![]() |
MQTT Client 1.0.0
|
Classes | |
struct | mqtt_client_ctx_cbs |
struct | mqtt_client_ctx_cfg |
struct | mqtt_client_lib_cfg |
Defines | |
#define | MQTT_CLIENT_VERSTR "1.0.0" |
#define | VHB_CONNACK_RC(vh_buf) (vh_buf[1]) |
#define | MQP_CONNACK_RC(mqp) (mqp->buffer[3]) |
#define | VHB_CONNACK_SP(vh_buf) (vh_buf[0] & 0x1) |
#define | MQP_CONNACK_SP(mqp) (mqp->buffer[2] & 0x1) |
#define | VHB_CONNACK_VH16(vh_buf) ((vh_buf[0] << 8) | vh_buf[1]) |
#define | MQP_CONNACK_VH16(mqp) ((mqp->buffer[2] << 8) | mqp->buffer[3]) |
Functions | |
u16 | mqtt_client_new_msg_id (void) |
bool | mqtt_client_is_connected (void *ctx) |
i32 | mqtt_connect_msg_send (void *ctx, bool clean_session, u16 ka_secs) |
i32 | mqtt_client_pub_msg_send (void *ctx, const struct utf8_string *topic, const u8 *data_buf, u32 data_len, enum mqtt_qos qos, bool retain) |
i32 | mqtt_client_pub_dispatch (void *ctx, struct mqtt_packet *mqp, enum mqtt_qos qos, bool retain) |
i32 | mqtt_sub_msg_send (void *ctx, const struct utf8_strqos *qos_topics, u32 count) |
i32 | mqtt_sub_dispatch (void *ctx, struct mqtt_packet *mqp) |
i32 | mqtt_unsub_msg_send (void *ctx, const struct utf8_string *topics, u32 count) |
i32 | mqtt_unsub_dispatch (void *ctx, struct mqtt_packet *mqp) |
i32 | mqtt_pingreq_send (void *ctx) |
i32 | mqtt_disconn_send (void *ctx) |
i32 | mqtt_client_send_progress (void *ctx) |
i32 | mqtt_client_ctx_await_msg (void *ctx, u8 msg_type, struct mqtt_packet *mqp, u32 wait_secs) |
i32 | mqtt_client_ctx_run (void *ctx, u32 wait_secs) |
i32 | mqtt_client_await_msg (struct mqtt_packet *mqp, u32 wait_secs, void **app) |
i32 | mqtt_client_run (u32 wait_secs) |
struct mqtt_packet * | mqp_client_alloc (u8 msg_type, u8 offset) |
i32 | mqtt_client_buffers_register (u32 num_mqp, struct mqtt_packet *mqp_vec, u32 buf_len, u8 *buf_vec) |
i32 | mqtt_client_ctx_info_register (void *ctx, const struct utf8_string *client_id, const struct utf8_string *user_name, const struct utf8_string *pass_word) |
i32 | mqtt_client_ctx_will_register (void *ctx, const struct utf8_string *will_top, const struct utf8_string *will_msg, enum mqtt_qos will_qos, bool retain) |
i32 | mqtt_client_net_svc_register (const struct device_net_services *net) |
i32 | mqtt_client_ctx_create (const struct mqtt_client_ctx_cfg *ctx_cfg, const struct mqtt_client_ctx_cbs *ctx_cbs, void *app, void **ctx) |
i32 | mqtt_client_ctx_delete (void *ctx) |
i32 | mqtt_client_lib_init (const struct mqtt_client_lib_cfg *cfg) |
i32 | mqtt_client_lib_exit (void) |
Define Documentation
#define MQP_CONNACK_RC | ( | mqp | ) | (mqp->buffer[3]) |
CONNACK MQP:: Return Code
#define MQP_CONNACK_SP | ( | mqp | ) | (mqp->buffer[2] & 0x1) |
CONNACK MQP:: \ Session Bit
#define MQTT_CLIENT_VERSTR "1.0.0" |
Version of Client LIB
#define VHB_CONNACK_RC | ( | vh_buf | ) | (vh_buf[1]) |
Helper functions & macros to derive 16 bit CONNACK Return Code from broker. CONNACK VH:: Return Code
#define VHB_CONNACK_SP | ( | vh_buf | ) | (vh_buf[0] & 0x1) |
CONNACK VH:: Session Bit
Function Documentation
struct mqtt_packet* mqp_client_alloc | ( | u8 | msg_type, |
u8 | offset | ||
) | [read] |
Allocates a free MQTT Packet Buffer. The pool that will be used by the library to allocate a free MQP buffer must be configured (i.e. registered) a-priori by the app.
