DexelaDetector API
|
This class is used to control any interface-type Detector and acquire images from it. It will provide all the basic functionality required for all different Dexela detectors. For interface specific functionality please see the interface specific classes (e.g. DexelaDetectorGE, DexelaDetectorCL). More...
#include <DexelaDetector.h>
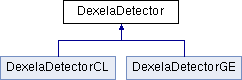
Public Member Functions | |||||||||||
DexelaDetector (DevInfo &devInfo) | |||||||||||
Constructor for DexelaDetector. This version uses the DevInfo struct returned from a GetDevice, GetDeviceGE or GetDeviceCL call. More... | |||||||||||
DexelaDetector (DetectorInterface transport, int unit, const char *params) | |||||||||||
Constructor for DexelaDetector. This version assumes you know the interface and the correct parameters to connect to the detector. More... | |||||||||||
virtual | ~DexelaDetector (void) | ||||||||||
Destructor for DexelaDetector. More... | |||||||||||
virtual void | OpenBoard () | ||||||||||
Opens the connection to the detector. Every open should be matched with a close to free resources. More... | |||||||||||
void | OpenBoard (int NumBufs) | ||||||||||
Opens the connection to the detector and sets the number of buffers to use/allocate. Every open should be matched with a close to free resources. More... | |||||||||||
void | CloseBoard () | ||||||||||
Closes the connection to the detector. More... | |||||||||||
int | GetBufferXdim (void) | ||||||||||
Get the x dimension of the transport buffer (in bytes) More... | |||||||||||
int | GetBufferYdim (void) | ||||||||||
Get the y dimension of the transport buffer (in pixels) More... | |||||||||||
int | GetNumBuffers (void) | ||||||||||
Get the number of internal buffers that are currently allocated for the detector. More... | |||||||||||
int | GetCapturedBuffer (void) | ||||||||||
Gets the number of the buffer just captured. This can be used to determine which buffer to read-out. More... | |||||||||||
int | GetFieldCount (void) | ||||||||||
Gets the number of fields(frames) captured so far. More... | |||||||||||
void | ReadBuffer (int bufNum, byte *buffer) | ||||||||||
Reads the specified transport buffer into the passed in buffer (byte*). Note: GetCapturedBuffer can be used to get the number of the lastest buffer to be filled. More... | |||||||||||
void | ReadBuffer (int bufNum, DexImage &img, int iZ=0) | ||||||||||
Reads the specified transport buffer into the passed in DexImage object at the passed in plane. Note: GetCapturedBuffer can be used to get the number of the lastest buffer to be filled. More... | |||||||||||
void | WriteBuffer (int bufNum, byte *buffer) | ||||||||||
Writes data to the specified transport buffer. More... | |||||||||||
void | SetFullWellMode (FullWellModes fwm) | ||||||||||
Sets the full well mode parameter of the detector. More... | |||||||||||
void | SetExposureMode (ExposureModes mode) | ||||||||||
Sets the ExposureMode parameter of the detector More... | |||||||||||
void | SetExposureTime (float timems) | ||||||||||
Sets the exposure time parameter of the detector More... | |||||||||||
void | SetBinningMode (bins flag) | ||||||||||
Sets the binning mode of the detector. More... | |||||||||||
void | SetTestMode (BOOL SetTestOn) | ||||||||||
Enables/disables test mode. The detector will output a generated test pattern if this mode is turned on. More... | |||||||||||
void | SetTriggerSource (ExposureTriggerSource ets) | ||||||||||
Sets the trigger source setting on the detector More... | |||||||||||
void | SetNumOfExposures (int num) | ||||||||||
Sets the number of exposures to acquire after a trigger. This is only relevant in Sequence_Exposure and Frame_Rate_exposure modes of operation. More... | |||||||||||
int | GetNumOfExposures () | ||||||||||
Gets the number of exposures setting from the detector. This is only relevant in Sequence_Exposure and Frame_Rate_exposure modes of operation. More... | |||||||||||
void | SetGapTime (float timems) | ||||||||||
Sets the gap-time setting of the detector. When run in Frame_Rate_exposure mode the detector will insert this gap period between consecutive frames in an image sequence. Note: The minimum time for the gap-time setting is the current readout-time for the detector. Attempting to write anything smaller to the detector will result in a gap-time equal to the readout-time. More... | |||||||||||
float | GetGapTime () | ||||||||||
Gets the current gap-time setting of the detector. When run in Frame_Rate_exposure mode the detector will insert this gap period between consecutive frames in an image sequence. Note: The minimum time for the gap-time setting is the current readout-time for the detector. Attempting to write anything smaller to the detector will result in a gap-time equal to the readout-time. More... | |||||||||||
bool | IsConnected () | ||||||||||
Check to see if the connection to the detector is open (i.e. OpenBoard) More... | |||||||||||
ExposureModes | GetExposureMode () | ||||||||||
Gets the ExposureMode parameter of the detector. More... | |||||||||||
float | GetExposureTime () | ||||||||||
Gets the exposure time parameter of the detector (ms). More... | |||||||||||
DetStatus | GetDetectorStatus () | ||||||||||
Returns the current settings of the detector in the form of a DetStatus object. More... | |||||||||||
ExposureTriggerSource | GetTriggerSource () | ||||||||||
Gets the current trigger source setting from the detector More... | |||||||||||
BOOL | GetTestMode () | ||||||||||
Gets the current state of the detector test mode (on/off) More... | |||||||||||
FullWellModes | GetFullWellMode () | ||||||||||
Gets the current detector well-mode. More... | |||||||||||
bins | GetBinningMode () | ||||||||||
Gets the current state of the detector binning mode More... | |||||||||||
int | GetSerialNumber () | ||||||||||
Gets the detector serial number. More... | |||||||||||
int | GetModelNumber () | ||||||||||
Gets the detector model number. More... | |||||||||||
int | GetFirmwareVersion () | ||||||||||
Gets the detector firmware version number. More... | |||||||||||
