QwtPlotSpectrogram Class Reference
A plot item, which displays a spectrogram. More...
#include <qwt_plot_spectrogram.h>
Public Types | |
enum | DisplayMode { ImageMode = 1, ContourMode = 2 } |
Public Member Functions | |
QwtPlotSpectrogram (const QString &title=QString::null) | |
virtual | ~QwtPlotSpectrogram () |
void | setDisplayMode (DisplayMode, bool on=true) |
bool | testDisplayMode (DisplayMode) const |
void | setData (const QwtRasterData &data) |
const QwtRasterData & | data () const |
void | setColorMap (const QwtColorMap &) |
const QwtColorMap & | colorMap () const |
virtual QwtDoubleRect | boundingRect () const |
virtual QSize | rasterHint (const QwtDoubleRect &) const |
void | setDefaultContourPen (const QPen &) |
QPen | defaultContourPen () const |
virtual QPen | contourPen (double level) const |
void | setConrecAttribute (QwtRasterData::ConrecAttribute, bool on) |
bool | testConrecAttribute (QwtRasterData::ConrecAttribute) const |
void | setContourLevels (const QwtValueList &) |
QwtValueList | contourLevels () const |
virtual int | rtti () const |
virtual void | draw (QPainter *p, const QwtScaleMap &xMap, const QwtScaleMap &yMap, const QRect &rect) const |
Protected Member Functions | |
virtual QImage | renderImage (const QwtScaleMap &xMap, const QwtScaleMap &yMap, const QwtDoubleRect &rect) const |
virtual QSize | contourRasterSize (const QwtDoubleRect &, const QRect &) const |
virtual QwtRasterData::ContourLines | renderContourLines (const QwtDoubleRect &rect, const QSize &raster) const |
virtual void | drawContourLines (QPainter *p, const QwtScaleMap &xMap, const QwtScaleMap &yMap, const QwtRasterData::ContourLines &lines) const |
Detailed Description
A plot item, which displays a spectrogram.
A spectrogram displays threedimenional data, where the 3rd dimension ( the intensity ) is displayed using colors. The colors are calculated from the values using a color map.
In ContourMode contour lines are painted for the contour levels.
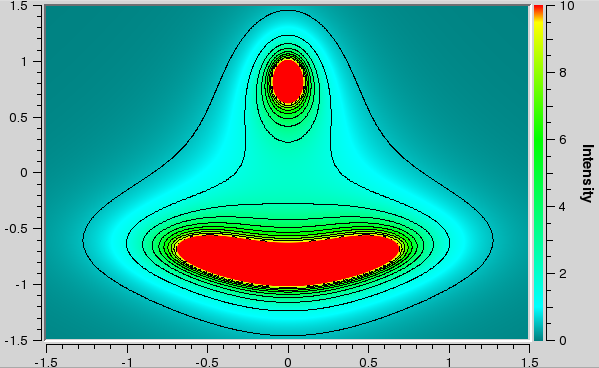
- See also:
- QwtRasterData, QwtColorMap
Member Enumeration Documentation
The display mode controls how the raster data will be represented.
- ImageMode
The values are mapped to colors using a color map. - ContourMode
The data is displayed using contour lines
When both modes are enabled the contour lines are painted on top of the spectrogram. The default setting enables ImageMode.
- See also:
- setDisplayMode(), testDisplayMode()
Constructor & Destructor Documentation
QwtPlotSpectrogram::QwtPlotSpectrogram | ( | const QString & | title = QString::null |
) | [explicit] |
Sets the following item attributes:
- QwtPlotItem::AutoScale: true
- QwtPlotItem::Legend: false
The z value is initialized by 8.0.
- Parameters:
-
title Title
QwtPlotSpectrogram::~QwtPlotSpectrogram | ( | ) | [virtual] |
Destructor.
Member Function Documentation
QwtDoubleRect QwtPlotSpectrogram::boundingRect | ( | ) | const [virtual] |
- Returns:
- Bounding rect of the data
- See also:
- QwtRasterData::boundingRect()
Reimplemented from QwtPlotItem.
const QwtColorMap & QwtPlotSpectrogram::colorMap | ( | ) | const |
- Returns:
- Color Map used for mapping the intensity values to colors
- See also:
- setColorMap()
QwtValueList QwtPlotSpectrogram::contourLevels | ( | ) | const |
Return the levels of the contour lines.
The levels are sorted in increasing order.
QPen QwtPlotSpectrogram::contourPen | ( | double | level | ) | const [virtual] |
Calculate the pen for a contour line.
The color of the pen is the color for level calculated by the color map
- Parameters:
-
level Contour level
- Returns:
- Pen for the contour line
- Note:
- contourPen is only used if defaultContourPen().style() == Qt::NoPen
QSize QwtPlotSpectrogram::contourRasterSize | ( | const QwtDoubleRect & | area, | |
const QRect & | rect | |||
) | const [protected, virtual] |
Return the raster to be used by the CONREC contour algorithm.
A larger size will improve the precisision of the CONREC algorithm, but will slow down the time that is needed to calculate the lines.
The default implementation returns rect.size() / 2 bounded to data().rasterHint().
- Parameters:
-
area Rect, where to calculate the contour lines rect Rect in pixel coordinates, where to paint the contour lines
- Returns:
- Raster to be used by the CONREC contour algorithm.