The parameter 'offset' is used to specify the number of bytes that are reserved for the header in the buffer
- Parameters:
-
[in] msg_type Message Type for which MQP buffer is being assigned. [in] offset Number of bytes to be reserved for MQTT headers.
- Returns:
- A NULL on error, otherwise a reference to a valid packet holder.
- See also:
- mqtt_client_register_buffers
i32 mqtt_client_await_msg | ( | struct mqtt_packet * | mqp, |
u32 | wait_secs, | ||
void ** | app | ||
) |
Block to receive any message for the grouped contexts within specified time. This service is valid only for the set-up, where the applicatiion has not configured the grouped contexts in the callback mode. The caller must provide a packet buffer of adequate size to hold the expected message from the server.
The wait time implies the maximum intermediate duration between the reception of two successive messages from the server. If no message is received before the expiry of the wait time, the routine returns. However, the routine would continue to block, in case, messages are being received within the successive period of wait time.
- Parameters:
-
[out] mqp packet buffer to hold the message received from the server. [in] wait_secs Maximum Time to wait for a message from the server. [out] app place holder to indicate application handle for the packet.
- Returns:
- On success, the number of bytes received for 'msg_type' from server, otherwise LIB defined error values (LIBRARY Generated Error Codes)
- Note:
- if the value of MQP_ERR_LIBQUIT is returned, then system must be restarted.
i32 mqtt_client_buffers_register | ( | u32 | num_mqp, |
struct mqtt_packet * | mqp_vec, | ||
u32 | buf_len, | ||
u8 * | buf_vec | ||
) |
Create a pool of MQTT Packet Buffers for the client library. This routine creates a pool of free MQTT Packet Buffers by attaching a buffer (buf) to a packet holder (mqp). The count of mqp elements and buf elements in the routine are same. And the size of the buffer in constant across all the elements.
The MQTT Packet Buffer pool should support (a) certain number of in-flight and stored packets that await ACK(s) from the server (b) certain number of packets from server whose processing would be deferred by the client app (to another context) (c) a packet to create a CONNECT message to re-establish transaction with the server.
A meaningful size of the pool is very much application specific and depends on the target functionality. For example, an application that intends to have only one in-flight message to the server would need atmost three MQP buffers (1 for TX (for Qos1 or 2 store), 1 for RX and 1 for CONNECT message). If the application sends only QoS0 messages to the server, then the number of MQP buffers would reduce to two (i.e. 1 Tx to support CONNECT / PUB out and 1 RX)
- Parameters:
-
[in] num_mqp Number or count of elements in mqp_vec and buf_vec. [in] mqp_vec An array of MQTT Packet Holder without a buffer. [in] buf_len The size or length of the buffer element in the 'buf_vec' [in] buf_vec An array of buffers. 0 on success otherwise -1 on error.
- Note:
- The parameters mqp_vec and buf_vec should be peristent entities.
- See also:
- mqtt_client_await_msg
- mqtt_client_run
i32 mqtt_client_ctx_await_msg | ( | void * | ctx, |
u8 | msg_type, | ||
struct mqtt_packet * | mqp, | ||
u32 | wait_secs | ||
) |
Block on the 'context' to receive a message type with-in specified wait time. This service is valid only for the configuration, where the application has not provided the callbacks to the client LIB 'context'. The caller must provide a packet buffer of adequate size to hold the expected message from the server.
