DexelaDetector API
|
This class is used to store and handle the images acquired from a detector. More...
#include <DexImage.h>
Public Member Functions | |
DexImage (void) | |
DexImage constructor. Creates a new (empty) image. More... | |
DexImage (const char *filename) | |
DexImage constructor. Creates a new image by reading in from the specified file. More... | |
DexImage (const DexImage &input) | |
DexImage copy constructor. Creates a new DexImage object by copying the input DexImage object. More... | |
void | operator= (const DexImage &input) |
DexImage assignment operator. Creates a new DexImage object by copying the input DexImage object. More... | |
~DexImage (void) | |
DexImage destructor. More... | |
void | ReadImage (const char *filename) |
Reads an image in from the specified file More... | |
void | WriteImage (const char *filename) |
Writes the image data to the specified file (SMV, HIS or TIF) Note: This will write the entire image stack out. Note2: See the function WriteImage for writing out a single (user specified) plane from the stack. More... | |
void | WriteImage (const char *filename, int iZ) |
Writes out a single image plane (user specified) from the stack (SMV, HIS or TIF) More... | |
void | Build (int iWidth, int iHeight, int iDepth, pType iPxType) |
Builds an image using the specified dimensions and pixel type More... | |
void | Build (int model, bins binFmt, int iDepth) |
Builds an image using the specified detector model and bining format More... | |
void * | GetDataPointerToPlane (int iZ=0) |
Gets the pointer to the image data for the specified plane. More... | |
int | GetImageXdim () |
Gets the image x dimension (width) in pixels. More... | |
int | GetImageYdim () |
Gets the image y dimension (height) in pixels. More... | |
int | GetImageDepth () |
Gets the image depth. More... | |
pType | GetImagePixelType () |
Gets the image pixel type. More... | |
float | PlaneAvg (int iZ=0) |
Calculates the average pixel value of the input image for the specified plane. More... | |
void | FixFlood () |
This image fixes the input flood image. This means that the reciprocal of the image is taken and normalized about 1. This is done to speed up the flood correction procedure (multiplication is faster than division). Note: The input flood image should be a median image (i.e. should have a depth of 1). This can be obtained by using the FindMedianofPlanes method. More... | |
void | FindMedianofPlanes () |
This calculates the median image from the input image stack. Note: This function will replace the input stack of images with a single (median) image More... | |
void | FindAverageofPlanes () |
This calculates the average image from the input image stack. Note: This function will replace the input stack of images with a single (average) image of type float More... | |
void | LinearizeData () |
Linearizes the pixel values of the image. This is done using a piece-wise linear approximation where the sections are defined by an array of integers (linearization starts). This allows the output of the detector to be made linear Note: A default set of linearization starts will be used unless the user specifies their own using the SetLinearizationStarts method. More... | |
void | SubtractDark () |
Subtracts a dark image from an input image. Note: The dark images must first be loaded before calling this function (see LoadDarkImage). Note2: A dark offset value will be added to the resulting image to prevent any negative numbers. This offset is set to 300 by default but can be changed using the SetDarkOffset method. More... | |
void | FloodCorrection () |
Performs flood correction on the input image using the passed in fixed-flood image. Note: The flood and dark images must first be loaded before calling this function (see LoadFloodImage, LoadDarkImage). More... | |
void | DefectCorrection (int DefectFlags=31) |
Function for performing defect corrections on the image. Note: The flood, dark and defect map images must first be loaded before calling this function (see LoadFloodImage, LoadDarkImage, LoadDefectMap). More... | |
void | SubImageDefectCorrection (int startCol, int startRow, int width, int height, int CorrectionsFlag=31) |
void | FullCorrection () |
This function performs the full correction (dark/offset, flood/gain and defect). Note: The flood, dark and defect map images must first be loaded before calling this function (see LoadFloodImage, LoadDarkImage, LoadDefectMap). More... | |
void | UnscrambleImage () |
This function unscrambles (sorts) a raw image acquired from a detector. Note: The model number and the binning mode of the detector that the image was captured from must be specified (using SetImageParameters) before calling this method. More... | |
void | AddImage () |
Adds another image (plane) to the stack. More... | |
void | LoadDarkImage (const DexImage &dark) |
