qwt_layout_metrics.h
00001 /* -*- mode: C++ ; c-file-style: "stroustrup" -*- ***************************** 00002 * Qwt Widget Library 00003 * Copyright (C) 1997 Josef Wilgen 00004 * Copyright (C) 2002 Uwe Rathmann 00005 * 00006 * This library is free software; you can redistribute it and/or 00007 * modify it under the terms of the Qwt License, Version 1.0 00008 *****************************************************************************/ 00009 00010 #ifndef QWT_LAYOUT_METRICS_H 00011 #define QWT_LAYOUT_METRICS_H 00012 00013 #include <qsize.h> 00014 #include <qrect.h> 00015 #include "qwt_polygon.h" 00016 #include "qwt_global.h" 00017 00018 class QPainter; 00019 class QString; 00020 class QFontMetrics; 00021 #if QT_VERSION < 0x040000 00022 class QWMatrix; 00023 #else 00024 class QMatrix; 00025 #endif 00026 class QPaintDevice; 00027 00043 class QWT_EXPORT QwtMetricsMap 00044 { 00045 public: 00046 QwtMetricsMap(); 00047 00048 bool isIdentity() const; 00049 00050 void setMetrics(const QPaintDevice *layoutMetrics, 00051 const QPaintDevice *deviceMetrics); 00052 00053 int layoutToDeviceX(int x) const; 00054 int deviceToLayoutX(int x) const; 00055 int screenToLayoutX(int x) const; 00056 int layoutToScreenX(int x) const; 00057 00058 int layoutToDeviceY(int y) const; 00059 int deviceToLayoutY(int y) const; 00060 int screenToLayoutY(int y) const; 00061 int layoutToScreenY(int y) const; 00062 00063 QPoint layoutToDevice(const QPoint &, const QPainter * = NULL) const; 00064 QPoint deviceToLayout(const QPoint &, const QPainter * = NULL) const; 00065 QPoint screenToLayout(const QPoint &) const; 00066 QPoint layoutToScreen(const QPoint &point) const; 00067 00068 00069 QSize layoutToDevice(const QSize &) const; 00070 QSize deviceToLayout(const QSize &) const; 00071 QSize screenToLayout(const QSize &) const; 00072 QSize layoutToScreen(const QSize &) const; 00073 00074 QRect layoutToDevice(const QRect &, const QPainter * = NULL) const; 00075 QRect deviceToLayout(const QRect &, const QPainter * = NULL) const; 00076 QRect screenToLayout(const QRect &) const; 00077 QRect layoutToScreen(const QRect &) const; 00078 00079 QwtPolygon layoutToDevice(const QwtPolygon &, 00080 const QPainter * = NULL) const; 00081 QwtPolygon deviceToLayout(const QwtPolygon &, 00082 const QPainter * = NULL) const; 00083 00084 #if QT_VERSION < 0x040000 00085 static QwtPolygon translate(const QWMatrix &, const QwtPolygon &); 00086 static QRect translate(const QWMatrix &, const QRect &); 00087 #else 00088 static QwtPolygon translate(const QMatrix &, const QwtPolygon &); 00089 static QRect translate(const QMatrix &, const QRect &); 00090 #endif 00091 00092 private: 00093 double d_screenToLayoutX; 00094 double d_screenToLayoutY; 00095 00096 double d_deviceToLayoutX; 00097 double d_deviceToLayoutY; 00098 }; 00099 00100 inline bool QwtMetricsMap::isIdentity() const 00101 { 00102 return d_deviceToLayoutX == 1.0 && d_deviceToLayoutY == 1.0; 00103 } 00104 00105 inline int QwtMetricsMap::layoutToDeviceX(int x) const 00106 { 00107 return qRound(x / d_deviceToLayoutX); 00108 } 00109 00110 inline int QwtMetricsMap::deviceToLayoutX(int x) const 00111 { 00112 return qRound(x * d_deviceToLayoutX); 00113 } 00114 00115 inline int QwtMetricsMap::screenToLayoutX(int x) const 00116 { 00117 return qRound(x * d_screenToLayoutX); 00118 } 00119 00120 inline int QwtMetricsMap::layoutToScreenX(int x) const 00121 { 00122 return qRound(x / d_screenToLayoutX); 00123 } 00124 00125 inline int QwtMetricsMap::layoutToDeviceY(int y) const 00126 { 00127 return qRound(y / d_deviceToLayoutY); 00128 } 00129 00130 inline int QwtMetricsMap::deviceToLayoutY(int y) const 00131 { 00132 return qRound(y * d_deviceToLayoutY); 00133 } 00134 00135 inline int QwtMetricsMap::screenToLayoutY(int y) const 00136 { 00137 return qRound(y * d_screenToLayoutY); 00138 } 00139 00140 inline int QwtMetricsMap::layoutToScreenY(int y) const 00141 { 00142 return qRound(y / d_screenToLayoutY); 00143 } 00144 00145 inline QSize QwtMetricsMap::layoutToDevice(const QSize &size) const 00146 { 00147 return QSize(layoutToDeviceX(size.width()), 00148 layoutToDeviceY(size.height())); 00149 } 00150 00151 inline QSize QwtMetricsMap::deviceToLayout(const QSize &size) const 00152 { 00153 return QSize(deviceToLayoutX(size.width()), 00154 deviceToLayoutY(size.height())); 00155 } 00156 00157 inline QSize QwtMetricsMap::screenToLayout(const QSize &size) const 00158 { 00159 return QSize(screenToLayoutX(size.width()), 00160 screenToLayoutY(size.height())); 00161 } 00162 00163 inline QSize QwtMetricsMap::layoutToScreen(const QSize &size) const 00164 { 00165 return QSize(layoutToScreenX(size.width()), 00166 layoutToScreenY(size.height())); 00167 } 00168 00169 #endif
Generated on Wed Sep 2 18:37:21 2009 for Qwt User's Guide by
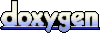