void | GetFirmwareBuild (int &iDayAndMonth, int &iYear, int &iTime) | ||||||||||
Gets the detector firmware build date. Note: This feature may not be supported on older detectors More... | |||||||||||
DetectorInterface | GetTransportMethod () | ||||||||||
Returns the communication method (i.e. interface) for the detector object. More... | |||||||||||
double | GetReadOutTime () | ||||||||||
This method will return the read-out time of the detector (in ms) for it's current binning mode. More... | |||||||||||
bool | IsCallbackActive () | ||||||||||
This method will inform the user if the callback mode (i.e. background thread) is currently active. More... | |||||||||||
bool | IsLive () | ||||||||||
This method will inform the user if detector is currently in Live mode. More... | |||||||||||
void | Snap (int buffer, int timeout) | ||||||||||
Snaps an image into the specified buffer. Note: If the detector trigger source is set to Internal_Software, this call will automatically trigger the detector. More... | |||||||||||
int | ReadRegister (int address, int sensorNum=1) | ||||||||||
Reads the specified register from the detector. The sensor number corresponds to the desired sensor from which to read the register. The SensorNumber will default to 1 (master-sensor) if not specified otherwise by the user. More... | |||||||||||
void | WriteRegister (int address, int value, int sensorNum=0) | ||||||||||
Writes the value to the specified register from the detector. The sensor number corresponds to the desired sensor to which the value will be written. The SensorNumber will default to 0 (broadcast to all sensors) if not specified otherwise by the user. More... | |||||||||||
void | ClearCameraBuffer (int i) | ||||||||||
Clears (i.e. zero-out) the specified camera buffer. More... | |||||||||||
void | ClearBuffers () | ||||||||||
Clears all the camera buffers More... | |||||||||||
void | LoadSensorConfigFile (char *filename) | ||||||||||
Loads the sensor configuration file into the detector. This file will write values to the ADC offset registers for each sensor in the detector. More... | |||||||||||
void | SoftReset (void) | ||||||||||
Cycles the power on the detector More... | |||||||||||
void | GoLiveSeq (int start, int stop, int numBuf) | ||||||||||
Sets the host computer up to be ready to recieve images into the specified buffer range. More... | |||||||||||
void | GoLiveSeq () | ||||||||||
Sets the host computer up to be ready to recieve images. This call will use all available buffers in a circular fashion (i.e. ring-buffer). More... | |||||||||||
void | GoUnLive () | ||||||||||
Exits live mode. The host computer will no longer be ready to recieve transmitted images. More... | |||||||||||
void | SoftwareTrigger () | ||||||||||
Sends a trigger to the detector (will only work if the trigger source is set to Internal_Software) More... | |||||||||||
void | EnablePulseGenerator (float frequency) | ||||||||||
This function will enable the pulse generator software trigger signal. In this mode the software trigger can be continuously sent to the detector at the desired frequency. Note: In order to use this mode the trigger source should be set to Internal_Software Note2: To actually enable the pulse train you must call ToggleGenerator. A SoftwareTrigger call will not work when in this mode. More... | |||||||||||
void | EnablePulseGenerator () | ||||||||||
This function is identical to EnablePulseGenerator, except that the frequency of the pulse train is set automatically. The frequency will be set such as to ensure continuous image acquisition for the current detector/binning mode. Note: In order to use this mode the trigger source should be set to Internal_Software Note2: To actually enable the pulse train you must call ToggleGenerator. A SoftwareTrigger call will not work when in this mode. More... | |||||||||||
void | DisablePulseGenerator () | ||||||||||
This function will disable Pulse Generator mode. After calling this you should be able to use the SoftwareTrigger call. More... | |||||||||||
void | ToggleGenerator (BOOL onOff) | ||||||||||
This function will control the pulse train. Note: In order to use this mode the pulse generator must be enabled. See EnablePulseGenerator. More... | |||||||||||
void | WaitImage (int timeout) | ||||||||||
This function will wait for the specified amount of time for an image to arrive. Note: If the image arrives before then it will return as soon as it does (i.e. it won't wait for the duration of the timeout period). If the image does not arrive in the specified time a DexelaException will be thrown. More... | |||||||||||
void | SetCallback (IMAGE_CALLBACK func) | ||||||||||
Sets the user defined callback funtction to be called for every image arrival event. More... | |||||||||||
void | StopCallback () | ||||||||||
This function will terminate the callback loop (i.e. will wait for all spawned threads to finish exectuing). More... | |||||||||||
void | CheckForCallbackError () | ||||||||||
This function will check to see if any errors have occurred in the background thread that is running when using callbacks. This thread is activated after a call to SetCallback and terminated with a call to StopCallback. If no error has occurred this method will just return. if an error has occurred a DexelaException will be thrown. More... | |||||||||||
void | CheckForLiveError () | ||||||||||
This function will check to see if any errors have occurred in the background thread that is running when using live-mode. This thread is activated after a call to GoLiveSeq and terminated with a call to GoUnLive. If no error has occurred this method will just return. if an error has occurred a DexelaException will be thrown. More... | |||||||||||
void | SetPreProgrammedExposureTimes (int numExposures, float *exposuretimes_ms) | ||||||||||
This method will set the exposure times for pre-programmed exposure mode. More... | |||||||||||
void | SetROICoordinates (unsigned short usStartColumn, unsigned short usStartRow, unsigned short usROIWidth, unsigned short usROIHeight) | ||||||||||
This method set the coordinates of the ROI when detector runs in ROI mode.
