![]() |
MQTT Client 1.0.0
|
D:/my_data/GIT/network_apps/netapps/mqtt/client/mqtt_client.h
Go to the documentation of this file.
00001 00002 /****************************************************************************** 00003 * 00004 * Copyright (C) 2014 Texas Instruments Incorporated 00005 * 00006 * All rights reserved. Property of Texas Instruments Incorporated. 00007 * Restricted rights to use, duplicate or disclose this code are 00008 * granted through contract. 00009 * 00010 * The program may not be used without the written permission of 00011 * Texas Instruments Incorporated or against the terms and conditions 00012 * stipulated in the agreement under which this program has been supplied, 00013 * and under no circumstances can it be used with non-TI connectivity device. 00014 * 00015 ******************************************************************************/ 00016 00017 /* 00018 mqtt_client.h 00019 00020 This module enumerates the public interfaces / API of the MQTT Client 00021 Library. 00022 */ 00023 00024 00025 #ifndef __MQTT_CLIENT_H__ 00026 #define __MQTT_CLIENT_H__ 00027 00076 #include "mqtt_common.h" 00077 00078 /*------------------------------------------------------------------------------ 00079 * MQTT Client Messaging Routines / Services 00080 *------------------------------------------------------------------------------ 00081 */ 00082 00087 #define MQTT_CLIENT_VERSTR "1.0.0" 00093 u16 mqtt_client_new_msg_id(void); 00094 00110 bool mqtt_client_is_connected(void *ctx); 00111 00137 i32 mqtt_connect_msg_send(void *ctx, bool clean_session, u16 ka_secs); 00138 00168 i32 mqtt_client_pub_msg_send(void *ctx, 00169 const struct utf8_string *topic, 00170 const u8 *data_buf, u32 data_len, 00171 enum mqtt_qos qos, bool retain); 00172 00211 i32 mqtt_client_pub_dispatch(void *ctx, struct mqtt_packet *mqp, 00212 enum mqtt_qos qos, bool retain); 00213 00243 i32 mqtt_sub_msg_send(void *ctx, const struct utf8_strqos *qos_topics, u32 count); 00244 00283 i32 mqtt_sub_dispatch(void *ctx, struct mqtt_packet *mqp); 00284 00315 i32 mqtt_unsub_msg_send(void *ctx, const struct utf8_string *topics, u32 count); 00316 00356 i32 mqtt_unsub_dispatch(void *ctx, struct mqtt_packet *mqp); 00357 00365 i32 mqtt_pingreq_send(void *ctx); 00366 00373 i32 mqtt_disconn_send(void *ctx); 00374 00375 00431 i32 mqtt_client_send_progress(void *ctx); 00432 00455 i32 mqtt_client_ctx_await_msg(void *ctx, u8 msg_type, struct mqtt_packet *mqp, 00456 u32 wait_secs); 00457 00462 static inline 00463 i32 mqtt_client_ctx_recv(void *ctx, struct mqtt_packet *mqp, u32 wait_secs) 00464 { 00465 /* Receive next and any MQTT Message from the broker */ 00466 return mqtt_client_ctx_await_msg(ctx, 0x00, mqp, wait_secs); 00467 } 00468 00491 i32 mqtt_client_ctx_run(void *ctx, u32 wait_secs); 00492 00513 i32 mqtt_client_await_msg(struct mqtt_packet *mqp, u32 wait_secs, void **app); 00514 00537 i32 mqtt_client_run(u32 wait_secs); 00538 00539 /*------------------------------------------------------------------------------ 00540 * MQTT Client Library: Packet Buffer Pool and its management 00541 *------------------------------------------------------------------------------ 