The wait time implies the maximum intermediate duration between the reception of two successive messages from the server. If no message is received before the expiry of the wait time, the routine returns. However, the routine would continue to block, in case, messages are being received within the successive period of wait time and these messages are not the one that client is waiting for.
- Parameters:
-
[in] ctx handle to the underlying network context in the LIB
- See also:
- mqtt_client_ctx_create
- Parameters:
-
[in] msg_type message type to receive. A value of 0 would imply that caller is ready to receive the next message, whatsoever, from the server. [out] mqp packet buffer to hold the message received from the server. [in] wait_secs maximum Time to wait for a message from the server.
- Returns:
- On success, the number of bytes received for 'msg_type' from server, otherwise LIB defined error values (LIBRARY Generated Error Codes)
i32 mqtt_client_ctx_create | ( | const struct mqtt_client_ctx_cfg * | ctx_cfg, |
const struct mqtt_client_ctx_cbs * | ctx_cbs, | ||
void * | app, | ||
void ** | ctx | ||
) |
Create a Network Connection Context. This routine sets-up the parameters that are required to operate and manage the network connection with a MQTT server / broker. As part of the creation of a context, the implementation also records the handle, if provided, by the application. In addition, the invoker of the routine must facilitate a place holder to enable the client LIB to provision the reference to the 'context', so created.
Specifically, this routine associates or ties-up, in an one-to-one manner, the caller provided handle 'app' and the client LIB provisioned handle 'ctx'. The parameter 'app' is returned by the client LIB in certain other routines to indicate the underlying 'context' with which network transaction or event is associated. Similarly, the caller must specify the context handle 'ctx' for which the services are being invoked.
A user or a task prior to utilizing the services of the library to schedule MQTT transactions must create a 'context'. A client LIB 'context' can be operated in two modes: (a) sync-wait or explicit receive mode and (b) the callback mode. Provisioning or absence of the callback parameter in this routine defines the mode of operation of the 'context'.
Explicit receive mode is analogous to the paradigm of the socket programming in which an application utilize the recv() function call. It is anticipated that applications which would make use of limited set of MQTT messages may find this mode of operation useful. Applications which intend to operate the 'context' in this mode must not provision any callbacks.
On the other hand, certain applications, may prefer an asynchronous mode of operation and would want the client LIB 'context' to raise callbacks into the application, as and when, packets arrive from the server. And such applications must provide the callback routines.
- Parameters:
-
[in] ctx_cfg configuration information for the Network Context. [in] ctx_cbs callback routines. Must be set to NULL, if the application intends to operate the context in the sync-wait / explicit receive mode. [in] app handle to application. Returned by LIB in other routines to refere to the underlying context. [out] ctx reference to the context created and is provisioned by the implementation. (Valid only if routine returns a success)
- Returns:
- 0 on success otherwise -1.
i32 mqtt_client_ctx_delete | ( | void * | ctx | ) |
Delete a Network Connection Context. This routines destroys the previously created network 'context' and releases resources that would be assigned for maintaining the information about the 'context'.
A user or a task prior to deleting the 'context' must ensure that there is no active MQTT connection on this context.
- Parameters:
-
[in] ctx handle to network context to be deleted. The context must have been previously created.
- Returns:
- 0 on success otherwise -1
i32 mqtt_client_ctx_info_register | ( | void * | ctx, |
const struct utf8_string * | client_id, | ||
const struct utf8_string * | user_name, | ||
const struct utf8_string * | pass_word | ||
) |
Register application info and its credentials with the client library. This routine registers information for all the specificed parameters, therefore, an upate to single element would imply re-specification of the other paramters, as well.
- Note:
- Contents embedded in the parameters is not copied by the routine, and instead a reference to the listed constructs is retained. Therefore, the app must enable the parameter contents for persistency.
- Parameters:
-
[in] ctx handle to the underlying network context in the LIB
- See also:
- mqtt_client_ctx_create
- Parameters:
-
[in] client_id MQTT UTF8 identifier of the client. If set to NULL, then the client will be treated as zero length entity. [in] user_name MQTT UTF8 user name for the client. If not used, set it to NULL. If used, then it can't be of zero length. [in] pass_word MQTT UTF8 pass word for the client. If not used, set it to NULL, If used, then it can't be of zero length.