| |||||||||||
void | GetROICoordinates (unsigned short &usStartColumn, unsigned short &usStartRow, unsigned short &usROIWidth, unsigned short &usROIHeight) | ||||||||||
This method retrieves the coordinates of the region of interest (ROI) set within the detector. More... | |||||||||||
void | EnableROIMode (bool bEnableROI) | ||||||||||
This method activates or deactivates the ROI mode of the detector. More... | |||||||||||
bool | GetROIState () | ||||||||||
This method retrieves the enabled state of the region of interest of the detector. More... | |||||||||||
unsigned short | GetSensorHeight (unsigned short uiSensorID=1) | ||||||||||
Gets the height of the sensor in pixels. More... | |||||||||||
unsigned short | GetSensorWidth (unsigned short uiSensorID=1) | ||||||||||
Gets the width of the sensor in pixels. More... | |||||||||||
bool | IsFrameCntWithinImage () | ||||||||||
Checks if framecounter is displayed within the image data (being the 2nd pixel). More... | |||||||||||
void | EnableFrameCntWithinImage (unsigned short usEnable) | ||||||||||
Enables displaying the framecounter in the image (being the 2nd pixel). More... | |||||||||||
void | SetSlowed (bool flag) | ||||||||||
This method can specify to the api that the detector being used is a slowed-down detector (e.g. mammo detector). This should not be necessary as the API should be able to determine most of the time whether the firmwarwe version is a slowed down one. However, for certain older detectors/firmwares this may not be possible. In this case this method can be used to inform the library that the firmware is slowed down and it will use the correct read-out times. More... | |||||||||||
void | SetReadoutMode (ReadoutModes mode) | ||||||||||
Sets the ReadoutMode parameter of the detector More... | |||||||||||
ReadoutModes | GetReadoutMode () | ||||||||||
Gets the ReadoutModes parameter of the detector. More... | |||||||||||
int | QueryReadoutMode (ReadoutModes mode) | ||||||||||
Query the detector to see if the desired readout mode is present (i.e. available). Note: Older detectors may not support the querying of features. In this case a value of -1 will be returned indicating that it is uknown whether the feature is present. In this case it is possible that the feature is present but that the detector is unable to report so. It may still be possible to use the feature in this case but no guarantees can be made on whether or not it will work. More... | |||||||||||
int | QueryExposureMode (ExposureModes mode) | ||||||||||
Query the detector to see if the desired exposure mode is present (i.e. available). Note: Older detectors may not support the querying of features. In this case a value of -1 will be returned indicating that it is uknown whether the feature is present. In this case it is possible that the feature is present but that the detector is unable to report so. It may still be possible to use the feature in this case but no guarantees can be made on whether or not it will work. More... | |||||||||||
int | QueryTriggerSource (ExposureTriggerSource ets) | ||||||||||
Query the detector to see if the desired trigger source is present (i.e. available). Note: Older detectors may not support the querying of features. In this case a value of -1 will be returned indicating that it is uknown whether the feature is present. In this case it is possible that the feature is present but that the detector is unable to report so. It may still be possible to use the feature in this case but no guarantees can be made on whether or not it will work. More... | |||||||||||
int | QueryFullWellMode (FullWellModes fwm) | ||||||||||
Query the detector to see if the desired full-well mode is present (i.e. available). Note: Older detectors may not support the querying of features. In this case a value of -1 will be returned indicating that it is uknown whether the feature is present. In this case it is possible that the feature is present but that the detector is unable to report so. It may still be possible to use the feature in this case but no guarantees can be made on whether or not it will work. More... | |||||||||||
int | QueryBinningMode (bins flag) | ||||||||||
Query the detector to see if the desired binning mode is present (i.e. available). Note: Older detectors may not support the querying of features. In this case a value of -1 will be returned indicating that it is uknown whether the feature is present. In this case it is possible that the feature is present but that the detector is unable to report so. It may still be possible to use the feature in this case but no guarantees can be made on whether or not it will work. More... | |||||||||||
Protected Attributes | |
boost::shared_ptr< baseDetector > | base |
boost::shared_ptr< gigEDetector > | gigeDet |
boost::shared_ptr < camLinkDetector > | clDet |
Friends | |
class | baseBusScanner |
class | MockSetter |
class | DexelaDetectorPy |
class | Dex_CL |
Detailed Description
This class is used to control any interface-type Detector and acquire images from it. It will provide all the basic functionality required for all different Dexela detectors. For interface specific functionality please see the interface specific classes (e.g. DexelaDetectorGE, DexelaDetectorCL).
