![]() |
MQTT Server 1.0.0
|
Public Attributes | |
i32(* | open )(u32 nwconn_opts, const i8 *server_addr, u16 port_number, const struct secure_conn *nw_security) |
i32(* | send )(i32 comm, const u8 *buf, u32 len, void *ctx) |
i32(* | recv )(i32 comm, u8 *buf, u32 len, u32 wait_secs, bool *err_timeo, void *ctx) |
i32(* | send_dest )(i32 comm, const u8 *buf, u32 len, u16 dest_port, const i8 *dest_ip, u32 ip_len) |
i32(* | recv_from )(i32 comm, u8 *buf, u32 len, u16 *from_port, i8 *from_ip, u32 *ip_len) |
i32(* | close )(i32 comm) |
i32(* | listen )(u32 nwconn_opts, u16 port_number, const struct secure_conn *nw_security) |
i32(* | accept )(i32 listen, i8 *client_ip, u32 *ip_length) |
i32(* | io_mon )(i32 *recv_cvec, i32 *send_cvec, i32 *rsvd_cvec, u32 wait_secs) |
u32(* | time )(void) |
Member Data Documentation
i32(* device_net_services::accept)(i32 listen, i8 *client_ip, u32 *ip_length) |
Accept an incominng connection. This routine creates a new communication channel for the (remote) requesting client.
- Parameters:
-
[in] listen handle to listen for the incoming connection requests from the remote clients [out] client_ip IP address of the connected client. This value is valid only on successful return of the routine. The place holder must provide memory to store atleast 16 bytes. [in,out] ip_length Length of IP address. It is provided by the caller to declare the length of the place holder and updated by routine to indicate the length of the connected client's IP address.
- Returns:
- on success, a valid handle to the new connection, otherwise NULL
i32(* device_net_services::close)(i32 comm) |
Close communication connection
i32(* device_net_services::io_mon)(i32 *recv_cvec, i32 *send_cvec, i32 *rsvd_cvec, u32 wait_secs) |
Monitor activity on communication handles. The routine blocks for the specified period of time to monitor activity, if any, on each of the communication handle that has been provided in one or more vector sets. At the expiry of the wait time, this function must identify the handles, on which, acitvities were observed.
A particular collection of communication handles are packed as an array or in a vector and is passed to the routine. A NULL handle in the vector indicates the termination of the vector and can effectively used to account for the size of the vector.
Similarly, at end the end of the wait period, the routine must provide a vector of the handles for which activity was observed.
- Parameters:
-
[in,out] recv_hvec a vector of handles which must be monitored for receive activities. [in,out] send_hvec a vector of handles which must be monitored for send activities. [in,out] rsvd_hvec reserved for future use. [in] wait_secs time to wait and monitor activity on communication handles provided in one or more sets. If set to 0, the routine returns immediately.
- Returns:
- on success, the total number of handles for which activity was observed. This number can be 0, if no activity was observed on any of the provided handle in the specified time. Otherwise, -1 on error.
i32(* device_net_services::listen)(u32 nwconn_opts, u16 port_number, const struct secure_conn *nw_security) |
Listen to incoming connection from clients. This routine prepares the system to listen on the specified port for the incoming network connections from the remote clients.
- Parameters:
-
[in] nwconn_opts Implementation specific construct to enumerate server address and / or connection related details [in] port_number Network port number, typically, 1883 or 8883 [in] nw_security Information to establish a secure connection with client. Set it to NULL, if not used. Information to establish a secure connection.
- Returns:
- a valid handle to listening contruct, otherwise NULL
i32(* device_net_services::open)(u32 nwconn_opts, const i8 *server_addr, u16 port_number, const struct secure_conn *nw_security) |
Set up a communication channel with a server or set up a local port. This routine opens up either a "connection oriented" communication channel with the specified server or set up a local configuration for "connectionless" transactions.