- Returns:
- 0 on success otherwise -1
User name without a pass word is a valid configuration. A pass word won't be processed if it is not associated with a valid user name.
i32 mqtt_client_ctx_run | ( | void * | ctx, |
u32 | wait_secs | ||
) |
Run the context for the specificed wait time. This service is valid only for the configuration, where the application has populated the callbacks that can be invoked by the client LIB 'context'.
This routine yields the control back to the application after the duration of the wait time. Such an arrangement enable the application to make overall progress to meet its intended functionality.
The wait time implies the maximum intermediate duration between the reception of two successive messages from the server. If no message is received before the expiry of the wait time, the routine returns. However, the routine would continue to block, in case, messages are being received within the successive period of the wait time.
- Parameters:
-
[in] ctx handle to the underlying network context in the LIB
- See also:
- mqtt_client_ctx_create
- Parameters:
-
[in] wait_secs maximum time to wait for a message from the server
- Returns:
- MQP_ERR_NOTCONN if MQTT connection is closed by the application, MQP_ERR_TIMEOUT if there was no MQTT transaction in the interval of wait time and other values (LIBRARY Generated Error Codes)
i32 mqtt_client_ctx_will_register | ( | void * | ctx, |
const struct utf8_string * | will_top, | ||
const struct utf8_string * | will_msg, | ||
enum mqtt_qos | will_qos, | ||
bool | retain | ||
) |
Register WILL information of the client application. This routine registers information for all the specificed parameters, therefore, an update to single element would imply re-specification of the other paramters, as well.
- Note:
- Contents embedded in the parameters is not copied by the routine, and instead a reference to the listed constructs is retained. Therefore, the app must enable the parameter contents for persistency.
- Parameters:
-
[in] ctx handle to the underlying network context in the LIB
- See also:
- mqtt_client_ctx_create
- Parameters:
-
[in] will_top UTF8 WILL Topic on which WILL message is to be published. [in] will_msg UTF8 WILL message. [in] will_qos QOS for the WILL message [in] retain asserted to indicate that published WILL must be retained
- Returns:
- 0 on success otherwise -1.
Both will_top and will_msg should be either present or should be NULL. will_qos and retain are relevant only for a valid Topic and Message combo.
bool mqtt_client_is_connected | ( | void * | ctx | ) |
Ascertain whether connection / session with the server is active or not. Prior to sending out any information any message to server, the application can use this routine to check the status of the connection. If connection does not exist, then client should first CONNECT to the broker.
A connection to server could have been closed unsolicitedly either due to keep alive time-out or due to error in RX / TX transactions.
- Note:
- this API does not refer to network layer connection
- Parameters:
-
[in] ctx handle to the underlying network context in the LIB
- See also:
- mqtt_client_ctx_create
- Returns:
- true if connection is active otherwise false.
i32 mqtt_client_lib_exit | ( | void | ) |
Exit the MQTT client library.
- Returns:
- 0 on success otherwise -1.
i32 mqtt_client_lib_init | ( | const struct mqtt_client_lib_cfg * | cfg | ) |
Initialize the MQTT client library. This routine initializes all the common constructs that are required to manage the multiple network connetions. The client LIB must be initialized prior to invoking of any other routine or service.
- Note:
- This routine must be invoked only once in an run of the system.
Depending upon the deployment needs, this routine can be invoked either as part of the platform initialization sequence or as part of the application. Deployments that have more than one application utilizing the services of the client LIB should try to invoke the routine from the initialization sequence of the platform.
In addition, if an application has to manage more than one network connections (i.e. in other words, if the application has to handle a group of connections), then certain configuration must be set in the LIB
- See also:
- struct mqtt_client_lib_cfg
- Note:
- There must be only one group of network connetions in the system.
- Parameters:
-
[in] cfg Configuration information for the MQTT client Library.
