![]() |
MQTT Server 1.0.0
|
#include <server_pkts.h>
Public Attributes | |
u16(* | connect_rx )(void *ctx_cl, u8 conn_flags, struct utf8_string *const *utf8_vec, void **usr) |
bool(* | sub_msg_rx )(void *usr_cl, const struct utf8_strqos *qos_topics, u32 n_topics, u16 msg_id, u8 *acks) |
bool(* | un_sub_msg )(void *usr_cl, const struct utf8_string *topics, u32 n_topics, u16 msg_id) |
bool(* | pub_msg_rx )(void *usr_cl, const struct utf8_string *topic, const u8 *data_buf, u32 data_len, u16 msg_id, bool dup, enum mqtt_qos qos, bool retain) |
bool(* | ack_notify )(void *usr_cl, u8 msg_type, u16 msg_id) |
void(* | on_cl_net_close )(void *usr_cl, bool due2err) |
void(* | on_connack_send )(void *usr_cl, bool clean_session) |
Detailed Description
Working Principle for implementing the call-back services: Implementation of the call-back services should report in return value, only about errors found in the RX processing. Specifically, the status of TX as a follow-up to RX message (represented as a call-back service) need not be reported to the server packet library.
Error observed in TX (supported by appropriate API(s) / services in the service packet library) is recorded in the 'context' and shall be dealt in the next iteration of RX loop.
Member Data Documentation
bool(* mqtt_server_msg_cbs::ack_notify)(void *usr_cl, u8 msg_type, u16 msg_id) |
Notify the acknowledgement that was received from the remote client. Following are the messages that are notified by the server LIB.
PUBACK, PUBREC, PUBREL, PUBCOMP
On return from this routine, if the application has found problem in processing the ACK message, then the LIB will simply terminate the associated network connection
- Parameters:
-
[in] usr_cl handle to connection context in the application [in] msg_type refers to the type of ACK message [in] msg_id the associated message ID provided by the client
- Returns:
- application should return false if the ACK was not expected by it or no reference was found for it. Otherwise true.
u16(* mqtt_server_msg_cbs::connect_rx)(void *ctx_cl, u8 conn_flags, struct utf8_string *const *utf8_vec, void **usr) |
Indicate the CONNECT Message from the client This routine provides, to the application, information about the connection request that a remote client has made. The application should utilize the credential and other data presented by the LIB to authenticate, analyze and finally, approve or deny the request.
Application at its discretion can also imply deployment specific policies to make decision about allowing or refusing the request.
The return value of this routine is a 16bit value that commensurates with the 'variable header' of the CONNACK message. Specifically, the LSB of the 16bit return value corresponds to the 8bit 'return code' parameter in the CONNACK message and the MSB to the 'acknowledge flags'. The application must set a valid return value.
The LIB uses the return value to compose the CONNACK message to the remote client. If the LSB of return value is a 'non zero' value, then the LIB, after sending the CONNACK message to the remote client, will terminate the network connection.
- Parameters:
-
[in] ctx_cl handle to the underlying network context in the LIB [in] conn_flags options received in CONNECT message from server [in] utf8_vec vector / array of pointers to UTF8 information. The order of UTF8 information is client-id, will topic, will-message, user-name and pass-word. A NULL in vector element indicates absence of that particular UTF8 information. [out] usr place holder for application to provide connection specific handle. In subsequent calls from the implementation this handle will be passed as a parameter to enable application to refer to the particular active connection.
- Returns:
- 16bit value for the variable header of the CONNACK message
void(* mqtt_server_msg_cbs::on_cl_net_close)(void *usr_cl, bool due2err) |
Notify that network connection to client has been closed. This routine is invoked by the LIB to declare to the application that the network connection to a particular client has been terminated and follow-up, if needed, can now commence. If configured, removal of the client session and / or dispatch of the WILL message, will be typical aspects, among others, to follow-up. The routine aids the application by indicating if an error condition had caused the closure.
