GD32F1x0
|
usb_buf.c
Go to the documentation of this file.
void FreeUserBuffer(uint8_t EpID, uint8_t Dir)
Free buffer used from application by toggling the SW_BUF byte.
Definition: usb_buf.c:36
#define _ToggleSWBUF_TX(EpID)
Toggle SW_BUF bit in the double buffered endpoint.
Definition: usb_regs.h:516
void BufferCopyToUser(uint8_t *UsrBuf, uint16_t BufAddr, uint16_t Bytes)
Copy a buffer from the allocation buffer area to user memory area.
Definition: usb_buf.c:78
void UserCopyToBuffer(uint8_t *UsrBuf, uint16_t BufAddr, uint16_t Bytes)
Copy a buffer from user memory area to the allocation buffer area.
Definition: usb_buf.c:55
USB buffer interface functions prototypes.
Generated by
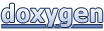