Constructor & Destructor Documentation
DexelaDetector::DexelaDetector | ( | DevInfo & | devInfo | ) |
Constructor for DexelaDetector. This version uses the DevInfo struct returned from a GetDevice, GetDeviceGE or GetDeviceCL call.
- Parameters
-
devInfo The DevInfo object for the desired detector.
- Exceptions
-
DexelaException
DexelaDetector::DexelaDetector | ( | DetectorInterface | transport, |
int | unit, | ||
const char * | params | ||
) |
Constructor for DexelaDetector. This version assumes you know the interface and the correct parameters to connect to the detector.
- Parameters
-
transport The DetectorInterface for the detector (i.e. CL or GIGE) unit The unit number for CL type detectors. For GIGE detectors this can be set to 0 params The parameter string for connection to the detector. For GIGE detectors this should be the detector IP address. For CL detectors this parameter will be ignored.
- Exceptions
-
DexelaException
|
virtual |
Destructor for DexelaDetector.
Member Function Documentation
void DexelaDetector::CheckForCallbackError | ( | ) |
This function will check to see if any errors have occurred in the background thread that is running when using callbacks. This thread is activated after a call to SetCallback and terminated with a call to StopCallback. If no error has occurred this method will just return. if an error has occurred a DexelaException will be thrown.
- Exceptions
-
DexelaException
void DexelaDetector::CheckForLiveError | ( | ) |
This function will check to see if any errors have occurred in the background thread that is running when using live-mode. This thread is activated after a call to GoLiveSeq and terminated with a call to GoUnLive. If no error has occurred this method will just return. if an error has occurred a DexelaException will be thrown.
- Exceptions
-
DexelaException
void DexelaDetector::ClearBuffers | ( | ) |
Clears all the camera buffers
- Exceptions
-
DexelaException
void DexelaDetector::ClearCameraBuffer | ( | int | i | ) |
Clears (i.e. zero-out) the specified camera buffer.
- Parameters
-
i The buffer number to clear.
- Exceptions
-
DexelaException
void DexelaDetector::CloseBoard | ( | ) |
Closes the connection to the detector.
- Exceptions
-
DexelaException
void DexelaDetector::DisablePulseGenerator | ( | ) |
This function will disable Pulse Generator mode. After calling this you should be able to use the SoftwareTrigger call.
- Exceptions
-
DexelaException
void DexelaDetector::EnableFrameCntWithinImage | ( | unsigned short | usEnable | ) |
Enables displaying the framecounter in the image (being the 2nd pixel).
- Parameters
-
usEnable Enables / disabled frame counter within image.
- Exceptions
-
DexelaException
void DexelaDetector::EnablePulseGenerator | ( | float | frequency | ) |
This function will enable the pulse generator software trigger signal. In this mode the software trigger can be continuously sent to the detector at the desired frequency.
Note: In order to use this mode the trigger source should be set to Internal_Software
Note2: To actually enable the pulse train you must call ToggleGenerator. A SoftwareTrigger call will not work when in this mode.
- Parameters
-
frequency The frequency that the software trigger signal will be run at.
- Exceptions
-
DexelaException
void DexelaDetector::EnablePulseGenerator | ( | ) |
This function is identical to EnablePulseGenerator, except that the frequency of the pulse train is set automatically. The frequency will be set such as to ensure continuous image acquisition for the current detector/binning mode.
Note: In order to use this mode the trigger source should be set to Internal_Software
Note2: To actually enable the pulse train you must call ToggleGenerator. A SoftwareTrigger call will not work when in this mode.
- Exceptions
-
DexelaException
void DexelaDetector::EnableROIMode | ( | bool | bEnableROI | ) |
This method activates or deactivates the ROI mode of the detector.
- Parameters
-
bEnableROI This parameter can have the following values:
value 0: Disable ROI mode
value 1: Enable ROI mode
- Exceptions
-
DexelaException
bins DexelaDetector::GetBinningMode | ( | ) |
Gets the current state of the detector binning mode
- Returns
- A member of the bins enumeration detailing the current detetor binning mode.
- Exceptions
-
DexelaException
int DexelaDetector::GetBufferXdim | ( | void | ) |
Get the x dimension of the transport buffer (in bytes)
- Returns
- The x dimension of the transport buffer (in bytes)
- Exceptions
-
DexelaException
int DexelaDetector::GetBufferYdim | ( | void | ) |
Get the y dimension of the transport buffer (in pixels)
- Returns
- The y dimension of the transport buffer (in pixels)
- Exceptions
-
DexelaException
int DexelaDetector::GetCapturedBuffer | ( | void | ) |
Gets the number of the buffer just captured. This can be used to determine which buffer to read-out.
