 01071 /* Send Reg value to Read */ 01072 LCD_IO_WriteReg(Reg); 01073 01074 /* Reset LCD control line(/CS) and Send command */ 01075 LCD_CS_LOW(); 01076 01077 /* Send Start Byte */ 01078 SPIx_Write(START_BYTE | LCD_READ_REG); 01079 01080 /* Read Upper Byte */ 01081 SPIx_Write(0xFF); 01082 readvalue = SPIx_Read(); 01083 readvalue = readvalue << 8; 01084 readvalue |= SPIx_Read(); 01085 01086 HAL_Delay(10); 01087 01088 /* Deselect : Chip Select high */ 01089 LCD_CS_HIGH(); 01090 return readvalue; 01091 } 01092 01093 /** 01094 * @brief Wait for loop in ms. 01095 * @param Delay in ms. 01096 */ 01097 void LCD_Delay (uint32_t Delay) 01098 { 01099 HAL_Delay(Delay); 01100 } 01101 01102 /******************************** LINK SD Card ********************************/ 01103 01104 /** 01105 * @brief Initializes the SD Card and put it into StandBy State (Ready for 01106 * data transfer). 01107 * @retval None 01108 */ 01109 void SD_IO_Init(void) 01110 { 01111 GPIO_InitTypeDef GPIO_InitStruct; 01112 uint8_t counter; 01113 01114 /* SD_CS_GPIO and SD_DETECT_GPIO Periph clock enable */ 01115 SD_CS_GPIO_CLK_ENABLE(); 01116 SD_DETECT_GPIO_CLK_ENABLE(); 01117 01118 /* Configure SD_CS_PIN pin: SD Card CS pin */ 01119 GPIO_InitStruct.Pin = SD_CS_PIN; 01120 GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_OD; 01121 GPIO_InitStruct.Pull = GPIO_PULLUP; 01122 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_MEDIUM; 01123 HAL_GPIO_Init(SD_CS_GPIO_PORT, &GPIO_InitStruct); 01124 01125 /* Configure SD_DETECT_PIN pin: SD Card detect pin */ 01126 GPIO_InitStruct.Pin = SD_DETECT_PIN; 01127 GPIO_InitStruct.Mode = GPIO_MODE_IT_RISING_FALLING; 01128 GPIO_InitStruct.Pull = GPIO_PULLUP; 01129 HAL_GPIO_Init(SD_DETECT_GPIO_PORT, &GPIO_InitStruct); 01130 01131 /* Configure LCD_CS_PIN pin: LCD Card CS pin */ 01132 GPIO_InitStruct.Pin = LCD_NCS_PIN; 01133 GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP; 01134 GPIO_InitStruct.Pull = GPIO_NOPULL; 01135 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_MEDIUM; 01136 HAL_GPIO_Init(LCD_NCS_GPIO_PORT, &GPIO_InitStruct); 01137 LCD_CS_HIGH(); 01138 01139 /* Enable and set SD EXTI Interrupt to the lowest priority */ 01140 HAL_NVIC_SetPriority(SD_DETECT_EXTI_IRQn, 0x03, 0); 01141 HAL_NVIC_EnableIRQ(SD_DETECT_EXTI_IRQn); 01142 01143 /*------------Put SD in SPI mode--------------*/ 01144 /* SD SPI Config */ 01145 SPIx_Init(); 01146 01147 /* SD chip select high */ 01148 SD_CS_HIGH(); 01149 01150 /* Send dummy byte 0xFF, 10 times with CS high */ 01151 /* Rise CS and MOSI for 80 clocks cycles */ 01152 for (counter = 0; counter <= 9; counter++) 01153 { 01154 /* Send dummy byte 0xFF */ 01155 SD_IO_WriteByte(SD_DUMMY_BYTE); 01156 } 01157 } 01158 01159 01160 void SD_IO_CSState(uint8_t val) 01161 { 01162 if(val == 1) 01163 { 01164 SD_CS_HIGH(); 01165 } 01166 else 01167 { 01168 SD_CS_LOW(); 01169 } 01170 } 01171 01172 /** 01173 * @brief Write a byte on the SD. 01174 * @param DataIn byte to send. 01175 * @param DataOut read byte. 01176 * @param DataLength data length. 