![]() |
MQTT Server 1.0.0
|
#include <stdbool.h>
#include <stdlib.h>
#include <stdio.h>
Go to the source code of this file.
Classes | |
struct | mqtt_packet |
struct | utf8_string |
struct | mqtt_ack_wlist |
struct | utf8_strqos |
struct | secure_conn |
struct | device_net_services |
struct | pub_qos2_cq |
struct | client_ctx |
Defines | |
#define | MQTT_COMMON_VERSTR "1.0.0" |
#define | MIN(a, b) ((a > b)? b : a) |
#define | MQTT_CONNECT 0x01 |
#define | MQTT_CONNACK 0x02 |
#define | MQTT_PUBLISH 0x03 |
#define | MQTT_PUBACK 0x04 |
#define | MQTT_PUBREC 0x05 |
#define | MQTT_PUBREL 0x06 |
#define | MQTT_PUBCOMP 0x07 |
#define | MQTT_SUBSCRIBE 0x08 |
#define | MQTT_SUBACK 0x09 |
#define | MQTT_UNSUBSCRIBE 0x0A |
#define | MQTT_UNSUBACK 0x0B |
#define | MQTT_PINGREQ 0x0C |
#define | MQTT_PINGRSP 0x0D |
#define | MQTT_DISCONNECT 0x0E |
#define | MAX_FH_LEN 0x05 |
#define | MAX_REMLEN_BYTES (MAX_FH_LEN - 1) |
#define | MAKE_FH_BYTE1(msg_type,flags) (u8)((msg_type << 4) | flags) |
#define | MAKE_FH_FLAGS(bool_dup, enum_qos, bool_retain) (u8)(((bool_dup << 3) | (enum_qos << 1) | bool_retain) & 0xF) |
#define | QOS_VALUE(enum_qos) (u8)(enum_qos & 0x3) |
#define | QFL_VALUE 0x80 |
#define | DUP_FLAG_VAL(bool_val) (u8)(bool_val << 3) |
#define | BOOL_RETAIN(fh_byte1) ((fh_byte1 & 0x1)? true : false) |
#define | BOOL_DUP(fh_byte1) ((fh_byte1 & 0x8)? true : false) |
#define | ENUM_QOS(fh_byte1) (enum mqtt_qos)((fh_byte1 & 0x6) >> 1) |
#define | MSG_TYPE(fh_byte1) (u8)((fh_byte1 & 0xf0) >> 4) |
#define | MQP_FHEADER_BUF(mqp) (mqp->buffer + mqp->offset) |
#define | MQP_VHEADER_BUF(mqp) (MQP_FHEADER_BUF(mqp) + mqp->fh_len) |
#define | MQP_PAYLOAD_BUF(mqp) (MQP_VHEADER_BUF(mqp) + mqp->vh_len) |
#define | MQP_CONTENT_LEN(mqp) (mqp->fh_len + mqp->vh_len + mqp->pl_len) |
#define | MQP_FREEBUF_LEN(mqp) |
#define | MQP_FHEADER_VAL(mqp) (mqp->fh_byte1) |
#define | MQP_FHEADER_MSG(mqp) (MSG_TYPE(MQP_FHEADER_VAL(mqp))) |
#define | MQP_FHEADER_FLG(mqp) (MSG_FLAGS(MQP_FHEADER_VAL(mqp))) |
#define | DEFINE_MQP_VEC(num_mqp, mqp_vec) static struct mqtt_packet mqp_vec[num_mqp]; |
#define | DEFINE_MQP_BUF_VEC(num_mqp, mqp_vec, buf_len, buf_vec) |
#define | MQP_PUB_TOP_BUF(mqp) (MQP_VHEADER_BUF(mqp) + 2) |
#define | MQP_PUB_TOP_LEN(mqp) (mqp->vh_len - 2 - (mqp->msg_id? 2 : 0)) |
#define | MQP_PUB_PAY_BUF(mqp) (mqp->pl_len? MQP_PAYLOAD_BUF(mqp) : NULL) |
#define | MQP_PUB_PAY_LEN(mqp) (mqp->pl_len) |
#define | MQP_ERR_NETWORK (-1) |
#define | MQP_ERR_TIMEOUT (-2) |
#define | MQP_ERR_NET_OPS (-3) |
#define | MQP_ERR_FNPARAM (-4) |
#define | MQP_ERR_PKT_AVL (-5) |
#define | MQP_ERR_PKT_LEN (-6) |
#define | MQP_ERR_NOTCONN (-7) |
#define | MQP_ERR_BADCALL (-8) |
#define | MQP_ERR_CONTENT (-9) |
#define | MQP_ERR_LIBQUIT (-10) |
#define | MQP_ERR_NOT_DEF (-32) |
#define | DEV_NETCONN_OPT_TCP 0x01 |
#define | DEV_NETCONN_OPT_UDP 0x02 |
#define | DEV_NETCONN_OPT_IP6 0x04 |
#define | DEV_NETCONN_OPT_URL 0x08 |
#define | DEV_NETCONN_OPT_SEC 0x10 |
#define | MAX_PUBREL_INFLT 8 |
#define | KA_TIMEOUT_NONE 0xffffffff |
Typedefs | |
typedef int | i32 |
typedef unsigned int | u32 |
typedef unsigned char | u8 |
typedef char | i8 |
typedef unsigned short | u16 |
typedef short | i16 |
Enumerations | |
enum | mqtt_qos { MQTT_QOS0, MQTT_QOS1, MQTT_QOS2 } |
Functions | |
void | mqp_free (struct mqtt_packet *mqp) |
void | mqp_reset (struct mqtt_packet *mqp) |