Loads the dark image from the specified DexImage object. Note: This dark image will then automatically be used for the various corrections. Note2: This image should be a single plane (e.g. median) image. If it's not then the median image will be calulated (using FindMedianofPlanes) and stored. Note3: This image should be of type Offset. More... | |
void | LoadDarkImage (const char *filename) |
Loads the dark image from the specified file. Note: This dark image will then automatically be used for the various corrections. Note2: This image should be a single plane (e.g. median) image. If it's not then the median image will be calulated (using FindMedianofPlanes) and stored. Note3: This image should be of type Offset. More... | |
void | LoadFloodImage (const DexImage &flood) |
Loads the flood image from the specified DexImage object. Note: This flood image will then automatically be used for offset corrections. Note2: This image should be a single plane, fixed floating point image. If it's not then the median image will be calulated (using FindMedianofPlanes), then the image will be fixed (using FixFlood) and stored. Note3: This image should be of type Gain. More... | |
void | LoadFloodImage (const char *filename) |
Loads the flood image from the specified file. Note: This flood image will then automatically be used for gain corrections. Note2: This image should be a single plane, fixed floating point image. If it's not then the median image will be calulated (using FindMedianofPlanes), then the image will be fixed (using FixFlood) and stored. Note3: This image should be of type Gain. More... | |
void | LoadDefectMap (const DexImage &defect) |
Loads the defect-map image from the specified DexImage object. Note: This defect-map image will then automatically be used for defect corrections. Note2: This image should be of type Defect. More... | |
void | LoadDefectMap (const char *filename) |
Loads the defect-map image from the specified file. Note: This defect-map image will then automatically be used for defect corrections. Note2: This image should be of type Defect.. More... | |
DexImage | GetDarkImage () |
Gets the dark image (which is used in offset/dark correction). More... | |
DexImage | GetFloodImage () |
Gets the flood image (which is used in gain/flood correction). More... | |
DexImage | GetDefectMap () |
Gets the defect-map image (which is used in defect correction). More... | |
DexImage | GetImagePlane (int iZ) |
Creates a new DexImage object from the data at the specified plane. More... | |
DexImageTypes | GetImageType () |
Gets the image type (e.g. offset, gain, data, defect map). More... | |
void | SetImageType (DexImageTypes type) |
Sets the image type to the desired type. More... | |
void | SetDarkOffset (int offset) |
Sets the dark offset value to be used for various corrections (e.g. dark correction). This value is used as an offset to prevent from the possibility of getting negative pixel values. More... | |
int | GetDarkOffset () |
Gets the current dark offset value. This value is used as an offset to prevent from the possibility of getting negative pixel values. More... | |
void | SetLinearizationStarts (unsigned int *msArray, int msLength) |
Sets the linearization section numbers that are used for the linearization correciton (LinearizeData). More... | |
unsigned int * | GetLinearizationStarts (int &msLength) |
Gets the linearization section numbers that are used for the linearization correciton(LinearizeData). More... | |
void | SetImageParameters (bins binningMode, int modelNumber) |
Sets the model number and the binning mode of the detector that was used to acquire the image. This is used for the data-sorting (UnscrambleImage). More... | |
int | GetImageModel () |
Gets the model number of the detector that was used to acquire the image. More... | |
bins | GetImageBinning () |
Gets the binning mode of the detector that was used to acquire the image. More... | |
bool | IsEmpty () |
This method returns whether the image is empty or not More... | |
void | SetScrambledFlag (bool onOff) |
This method can be used to manually set the scrambled flag of the image. This flag stores whether the data from teh detector has already been unscrambled or not. This flag is automatically set when an image is unscrambled and consequently this method should not be required for most use-cases. More... | |
void | SetROIParameters (unsigned short usStartColumn, unsigned short usStartRow, unsigned short usROIWidth, unsigned short usROIHeight) |
Detailed Description
This class is used to store and handle the images acquired from a detector.
Constructor & Destructor Documentation
DexImage::DexImage | ( | void | ) |
DexImage constructor. Creates a new (empty) image.
- Exceptions
-
DexelaException
DexImage::DexImage | ( | const char * | filename | ) |
DexImage constructor. Creates a new image by reading in from the specified file.
- Parameters
-
filename Path to file to read image in from
- Exceptions
-
DexelaException
DexImage::DexImage | ( | const DexImage & | input | ) |
DexImage copy constructor. Creates a new DexImage object by copying the input DexImage object.
