MotionFX Software Library
MotionFX Software Library Documentation
|
motion_fx.h
Go to the documentation of this file.
90 unsigned char modx; /* setting to indicate the decimation, set to 1 in smartphone/tablet, set to >=1 in embedded solutions */
219 void MotionFX_update(MFX_output_t *data_out, MFX_input_t *data_in, float eml_deltatime, float *eml_q_update);
void MotionFX_propagate(MFX_output_t *data_out, MFX_input_t *data_in, float eml_deltatime)
Run the Kalman filter propagate.
void MotionFX_MagCal_init(int sampletime, unsigned short int enable)
Initialize the compass calibration library.
void MotionFX_MagCal_getParams(MFX_MagCal_output_t *data_out)
Get magnetic calibration parameters.
MFX_engine_state_t MotionFX_getStatus_6X(void)
Get the status of the 6 axes library.
Definition: motion_fx.h:132
Definition: motion_fx.h:123
Definition: motion_fx.h:68
void MotionFX_enable_6X(MFX_engine_state_t enable)
Enable or disable the 6 axes function (ACC + GYRO)
Definition: motion_fx.h:105
int start_automatic_gbias_calculation
Definition: motion_fx.h:95
Definition: motion_fx.h:121
Definition: motion_fx.h:78
Definition: motion_fx.h:122
Definition: motion_fx.h:69
void MotionFX_enable_9X(MFX_engine_state_t enable)
Enable or disable the 9 axes function (ACC + GYRO + MAG)
Definition: motion_fx.h:75
Definition: motion_fx.h:124
Definition: motion_fx.h:127
void MotionFX_update(MFX_output_t *data_out, MFX_input_t *data_in, float eml_deltatime, float *eml_q_update)
Run the Kalman filter update.
Definition: motion_fx.h:98
Definition: motion_fx.h:74
void MotionFX_MagCal_run(MFX_MagCal_input_t *data_in)
Run magnetic calibration algorithm.
MFX_engine_state_t MotionFX_getStatus_9X(void)
Get the status of the 9 axes library.
Generated on Fri May 12 2017 11:19:25 for MotionFX Software Library by
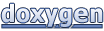