00542 */ 00543 00557 struct mqtt_packet *mqp_client_alloc(u8 msg_type, u8 offset); 00558 00564 static inline struct mqtt_packet *mqp_client_send_alloc(u8 msg_type) 00565 { 00566 return mqp_client_alloc(msg_type, MAX_FH_LEN); 00567 } 00568 00574 static inline struct mqtt_packet *mqp_client_recv_alloc(u8 msg_type) 00575 { 00576 return mqp_client_alloc(msg_type, 0); 00577 } 00578 00609 i32 mqtt_client_buffers_register(u32 num_mqp, struct mqtt_packet *mqp_vec, 00610 u32 buf_len, u8 *buf_vec); 00611 00612 /*------------------------------------------------------------------------------ 00613 * MQTT Client Library: Register application, platform information and services. 00614 *------------------------------------------------------------------------------ 00615 */ 00616 00639 i32 mqtt_client_ctx_info_register(void *ctx, 00640 const struct utf8_string *client_id, 00641 const struct utf8_string *user_name, 00642 const struct utf8_string *pass_word); 00643 00664 i32 mqtt_client_ctx_will_register(void *ctx, 00665 const struct utf8_string *will_top, 00666 const struct utf8_string *will_msg, 00667 enum mqtt_qos will_qos, bool retain); 00668 00678 i32 mqtt_client_net_svc_register(const struct device_net_services *net); 00679 00682 #define VHB_CONNACK_RC(vh_buf) (vh_buf[1]) 00683 #define MQP_CONNACK_RC(mqp) (mqp->buffer[3]) 00685 #define VHB_CONNACK_SP(vh_buf) (vh_buf[0] & 0x1) 00686 #define MQP_CONNACK_SP(mqp) (mqp->buffer[2] & 0x1) 00689 #define VHB_CONNACK_VH16(vh_buf)((vh_buf[0] << 8) | vh_buf[1]) 00690 #define MQP_CONNACK_VH16(mqp) ((mqp->buffer[2] << 8) | mqp->buffer[3]) 00691 00693 struct mqtt_client_ctx_cbs { 00694 00729 bool (*publish_rx)(void *app, 00730 bool dup, enum mqtt_qos qos, bool retain, 00731 struct mqtt_packet *mqp); 00732 00757 void (*ack_notify)(void *app, u8 msg_type, u16 msg_id, u8 *buf, u32 len); 00758 00768 void (*disconn_cb)(void *app, i32 cause); 00769 }; 00770 00771 struct mqtt_client_ctx_cfg { 00772 00776 #define MQTT_CFG_PROTOCOL_V31 0x0001 00777 #define MQTT_CFG_APP_HAS_RTSK 0x0002 00778 #define MQTT_CFG_MK_GROUP_CTX 0x0004 00781 u16 config_opts; 00786 #define MQTT_NETCONN_OPT_IP6 DEV_NETCONN_OPT_IP6 00787 #define MQTT_NETCONN_OPT_URL DEV_NETCONN_OPT_URL 00788 #define MQTT_NETCONN_OPT_SEC DEV_NETCONN_OPT_SEC 00791 u32 nwconn_opts; 00793 i8 *server_addr; 00794 u16 port_number; 00795 struct secure_conn *nw_security; 00796 }; 00797 00840 i32 mqtt_client_ctx_create(const struct mqtt_client_ctx_cfg *ctx_cfg, 00841 const struct mqtt_client_ctx_cbs *ctx_cbs, 00842 void *app, void **ctx); 00843 00858 i32 mqtt_client_ctx_delete(void *ctx); 00859 00861 struct mqtt_client_lib_cfg { 00862 00867 u16 loopback_port; 00868 bool grp_uses_cbfn; 00871 void *mutex; 00872 void (*mutex_lockin)(void *mutex); 00873 void (*mutex_unlock)(void *mutex); 00875 i32 (*debug_printf)(const i8 *format, ...); 00876 bool aux_debug_en; 00877 }; 00878 00904 i32 mqtt_client_lib_init(const struct mqtt_client_lib_cfg *cfg); 00905 00909 i32 mqtt_client_lib_exit(void); 00910 /* End group client_api */ 00912 00913 #endif
Generated on Mon Nov 17 2014 12:11:04 for MQTT Client by
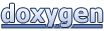