- Returns
- The number of the last buffer, which the last captured image was written to.
- Exceptions
-
DexelaException
DetStatus DexelaDetector::GetDetectorStatus | ( | ) |
Returns the current settings of the detector in the form of a DetStatus object.
- Returns
- A DetStatus structure containing settings retrieved.
- Exceptions
-
DexelaException
ExposureModes DexelaDetector::GetExposureMode | ( | ) |
Gets the ExposureMode parameter of the detector.
- Returns
- The ExposureModes enumeration member that the detector is currently set to.
- Exceptions
-
DexelaException
float DexelaDetector::GetExposureTime | ( | ) |
Gets the exposure time parameter of the detector (ms).
- Returns
- The exposure time (ms) that the detector is currently set to.
- Exceptions
-
DexelaException
int DexelaDetector::GetFieldCount | ( | void | ) |
Gets the number of fields(frames) captured so far.
- Returns
- The number of fields captured.
- Exceptions
-
DexelaException
void DexelaDetector::GetFirmwareBuild | ( | int & | iDayAndMonth, |
int & | iYear, | ||
int & | iTime | ||
) |
Gets the detector firmware build date.
Note: This feature may not be supported on older detectors
- Parameters
-
iDayAndMonth The day and month of the firmware build (DDMM format) iYear The year of the firwmare build (YYYY format). iTime The time of the firmware build (hhmm format).
- Exceptions
-
DexelaException
int DexelaDetector::GetFirmwareVersion | ( | ) |
Gets the detector firmware version number.
- Returns
- The detector firmware version number as read from the detector.
- Exceptions
-
DexelaException
FullWellModes DexelaDetector::GetFullWellMode | ( | ) |
Gets the current detector well-mode.
- Returns
- A member of the FullWellModes enumeration detailing the current detetor Full-Well mode.
- Exceptions
-
DexelaException
float DexelaDetector::GetGapTime | ( | ) |
Gets the current gap-time setting of the detector. When run in Frame_Rate_exposure mode the detector will insert this gap period between consecutive frames in an image sequence.
Note: The minimum time for the gap-time setting is the current readout-time for the detector. Attempting to write anything smaller to the detector will result in a gap-time equal to the readout-time.
- Returns
- The gap-time in ms.
- Exceptions
-
DexelaException
int DexelaDetector::GetModelNumber | ( | ) |
Gets the detector model number.
- Returns
- The detector model number as read from the detector.
- Exceptions
-
DexelaException
int DexelaDetector::GetNumBuffers | ( | void | ) |
Get the number of internal buffers that are currently allocated for the detector.
- Returns
- The number of internal buffers allocated
- Exceptions
-
DexelaException
int DexelaDetector::GetNumOfExposures | ( | ) |
Gets the number of exposures setting from the detector. This is only relevant in Sequence_Exposure and Frame_Rate_exposure modes of operation.
- Returns
- The number of exposues that will be acquired each trigger recieved.
- Exceptions
-
DexelaException
ReadoutModes DexelaDetector::GetReadoutMode | ( | ) |
Gets the ReadoutModes parameter of the detector.
- Returns
- The ReadoutModes enumeration member that the detector is currently set to.
- Exceptions
-
DexelaException
double DexelaDetector::GetReadOutTime | ( | ) |
This method will return the read-out time of the detector (in ms) for it's current binning mode.
- Returns
- The read-out time (in ms) for the current settings of the detector.
- Exceptions
-
DexelaException
void DexelaDetector::GetROICoordinates | ( | unsigned short & | usStartColumn, |
unsigned short & | usStartRow, | ||
unsigned short & | usROIWidth, | ||
unsigned short & | usROIHeight | ||
) |
This method retrieves the coordinates of the region of interest (ROI) set within the detector.
- Parameters
-
usStartColumn Index of first column of ROI. usROIWidth Height (number of columns) of the ROI. usStartRow Index of first row of ROI. usROIHeight Height (number of rows) of the ROI.
- Exceptions
-
DexelaException
bool DexelaDetector::GetROIState | ( | ) |
This method retrieves the enabled state of the region of interest of the detector.
- Parameters
-
bEnableROI This parameter can have the following values:
value 0: ROI mode disabled
value 1: ROI mode enabled
- Exceptions
-
DexelaException
unsigned short DexelaDetector::GetSensorHeight | ( | unsigned short | uiSensorID = 1 | ) |
Gets the height of the sensor in pixels.
- Parameters
-
usSensorID ID of sensor FPGA to send the query to. For "global reads" sensor FPGA 1 has to be queried, using usSensorID=1/param> - Exceptions
-
DexelaException
unsigned short DexelaDetector::GetSensorWidth | ( | unsigned short | uiSensorID = 1 | ) |
Gets the width of the sensor in pixels.