01177 * @retval None 01178 */ 01179 void SD_IO_WriteReadData(const uint8_t *DataIn, uint8_t *DataOut, uint16_t DataLength) 01180 { 01181 /* Send the byte */ 01182 SPIx_WriteReadData(DataIn, DataOut, DataLength); 01183 } 01184 01185 /** 01186 * @brief Writes a byte on the SD. 01187 * @param Data byte to send. 01188 * @retval None 01189 */ 01190 uint8_t SD_IO_WriteByte(uint8_t Data) 01191 { 01192 uint8_t tmp; 01193 01194 /* Send the byte */ 01195 SPIx_WriteReadData(&Data,&tmp,1); 01196 return tmp; 01197 } 01198 01199 #endif /* HAL_SPI_MODULE_ENABLED */ 01200 01201 #if defined(HAL_I2C_MODULE_ENABLED) 01202 /********************************* LINK I2C EEPROM *****************************/ 01203 /** 01204 * @brief Initializes peripherals used by the I2C EEPROM driver. 01205 * @retval None 01206 */ 01207 void EEPROM_IO_Init(void) 01208 { 01209 I2C1_Init(); 01210 } 01211 01212 /** 01213 * @brief Write data to I2C EEPROM driver 01214 * @param DevAddress Target device address 01215 * @param MemAddress Internal memory address 01216 * @param pBuffer Pointer to data buffer 01217 * @param BufferSize Amount of data to be sent 01218 * @retval HAL status 01219 */ 01220 HAL_StatusTypeDef EEPROM_IO_WriteData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize) 01221 { 01222 return (I2C1_WriteBuffer(DevAddress, MemAddress, I2C_MEMADD_SIZE_16BIT, pBuffer, BufferSize)); 01223 } 01224 01225 /** 01226 * @brief Read data from I2C EEPROM driver 01227 * @param DevAddress Target device address 01228 * @param MemAddress Internal memory address 01229 * @param pBuffer Pointer to data buffer 01230 * @param BufferSize Amount of data to be read 01231 * @retval HAL status 01232 */ 01233 HAL_StatusTypeDef EEPROM_IO_ReadData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize) 01234 { 01235 return (I2C1_ReadBuffer(DevAddress, MemAddress, I2C_MEMADD_SIZE_16BIT, pBuffer, BufferSize)); 01236 } 01237 01238 /** 01239 * @brief Checks if target device is ready for communication. 01240 * @note This function is used with Memory devices 01241 * @param DevAddress Target device address 01242 * @param Trials Number of trials 01243 * @retval HAL status 01244 */ 01245 HAL_StatusTypeDef EEPROM_IO_IsDeviceReady(uint16_t DevAddress, uint32_t Trials) 01246 { 01247 return (I2C1_IsDeviceReady(DevAddress, Trials)); 01248 } 01249 01250 /********************************* LINK I2C TEMPERATURE SENSOR *****************************/ 01251 /** 01252 * @brief Initializes peripherals used by the I2C Temperature Sensor driver. 01253 * @retval None 01254 */ 01255 void TSENSOR_IO_Init(void) 01256 { 01257 I2C1_Init(); 01258 } 01259 01260 /** 01261 * @brief Writes one byte to the TSENSOR. 01262 * @param DevAddress Target device address 01263 * @param pBuffer Pointer to data buffer 01264 * @param WriteAddr TSENSOR's internal address to write to. 01265 * @param Length Number of data to write 01266 * @retval None 01267 */ 01268 void TSENSOR_IO_Write(uint16_t DevAddress, uint8_t* pBuffer, uint8_t WriteAddr, uint16_t Length) 01269 { 01270 I2C1_WriteBuffer(DevAddress, WriteAddr, I2C_MEMADD_SIZE_8BIT, pBuffer, Length); 01271 } 01272 01273 /** 01274 * @brief Reads one byte from the TSENSOR. 01275 * @param DevAddress Target device address 01276 * @param pBuffer pointer to the buffer that receives the data read from the TSENSOR. 01277 * @param ReadAddr TSENSOR's internal address to read from. 01278 * @param Length Number of data to read 01279 * @retval None 01280 */ 01281 void TSENSOR_IO_Read(uint16_t DevAddress, uint8_t* pBuffer, uint8_t ReadAddr, uint16_t Length) 01282 { 01283 I2C1_ReadBuffer(DevAddress, ReadAddr, I2C_MEMADD_SIZE_8BIT, pBuffer, Length); 01284 } 01285 01286 /** 01287 * @brief Checks if Temperature Sensor is ready for communication. 