GD32F1x0
|
usb_int.c
Go to the documentation of this file.
156 /* Read BESL field from subendpoint0 register which coressponds to HIRD parameter in LPM spec */
159 /* Read BREMOTEWAKE bit from subendpoint0 register which corresponding to bRemoteWake bit in LPM request */
Definition: usb_core.h:212
Definition: usbd_it.h:36
USB device interrupt management header file.
Generated by
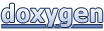