DexelaDetector API
|
This class is used to control GigE Type Detectors. It will give access to functions that are not available to other interface-type detectors.
Note: For all standard detector function calls please see the DexelaDetector class (these functions are also available to DexelaDetectorGE objects)
More...
#include <DexelaDetectorGE.h>
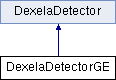
Public Member Functions | |||||||||||
DexelaDetectorGE (DevInfo &devInfo) | |||||||||||
Constructor for DexelaDetectorGE. Identical to the DexelaDetector constructor, except with an additional check for the correct (GIGE) interface. Note: A
| |||||||||||
DexelaDetectorGE (DetectorInterface transport, int unit, const char *params) | |||||||||||
Constructor for DexelaDetectorGE. This version assumes you know the interface and the correct parameters to connect to the detector. More... | |||||||||||
virtual | ~DexelaDetectorGE (void) | ||||||||||
Destructor for DexelaDetectorGE. More... | |||||||||||
void | SetPersistentIPAddress (int firstByte, int secondByte, int thirdByte, int fourthByte) | ||||||||||
Function for setting a new persistent IP address for the detector. After power-cycling the detector it should come up with the desired IP address More... | |||||||||||
void | OpenBoard () | ||||||||||
Identical to the OpenBoard call. The only difference is a check to make sure the detector has the correct (GIGE) interface. More... | |||||||||||
void | OpenBoard (int NumBufs) | ||||||||||
Identical to the OpenBoard call. The only difference is a check to make sure the detector has the correct (GIGE) interface. More... | |||||||||||
![]() | |||||||||||
DexelaDetector (DevInfo &devInfo) | |||||||||||
Constructor for DexelaDetector. This version uses the DevInfo struct returned from a GetDevice, GetDeviceGE or GetDeviceCL call. More... | |||||||||||
DexelaDetector (DetectorInterface transport, int unit, const char *params) | |||||||||||
Constructor for DexelaDetector. This version assumes you know the interface and the correct parameters to connect to the detector. More... | |||||||||||
virtual | ~DexelaDetector (void) | ||||||||||
Destructor for DexelaDetector. More... | |||||||||||
void | OpenBoard (int NumBufs) | ||||||||||
Opens the connection to the detector and sets the number of buffers to use/allocate. Every open should be matched with a close to free resources. More... | |||||||||||
void | CloseBoard () | ||||||||||
Closes the connection to the detector. More... | |||||||||||
int | GetBufferXdim (void) | ||||||||||
Get the x dimension of the transport buffer (in bytes) More... | |||||||||||
int | GetBufferYdim (void) | ||||||||||
Get the y dimension of the transport buffer (in pixels) More... | |||||||||||
int | GetNumBuffers (void) | ||||||||||
Get the number of internal buffers that are currently allocated for the detector. More... | |||||||||||
int | GetCapturedBuffer (void) | ||||||||||
Gets the number of the buffer just captured. This can be used to determine which buffer to read-out. More... | |||||||||||
int | GetFieldCount (void) | ||||||||||
Gets the number of fields(frames) captured so far. More... | |||||||||||
void | ReadBuffer (int bufNum, byte *buffer) | ||||||||||
Reads the specified transport buffer into the passed in buffer (byte*). Note: GetCapturedBuffer can be used to get the number of the lastest buffer to be filled. More... | |||||||||||
void | ReadBuffer (int bufNum, DexImage &img, int iZ=0) | ||||||||||
Reads the specified transport buffer into the passed in DexImage object at the passed in plane. Note: GetCapturedBuffer can be used to get the number of the lastest buffer to be filled. More... | |||||||||||
void | WriteBuffer (int bufNum, byte *buffer) | ||||||||||
Writes data to the specified transport buffer. More... | |||||||||||
void | SetFullWellMode (FullWellModes fwm) | ||||||||||
Sets the full well mode parameter of the detector. More... | |||||||||||
void | SetExposureMode (ExposureModes mode) | ||||||||||
Sets the ExposureMode parameter of the detector More... | |||||||||||
void | SetExposureTime (float timems) | ||||||||||
Sets the exposure time parameter of the detector More... | |||||||||||
void | SetBinningMode (bins flag) | ||||||||||
Sets the binning mode of the detector. More... | |||||||||||
void | SetTestMode (BOOL SetTestOn) | ||||||||||
Enables/disables test mode. The detector will output a generated test pattern if this mode is turned on. More... | |||||||||||
