QwtSlider Class Reference
The Slider Widget. More...
#include <qwt_slider.h>
Public Types | |
enum | ScalePos { NoScale, LeftScale, RightScale, TopScale, BottomScale } |
enum | BGSTYLE { BgTrough = 0x1, BgSlot = 0x2, BgBoth = BgTrough | BgSlot } |
Public Member Functions | |
QwtSlider (QWidget *parent, Qt::Orientation=Qt::Horizontal, ScalePos=NoScale, BGSTYLE bgStyle=BgTrough) | |
virtual void | setOrientation (Qt::Orientation) |
void | setBgStyle (BGSTYLE) |
BGSTYLE | bgStyle () const |
void | setScalePosition (ScalePos s) |
ScalePos | scalePosition () const |
int | thumbLength () const |
int | thumbWidth () const |
int | borderWidth () const |
void | setThumbLength (int l) |
void | setThumbWidth (int w) |
void | setBorderWidth (int bw) |
void | setMargins (int x, int y) |
virtual QSize | sizeHint () const |
virtual QSize | minimumSizeHint () const |
void | setScaleDraw (QwtScaleDraw *) |
const QwtScaleDraw * | scaleDraw () const |
Protected Member Functions | |
virtual double | getValue (const QPoint &p) |
virtual void | getScrollMode (const QPoint &p, int &scrollMode, int &direction) |
void | draw (QPainter *p, const QRect &update_rect) |
virtual void | drawSlider (QPainter *p, const QRect &r) |
virtual void | drawThumb (QPainter *p, const QRect &, int pos) |
virtual void | resizeEvent (QResizeEvent *e) |
virtual void | paintEvent (QPaintEvent *e) |
virtual void | valueChange () |
virtual void | rangeChange () |
virtual void | scaleChange () |
virtual void | fontChange (const QFont &oldFont) |
void | layoutSlider (bool update=true) |
int | xyPosition (double v) const |
QwtScaleDraw * | scaleDraw () |
Detailed Description
The Slider Widget.
QwtSlider is a slider widget which operates on an interval of type double. QwtSlider supports different layouts as well as a scale.
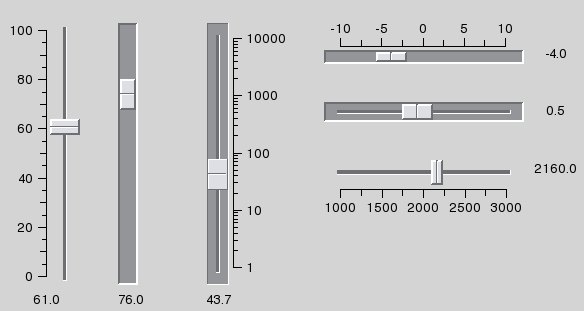
- See also:
- QwtAbstractSlider and QwtAbstractScale for the descriptions of the inherited members.
Member Enumeration Documentation
enum QwtSlider::BGSTYLE |
Background style.
- See also:
- QwtSlider()
enum QwtSlider::ScalePos |
Scale position. QwtSlider tries to enforce valid combinations of its orientation and scale position:
- Qt::Horizonal combines with NoScale, TopScale and BottomScale
- Qt::Vertical combines with NoScale, LeftScale and RightScale
- See also:
- QwtSlider()
Constructor & Destructor Documentation
QwtSlider::QwtSlider | ( | QWidget * | parent, | |
Qt::Orientation | orientation = Qt::Horizontal , |
|||
ScalePos | scalePos = NoScale , |
|||
BGSTYLE | bgStyle = BgTrough | |||
) | [explicit] |
Constructor.
- Parameters:
-
parent parent widget orientation Orientation of the slider. Can be Qt::Horizontal or Qt::Vertical. Defaults to Qt::Horizontal. scalePos Position of the scale. Defaults to QwtSlider::NoScale. bgStyle Background style. QwtSlider::BgTrough draws the slider button in a trough, QwtSlider::BgSlot draws a slot underneath the button. An or-combination of both may also be used. The default is QwtSlider::BgTrough.
QwtSlider enforces valid combinations of its orientation and scale position. If the combination is invalid, the scale position will be set to NoScale. Valid combinations are:
- Qt::Horizonal with NoScale, TopScale, or BottomScale;
- Qt::Vertical with NoScale, LeftScale, or RightScale.
Member Function Documentation
QwtSlider::BGSTYLE QwtSlider::bgStyle | ( | ) | const |
- Returns:
- the background style.
int QwtSlider::borderWidth | ( | ) | const |
- Returns:
- the border width.
void QwtSlider::draw | ( | QPainter * | p, | |
const QRect & | update_rect | |||
) | [protected] |
Draw the QwtSlider.
void QwtSlider::drawSlider | ( | QPainter * | painter, | |
const QRect & | r | |||
) | [protected, virtual] |
Draw the slider into the specified rectangle.