- Returns:
- 0 on success otherwise -1.
i32 mqtt_client_net_svc_register | ( | const struct device_net_services * | net | ) |
Abstraction for the device specific network services. Network services for communication with the server
- Parameters:
-
[in] net refers to network services supported by the platform
- Returns:
- on success, 0, otherwise -1
Abstraction of Network Services on a platform
- Note:
- all entries in net must be supported by the platform.
u16 mqtt_client_new_msg_id | ( | void | ) |
Provides a new MSG Identifier for a packet dispatch to server
- Returns:
- MSG / PKT Transaction identifier
i32 mqtt_client_pub_dispatch | ( | void * | ctx, |
struct mqtt_packet * | mqp, | ||
enum mqtt_qos | qos, | ||
bool | retain | ||
) |
Dispatch application constructed PUBLISH message to the server. Prior to sending the message to the server, this routine will prepare a fixed header to account for the size of the contents and the flags that have been indicated by the caller.
After the packet has been sent to the server, if the associated QoS of the dispatched packet is ether level 1 or 2, the client LIB 'context' will then store the packet until the time, a corresponding PUB-ACK (for QoS1) or PUB-REC (QoS2) message is received from the server.
If the client LIB 'context' has been configured to assert 'clean session', then the references to all the stored and unacknowledged PUBLISH messages are dropped at the time of MQTT disconnection (or network disconnection). Otherwise, these unacknowledged packets continue to be availalbe for the next iteration of the MQTT connection. However, if the client application asserts the 'clean session' parameter in the next iteration of the CONNECT operation, then references to all the stored PUBLISH messages, if any, are dropped.
The caller must populate the payload information with topic and data before invoking this service.
This service facilitates direct writing of topic and (real-time) payload data into the buffer, thereby, avoiding power consuming and wasteful intermediate data copies.
In case, the routine returns an error, the caller is responsbile for freeing up or re-using the packet buffer. For all other cases, the client library will manage the return of the packet buffer to the pool.
- Parameters:
-
[in] ctx handle to the underlying network context in the LIB
- See also:
- mqtt_client_ctx_create
- Parameters:
-
[in] mqp app created PUBLISH message without the fixed header [in] qos QoS with which the message needs to send to server [in] retain Asserted if the message is to be retained by server.
- Returns:
- on success, the transaction Message ID, otherwise LIB defined errors (LIBRARY Generated Error Codes)
i32 mqtt_client_pub_msg_send | ( | void * | ctx, |
const struct utf8_string * | topic, | ||
const u8 * | data_buf, | ||
u32 | data_len, | ||
enum mqtt_qos | qos, | ||
bool | retain | ||
) |
Send a PUBLISH message to the server (don't wait for PUBACK / PUBREC). This routine creates a PUBLISH message in an internally allocated packet buffer by embedding the 'topic' and 'data' contents, then prepares the packet header and finally, dispatches the message to the server.
After the packet has been sent to the server, if the associated QoS of the dispatched packet is ether level 1 or 2, the client LIB 'context' will then store the packet until the time, a corresponding PUB-ACK (for QoS1) or PUB-REC (QoS2) message is received from the server.
If the client LIB 'context' has been configured to assert 'clean session', then the references to all the stored and unacknowledged PUBLISH messages are dropped at the time of MQTT disconnection (or network disconnection). Otherwise, these unacknowledged packets continue to be availalbe for the next iteration of the MQTT connection. However, if the client application asserts the 'clean session' parameter in the next iteration of the CONNECT operation, then references to all the stored PUBLISH messages, if any, are dropped.
- Parameters:
-
[in] ctx handle to the underlying network context in the LIB
- See also:
- mqtt_client_ctx_create
- Parameters:
-
[in] topic UTF8 based Topic Name for which data is being published. [in] data_buf The binary data that is being published for the topic. [in] data_len The length of the binary data. [in] qos quality of service of the message [in] retain should the server retain the message.