void | SetTriggerSource (ExposureTriggerSource ets) | ||||||||||
Sets the trigger source setting on the detector More... | |||||||||||
void | SetNumOfExposures (int num) | ||||||||||
Sets the number of exposures to acquire after a trigger. This is only relevant in Sequence_Exposure and Frame_Rate_exposure modes of operation. More... | |||||||||||
int | GetNumOfExposures () | ||||||||||
Gets the number of exposures setting from the detector. This is only relevant in Sequence_Exposure and Frame_Rate_exposure modes of operation. More... | |||||||||||
void | SetGapTime (float timems) | ||||||||||
Sets the gap-time setting of the detector. When run in Frame_Rate_exposure mode the detector will insert this gap period between consecutive frames in an image sequence. Note: The minimum time for the gap-time setting is the current readout-time for the detector. Attempting to write anything smaller to the detector will result in a gap-time equal to the readout-time. More... | |||||||||||
float | GetGapTime () | ||||||||||
Gets the current gap-time setting of the detector. When run in Frame_Rate_exposure mode the detector will insert this gap period between consecutive frames in an image sequence. Note: The minimum time for the gap-time setting is the current readout-time for the detector. Attempting to write anything smaller to the detector will result in a gap-time equal to the readout-time. More... | |||||||||||
bool | IsConnected () | ||||||||||
Check to see if the connection to the detector is open (i.e. OpenBoard) More... | |||||||||||
ExposureModes | GetExposureMode () | ||||||||||
Gets the ExposureMode parameter of the detector. More... | |||||||||||
float | GetExposureTime () | ||||||||||
Gets the exposure time parameter of the detector (ms). More... | |||||||||||
DetStatus | GetDetectorStatus () | ||||||||||
Returns the current settings of the detector in the form of a DetStatus object. More... | |||||||||||
ExposureTriggerSource | GetTriggerSource () | ||||||||||
Gets the current trigger source setting from the detector More... | |||||||||||
BOOL | GetTestMode () | ||||||||||
Gets the current state of the detector test mode (on/off) More... | |||||||||||
FullWellModes | GetFullWellMode () | ||||||||||
Gets the current detector well-mode. More... | |||||||||||
bins | GetBinningMode () | ||||||||||
Gets the current state of the detector binning mode More... | |||||||||||
int | GetSerialNumber () | ||||||||||
Gets the detector serial number. More... | |||||||||||
int | GetModelNumber () | ||||||||||
Gets the detector model number. More... | |||||||||||
int | GetFirmwareVersion () | ||||||||||
Gets the detector firmware version number. More... | |||||||||||
void | GetFirmwareBuild (int &iDayAndMonth, int &iYear, int &iTime) | ||||||||||
Gets the detector firmware build date. Note: This feature may not be supported on older detectors More... | |||||||||||
DetectorInterface | GetTransportMethod () | ||||||||||
Returns the communication method (i.e. interface) for the detector object. More... | |||||||||||
double | GetReadOutTime () | ||||||||||
This method will return the read-out time of the detector (in ms) for it's current binning mode. More... | |||||||||||
bool | IsCallbackActive () | ||||||||||
This method will inform the user if the callback mode (i.e. background thread) is currently active. More... | |||||||||||
bool | IsLive () | ||||||||||
This method will inform the user if detector is currently in Live mode. More... | |||||||||||
void | Snap (int buffer, int timeout) | ||||||||||
Snaps an image into the specified buffer. Note: If the detector trigger source is set to Internal_Software, this call will automatically trigger the detector. More... | |||||||||||
int | ReadRegister (int address, int sensorNum=1) | ||||||||||
Reads the specified register from the detector. The sensor number corresponds to the desired sensor from which to read the register. The SensorNumber will default to 1 (master-sensor) if not specified otherwise by the user. More... | |||||||||||
void | WriteRegister (int address, int value, int sensorNum=0) | ||||||||||
Writes the value to the specified register from the detector. The sensor number corresponds to the desired sensor to which the value will be written. The SensorNumber will default to 0 (broadcast to all sensors) if not specified otherwise by the user. More... | |||||||||||
void | ClearCameraBuffer (int i) | ||||||||||
