qwt_double_interval.h
00001 /* -*- mode: C++ ; c-file-style: "stroustrup" -*- ***************************** 00002 * Qwt Widget Library 00003 * Copyright (C) 1997 Josef Wilgen 00004 * Copyright (C) 2002 Uwe Rathmann 00005 * 00006 * This library is free software; you can redistribute it and/or 00007 * modify it under the terms of the Qwt License, Version 1.0 00008 *****************************************************************************/ 00009 00010 #ifndef QWT_DOUBLE_INTERVAL_H 00011 #define QWT_DOUBLE_INTERVAL_H 00012 00013 #include "qwt_global.h" 00014 00021 class QWT_EXPORT QwtDoubleInterval 00022 { 00023 public: 00038 enum BorderMode 00039 { 00040 IncludeBorders = 0, 00041 00042 ExcludeMinimum = 1, 00043 ExcludeMaximum = 2, 00044 00045 ExcludeBorders = ExcludeMinimum | ExcludeMaximum 00046 }; 00047 00048 inline QwtDoubleInterval(); 00049 inline QwtDoubleInterval(double minValue, double maxValue, 00050 int borderFlags = IncludeBorders); 00051 00052 inline void setInterval(double minValue, double maxValue, 00053 int borderFlags = IncludeBorders); 00054 00055 QwtDoubleInterval normalized() const; 00056 QwtDoubleInterval inverted() const; 00057 QwtDoubleInterval limited(double minValue, double maxValue) const; 00058 00059 inline int operator==(const QwtDoubleInterval &) const; 00060 inline int operator!=(const QwtDoubleInterval &) const; 00061 00062 inline void setBorderFlags(int); 00063 inline int borderFlags() const; 00064 00065 inline double minValue() const; 00066 inline double maxValue() const; 00067 00068 inline double width() const; 00069 00070 inline void setMinValue(double); 00071 inline void setMaxValue(double); 00072 00073 bool contains(double value) const; 00074 00075 bool intersects(const QwtDoubleInterval &) const; 00076 QwtDoubleInterval intersect(const QwtDoubleInterval &) const; 00077 QwtDoubleInterval unite(const QwtDoubleInterval &) const; 00078 00079 inline QwtDoubleInterval operator|(const QwtDoubleInterval &) const; 00080 inline QwtDoubleInterval operator&(const QwtDoubleInterval &) const; 00081 00082 QwtDoubleInterval &operator|=(const QwtDoubleInterval &); 00083 QwtDoubleInterval &operator&=(const QwtDoubleInterval &); 00084 00085 QwtDoubleInterval extend(double value) const; 00086 inline QwtDoubleInterval operator|(double) const; 00087 QwtDoubleInterval &operator|=(double); 00088 00089 inline bool isValid() const; 00090 inline bool isNull() const; 00091 inline void invalidate(); 00092 00093 QwtDoubleInterval symmetrize(double value) const; 00094 00095 private: 00096 double d_minValue; 00097 double d_maxValue; 00098 int d_borderFlags; 00099 }; 00100 00107 inline QwtDoubleInterval::QwtDoubleInterval(): 00108 d_minValue(0.0), 00109 d_maxValue(-1.0), 00110 d_borderFlags(IncludeBorders) 00111 { 00112 } 00113 00123 inline QwtDoubleInterval::QwtDoubleInterval( 00124 double minValue, double maxValue, int borderFlags): 00125 d_minValue(minValue), 00126 d_maxValue(maxValue), 00127 d_borderFlags(borderFlags) 00128 { 00129 } 00130 00138 inline void QwtDoubleInterval::setInterval( 00139 double minValue, double maxValue, int borderFlags) 00140 { 00141 d_minValue = minValue; 00142 d_maxValue = maxValue; 00143 d_borderFlags = borderFlags; 00144 } 00145 00152 inline void QwtDoubleInterval::setBorderFlags(int borderFlags) 00153 { 00154 d_borderFlags = borderFlags; 00155 } 00156 00161 inline int QwtDoubleInterval::borderFlags() const 00162 { 00163 return d_borderFlags; 00164 } 00165 00171 inline void QwtDoubleInterval::setMinValue(double minValue) 00172 { 00173 d_minValue = minValue; 00174 } 00175 00181 inline void QwtDoubleInterval::setMaxValue(double maxValue) 00182 { 00183 d_maxValue = maxValue; 00184 } 00185 00187 inline double QwtDoubleInterval::minValue() const 00188 { 00189 return d_minValue; 00190 } 00191 00193 inline double QwtDoubleInterval::maxValue() const 00194 { 00195 return d_maxValue; 00196 } 00197 00205 inline double QwtDoubleInterval::width() const 00206 { 00207 return isValid() ? (d_maxValue - d_minValue) : 0.0; 00208 } 00209 00214 inline QwtDoubleInterval QwtDoubleInterval::operator&( 00215 const QwtDoubleInterval &interval ) const 00216 { 00217 return intersect(interval); 00218 } 00219 00224 inline QwtDoubleInterval QwtDoubleInterval::operator|( 00225 const QwtDoubleInterval &interval) const 00226 { 00227 return unite(interval); 00228 } 00229 00231 inline int QwtDoubleInterval::operator==(const QwtDoubleInterval &other) const 00232 { 00233 return (d_minValue == other.d_minValue) && 00234 (d_maxValue == other.d_maxValue) && 00235 (d_borderFlags == other.d_borderFlags); 00236 } 00237 00239 inline int QwtDoubleInterval::operator!=(const QwtDoubleInterval &other) const 00240 { 00241 return (!(*this == other)); 00242 } 00243 00248 inline QwtDoubleInterval QwtDoubleInterval::operator|(double value) const 00249 { 00250 return extend(value); 00251 } 00252 00254 inline bool QwtDoubleInterval::isNull() const 00255 { 00256 return isValid() && d_minValue >= d_maxValue; 00257 } 00258 00264 inline bool QwtDoubleInterval::isValid() const 00265 { 00266 if ( (d_borderFlags & ExcludeBorders) == 0 ) 00267 return d_minValue <= d_maxValue; 00268 else 00269 return d_minValue < d_maxValue; 00270 } 00271 00278 inline void QwtDoubleInterval::invalidate() 00279 { 00280 d_minValue = 0.0; 00281 d_maxValue = -1.0; 00282 } 00283 00284 #endif
Generated on Wed Sep 2 18:37:21 2009 for Qwt User's Guide by
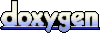