- Parameters:
-
painter Painter r Rectangle
void QwtSlider::drawThumb | ( | QPainter * | painter, | |
const QRect & | sliderRect, | |||
int | pos | |||
) | [protected, virtual] |
Draw the thumb at a position
- Parameters:
-
painter Painter sliderRect Bounding rectangle of the slider pos Position of the slider thumb
void QwtSlider::fontChange | ( | const QFont & | oldFont | ) | [protected, virtual] |
Notify change in font.
void QwtSlider::getScrollMode | ( | const QPoint & | p, | |
int & | scrollMode, | |||
int & | direction | |||
) | [protected, virtual] |
Determine scrolling mode and direction.
- Parameters:
-
p point scrollMode Scrolling mode direction Direction
Implements QwtAbstractSlider.
double QwtSlider::getValue | ( | const QPoint & | pos | ) | [protected, virtual] |
Determine the value corresponding to a specified mouse location.
- Parameters:
-
pos Mouse position
Implements QwtAbstractSlider.
void QwtSlider::layoutSlider | ( | bool | update_geometry = true |
) | [protected] |
Recalculate the slider's geometry and layout based on the current rect and fonts.
- Parameters:
-
update_geometry notify the layout system and call update to redraw the scale
QSize QwtSlider::minimumSizeHint | ( | ) | const [virtual] |
Return a minimum size hint.
- Warning:
- The return value of QwtSlider::minimumSizeHint() depends on the font and the scale.
void QwtSlider::paintEvent | ( | QPaintEvent * | event | ) | [protected, virtual] |
Qt paint event
- Parameters:
-
event Paint event
void QwtSlider::rangeChange | ( | ) | [protected, virtual] |
Notify change of range.
Reimplemented from QwtDoubleRange.
void QwtSlider::resizeEvent | ( | QResizeEvent * | e | ) | [protected, virtual] |
Qt resize event.
void QwtSlider::scaleChange | ( | ) | [protected, virtual] |
Notify changed scale.
Reimplemented from QwtAbstractScale.
QwtScaleDraw * QwtSlider::scaleDraw | ( | ) | [protected] |
- Returns:
- the scale draw of the slider
- See also:
- setScaleDraw()
const QwtScaleDraw * QwtSlider::scaleDraw | ( | ) | const |
- Returns:
- the scale draw of the slider
- See also:
- setScaleDraw()
QwtSlider::ScalePos QwtSlider::scalePosition | ( | ) | const |
Return the scale position.
void QwtSlider::setBgStyle | ( | BGSTYLE | st | ) |
Set the background style.
void QwtSlider::setBorderWidth | ( | int | bd | ) |
Change the slider's border width.
- Parameters:
-
bd border width
void QwtSlider::setMargins | ( | int | xMargin, | |
int | yMargin | |||
) |
Set distances between the widget's border and internals.
- Parameters:
-
xMargin Horizontal margin yMargin Vertical margin
void QwtSlider::setOrientation | ( | Qt::Orientation | o | ) | [virtual] |
Set the orientation.
- Parameters:
-
o Orientation. Allowed values are Qt::Horizontal and Qt::Vertical.
If the new orientation and the old scale position are an invalid combination, the scale position will be set to QwtSlider::NoScale.
- See also:
- QwtAbstractSlider::orientation()
Reimplemented from QwtAbstractSlider.
void QwtSlider::setScaleDraw | ( | QwtScaleDraw * | scaleDraw | ) |
Set a scale draw.
For changing the labels of the scales, it is necessary to derive from QwtScaleDraw and overload QwtScaleDraw::label().
- Parameters:
-
scaleDraw ScaleDraw object, that has to be created with new and will be deleted in ~QwtSlider or the next call of setScaleDraw().
void QwtSlider::setScalePosition | ( | ScalePos | s | ) |
Change the scale position (and slider orientation).
- Parameters:
-
s Position of the scale.
A valid combination of scale position and orientation is enforced:
- if the new scale position is Left or Right, the scale orientation will become Qt::Vertical;
- if the new scale position is Bottom or Top the scale orientation will become Qt::Horizontal;
- if the new scale position is QwtSlider::NoScale, the scale orientation will not change.
void QwtSlider::setThumbLength | ( | int | thumbLength | ) |
Set the slider's thumb length.
- Parameters:
-
thumbLength new length
void QwtSlider::setThumbWidth | ( | int | w | ) |
Change the width of the thumb.
- Parameters:
-
w new width
QSize QwtSlider::sizeHint | ( | ) | const [virtual] |
- Returns:
- QwtSlider::minimumSizeHint()
int QwtSlider::thumbLength | ( | ) | const |
- Returns:
- the thumb length.
int QwtSlider::thumbWidth | ( | ) | const |
- Returns:
- the thumb width.
void QwtSlider::valueChange | ( | ) | [protected, virtual] |
Notify change of value.
Reimplemented from QwtAbstractSlider.
int QwtSlider::xyPosition | ( | double | value | ) | const [protected] |
Find the x/y position for a given value v
- Parameters:
-
value Value
Generated on Wed Sep 2 18:37:27 2009 for Qwt User's Guide by
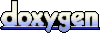