This routine is invoked by the LIB irrespective of the source entity, server or client, that has caused the closure of the network.
- Parameters:
-
[in] usr_cl handle to connection context in the application [in] due2err has the connection been closed due to an error?
void(* mqtt_server_msg_cbs::on_connack_send)(void *usr_cl, bool clean_session) |
Notify that CONNACK has been sent to the specified client. This routine is invoked by the LIB to enable the application to make progress and follow-up on the session information for the particular client. Specifically, this routine facilitates the application to either delete the session or re-send / sync-up the pending messages associated with the client. The follow-up action is depenedent upon the 'clean_session' option in the CONNECT message from the client.
- Parameters:
-
[in] usr_cl handle to connection context in the application [in] clean_session was a clean session requested in CONNECT?
bool(* mqtt_server_msg_cbs::pub_msg_rx)(void *usr_cl, const struct utf8_string *topic, const u8 *data_buf, u32 data_len, u16 msg_id, bool dup, enum mqtt_qos qos, bool retain) |
Indicate the PUBLISH Message from the client. This routine provides, to the application, the binary data along-with its qualifiers and the topic to which a remote client has published data.
On return from this routine, if the application has found a problem in processing of the contents of the PUBLISH message, the LIB will simply terminate the associated network connection. Otherwise, depending upon the QoS level of the PUBLISH message, the LIB shall dispatch the ACK (PUBACK or PUBREC) to the client, thereby, relieveing the application from this support.
- Parameters:
-
[in] usr_cl handle to connection context in the application [in] topic UTF8 Topic Name for which data has been published [in] data_buf the published binary data for the topic [in] data_len the length of the binary data [in] msg_id the associated message ID provided by the client [in] dup has client indicated this as a duplicate message [in] qos quality of service of the message [in] retain should the server retain the data?
- Returns:
- The application should return false, if it encounters any problem in the processing of data, topic and related resources. Otherwise, true.
bool(* mqtt_server_msg_cbs::sub_msg_rx)(void *usr_cl, const struct utf8_strqos *qos_topics, u32 n_topics, u16 msg_id, u8 *acks) |
Indicate the SUBSCRIBE Message from the client This routine provides, to the application, information about the topics that the remote client intends to subscribe to.
On return from this routine, if the application has found a problem in the processing of message, then the LIB will simply terminate the associated network connection.
- Parameters:
-
[in] usr_cl handle to connection context in the application [in] qos_topics an array of topic along-with its qos [in] n_topics the count / number of elements in the array [in] msg_id the associated message ID provided by the client [in] acks place holder array for the application to provide finalized qos for each of the subscribed topic. The order of ack is same as that of qos_topics
- Returns:
- The application should return false, if it encounters any problem in the processing of topic. Otherwise, true.
- Note:
- The memory space pointed by the 'buffer' field in the elements of 'qos_topics' array has an additional byte available beyond the size of memory indicated by the 'length' field. This extra byte can be used by the application to NUL terminate the buffer. This quirk is applicable to this routine only.
bool(* mqtt_server_msg_cbs::un_sub_msg)(void *usr_cl, const struct utf8_string *topics, u32 n_topics, u16 msg_id) |
Indicate the UNSUBSCRIBE Message from the client This routine provides, to the application, information about the topics that the remote client intends to unsubscribe.
On return from this routine, if the application has found a problem in the processing of message, then the LIB will simply terminate the associated network connection.
- Parameters:
-
[in] usr_cl handle to connection context in the application [in] topics an array of topic in the message [in] n_topics the count / number of elements in the array [in] msg_id the associated message ID provided by the client
- Returns:
- The application should return false, if it encounters any problem in the processing of topic. Otherwise, true.
The documentation for this struct was generated from the following file:
- D:/my_data/GIT/network_apps/netapps/mqtt/server/server_pkts.h
Generated on Mon Nov 17 2014 12:12:08 for MQTT Server by
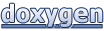