- Returns:
- on success, a transaction message id otherwise, LIB defined errors (LIBRARY Generated Error Codes)
i32 mqtt_client_run | ( | u32 | wait_secs | ) |
Run the LIB for the specified wait time. This service is valid only for the set-up of grouped contexts, where the application has populated the callbacks that can be invoked by the LIB.
This routine yields the control back to the application after the specified duration of wait time. Such an arrangement enable the application to make overall progress to meet it intended functionality.
The wait time implies the maximum intermediate duration between the reception of two successive messages from the server. If no message is received before the expiry of the wait time, the routine returns. However, the routine would continue to block, in case, messages are being received within the successive period of wait time.
- Parameters:
-
[in] wait_secs maximum time to wait for a message from the server
- Returns:
- on connection close by client app, number of bytes received for the last msg from broker, otherwise LIB defined error values.
- Note:
- if the value of MQP_ERR_LIBQUIT is returned, then system must be restarted.
i32 mqtt_client_send_progress | ( | void * | ctx | ) |
Send remaining data or contents of the scheduled message to the server. This routine tries to send the remaining data in an active transfer of a message to the server. This service is valid, only if the network layer of the platform is not able to send out the entire message in one TCP packet and has to "back-off" or "give up the control" before it can schedule or dispatch the next packet. In such a scenario, the network layer sends the first part (segment) of the scheduled message in the mqtt_xxx_send() API and the subsequent parts or the segments are sent using this routine.
This routine is not applicable to the platforms or the scenarios, where the implementation of the platform can segment the MQTT message in a manner to schedule consercutive or back-to-back blocking socket transactions. Specifically, this API must be used by an application, only if the network layer can indicate in an asynchronous manner, its readiness to send the next packet to the server. And the mechanism to indicate readiness of the network layer for the next send transaction is out of band and out of scope for the Client LIB and depends on the platform.
A platform that resorts to partial send of a message and has to back-off from transmission implies the following the considerations on to the application. (a) The next segment / part of the currently active MQTT packet can be sent or scheduled only after receiving the indication from the network layer to do so. (b) The next or new MQTT message (or its first segment) can be scheduled for transmission only after receiving the indication for completion of handling of the last segment of currently active message.
- Note:
- The application developer should refer to the platform specific network implementation for details.
The routine returns the number of remaining bytes in the message to be sent. However, as described earlier, the application is expected to wait for an indication about the readiness of the network layer prior to sending or scheduling another segment, if so available, to the server. Now, the new segment can be a next part of the currently active message or it can be the first segment of a new message. A return value of zero means that there is no more data left in the scheduled message to be sent to the server and the application should wait for an appropriate event to indicate the transmission of the last segment.
In case of an error, the transfer of the remaining segments or parts of the scheduled message is aborted. Depending on the configuration of the 'clean session' in-conjunction with the revision of the MQTT protocol, the active message would be stored for re-transmission, MQTT connection is established again. To store a message for re-transmission, at least one segment of the message must have been successfully sent to the server.
- Note:
- This API must be used by the application only if the platform has the capability to indicate the completion of the sending of an active segment.
- Parameters:
-
[in] ctx handle to the underlying network context in the LIB
- See also:
- mqtt_client_ctx_create
- Returns:
- the number of bytes remaining to be sent in the message. Otherwise, LIB defined errors (LIBRARY Generated Error Codes)
i32 mqtt_connect_msg_send | ( | void * | ctx, |
bool | clean_session, | ||
u16 | ka_secs | ||
) |
Send the CONNECT message to the server (and don't wait for CONNACK). This routine accomplishes multiple sequences. As a first step, it tries to establish a network connection with the server. Then, it populates an internaly allocated packet buffer with all the previously provided payload data information, prepares the requisite headers and finally, dispatches the constructed message to the server.
Prior to invoking this service, the client application should provision the intended payload contents of the CONNECT message by using the API(s) mqtt_client_ctx_info_register and mqtt_client_ctx_will_register. And information about the server of interest must be provided in the client LIB 'context' creation (mqtt_client_ctx_create).