void | mqp_init (struct mqtt_packet *mqp, u8 offset) |
i32 | mqp_buf_wr_utf8 (u8 *buf, const struct utf8_string *utf8) |
i32 | mqp_buf_tail_wr_remlen (u8 *buf, u32 remlen) |
i32 | mqp_buf_rd_remlen (u8 *buf, u32 *remlen) |
i32 | mqp_pub_append_topic (struct mqtt_packet *mqp, const struct utf8_string *topic, u16 msg_id) |
i32 | mqp_pub_append_data (struct mqtt_packet *mqp, const u8 *data_buf, u32 data_len) |
bool | mqp_proc_msg_id_ack_rx (struct mqtt_packet *mqp_raw, bool has_payload) |
bool | mqp_proc_pub_rx (struct mqtt_packet *mqp_raw) |
bool | mqp_ack_wlist_append (struct mqtt_ack_wlist *list, struct mqtt_packet *elem) |
struct mqtt_packet * | mqp_ack_wlist_remove (struct mqtt_ack_wlist *list, u16 msg_id) |
void | mqp_ack_wlist_purge (struct mqtt_ack_wlist *list) |
i32 | mqp_prep_fh (struct mqtt_packet *mqp, u8 flags) |
i32 | mqp_recv (i32 net, const struct device_net_services *net_ops, struct mqtt_packet *mqp, u32 wait_secs, bool *timed_out, void *ctx) |
void | qos2_pub_cq_reset (struct pub_qos2_cq *cq) |
bool | qos2_pub_cq_logup (struct pub_qos2_cq *cq, u16 msg_id) |
bool | qos2_pub_cq_unlog (struct pub_qos2_cq *cq, u16 msg_id) |
bool | qos2_pub_cq_check (struct pub_qos2_cq *cq, u16 msg_id) |
void | cl_ctx_reset (struct client_ctx *cl_ctx) |
void | cl_ctx_timeout_insert (struct client_ctx **head, struct client_ctx *elem) |
void | cl_ctx_remove (struct client_ctx **head, struct client_ctx *elem) |
void | cl_ctx_timeout_update (struct client_ctx *cl_ctx, u32 now_secs) |
Detailed Description
This file incorporates constructs that are common to both client and server implementation.
The applications are not expected to utlize the routines made available in this module module.
- Note:
- the routines in this module do not check for availability and correctness of the input parameters
- Warning:
- The module is expected to under-go changes whilst incorporating support for the server. Therefore, it is suggested that applications do not rely on the services provided in this module.
Define Documentation
#define DEFINE_MQP_BUF_VEC | ( | num_mqp, | |
mqp_vec, | |||
buf_len, | |||
buf_vec | |||
) |
DEFINE_MQP_VEC(num_mqp, mqp_vec); \
static u8 buf_vec[num_mqp][buf_len];
#define MAX_FH_LEN 0x05 |
MAX Length of Fixed Header
#define MAX_REMLEN_BYTES (MAX_FH_LEN - 1) |
Max number of bytes in remaining length field
#define MQP_FREEBUF_LEN | ( | mqp | ) |
(mqp->maxlen - mqp->offset - \ MQP_CONTENT_LEN(mqp))
#define MQTT_COMMON_VERSTR "1.0.0" |
Version of Common LIB
#define MQTT_CONNECT 0x01 |
MQTT Message Types
#define QFL_VALUE 0x80 |
QOS Failure value (SUBACK)
Enumeration Type Documentation
enum mqtt_qos |
Function Documentation
i32 mqp_buf_rd_remlen | ( | u8 * | buf, |
u32 * | remlen | ||
) |
Read MQTT construct 'Remaining Length' from leading bytes of the buffer. The 'remaining length' is written in the format as outlined in the MQTT specification.
- Parameters:
-
[in] buf refers to memory to head-read 'Remaining Length' from [in] remlen place-holder for The 'Remaining Length' value
- Returns:
- in success, number of header bytes read, otherwise -1 on error
i32 mqp_buf_tail_wr_remlen | ( | u8 * | buf, |
u32 | remlen | ||
) |
Write the MQTT construct 'Remaining Length' into trailing end of buffer. The 'remaining length' is written in the format as outlined in the MQTT specification.