- Parameters
-
usSensorID ID of sensor FPGA to send the query to. For "global reads" sensor FPGA 1 has to be queried, using usSensorID=1/param> - Exceptions
-
DexelaException
int DexelaDetector::GetSerialNumber | ( | ) |
Gets the detector serial number.
- Returns
- The detector serial number as read from the detector.
- Exceptions
-
DexelaException
BOOL DexelaDetector::GetTestMode | ( | ) |
Gets the current state of the detector test mode (on/off)
- Returns
- Boolean value containing the current state of the detector test mode.
- Exceptions
-
DexelaException
DetectorInterface DexelaDetector::GetTransportMethod | ( | ) |
Returns the communication method (i.e. interface) for the detector object.
- Returns
- The DetectorInterface enumeration used by the detector
- Exceptions
-
DexelaException
ExposureTriggerSource DexelaDetector::GetTriggerSource | ( | ) |
Gets the current trigger source setting from the detector
- Returns
- A member of the ExposureTriggerSource enumeration detailing the current trigger source of the detector.
- Exceptions
-
DexelaException
void DexelaDetector::GoLiveSeq | ( | int | start, |
int | stop, | ||
int | numBuf | ||
) |
Sets the host computer up to be ready to recieve images into the specified buffer range.
- Parameters
-
start Number of the first buffer to use for acquisition stop Number of the last buffer to use for acquisition numBuf Number of frames to acquire. If this is set to 0 the buffer will be circular (i.e. ring-buffer).
- Exceptions
-
DexelaException
void DexelaDetector::GoLiveSeq | ( | ) |
Sets the host computer up to be ready to recieve images. This call will use all available buffers in a circular fashion (i.e. ring-buffer).
- Exceptions
-
DexelaException
void DexelaDetector::GoUnLive | ( | ) |
Exits live mode. The host computer will no longer be ready to recieve transmitted images.
- Exceptions
-
DexelaException
bool DexelaDetector::IsCallbackActive | ( | ) |
This method will inform the user if the callback mode (i.e. background thread) is currently active.
- Returns
- A boolean value indicating whether the callback mode is active.
- Exceptions
-
DexelaException
bool DexelaDetector::IsConnected | ( | ) |
Check to see if the connection to the detector is open (i.e. OpenBoard)
- Returns
- A boolean indicating whether the connection to detector is open.
- Exceptions
-
DexelaException
bool DexelaDetector::IsFrameCntWithinImage | ( | ) |
Checks if framecounter is displayed within the image data (being the 2nd pixel).
- Parameters
-
usEnable 0 if not enabled; 1 if enabled
- Exceptions
-
DexelaException
bool DexelaDetector::IsLive | ( | ) |
This method will inform the user if detector is currently in Live mode.
- Returns
- A boolean value indicating whether the detector is in Live mode.
- Exceptions
-
DexelaException
void DexelaDetector::LoadSensorConfigFile | ( | char * | filename | ) |
Loads the sensor configuration file into the detector. This file will write values to the ADC offset registers for each sensor in the detector.
- Parameters
-
filename The path to the sensor configuration to use.
- Exceptions
-
DexelaException
|
virtual |
Opens the connection to the detector. Every open should be matched with a close to free resources.
- Exceptions
-
DexelaException
Reimplemented in DexelaDetectorCL, and DexelaDetectorGE.
void DexelaDetector::OpenBoard | ( | int | NumBufs | ) |
Opens the connection to the detector and sets the number of buffers to use/allocate. Every open should be matched with a close to free resources.
- Parameters
-
NumBufs Number of buffers to use/allocate
- Exceptions
-
DexelaException
int DexelaDetector::QueryBinningMode | ( | bins | flag | ) |
Query the detector to see if the desired binning mode is present (i.e. available).
Note: Older detectors may not support the querying of features. In this case a value of -1 will be returned indicating that it is uknown whether the feature is present. In this case it is possible that the feature is present but that the detector is unable to report so. It may still be possible to use the feature in this case but no guarantees can be made on whether or not it will work.
- Parameters
-
mode The bins enumeration member to be checked for.
- Returns
- An integer value representing whether the feature is present. A value of 1 indicates that the feature is present. A value of 0 indicates that the feature is not present. A value of -1 indicates that it is uknown whether the feature is presetn.
- Exceptions
-
DexelaException
int DexelaDetector::QueryExposureMode | ( | ExposureModes | mode | ) |
Query the detector to see if the desired exposure mode is present (i.e. available).
Note: Older detectors may not support the querying of features. In this case a value of -1 will be returned indicating that it is uknown whether the feature is present. In this case it is possible that the feature is present but that the detector is unable to report so. It may still be possible to use the feature in this case but no guarantees can be made on whether or not it will work.
- Parameters
-
mode The ExposureModes enumeration member to be checked for.
- Returns
- An integer value representing whether the feature is present. A value of 1 indicates that the feature is present. A value of 0 indicates that the feature is not present. A value of -1 indicates that it is uknown whether the feature is presetn.
- Exceptions
-
DexelaException
int DexelaDetector::QueryFullWellMode | ( | FullWellModes | fwm | ) |
Query the detector to see if the desired full-well mode is present (i.e. available).