The client application must invoke an appropriate receive routine to know about the corresponding response CONNACK from the server. The client LIB will close the network connection to the server, if the server happens to refuse the CONNECT request.
- Parameters:
-
[in] ctx handle to the underlying network context in the LIB
- See also:
- mqtt_client_ctx_create
- Parameters:
-
[in] clean_session asserted to delete references to previous session at both server and client [in] ka_secs Keep Alive Time
- Returns:
- number of bytes sent or LIB defined errors (LIBRARY Generated Error Codes)
i32 mqtt_disconn_send | ( | void * | ctx | ) |
Send a DISCONNECT message to the server.
- Parameters:
-
[in] ctx handle to the underlying network context in the LIB
- See also:
- mqtt_client_ctx_create
- Returns:
- number of bytes sent or Lib define errors (LIBRARY Generated Error Codes)
i32 mqtt_pingreq_send | ( | void * | ctx | ) |
Send a PINGREQ message to the server (and don't wait for PINGRSP).
- Parameters:
-
[in] ctx handle to the underlying network context in the LIB
- See also:
- mqtt_client_ctx_create
- Returns:
- number of bytes sent or Lib define errors (LIBRARY Generated Error Codes)
i32 mqtt_sub_dispatch | ( | void * | ctx, |
struct mqtt_packet * | mqp | ||
) |
Dispatch application constructed SUSBSCRIBE message to the server. Prior to sending the message to the server, this routine will prepare a fixed header to account for the size of the size of the contents.
After the packet has been dispatched to the server, the library will store the packet until the time, a corresponding SUB-ACK has been received from the server. This mechanism enables the client LIB 'context' to trace the sequence of the message-ID and / or resend the SUB packets to the server.
The client LIB 'context', if configured to operate in the MQTT 3.1.1 mode will drop or remove the un-acknowledged SUB messages at the time of the termination of the network connection.
In the MQTT 3.1 mode, the client LIB 'context' will remove the unacknowledged SUB messages at the time of the termination of the network connection, if the 'clean session' has been asserted. In case, the 'clean session' has not been asserted, the stored SUB messages will continue to be available for the next iteration of the MQTT connection. However, if the client application asserts the 'clean session' parameter in the next iteration of the CONNECT operation, then references to all the stored SUBSCRIBE messages, if any, are dropped.
The caller must populate the payload information of topic along with qos before invoking this service.
This service facilitates direct writing of topic and (real-time) payload data into the buffer, thereby, avoiding power consuming and wasteful intermediate data copies.
In case, the routine returns an error, the caller is responsbile for freeing up or re-using the packet buffer. For all other cases, the client library will manage the return of the packet buffer to the pool.
- Parameters:
-
[in] ctx handle to the underlying network context in the LIB
- See also:
- mqtt_client_ctx_create
- Parameters:
-
[in] mqp app created SUBSCRIBE message without the fixed header.
- Returns:
- on success, the transaction Message ID, otherwise Lib defined errors (LIBRARY Generated Error Codes)
i32 mqtt_sub_msg_send | ( | void * | ctx, |
const struct utf8_strqos * | qos_topics, | ||
u32 | count | ||
) |
Send a SUBSCRIBE message to the server (and don't wait for SUBACK). This routine creates a SUBSCRIBE message in an internally allocated packet buffer by embedding the 'qos_topics', then prepares the message header and finally, dispatches the packet to the server.
After the packet has been dispatched to the server, the library will store the packet until the time, a corresponding SUB-ACK has been received from the server. This mechanism enables the client LIB 'context' to trace the sequence of the message-ID and / or resend the SUB packets to the server.
The client LIB 'context', if configured to operate in the MQTT 3.1.1 mode will drop or remove the un-acknowledged SUB messages at the time of the termination of the network connection.
In the MQTT 3.1 mode, the client LIB 'context' will remove the unacknowledged SUB messages at the time of the termination of the network connection, if the 'clean session' has been asserted. In case, the 'clean session' has not been asserted, the stored SUB messages will continue to be available for the next iteration of the MQTT connection. However, if the client application asserts the 'clean session' parameter in the next iteration of the CONNECT operation, then references to all the stored SUBSCRIBE messages, if any, are dropped.