The implementation assumes availability of at-least 4 bytes in the buffer. Depending on the value of 'Remaining Length' appropriate trailing bytes in the buffer would be used.
- Parameters:
-
[in] buf refers to memory to tail-write 'Remaining Length' into [in] remlen The 'Remaining Length' value
- Returns:
- in success, number of trailing bytes used, otherwise -1 on error
i32 mqp_buf_wr_utf8 | ( | u8 * | buf, |
const struct utf8_string * | utf8 | ||
) |
Write UTF8 information into the buffer. The UTF8 information includes content and its length.
- Warning:
- The routine does not check for correctness of the paramters.
- Parameters:
-
[in] buf refers to memory to write UTF8 information into [in] utf8 contains UTF8 information to be written
- Returns:
- on success, number of bytes written, otherwise -1 on error.
void mqp_free | ( | struct mqtt_packet * | mqp | ) |
Free a MQTT Packet Buffer Puts back the packet buffer in to the appropriate pool.
- Parameters:
-
[in] mqp packet buffer to be freed
- Returns:
- none
void mqp_init | ( | struct mqtt_packet * | mqp, |
u8 | offset | ||
) |
Initializes attributes of the MQTT Packet Holder. This routine sets number of users of the MQTT Packet Holder to 1. However, it leaves, if already provisioned, the reference to buffer and its size un-altered.
- Parameters:
-
[in] mqp packet buffer to be initialized [in] offset index in buffer to indicate start of the contents
- Returns:
- none
i32 mqp_prep_fh | ( | struct mqtt_packet * | mqp, |
u8 | flags | ||
) |
Prepare the Fixed-Header of the MQTT Packet (before being sent to network) Based on the contents of the mqtt packet and the combination of DUP, QoS and Retain flags as outlined the MQTT specification, the routine updates, among others, significant internal fields such as 'remaining length' and 'fixed header length' in the packet construct and embeds the fixed header, so created, in the packet buffer.
This service must be utilized on a packet that has been already populated with all the payload data, topics and other contents. The fixed header must be the final step in the compostion of MQTT packet prior to its dispatch to the server.
Returns size, in bytes, of the fixed-header, otherwise -1 on error.
bool mqp_proc_msg_id_ack_rx | ( | struct mqtt_packet * | mqp_raw, |
bool | has_payload | ||
) |
Construct a packet for Message ID enabled ACK received from network Process the raw ACK message information to update the packet holder.
- Warning:
- This routine does not check for correctness of the input parameters.
- Parameters:
-
[in] mqp_raw holds a raw buffer from the network [in] has_payload asserted, if ACK message should have a payload
- Returns:
- on success, true, otherwise false
bool mqp_proc_pub_rx | ( | struct mqtt_packet * | mqp_raw | ) |
Construct a packet for PUBLISH message received from the network Process the raw PUB message information to update the packet holder.
- Warning:
- This routine does not check for correctness of the input parameters.
- Parameters:
-
[in] mqp_raw holds a raw buffer from the network
- Returns:
- on success, true, other wise false
i32 mqp_pub_append_data | ( | struct mqtt_packet * | mqp, |
const u8 * | data_buf, | ||
u32 | data_len | ||
) |
Include payload data for publishing The payload data is associated with a topic.
- Warning:
- This routine does not check for correctness of the input parameters.
- Parameters:
-
[in] mqp packet buffer in which payload data must be included. [in] data_buf data to be included in the packet buffer [in] data_len length of the data to be included in the packet.
- Returns:
- on success, number of bytes appended, otherwise -1 on error.
- Note:
- A 'topic' must be appended prior to inclusion of pulished data.
i32 mqp_pub_append_topic | ( | struct mqtt_packet * | mqp, |
const struct utf8_string * | topic, | ||
u16 | msg_id | ||
) |
Include variable header Topic as part of PUB Message construction. Inclusion of a Topic also encompasses incorporation of the message ID.
The topic refers to the subject for which data will be published by the client or the server. The topic entity must be appended into the packet buffer prior to the inclusion of the payload (data).
- Warning:
- This routine does not check for correctness of the input parameters.
- Parameters:
-
[in] mqp packet buffer in which topic must be included. [in] topic UTF8 information [in] msg_id Message or Packet transaction ID
- Returns:
- on success, number of bytes appended, otherwise -1 on error.
- Note:
- A 'topic' must be appended prior to inclusion of pulished data.
void mqp_reset | ( | struct mqtt_packet * | mqp | ) |
Resets the attributes of MQTT Packet Holder to its init state Not all fields are reset - entities such as offset, n_refs in addition to buffer information are not updated.
- Parameters:
-
[in] mqp packet buffer to be reset
- Returns:
- none
- See also:
- mqp_init
Generated on Mon Nov 17 2014 12:12:08 for MQTT Server by
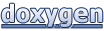