Clears (i.e. zero-out) the specified camera buffer. More... | |||||||||||
void | ClearBuffers () | ||||||||||
Clears all the camera buffers More... | |||||||||||
void | LoadSensorConfigFile (char *filename) | ||||||||||
Loads the sensor configuration file into the detector. This file will write values to the ADC offset registers for each sensor in the detector. More... | |||||||||||
void | SoftReset (void) | ||||||||||
Cycles the power on the detector More... | |||||||||||
void | GoLiveSeq (int start, int stop, int numBuf) | ||||||||||
Sets the host computer up to be ready to recieve images into the specified buffer range. More... | |||||||||||
void | GoLiveSeq () | ||||||||||
Sets the host computer up to be ready to recieve images. This call will use all available buffers in a circular fashion (i.e. ring-buffer). More... | |||||||||||
void | GoUnLive () | ||||||||||
Exits live mode. The host computer will no longer be ready to recieve transmitted images. More... | |||||||||||
void | SoftwareTrigger () | ||||||||||
Sends a trigger to the detector (will only work if the trigger source is set to Internal_Software) More... | |||||||||||
void | EnablePulseGenerator (float frequency) | ||||||||||
This function will enable the pulse generator software trigger signal. In this mode the software trigger can be continuously sent to the detector at the desired frequency. Note: In order to use this mode the trigger source should be set to Internal_Software Note2: To actually enable the pulse train you must call ToggleGenerator. A SoftwareTrigger call will not work when in this mode. More... | |||||||||||
void | EnablePulseGenerator () | ||||||||||
This function is identical to EnablePulseGenerator, except that the frequency of the pulse train is set automatically. The frequency will be set such as to ensure continuous image acquisition for the current detector/binning mode. Note: In order to use this mode the trigger source should be set to Internal_Software Note2: To actually enable the pulse train you must call ToggleGenerator. A SoftwareTrigger call will not work when in this mode. More... | |||||||||||
void | DisablePulseGenerator () | ||||||||||
This function will disable Pulse Generator mode. After calling this you should be able to use the SoftwareTrigger call. More... | |||||||||||
void | ToggleGenerator (BOOL onOff) | ||||||||||
This function will control the pulse train. Note: In order to use this mode the pulse generator must be enabled. See EnablePulseGenerator. More... | |||||||||||
void | WaitImage (int timeout) | ||||||||||
This function will wait for the specified amount of time for an image to arrive. Note: If the image arrives before then it will return as soon as it does (i.e. it won't wait for the duration of the timeout period). If the image does not arrive in the specified time a DexelaException will be thrown. More... | |||||||||||
void | SetCallback (IMAGE_CALLBACK func) | ||||||||||
Sets the user defined callback funtction to be called for every image arrival event. More... | |||||||||||
void | StopCallback () | ||||||||||
This function will terminate the callback loop (i.e. will wait for all spawned threads to finish exectuing). More... | |||||||||||
void | CheckForCallbackError () | ||||||||||
This function will check to see if any errors have occurred in the background thread that is running when using callbacks. This thread is activated after a call to SetCallback and terminated with a call to StopCallback. If no error has occurred this method will just return. if an error has occurred a DexelaException will be thrown. More... | |||||||||||
void | CheckForLiveError () | ||||||||||
This function will check to see if any errors have occurred in the background thread that is running when using live-mode. This thread is activated after a call to GoLiveSeq and terminated with a call to GoUnLive. If no error has occurred this method will just return. if an error has occurred a DexelaException will be thrown. More... | |||||||||||
void | SetPreProgrammedExposureTimes (int numExposures, float *exposuretimes_ms) | ||||||||||
This method will set the exposure times for pre-programmed exposure mode. More... | |||||||||||
void | SetROICoordinates (unsigned short usStartColumn, unsigned short usStartRow, unsigned short usROIWidth, unsigned short usROIHeight) | ||||||||||
This method set the coordinates of the ROI when detector runs in ROI mode.