- Parameters:
-
[in] ctx handle to the underlying network context in the LIB
- See also:
- mqtt_client_ctx_create
- Parameters:
-
[in] qos_topics an array of topic along-with its qos [in] count the number of elements in the array
- Returns:
- on success, the transaction Message ID, otherwise Lib defined errors (LIBRARY Generated Error Codes)
i32 mqtt_unsub_dispatch | ( | void * | ctx, |
struct mqtt_packet * | mqp | ||
) |
Dispatch application constructed UNSUSBSCRIBE message to the server. Prior to sending the message to the server, this routine will prepare a fixed header to account for the size of the size of the contents.
After the packet has been dispatched to the server, the library will store the packet until the time, a corresponding UNSUB-ACK has been received from the server. This mechanism enables the client LIB 'context' to trace the sequence of the message-ID and / or resend the UNSUB packets to the server.
The client LIB 'context', if configured to operate in the MQTT 3.1.1 mode will drop or remove the un-acknowledged SUB messages at the time of the termination of the network connection.
In the MQTT 3.1 mode, the client LIB 'context' will remove the unacknowledged UNSUB messages at the time of the termination of the network connection, if the 'clean session' has been asserted. In case, the 'clean session' has not been asserted, the stored UNSUB messages will continue to be available for the next iteration of the MQTT connection. However, if the client application asserts the 'clean session' parameter in the next iteration of the CONNECT operation, then references to all the stored UNSUBSCRIBE messages, if any, are dropped.
The caller must populate the payload information of topics before invoking this service.
This service facilitates direct writing of topic and (real-time) payload data into the buffer, thereby, avoiding power consuming and wasteful intermediate data copies.
In case, the routine returns an error, the caller is responsbile for freeing up or re-using the packet buffer. For all other cases, the client library will manage the return of the packet buffer to the pool.
- Parameters:
-
[in] ctx handle to the underlying network context in the LIB
- See also:
- mqtt_client_ctx_create
- Parameters:
-
[in] Packet Buffer that holds UNSUBSCRIBE message without a fixed header
- Returns:
- on success, the transaction Message ID, otherwise LIB defined errors (LIBRARY Generated Error Codes)
i32 mqtt_unsub_msg_send | ( | void * | ctx, |
const struct utf8_string * | topics, | ||
u32 | count | ||
) |
Send an UNSUBSCRIBE message to the server (and don't wait for UNSUBACK). This routine creates an UNSUBSCRIBE message in an internally allocated packet buffer by embedding the 'topics', then prepares the message header and finally, dispatches the packet to the server.
After the packet has been dispatched to the server, the library will store the packet until the time, a corresponding UNSUB-ACK has been received from the server. This mechanism enables the client LIB 'context' to trace the sequence of the message-ID and / or resend the UNSUB packets to the server.
The client LIB 'context', if configured to operate in the MQTT 3.1.1 mode will drop or remove the un-acknowledged SUB messages at the time of the termination of the network connection.
In the MQTT 3.1 mode, the client LIB 'context' will remove the unacknowledged UNSUB messages at the time of the termination of the network connection, if the 'clean session' has been asserted. In case, the 'clean session' has not been asserted, the stored UNSUB messages will continue to be available for the next iteration of the MQTT connection. However, if the client application asserts the 'clean session' parameter in the next iteration of the CONNECT operation, then references to all the stored UNSUBSCRIBE messages, if any, are dropped.
- Parameters:
-
[in] ctx handle to the underlying network context in the LIB
- See also:
- mqtt_client_ctx_create
- Parameters:
-
[in] topics an array of topic to unsubscribe [in] count the number of elements in the array
- Returns:
- on success, the transaction Message ID, otherwise Lib defined errors (LIBRARY Generated Error Codes)
Generated on Mon Nov 17 2014 12:11:04 for MQTT Client by
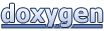