- Note:
- The size will be bounded to rect.size().
const QwtRasterData & QwtPlotSpectrogram::data | ( | ) | const |
- Returns:
- Spectrogram data
- See also:
- setData()
QPen QwtPlotSpectrogram::defaultContourPen | ( | ) | const |
- Returns:
- Default contour pen
- See also:
- setDefaultContourPen()
void QwtPlotSpectrogram::draw | ( | QPainter * | painter, | |
const QwtScaleMap & | xMap, | |||
const QwtScaleMap & | yMap, | |||
const QRect & | canvasRect | |||
) | const [virtual] |
Draw the spectrogram.
- Parameters:
-
painter Painter xMap Maps x-values into pixel coordinates. yMap Maps y-values into pixel coordinates. canvasRect Contents rect of the canvas in painter coordinates
Reimplemented from QwtPlotRasterItem.
void QwtPlotSpectrogram::drawContourLines | ( | QPainter * | painter, | |
const QwtScaleMap & | xMap, | |||
const QwtScaleMap & | yMap, | |||
const QwtRasterData::ContourLines & | contourLines | |||
) | const [protected, virtual] |
Paint the contour lines
- Parameters:
-
painter Painter xMap Maps x-values into pixel coordinates. yMap Maps y-values into pixel coordinates. contourLines Contour lines
- See also:
- renderContourLines(), defaultContourPen(), contourPen()
QSize QwtPlotSpectrogram::rasterHint | ( | const QwtDoubleRect & | rect | ) | const [virtual] |
Returns the recommended raster for a given rect.
F.e the raster hint is used to limit the resolution of the image that is rendered.
- Parameters:
-
rect Rect for the raster hint
- Returns:
- data().rasterHint(rect)
Reimplemented from QwtPlotRasterItem.
QwtRasterData::ContourLines QwtPlotSpectrogram::renderContourLines | ( | const QwtDoubleRect & | rect, | |
const QSize & | raster | |||
) | const [protected, virtual] |
Calculate contour lines
- Parameters:
-
rect Rectangle, where to calculate the contour lines raster Raster, used by the CONREC algorithm
QImage QwtPlotSpectrogram::renderImage | ( | const QwtScaleMap & | xMap, | |
const QwtScaleMap & | yMap, | |||
const QwtDoubleRect & | area | |||
) | const [protected, virtual] |
Render an image from the data and color map.
The area is translated into a rect of the paint device. For each pixel of this rect the intensity is mapped into a color.
- Parameters:
-
xMap X-Scale Map yMap Y-Scale Map area Area that should be rendered in scale coordinates.
- Returns:
- A QImage::Format_Indexed8 or QImage::Format_ARGB32 depending on the color map.
- See also:
- QwtRasterData::intensity(), QwtColorMap::rgb(), QwtColorMap::colorIndex()
Implements QwtPlotRasterItem.
int QwtPlotSpectrogram::rtti | ( | ) | const [virtual] |
- Returns:
- QwtPlotItem::Rtti_PlotSpectrogram
Reimplemented from QwtPlotItem.
void QwtPlotSpectrogram::setColorMap | ( | const QwtColorMap & | colorMap | ) |
Change the color map
Often it is useful to display the mapping between intensities and colors as an additional plot axis, showing a color bar.
- Parameters:
-
colorMap Color Map
- See also:
- colorMap(), QwtScaleWidget::setColorBarEnabled(), QwtScaleWidget::setColorMap()
void QwtPlotSpectrogram::setConrecAttribute | ( | QwtRasterData::ConrecAttribute | attribute, | |
bool | on | |||
) |
Modify an attribute of the CONREC algorithm, used to calculate the contour lines.
- Parameters:
-
attribute CONREC attribute on On/Off
void QwtPlotSpectrogram::setContourLevels | ( | const QwtValueList & | levels | ) |
Set the levels of the contour lines
- Parameters:
-
levels Values of the contour levels
- Note:
- contourLevels returns the same levels but sorted.
void QwtPlotSpectrogram::setData | ( | const QwtRasterData & | data | ) |
void QwtPlotSpectrogram::setDefaultContourPen | ( | const QPen & | pen | ) |
Set the default pen for the contour lines.
If the spectrogram has a valid default contour pen a contour line is painted using the default contour pen. Otherwise (pen.style() == Qt::NoPen) the pen is calculated for each contour level using contourPen().
- See also:
- defaultContourPen(), contourPen()
void QwtPlotSpectrogram::setDisplayMode | ( | DisplayMode | mode, | |
bool | on = true | |||
) |
The display mode controls how the raster data will be represented.
- Parameters:
-
mode Display mode on On/Off
The default setting enables ImageMode.
- See also:
- DisplayMode, displayMode()
bool QwtPlotSpectrogram::testConrecAttribute | ( | QwtRasterData::ConrecAttribute | attribute | ) | const |
Test an attribute of the CONREC algorithm, used to calculate the contour lines.
- Parameters:
-
attribute CONREC attribute
- Returns:
- true, is enabled
bool QwtPlotSpectrogram::testDisplayMode | ( | DisplayMode | mode | ) | const |
The display mode controls how the raster data will be represented.
- Parameters:
-
mode Display mode
- Returns:
- true if mode is enabled
Generated on Wed Sep 2 18:37:26 2009 for Qwt User's Guide by
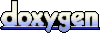