- Parameters
-
input DexImage object to copy from.
- Exceptions
-
DexelaException
DexImage::~DexImage | ( | void | ) |
DexImage destructor.
Member Function Documentation
void DexImage::AddImage | ( | ) |
Adds another image (plane) to the stack.
- Exceptions
-
DexelaException
void DexImage::Build | ( | int | iWidth, |
int | iHeight, | ||
int | iDepth, | ||
pType | iPxType | ||
) |
Builds an image using the specified dimensions and pixel type
- Parameters
-
iWidth Desired width (in pixels) for the image. iHeight Desired height (in pixels) for the image. iDepth Desired depth for the image. iPxType A member of the pType enumeration representing the pixel type for the image.
- Exceptions
-
DexelaException
void DexImage::Build | ( | int | model, |
bins | binFmt, | ||
int | iDepth | ||
) |
Builds an image using the specified detector model and bining format
- Parameters
-
model Detector model type corresponding to the image. binFmt A member of the bins enumeration representing the binning mode corresponding to the image. iDepth Desired depth for the image.
- Exceptions
-
DexelaException
void DexImage::DefectCorrection | ( | int | DefectFlags = 31 | ) |
Function for performing defect corrections on the image.
Note: The flood, dark and defect map images must first be loaded before calling this function (see LoadFloodImage, LoadDarkImage, LoadDefectMap).
- Exceptions
-
DexelaException
void DexImage::FindAverageofPlanes | ( | ) |
This calculates the average image from the input image stack.
Note: This function will replace the input stack of images with a single (average) image of type float
- Exceptions
-
DexelaException
void DexImage::FindMedianofPlanes | ( | ) |
This calculates the median image from the input image stack.
Note: This function will replace the input stack of images with a single (median) image
- Exceptions
-
DexelaException
void DexImage::FixFlood | ( | ) |
This image fixes the input flood image. This means that the reciprocal of the image is taken and normalized about 1. This is done to speed up the flood correction procedure (multiplication is faster than division).
Note: The input flood image should be a median image (i.e. should have a depth of 1). This can be obtained by using the FindMedianofPlanes method.
- Exceptions
-
DexelaException
void DexImage::FloodCorrection | ( | ) |
Performs flood correction on the input image using the passed in fixed-flood image.
Note: The flood and dark images must first be loaded before calling this function (see LoadFloodImage, LoadDarkImage).
- Exceptions
-
DexelaException
void DexImage::FullCorrection | ( | ) |
This function performs the full correction (dark/offset, flood/gain and defect).
Note: The flood, dark and defect map images must first be loaded before calling this function (see LoadFloodImage, LoadDarkImage, LoadDefectMap).
- Exceptions
-
DexelaException
DexImage DexImage::GetDarkImage | ( | ) |
Gets the dark image (which is used in offset/dark correction).
- Returns
- The DexImage object that is used for offset/dark corrections
- Exceptions
-
DexelaException
int DexImage::GetDarkOffset | ( | ) |
Gets the current dark offset value. This value is used as an offset to prevent from the possibility of getting negative pixel values.
- Returns
- The offset value (in ADU).
- Exceptions
-
DexelaException
void * DexImage::GetDataPointerToPlane | ( | int | iZ = 0 | ) |
Gets the pointer to the image data for the specified plane.
- Parameters
-
iZ The number of the desired image plane.
- Returns
- Pointer to the image data for the specified plane.
- Exceptions
-
DexelaException
DexImage DexImage::GetDefectMap | ( | ) |
Gets the defect-map image (which is used in defect correction).
- Returns
- The DexImage object that is used for defect corrections
- Exceptions
-
DexelaException
DexImage DexImage::GetFloodImage | ( | ) |
Gets the flood image (which is used in gain/flood correction).
- Returns
- The DexImage object that is used for gain/flood corrections
- Exceptions
-
DexelaException
bins DexImage::GetImageBinning | ( | ) |
Gets the binning mode of the detector that was used to acquire the image.
- Returns
- A member of the bins enumeration representing the binning mode of the detector.
- Exceptions
-
DexelaException
int DexImage::GetImageDepth | ( | ) |
int DexImage::GetImageModel | ( | ) |
Gets the model number of the detector that was used to acquire the image.