| |||||||||||
void | GetROICoordinates (unsigned short &usStartColumn, unsigned short &usStartRow, unsigned short &usROIWidth, unsigned short &usROIHeight) | ||||||||||
This method retrieves the coordinates of the region of interest (ROI) set within the detector. More... | |||||||||||
void | EnableROIMode (bool bEnableROI) | ||||||||||
This method activates or deactivates the ROI mode of the detector. More... | |||||||||||
bool | GetROIState () | ||||||||||
This method retrieves the enabled state of the region of interest of the detector. More... | |||||||||||
unsigned short | GetSensorHeight (unsigned short uiSensorID=1) | ||||||||||
Gets the height of the sensor in pixels. More... | |||||||||||
unsigned short | GetSensorWidth (unsigned short uiSensorID=1) | ||||||||||
Gets the width of the sensor in pixels. More... | |||||||||||
bool | IsFrameCntWithinImage () | ||||||||||
Checks if framecounter is displayed within the image data (being the 2nd pixel). More... | |||||||||||
void | EnableFrameCntWithinImage (unsigned short usEnable) | ||||||||||
Enables displaying the framecounter in the image (being the 2nd pixel). More... | |||||||||||
void | SetSlowed (bool flag) | ||||||||||
This method can specify to the api that the detector being used is a slowed-down detector (e.g. mammo detector). This should not be necessary as the API should be able to determine most of the time whether the firmwarwe version is a slowed down one. However, for certain older detectors/firmwares this may not be possible. In this case this method can be used to inform the library that the firmware is slowed down and it will use the correct read-out times. More... | |||||||||||
void | SetReadoutMode (ReadoutModes mode) | ||||||||||
Sets the ReadoutMode parameter of the detector More... | |||||||||||
ReadoutModes | GetReadoutMode () | ||||||||||
Gets the ReadoutModes parameter of the detector. More... | |||||||||||
int | QueryReadoutMode (ReadoutModes mode) | ||||||||||
Query the detector to see if the desired readout mode is present (i.e. available). Note: Older detectors may not support the querying of features. In this case a value of -1 will be returned indicating that it is uknown whether the feature is present. In this case it is possible that the feature is present but that the detector is unable to report so. It may still be possible to use the feature in this case but no guarantees can be made on whether or not it will work. More... | |||||||||||
int | QueryExposureMode (ExposureModes mode) | ||||||||||
Query the detector to see if the desired exposure mode is present (i.e. available). Note: Older detectors may not support the querying of features. In this case a value of -1 will be returned indicating that it is uknown whether the feature is present. In this case it is possible that the feature is present but that the detector is unable to report so. It may still be possible to use the feature in this case but no guarantees can be made on whether or not it will work. More... | |||||||||||
int | QueryTriggerSource (ExposureTriggerSource ets) | ||||||||||
Query the detector to see if the desired trigger source is present (i.e. available). Note: Older detectors may not support the querying of features. In this case a value of -1 will be returned indicating that it is uknown whether the feature is present. In this case it is possible that the feature is present but that the detector is unable to report so. It may still be possible to use the feature in this case but no guarantees can be made on whether or not it will work. More... | |||||||||||
int | QueryFullWellMode (FullWellModes fwm) | ||||||||||
