QwtPlotRescaler Class Reference
QwtPlotRescaler takes care of fixed aspect ratios for plot scales. More...
#include <qwt_plot_rescaler.h>
Public Types | |
enum | RescalePolicy { Fixed, Expanding, Fitting } |
enum | ExpandingDirection { ExpandUp, ExpandDown, ExpandBoth } |
Public Member Functions | |
QwtPlotRescaler (QwtPlotCanvas *, int referenceAxis=QwtPlot::xBottom, RescalePolicy=Expanding) | |
virtual | ~QwtPlotRescaler () |
void | setEnabled (bool) |
bool | isEnabled () const |
void | setRescalePolicy (RescalePolicy) |
RescalePolicy | rescalePolicy () const |
void | setExpandingDirection (ExpandingDirection) |
void | setExpandingDirection (int axis, ExpandingDirection) |
ExpandingDirection | expandingDirection (int axis) const |
void | setReferenceAxis (int axis) |
int | referenceAxis () const |
void | setAspectRatio (double ratio) |
void | setAspectRatio (int axis, double ratio) |
double | aspectRatio (int axis) const |
void | setIntervalHint (int axis, const QwtDoubleInterval &) |
QwtDoubleInterval | intervalHint (int axis) const |
QwtPlotCanvas * | canvas () |
const QwtPlotCanvas * | canvas () const |
QwtPlot * | plot () |
const QwtPlot * | plot () const |
virtual bool | eventFilter (QObject *, QEvent *) |
void | rescale () const |
Protected Member Functions | |
virtual void | canvasResizeEvent (QResizeEvent *) |
virtual void | rescale (const QSize &oldSize, const QSize &newSize) const |
virtual QwtDoubleInterval | expandScale (int axis, const QSize &oldSize, const QSize &newSize) const |
virtual QwtDoubleInterval | syncScale (int axis, const QwtDoubleInterval &reference, const QSize &size) const |
virtual void | updateScales (QwtDoubleInterval intervals[QwtPlot::axisCnt]) const |
Qt::Orientation | orientation (int axis) const |
QwtDoubleInterval | interval (int axis) const |
QwtDoubleInterval | expandInterval (const QwtDoubleInterval &, double width, ExpandingDirection) const |
Detailed Description
QwtPlotRescaler takes care of fixed aspect ratios for plot scales.
QwtPlotRescaler autoadjusts the axes of a QwtPlot according to fixed aspect ratios.
Member Enumeration Documentation
Rescale Policy.
The rescale policy defines how to rescale the reference axis and their depending axes.
- Fixed
The interval of the reference axis remains unchanged, when the geometry of the canvas changes. All other axes will be adjusted according to their aspect ratio.
- Expanding
The interval of the reference axis will be shrinked/expanded, when the geometry of the canvas changes. All other axes will be adjusted according to their aspect ratio.
The interval, that is represented by one pixel is fixed.
- Fitting
The intervals of the axes are calculated, so that all axes include their minimal interval.
Constructor & Destructor Documentation
QwtPlotRescaler::QwtPlotRescaler | ( | QwtPlotCanvas * | canvas, | |
int | referenceAxis = QwtPlot::xBottom , |
|||
RescalePolicy | policy = Expanding | |||
) | [explicit] |
Constructor
- Parameters:
-
canvas Canvas referenceAxis Reference axis, see RescalePolicy policy Rescale policy
- See also:
- setRescalePolicy(), setReferenceAxis()
QwtPlotRescaler::~QwtPlotRescaler | ( | ) | [virtual] |
Destructor.
Member Function Documentation
double QwtPlotRescaler::aspectRatio | ( | int | axis | ) | const |
Return aspect ratio between an axis and the reference axis.
- Parameters:
-
axis Axis index ( see QwtPlot::AxisId )
- See also:
- setAspectRatio()
const QwtPlotCanvas * QwtPlotRescaler::canvas | ( | ) | const |
- Returns:
- plot canvas
QwtPlotCanvas * QwtPlotRescaler::canvas | ( | ) |
- Returns:
- plot canvas
bool QwtPlotRescaler::eventFilter | ( | QObject * | o, | |
QEvent * | e | |||
) | [virtual] |
Event filter for the plot canvas.