- Returns
- The detector model number.
- Exceptions
-
DexelaException
pType DexImage::GetImagePixelType | ( | ) |
Gets the image pixel type.
- Returns
- A member of the pType enumeration specifying the image pixel type.
- Exceptions
-
DexelaException
DexImage DexImage::GetImagePlane | ( | int | iZ | ) |
Creates a new DexImage object from the data at the specified plane.
- Parameters
-
iZ The index of the plane to get the data from.
- Returns
- A new DexImage object that consists of the data from the desired plane
- Exceptions
-
DexelaException
DexImageTypes DexImage::GetImageType | ( | ) |
Gets the image type (e.g. offset, gain, data, defect map).
- Returns
- A member of the DexImageTypes enumeration specifying the image type.
- Exceptions
-
DexelaException
int DexImage::GetImageXdim | ( | ) |
Gets the image x dimension (width) in pixels.
- Returns
- Image x dimension (width) in pixels.
- Exceptions
-
DexelaException
int DexImage::GetImageYdim | ( | ) |
Gets the image y dimension (height) in pixels.
- Returns
- Image y dimension (height) in pixels.
- Exceptions
-
DexelaException
unsigned int * DexImage::GetLinearizationStarts | ( | int & | msLength | ) |
Gets the linearization section numbers that are used for the linearization correciton(LinearizeData).
- Parameters
-
msLength An integer that will be set to the length of the linearization section numbers array.
- Returns
- An array of unsigned integers representing the section numbers used for linearization correction.
- Exceptions
-
DexelaException
bool DexImage::IsEmpty | ( | ) |
This method returns whether the image is empty or not
- Returns
- A boolean indicating whether the image is empty.
- Exceptions
-
DexelaException
void DexImage::LinearizeData | ( | ) |
Linearizes the pixel values of the image. This is done using a piece-wise linear approximation where the sections are defined by an array of integers (linearization starts). This allows the output of the detector to be made linear
Note: A default set of linearization starts will be used unless the user specifies their own using the SetLinearizationStarts method.
- Exceptions
-
DexelaException
void DexImage::LoadDarkImage | ( | const DexImage & | dark | ) |
Loads the dark image from the specified DexImage object.
Note: This dark image will then automatically be used for the various corrections.
Note2: This image should be a single plane (e.g. median) image. If it's not then the median image will be calulated (using FindMedianofPlanes) and stored.
Note3: This image should be of type Offset.
- Parameters
-
dark The DexImage object that should be used for dark corrections.
- Exceptions
-
DexelaException
void DexImage::LoadDarkImage | ( | const char * | filename | ) |
Loads the dark image from the specified file.
Note: This dark image will then automatically be used for the various corrections.
Note2: This image should be a single plane (e.g. median) image. If it's not then the median image will be calulated (using FindMedianofPlanes) and stored.
Note3: This image should be of type Offset.
- Parameters
-
filename The path to the image file that should be read in and used for dark corrections.
- Exceptions
-
DexelaException
void DexImage::LoadDefectMap | ( | const DexImage & | defect | ) |
Loads the defect-map image from the specified DexImage object.
Note: This defect-map image will then automatically be used for defect corrections.
Note2: This image should be of type Defect.
- Parameters
-
defect The DexImage object that should be used for defect corrections.
- Exceptions
-
DexelaException
void DexImage::LoadDefectMap | ( | const char * | filename | ) |
Loads the defect-map image from the specified file.
Note: This defect-map image will then automatically be used for defect corrections.
Note2: This image should be of type Defect..
- Parameters
-
filename The path to the image file that should be read in and used for defect corrections.
- Exceptions
-
DexelaException
void DexImage::LoadFloodImage | ( | const DexImage & | flood | ) |
Loads the flood image from the specified DexImage object.
Note: This flood image will then automatically be used for offset corrections.
Note2: This image should be a single plane, fixed floating point image. If it's not then the median image will be calulated (using FindMedianofPlanes), then the image will be fixed (using FixFlood) and stored.
Note3: This image should be of type Gain.
- Parameters
-
flood The DexImage object that should be used for flood corrections.
- Exceptions
-
DexelaException
void DexImage::LoadFloodImage | ( | const char * | filename | ) |
Loads the flood image from the specified file.
Note: This flood image will then automatically be used for gain corrections.