Note: Older detectors may not support the querying of features. In this case a value of -1 will be returned indicating that it is uknown whether the feature is present. In this case it is possible that the feature is present but that the detector is unable to report so. It may still be possible to use the feature in this case but no guarantees can be made on whether or not it will work.
- Parameters
-
mode The FullWellModes enumeration member to be checked for.
- Returns
- An integer value representing whether the feature is present. A value of 1 indicates that the feature is present. A value of 0 indicates that the feature is not present. A value of -1 indicates that it is uknown whether the feature is presetn.
- Exceptions
-
DexelaException
int DexelaDetector::QueryReadoutMode | ( | ReadoutModes | mode | ) |
Query the detector to see if the desired readout mode is present (i.e. available).
Note: Older detectors may not support the querying of features. In this case a value of -1 will be returned indicating that it is uknown whether the feature is present. In this case it is possible that the feature is present but that the detector is unable to report so. It may still be possible to use the feature in this case but no guarantees can be made on whether or not it will work.
- Parameters
-
mode The ReadoutModes enumeration member to be checked for.
- Returns
- An integer value representing whether the feature is present. A value of 1 indicates that the feature is present. A value of 0 indicates that the feature is not present. A value of -1 indicates that it is uknown whether the feature is presetn.
- Exceptions
-
DexelaException
int DexelaDetector::QueryTriggerSource | ( | ExposureTriggerSource | ets | ) |
Query the detector to see if the desired trigger source is present (i.e. available).
Note: Older detectors may not support the querying of features. In this case a value of -1 will be returned indicating that it is uknown whether the feature is present. In this case it is possible that the feature is present but that the detector is unable to report so. It may still be possible to use the feature in this case but no guarantees can be made on whether or not it will work.
- Parameters
-
mode The ExposureTriggerSource enumeration member to be checked for.
- Returns
- An integer value representing whether the feature is present. A value of 1 indicates that the feature is present. A value of 0 indicates that the feature is not present. A value of -1 indicates that it is uknown whether the feature is presetn.
- Exceptions
-
DexelaException
void DexelaDetector::ReadBuffer | ( | int | bufNum, |
byte * | buffer | ||
) |
Reads the specified transport buffer into the passed in buffer (byte*).
Note: GetCapturedBuffer can be used to get the number of the lastest buffer to be filled.
- Parameters
-
bufNum The index of the transport buffer to read from. buffer The user-created (byte*) buffer to write the image to.
- Exceptions
-
DexelaException
void DexelaDetector::ReadBuffer | ( | int | bufNum, |
DexImage & | img, | ||
int | iZ = 0 |
||
) |
Reads the specified transport buffer into the passed in DexImage object at the passed in plane.
Note: GetCapturedBuffer can be used to get the number of the lastest buffer to be filled.
- Parameters
-
bufNum The index of the transport buffer to read from. img The DexImage object that the image will be written into iZ The plane that the image should be written to in the DexImage object (defaults to 0).
- Exceptions
-
DexelaException
int DexelaDetector::ReadRegister | ( | int | address, |
int | sensorNum = 1 |
||
) |
Reads the specified register from the detector. The sensor number corresponds to the desired sensor from which to read the register. The SensorNumber will default to 1 (master-sensor) if not specified otherwise by the user.
- Parameters
-
address The address of the desired register to read. sensorNum The sensor number from which to read the register (defaults to master-sensor).
- Returns
- The integer value of the register.
- Exceptions
-
DexelaException
void DexelaDetector::SetBinningMode | ( | bins | flag | ) |
Sets the binning mode of the detector.
- Parameters
-
flag The bins enumeration member to be set.
- Exceptions
-
DexelaException
void DexelaDetector::SetCallback | ( | IMAGE_CALLBACK | func | ) |
Sets the user defined callback funtction to be called for every image arrival event.
- Parameters
-
func The call back function (IMAGE_CALLBACK) to be called for every image arrival event.
- Exceptions
-
DexelaException
void DexelaDetector::SetExposureMode | ( | ExposureModes | mode | ) |
Sets the ExposureMode parameter of the detector
- Parameters
-
mode The ExposureModes enumeration member to be set.
- Exceptions
-
DexelaException
void DexelaDetector::SetExposureTime | ( | float | timems | ) |
Sets the exposure time parameter of the detector
- Parameters
-
timems The exposure time (in milliseconds) to be set. Note: if you attempt to set an exposure time smaller then the current read-out time for the detector, it will be set to minimum.
- Exceptions
-
DexelaException
void DexelaDetector::SetFullWellMode | ( | FullWellModes | fwm | ) |
Sets the full well mode parameter of the detector.
- Parameters
-
fwm The FullWellModes enumeration member to be set.
- Exceptions
-
DexelaException
void DexelaDetector::SetGapTime | ( | float | timems | ) |
Sets the gap-time setting of the detector. When run in Frame_Rate_exposure mode the detector will insert this gap period between consecutive frames in an image sequence.
Note: The minimum time for the gap-time setting is the current readout-time for the detector. Attempting to write anything smaller to the detector will result in a gap-time equal to the readout-time.