QwtPlotRescaler::ExpandingDirection QwtPlotRescaler::expandingDirection | ( | int | axis | ) | const |
Return direction in which an axis should be expanded
- Parameters:
-
axis Axis index ( see QwtPlot::AxisId )
- See also:
- setExpandingDirection()
QwtDoubleInterval QwtPlotRescaler::expandInterval | ( | const QwtDoubleInterval & | interval, | |
double | width, | |||
ExpandingDirection | direction | |||
) | const [protected] |
Expand the interval
- Parameters:
-
interval Interval to be expanded width Distance to be added to the interval direction Direction of the expand operation
- Returns:
- Expanded interval
QwtDoubleInterval QwtPlotRescaler::expandScale | ( | int | axis, | |
const QSize & | oldSize, | |||
const QSize & | newSize | |||
) | const [protected, virtual] |
Calculate the new scale interval of a plot axis
- Parameters:
-
axis Axis index ( see QwtPlot::AxisId ) oldSize Previous size of the canvas newSize New size of the canvas
- Returns:
- Calculated new interval for the axis
QwtDoubleInterval QwtPlotRescaler::interval | ( | int | axis | ) | const [protected] |
Return interval of an axis
- Parameters:
-
axis Axis index ( see QwtPlot::AxisId )
bool QwtPlotRescaler::isEnabled | ( | ) | const |
- Returns:
- true when enabled, false otherwise
- See also:
- setEnabled, eventFilter()
Qt::Orientation QwtPlotRescaler::orientation | ( | int | axis | ) | const [protected] |
Return orientation of an axis
- Parameters:
-
axis Axis index ( see QwtPlot::AxisId )
const QwtPlot * QwtPlotRescaler::plot | ( | ) | const |
- Returns:
- plot widget
QwtPlot * QwtPlotRescaler::plot | ( | ) |
- Returns:
- plot widget
int QwtPlotRescaler::referenceAxis | ( | ) | const |
- Returns:
- Reference axis ( see RescalePolicy )
- See also:
- setReferenceAxis()
void QwtPlotRescaler::rescale | ( | const QSize & | oldSize, | |
const QSize & | newSize | |||
) | const [protected, virtual] |
Adjust the plot axes scales
- Parameters:
-
oldSize Previous size of the canvas newSize New size of the canvas
void QwtPlotRescaler::rescale | ( | ) | const |
Adjust the plot axes scales.
QwtPlotRescaler::RescalePolicy QwtPlotRescaler::rescalePolicy | ( | ) | const |
- Returns:
- Rescale policy
- See also:
- setRescalePolicy()
void QwtPlotRescaler::setAspectRatio | ( | int | axis, | |
double | ratio | |||
) |
Set the aspect ratio between the scale of the reference axis and another scale. The default ratio is 1.0
- Parameters:
-
axis Axis index ( see QwtPlot::AxisId ) ratio Aspect ratio
- See also:
- aspectRatio()
void QwtPlotRescaler::setAspectRatio | ( | double | ratio | ) |
Set the aspect ratio between the scale of the reference axis and the other scales. The default ratio is 1.0
- Parameters:
-
ratio Aspect ratio
- See also:
- aspectRatio()
void QwtPlotRescaler::setEnabled | ( | bool | on | ) |
En/disable the rescaler.
When enabled is true an event filter is installed for the canvas, otherwise the event filter is removed.
- Parameters:
-
on true or false
- See also:
- isEnabled(), eventFilter()
void QwtPlotRescaler::setExpandingDirection | ( | int | axis, | |
ExpandingDirection | direction | |||
) |
Set the direction in which an axis should be expanded
- Parameters:
-
axis Axis index ( see QwtPlot::AxisId ) direction Direction
- See also:
- expandingDirection()
void QwtPlotRescaler::setExpandingDirection | ( | ExpandingDirection | direction | ) |
Set the direction in which all axis should be expanded
- Parameters:
-
direction Direction
- See also:
- expandingDirection()
void QwtPlotRescaler::setReferenceAxis | ( | int | axis | ) |
Set the reference axis ( see RescalePolicy )
- Parameters:
-
axis Axis index ( QwtPlot::Axis )
- See also:
- referenceAxis()
void QwtPlotRescaler::setRescalePolicy | ( | RescalePolicy | policy | ) |
QwtDoubleInterval QwtPlotRescaler::syncScale | ( | int | axis, | |
const QwtDoubleInterval & | reference, | |||
const QSize & | size | |||
) | const [protected, virtual] |
Synchronize an axis scale according to the scale of the reference axis
- Parameters:
-
axis Axis index ( see QwtPlot::AxisId ) reference Interval of the reference axis size Size of the canvas
void QwtPlotRescaler::updateScales | ( | QwtDoubleInterval | intervals[QwtPlot::axisCnt] | ) | const [protected, virtual] |
Update the axes scales
- Parameters:
-
intervals Scale intervals
Generated on Wed Sep 2 18:37:26 2009 for Qwt User's Guide by
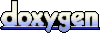