Note2: This image should be a single plane, fixed floating point image. If it's not then the median image will be calulated (using FindMedianofPlanes), then the image will be fixed (using FixFlood) and stored.
Note3: This image should be of type Gain.
- Parameters
-
flood The path to the image file that should be read in and used for flood corrections.
- Exceptions
-
DexelaException
void DexImage::operator= | ( | const DexImage & | input | ) |
DexImage assignment operator. Creates a new DexImage object by copying the input DexImage object.
- Parameters
-
input DexImage object to copy from.
- Exceptions
-
DexelaException
float DexImage::PlaneAvg | ( | int | iZ = 0 | ) |
Calculates the average pixel value of the input image for the specified plane.
- Parameters
-
iZ The plane of the image to work on
- Returns
- A floating point number that is the average pixel value of the specified image plane.
- Exceptions
-
DexelaException
void DexImage::ReadImage | ( | const char * | filename | ) |
Reads an image in from the specified file
- Parameters
-
filename The path to the file to read image from. Currently this is limited to SMV, HIS and TIF file types.
- Exceptions
-
DexelaException
void DexImage::SetDarkOffset | ( | int | offset | ) |
Sets the dark offset value to be used for various corrections (e.g. dark correction). This value is used as an offset to prevent from the possibility of getting negative pixel values.
- Parameters
-
offset The offset value (in ADU).
- Exceptions
-
DexelaException
void DexImage::SetImageParameters | ( | bins | binningMode, |
int | modelNumber | ||
) |
Sets the model number and the binning mode of the detector that was used to acquire the image. This is used for the data-sorting (UnscrambleImage).
- Parameters
-
binningMode A member of the bins enumeration representing the binning mode of the detector. modelNumber The model number of the detector
- Exceptions
-
DexelaException
void DexImage::SetImageType | ( | DexImageTypes | type | ) |
Sets the image type to the desired type.
- Parameters
-
type A member of the DexImageTypes enumeration that the image type should be set to.
- Exceptions
-
DexelaException
void DexImage::SetLinearizationStarts | ( | unsigned int * | msArray, |
int | msLength | ||
) |
Sets the linearization section numbers that are used for the linearization correciton (LinearizeData).
- Parameters
-
msArray An array of unsigned integers representing the section numbers used for linearization correction. msLength The length of the array
- Exceptions
-
DexelaException
void DexImage::SetScrambledFlag | ( | bool | onOff | ) |
This method can be used to manually set the scrambled flag of the image. This flag stores whether the data from teh detector has already been unscrambled or not. This flag is automatically set when an image is unscrambled and consequently this method should not be required for most use-cases.
- Parameters
-
onOff A boolean representing the desired state of the flag.
- Exceptions
-
DexelaException
void DexImage::SubtractDark | ( | ) |
Subtracts a dark image from an input image.
Note: The dark images must first be loaded before calling this function (see LoadDarkImage).
Note2: A dark offset value will be added to the resulting image to prevent any negative numbers. This offset is set to 300 by default but can be changed using the SetDarkOffset method.
- Exceptions
-
DexelaException
void DexImage::UnscrambleImage | ( | ) |
This function unscrambles (sorts) a raw image acquired from a detector.
Note: The model number and the binning mode of the detector that the image was captured from must be specified (using SetImageParameters) before calling this method.
- Exceptions
-
DexelaException
void DexImage::WriteImage | ( | const char * | filename | ) |
Writes the image data to the specified file (SMV, HIS or TIF)
Note: This will write the entire image stack out.
Note2: See the function WriteImage for writing out a single (user specified) plane from the stack.
- Parameters
-
filename The path to the file to write image to. Currently this is limited to SMV, HIS and TIF file types.
- Exceptions
-
DexelaException
void DexImage::WriteImage | ( | const char * | filename, |
int | iZ | ||
) |
Writes out a single image plane (user specified) from the stack (SMV, HIS or TIF)
- Parameters
-
filename The path to the file to write image to. Currently this is limited to SMV, HIS and TIF file types. iZ Image plane to write out
- Exceptions
-
DexelaException
The documentation for this class was generated from the following files:
- DexImage.h
- DexImage.cpp
Generated on Tue Nov 25 2014 10:22:45 for DexelaDetector API by
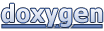