01288 * @param DevAddress Target device address 01289 * @param Trials Number of trials 01290 * @retval HAL status 01291 */ 01292 uint16_t TSENSOR_IO_IsDeviceReady(uint16_t DevAddress, uint32_t Trials) 01293 { 01294 return (I2C1_IsDeviceReady(DevAddress, Trials)); 01295 } 01296 01297 /****************************** LINK HDMI CEC *********************************/ 01298 /** 01299 * @brief Initializes CEC low level. 01300 * @retval None 01301 */ 01302 void HDMI_CEC_IO_Init (void) 01303 { 01304 GPIO_InitTypeDef GPIO_InitStruct; 01305 01306 /* Enable CEC clock */ 01307 __HAL_RCC_CEC_CLK_ENABLE(); 01308 01309 /* Enable CEC LINE GPIO clock */ 01310 HDMI_CEC_LINE_CLK_ENABLE(); 01311 01312 /* Configure CEC LINE GPIO as alternate function open drain */ 01313 GPIO_InitStruct.Pin = HDMI_CEC_LINE_PIN; 01314 GPIO_InitStruct.Mode = GPIO_MODE_AF_OD; 01315 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_HIGH; 01316 GPIO_InitStruct.Pull = GPIO_NOPULL; 01317 GPIO_InitStruct.Alternate = HDMI_CEC_LINE_AF; 01318 HAL_GPIO_Init(HDMI_CEC_LINE_GPIO_PORT, &GPIO_InitStruct); 01319 01320 /* CEC IRQ Channel configuration */ 01321 HAL_NVIC_SetPriority((IRQn_Type)HDMI_CEC_IRQn, 0x3, 0x0); 01322 HAL_NVIC_EnableIRQ((IRQn_Type)HDMI_CEC_IRQn); 01323 01324 /* Enable CEC HPD SINK GPIO clock */ 01325 HDMI_CEC_HPD_SINK_CLK_ENABLE(); 01326 01327 /* Configure CEC HPD SINK GPIO as output push pull */ 01328 GPIO_InitStruct.Pin = HDMI_CEC_HPD_SINK_PIN; 01329 GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP; 01330 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_LOW; 01331 GPIO_InitStruct.Pull = GPIO_PULLDOWN; 01332 HAL_GPIO_Init(HDMI_CEC_HPD_SINK_GPIO_PORT, &GPIO_InitStruct); 01333 01334 I2C1_Init(); 01335 01336 /* Enable CEC HPD SOURCE GPIO clock */ 01337 HDMI_CEC_HPD_SOURCE_CLK_ENABLE(); 01338 01339 /* Configure CEC HPD SOURCE GPIO as output push pull */ 01340 GPIO_InitStruct.Pin = HDMI_CEC_HPD_SOURCE_PIN; 01341 GPIO_InitStruct.Mode = GPIO_MODE_INPUT; 01342 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_LOW; 01343 GPIO_InitStruct.Pull = GPIO_PULLDOWN; 01344 HAL_GPIO_Init(HDMI_CEC_HPD_SOURCE_GPIO_PORT, &GPIO_InitStruct); 01345 01346 I2C2_Init(); 01347 } 01348 01349 /** 01350 * @brief Write data to I2C HDMI CEC driver 01351 * @param pBuffer Pointer to data buffer 01352 * @param BufferSize Amount of data to be sent 01353 * @retval HAL status 01354 */ 01355 HAL_StatusTypeDef HDMI_CEC_IO_WriteData(uint8_t * pBuffer, uint16_t BufferSize) 01356 { 01357 return (I2C1_TransmitData(pBuffer, BufferSize)); 01358 } 01359 01360 /** 01361 * @brief Read data to I2C HDMI CEC driver 01362 * @param DevAddress Target device address 01363 * @param pBuffer Pointer to data buffer 01364 * @param BufferSize Amount of data to be sent 01365 * @retval HAL status 01366 */ 01367 HAL_StatusTypeDef HDMI_CEC_IO_ReadData(uint16_t DevAddress, uint8_t * pBuffer, uint16_t BufferSize) 01368 { 01369 return (I2C2_ReceiveData(DevAddress, pBuffer, BufferSize)); 01370 } 01371 01372 #endif /* HAL_I2C_MODULE_ENABLED */ 01373 01374 /** 01375 * @} 01376 */ 01377 01378 /** 01379 * @} 01380 */ 01381 01382 /** 01383 * @} 01384 */ 01385 01386 /** 01387 * @} 01388 */ 01389 01390 /** 01391 * @} 01392 */ 01393 01394 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Wed Jul 5 2017 08:56:10 for STM32072B_EVAL BSP User Manual by
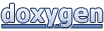