- Parameters
-
timems The gap-time in ms.
- Exceptions
-
DexelaException
void DexelaDetector::SetNumOfExposures | ( | int | num | ) |
Sets the number of exposures to acquire after a trigger. This is only relevant in Sequence_Exposure and Frame_Rate_exposure modes of operation.
- Parameters
-
num The number of exposues to acquire for each trigger recieved.
- Exceptions
-
DexelaException
void DexelaDetector::SetPreProgrammedExposureTimes | ( | int | numExposures, |
float * | exposuretimes_ms | ||
) |
This method will set the exposure times for pre-programmed exposure mode.
- Parameters
-
numExposures The number of exposures to set. This number should correspond to the number of exposures that will be acquired in pre-programmed exposure mode and should be between 2-4. exposuretimes_ms An array of floating point numbers representing the exposure times for pre-programmed exposure mode. The number of exposure times should correspond to the numExposures parameter.
- Exceptions
-
DexelaException
void DexelaDetector::SetReadoutMode | ( | ReadoutModes | mode | ) |
Sets the ReadoutMode parameter of the detector
- Parameters
-
mode The ReadoutModes enumeration member to be set.
- Exceptions
-
DexelaException
void DexelaDetector::SetSlowed | ( | bool | flag | ) |
This method can specify to the api that the detector being used is a slowed-down detector (e.g. mammo detector). This should not be necessary as the API should be able to determine most of the time whether the firmwarwe version is a slowed down one. However, for certain older detectors/firmwares this may not be possible. In this case this method can be used to inform the library that the firmware is slowed down and it will use the correct read-out times.
- Parameters
-
numExposures A boolean flag indicating wheter the detector is slowed down or not.
- Exceptions
-
DexelaException
void DexelaDetector::SetTestMode | ( | BOOL | SetTestOn | ) |
Enables/disables test mode. The detector will output a generated test pattern if this mode is turned on.
- Parameters
-
SetTestOn if set to true
The test pattern is turned on.
- Exceptions
-
DexelaException
void DexelaDetector::SetTriggerSource | ( | ExposureTriggerSource | ets | ) |
Sets the trigger source setting on the detector
- Parameters
-
ets A member of the ExposureTriggerSource enumeration to be set to the detector
- Exceptions
-
DexelaException
void DexelaDetector::Snap | ( | int | buffer, |
int | timeout | ||
) |
Snaps an image into the specified buffer.
Note: If the detector trigger source is set to Internal_Software, this call will automatically trigger the detector.
- Parameters
-
buffer The buffer number to snap to. The number of available buffers can be found by calling the GetNumBuffers method. timeout The amount of time (in ms) that the library will wait for an image before throwing a timeout exception.
- Exceptions
-
DexelaException
void DexelaDetector::SoftReset | ( | void | ) |
Cycles the power on the detector
- Exceptions
-
DexelaException
void DexelaDetector::SoftwareTrigger | ( | ) |
Sends a trigger to the detector (will only work if the trigger source is set to Internal_Software)
- Exceptions
-
DexelaException
void DexelaDetector::StopCallback | ( | ) |
This function will terminate the callback loop (i.e. will wait for all spawned threads to finish exectuing).
- Exceptions
-
DexelaException
void DexelaDetector::ToggleGenerator | ( | BOOL | onOff | ) |
This function will control the pulse train.
Note: In order to use this mode the pulse generator must be enabled. See EnablePulseGenerator.
- Parameters
-
onOff Boolean that will control the state of the pulse train (true = on, false = off).
- Exceptions
-
DexelaException
void DexelaDetector::WaitImage | ( | int | timeout | ) |
This function will wait for the specified amount of time for an image to arrive.
Note: If the image arrives before then it will return as soon as it does (i.e. it won't wait for the duration of the timeout period). If the image does not arrive in the specified time a DexelaException will be thrown.
- Parameters
-
timeout The timeout period (in ms) for which to wait before throwing a DexelaException.
- Exceptions
-
DexelaException
void DexelaDetector::WriteBuffer | ( | int | bufNum, |
byte * | buffer | ||
) |
Writes data to the specified transport buffer.
- Parameters
-
bufNum The index of the transport buffer to write to. buffer The user-created data (byte*) buffer to write to the transport buffer.
- Exceptions
-
DexelaException
void DexelaDetector::WriteRegister | ( | int | address, |
int | value, | ||
int | sensorNum = 0 |
||
) |
Writes the value to the specified register from the detector. The sensor number corresponds to the desired sensor to which the value will be written. The SensorNumber will default to 0 (broadcast to all sensors) if not specified otherwise by the user.
- Parameters
-
address The address of the desired register to write to.
+
- Parameters
-
value The value to write into the register sensorNum The sensor number that the register will be written to. This defaults to 0 which is a broadcast to all detector sensors.
- Exceptions
-
DexelaException
The documentation for this class was generated from the following files:
- DexelaDetector.h
- DexelaDetector.cpp
Generated on Tue Nov 25 2014 10:22:44 for DexelaDetector API by
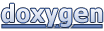