- Parameters:
-
[in] nwconn_opts Implementation specific construct to enumerate server address and / or connection related details [in] server_addr URL or IP address (string) or other server reference. For setting up a local (UDP) port, set it to NULL. [in] port_number Network port number, typically, 1883 or 8883 for remote severs. For setting up a local (UDP) port, use an intended port number. [in] nw_security Information to establish a secure connection with server. Set it to NULL, if not used. Information to establish a secure connection.
- Returns:
- a valid handle to connection, otherwise NULL
i32(* device_net_services::recv)(i32 comm, u8 *buf, u32 len, u32 wait_secs, bool *err_timeo, void *ctx) |
Receive data from the "connection oriented" channel. The routine blocks till the time, there is either a data that has been received from the server or the time to await data from the server has expired.
- Parameters:
-
[in] comm Handle to network connection as returned by accept(). [out] buf place-holder to which data from network should be written into. [in] len maximum length of 'buf' [in] wait_secs maximum time to await data from network. If exceeded, the routine returns error with the err_timeo flag set as true. [out] err_timeo if set, indicates that error is due to timeout. [in] ctx reference to the MQTT connection context
- Returns:
- on success, number of bytes received, 0 on connection reset, otherwise -1 on error. In case, error (-1) is due to the time-out, then the implementation should set flag err_timeo as true.
i32(* device_net_services::recv_from)(i32 comm, u8 *buf, u32 len, u16 *from_port, i8 *from_ip, u32 *ip_len) |
Receive data on a local port sent by any network element. The routine blocks till the time, data has been received on the local port from any remote network element.
- Parameters:
-
[in] comm handle to network connection as return by open(). [in] buf place-holder to which data from network should be written into. [in] len maximum lengh of 'buf' [out] from_port place-holder for the port of the sender network entity [out] from_ip place-holder to retrieve the IP address of the sender network entity. The memory space must be provisioned to store atleast 16 bytes. [in,out] ip_len length of IP address. It is provided by the caller to declare the length of the place holder and updated by routine to indicate the length of the remote network entity's IP address.
- Returns:
- on success, number of bytes received, 0 on connection reset, otherwise -1 on errir.
i32(* device_net_services::send)(i32 comm, const u8 *buf, u32 len, void *ctx) |
Send data onto the "connection oriented" network. The routine blocks till the time, the data has been copied into the network stack for dispatch on to the "connection oriented" network.
- Parameters:
-
[in] comm handle to network connection as returned by open(). [in] buf refers to the data that is intended to be sent [in] len length of the data [in] ctx reference to the MQTT connection context
- Returns:
- on success, the number of bytes sent, 0 on connection reset, otherwise -1
i32(* device_net_services::send_dest)(i32 comm, const u8 *buf, u32 len, u16 dest_port, const i8 *dest_ip, u32 ip_len) |
Send data to particular port on the specified network element. The routine blocks till the time, the data has been copied into the network stack for dispatch to the "specified" network entity.
- Parameters:
-
[in] comm handle to network connection as returned by open(). [in] buf refers to data that is intended to be sent [in] len length of the data [in] dest_port network port to which data is to be sent. [in] dest_ip IP address of the entity to which data is to be sent. [in] ip_len length of the destination IP address.
- Returns:
- on success, the number of bytes sent, 0 on connection reset, otherwise -1.
u32(* device_net_services::time)(void) |
Get Time (in seconds). Provides a monotonically incrementing value of a time service in unit of seconds. The implementation should ensure that associated timer hardware or the clock module remains active through the low power states of the system. Such an arrangement ensures that MQTT Library is able to track the Keep-Alive time across the cycles of low power states. It would be typical of battery operated systems to transition to low power states during the period of inactivity or otherwise to conserve battery.
In the absence of a sustained time reference across the low power states, if the system transitions away from the active state, the MQTT Library, then may not be able to effectively monitor the Keep Alive duration.
It is the responsbililty of the implementation to manage the roll- over problem of the hardware and ensure the integrity of the time value is maintained.
- Returns:
- time in seconds
The documentation for this struct was generated from the following file:
- D:/my_data/GIT/network_apps/netapps/mqtt/common/mqtt_common.h
Generated on Mon Nov 17 2014 12:12:08 for MQTT Server by
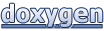