Query the detector to see if the desired full-well mode is present (i.e. available). Note: Older detectors may not support the querying of features. In this case a value of -1 will be returned indicating that it is uknown whether the feature is present. In this case it is possible that the feature is present but that the detector is unable to report so. It may still be possible to use the feature in this case but no guarantees can be made on whether or not it will work. More... | |||||||||||
int | QueryBinningMode (bins flag) | ||||||||||
Query the detector to see if the desired binning mode is present (i.e. available). Note: Older detectors may not support the querying of features. In this case a value of -1 will be returned indicating that it is uknown whether the feature is present. In this case it is possible that the feature is present but that the detector is unable to report so. It may still be possible to use the feature in this case but no guarantees can be made on whether or not it will work. More... | |||||||||||
Additional Inherited Members | |
![]() | |
boost::shared_ptr< baseDetector > | base |
boost::shared_ptr< gigEDetector > | gigeDet |
boost::shared_ptr < camLinkDetector > | clDet |
Detailed Description
This class is used to control GigE Type Detectors. It will give access to functions that are not available to other interface-type detectors.
Note: For all standard detector function calls please see the DexelaDetector class (these functions are also available to DexelaDetectorGE objects)
Constructor & Destructor Documentation
DexelaDetectorGE::DexelaDetectorGE | ( | DevInfo & | devInfo | ) |
Constructor for DexelaDetectorGE. Identical to the DexelaDetector constructor, except with an additional check for the correct (GIGE) interface.
Note: A
- Exceptions
-
DexelaException is thrown if the interface of the DevInfo object is not correct (i.e. GIGE)
- Parameters
-
devInfo The DevInfo object for the desired detector. This can be obtained from the GetDeviceGE method
- Exceptions
-
DexelaException
DexelaDetectorGE::DexelaDetectorGE | ( | DetectorInterface | transport, |
int | unit, | ||
const char * | params | ||
) |
Constructor for DexelaDetectorGE. This version assumes you know the interface and the correct parameters to connect to the detector.
- Parameters
-
transport The DetectorInterface for the detector (i.e. GIGE) unit For GIGE detectors this can be set to 0 params The parameter string for connection to the detector. For GIGE detectors this should be the detector IP address.
- Exceptions
-
DexelaException
|
virtual |
Destructor for DexelaDetectorGE.
Member Function Documentation
|
virtual |
Identical to the OpenBoard call. The only difference is a check to make sure the detector has the correct (GIGE) interface.
- Exceptions
-
DexelaException
Reimplemented from DexelaDetector.
void DexelaDetectorGE::OpenBoard | ( | int | NumBufs | ) |
Identical to the OpenBoard call. The only difference is a check to make sure the detector has the correct (GIGE) interface.
- Parameters
-
NumBufs Number of buffers to use/allocate
- Exceptions
-
DexelaException
void DexelaDetectorGE::SetPersistentIPAddress | ( | int | firstByte, |
int | secondByte, | ||
int | thirdByte, | ||
int | fourthByte | ||
) |
Function for setting a new persistent IP address for the detector. After power-cycling the detector it should come up with the desired IP address
- Parameters
-
firstByte The first byte of the desired IP address (e.g. 169 for the address 169.254.70.3) secondByte The second byte of the desired IP address (e.g. 254 for the address 169.254.70.3) thirdByte The third byte of the desired IP address (e.g. 70 for the address 169.254.70.3) fourthByte The fourth byte of the desired IP address (e.g. 3 for the address 169.254.70.3)
- Exceptions
-
DexelaException
The documentation for this class was generated from the following files:
- DexelaDetectorGE.h
- DexelaDetectorGE.cpp
Generated on Tue Nov 25 2014 10:22:45 for DexelaDetector API by
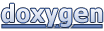