qwt_plot_curve.h
00001 /* -*- mode: C++ ; c-file-style: "stroustrup" -*- ***************************** 00002 * Qwt Widget Library 00003 * Copyright (C) 1997 Josef Wilgen 00004 * Copyright (C) 2002 Uwe Rathmann 00005 * 00006 * This library is free software; you can redistribute it and/or 00007 * modify it under the terms of the Qwt License, Version 1.0 00008 *****************************************************************************/ 00009 00010 #ifndef QWT_PLOT_CURVE_H 00011 #define QWT_PLOT_CURVE_H 00012 00013 #include <qpen.h> 00014 #include <qstring.h> 00015 #include "qwt_global.h" 00016 #include "qwt_plot_item.h" 00017 #include "qwt_text.h" 00018 #include "qwt_polygon.h" 00019 #include "qwt_data.h" 00020 00021 class QPainter; 00022 class QwtScaleMap; 00023 class QwtSymbol; 00024 class QwtCurveFitter; 00025 00054 class QWT_EXPORT QwtPlotCurve: public QwtPlotItem 00055 { 00056 public: 00073 enum CurveType 00074 { 00075 Yfx, 00076 Xfy 00077 }; 00078 00106 enum CurveStyle 00107 { 00108 NoCurve, 00109 00110 Lines, 00111 Sticks, 00112 Steps, 00113 Dots, 00114 00115 UserCurve = 100 00116 }; 00117 00134 enum CurveAttribute 00135 { 00136 Inverted = 1, 00137 Fitted = 2 00138 }; 00139 00158 enum PaintAttribute 00159 { 00160 PaintFiltered = 1, 00161 ClipPolygons = 2 00162 }; 00163 00164 explicit QwtPlotCurve(); 00165 explicit QwtPlotCurve(const QwtText &title); 00166 explicit QwtPlotCurve(const QString &title); 00167 00168 virtual ~QwtPlotCurve(); 00169 00170 virtual int rtti() const; 00171 00172 void setCurveType(CurveType); 00173 CurveType curveType() const; 00174 00175 void setPaintAttribute(PaintAttribute, bool on = true); 00176 bool testPaintAttribute(PaintAttribute) const; 00177 00178 void setRawData(const double *x, const double *y, int size); 00179 void setData(const double *xData, const double *yData, int size); 00180 void setData(const QwtArray<double> &xData, const QwtArray<double> &yData); 00181 #if QT_VERSION < 0x040000 00182 void setData(const QwtArray<QwtDoublePoint> &data); 00183 #else 00184 void setData(const QPolygonF &data); 00185 #endif 00186 void setData(const QwtData &data); 00187 00188 int closestPoint(const QPoint &pos, double *dist = NULL) const; 00189 00190 QwtData &data(); 00191 const QwtData &data() const; 00192 00193 int dataSize() const; 00194 inline double x(int i) const; 00195 inline double y(int i) const; 00196 00197 virtual QwtDoubleRect boundingRect() const; 00198 00200 inline double minXValue() const { return boundingRect().left(); } 00202 inline double maxXValue() const { return boundingRect().right(); } 00204 inline double minYValue() const { return boundingRect().top(); } 00206 inline double maxYValue() const { return boundingRect().bottom(); } 00207 00208 void setCurveAttribute(CurveAttribute, bool on = true); 00209 bool testCurveAttribute(CurveAttribute) const; 00210 00211 void setPen(const QPen &); 00212 const QPen &pen() const; 00213 00214 void setBrush(const QBrush &); 00215 const QBrush &brush() const; 00216 00217 void setBaseline(double ref); 00218 double baseline() const; 00219 00220 void setStyle(CurveStyle style); 00221 CurveStyle style() const; 00222 00223 void setSymbol(const QwtSymbol &s); 00224 const QwtSymbol& symbol() const; 00225 00226 void setCurveFitter(QwtCurveFitter *); 00227 QwtCurveFitter *curveFitter() const; 00228 00229 virtual void draw(QPainter *p, 00230 const QwtScaleMap &xMap, const QwtScaleMap &yMap, 00231 const QRect &) const; 00232 00233 virtual void draw(QPainter *p, 00234 const QwtScaleMap &xMap, const QwtScaleMap &yMap, 00235 int from, int to) const; 00236 00237 void draw(int from, int to) const; 00238 00239 virtual void updateLegend(QwtLegend *) const; 00240 00241 protected: 00242 00243 void init(); 00244 00245 virtual void drawCurve(QPainter *p, int style, 00246 const QwtScaleMap &xMap, const QwtScaleMap &yMap, 00247 int from, int to) const; 00248 00249 virtual void drawSymbols(QPainter *p, const QwtSymbol &, 00250 const QwtScaleMap &xMap, const QwtScaleMap &yMap, 00251 int from, int to) const; 00252 00253 void drawLines(QPainter *p, 00254 const QwtScaleMap &xMap, const QwtScaleMap &yMap, 00255 int from, int to) const; 00256 void drawSticks(QPainter *p, 00257 const QwtScaleMap &xMap, const QwtScaleMap &yMap, 00258 int from, int to) const; 00259 void drawDots(QPainter *p, 00260 const QwtScaleMap &xMap, const QwtScaleMap &yMap, 00261 int from, int to) const; 00262 void drawSteps(QPainter *p, 00263 const QwtScaleMap &xMap, const QwtScaleMap &yMap, 00264 int from, int to) const; 00265 00266 void fillCurve(QPainter *, 00267 const QwtScaleMap &, const QwtScaleMap &, 00268 QwtPolygon &) const; 00269 void closePolyline(const QwtScaleMap &, const QwtScaleMap &, 00270 QwtPolygon &) const; 00271 00272 private: 00273 QwtData *d_xy; 00274 00275 class PrivateData; 00276 PrivateData *d_data; 00277 }; 00278 00280 inline QwtData &QwtPlotCurve::data() 00281 { 00282 return *d_xy; 00283 } 00284 00286 inline const QwtData &QwtPlotCurve::data() const 00287 { 00288 return *d_xy; 00289 } 00290 00295 inline double QwtPlotCurve::x(int i) const 00296 { 00297 return d_xy->x(i); 00298 } 00299 00304 inline double QwtPlotCurve::y(int i) const 00305 { 00306 return d_xy->y(i); 00307 } 00308 00309 #endif
Generated on Wed Sep 2 18:37:21 2009 for Qwt User's Guide by
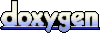