Bluetooth® Sample Application Programmer's Reference
|
Presentation
UserInterface.c/h contains the functions that are used for communication testing
Functions | |
T_GL_HGRAPHIC_LIB | guiStart (void) |
void | guiStop (void) |
int | guiScreen (char *title, char *text, int icon, int delay) |
int | guiScreenSize (int *x, int *y) |
int | guiMenu (int type, int sta, const char *hdr, const char **mnu) |
void | guiWidgetText (const char *text, int delay) |
int | guiDrawCreate (void) |
void | guiDrawDisplay (void) |
void | guiDrawText (short x, short y, unsigned short width, unsigned short height, char *text) |
void | guiDrawImage (short x, short y, const char *filename) |
void | guiDrawDestroy (void) |
unsigned long | guiImageDisplay (char *title, char *text, char *buf, int delay) |
unsigned long | guiProgressDisplay (char *title, char *text, char *loc, progressUpdate sta, int delay) |
unsigned long | guiMediaDisplay (char *title, char *text, char *loc, int vol, int delay) |
unsigned long | guiSliderDisplay (char *title, char *text, char *loc, short *val, sliderUpdate sta, int delay) |
unsigned long | enterStr (char *title, char *text, char *buf, int len) |
unsigned long | enterPwd (char *title, char *text, char *buf, int len) |
unsigned long | enterPwdTxt (char *title, char *text, char *buf, int len) |
unsigned long | enterAmt (char *title, char *text, char *buf, int len, int exp) |
unsigned long | enterTcp (char *title, char *text, char *buf, int len) |
unsigned long | enterTxt (char *title, char *text, char *buf, int len) |
unsigned long | enterDate (char *title, char *text, char *buf, int len) |
unsigned long | enterTime (char *title, char *text, char *buf, int len) |
Macros | |
#define | WORDHL(H, L) ((unsigned short)((((unsigned short)H)<<8)|((unsigned short)L))) |
Combine two bytes into a word. More... | |
#define | HBYTE(W) (unsigned char)(((unsigned short)W&0xFF00)>>8) |
Extract highest byte from a word. More... | |
#define | LBYTE(W) (unsigned char)((unsigned short)W&0x00FF) |
Extract lowest byte from a word. More... | |
#define | CARDHL(H, L) ((unsigned long)((((unsigned long)H)<<16)|((unsigned long)L))) |
Combine two words into a card. More... | |
#define | HWORD(C) (unsigned short)(((unsigned long)C&0xFFFF0000UL)>>16) |
Extract highest word from a card. More... | |
#define | LWORD(C) (unsigned short)((unsigned long)C&0x0000FFFFUL) |
Extract lowest word from a card. More... | |
Typedefs | |
typedef struct sGoalRange | tGoalRange |
typedef short(* | progressUpdate )(short val) |
virtual method function for progress bar More... | |
typedef void(* | sliderUpdate )(tGoalRange range) |
virtual method function for slider More... | |
Enumerations | |
enum | eMnuType { mnuTypeBeg, mnuTypeDialog, mnuTypeIcon, mnuTypeButton, mnuTypeEnd } |
Function Documentation
T_GL_HGRAPHIC_LIB guiStart | ( | void | ) |
Open the associated channel. Should be called before calling any GUI/GOAL Functions.
- Returns
- GOAL Graphic Library handler
Utilities.h UserInterface.c
void guiStop | ( | void | ) |
int guiScreen | ( | char * | title, |
char * | text, | ||
int | icon, | ||
int | delay | ||
) |
Dialog to display Info screen
- Parameters
-
title (I) title ofthe screen text (I) text to be displayed on the screen icon (I) Icon to be displayed with the message delay (I) Timeout delay
- Returns
- key returned
Utilities.h UserInterface.c
int guiScreenSize | ( | int * | x, |
int * | y | ||
) |
Obtain the screen size in pixels
- Parameters
-
x (O) width of the screen y (O) height of the screen
- Returns
- key returned
Utilities.h UserInterface.c
int guiMenu | ( | int | type, |
int | sta, | ||
const char * | hdr, | ||
const char ** | mnu | ||
) |
Display GOAL Menu Dialog There are 3 kinds of GOAL Menu displays
- ICON MENU
- ICON BUTTON MENU and
- DIALOG MENU
- Parameters
-
type (I) menu type hdr (I) The menu header mnu (I) menu items sta (I) current menu item selected
- Returns
- key returned
Utilities.h UserInterface.c
void guiWidgetText | ( | const char * | text, |
int | delay | ||
) |
Display a widget text
Utilities.h UserInterface.c
int guiDrawCreate | ( | void | ) |
Create a drawing surface
- Returns
- 0 if ok, negative otherwise Utilities.h UserInterface.c
void guiDrawDisplay | ( | void | ) |
Display a drawing surface
Utilities.h UserInterface.c
void guiDrawText | ( | short | x, |
short | y, | ||
unsigned short | width, | ||
unsigned short | height, | ||
char * | text | ||
) |
Add a text to the drawing surface
- Parameters
-
x (I) horizontal location on the screen y (I) vertical location on the screen width (I) width of the text height (I) height of the text text (I) text to be displayed
Utilities.h UserInterface.c
void guiDrawImage | ( | short | x, |
short | y, | ||
const char * | filename | ||
) |
Add an image to the drawing surface
- Parameters
-
x (I) horizontal location on the screen y (I) vertical location on the screen filename (I) location of the image file to be displayed
Utilities.h UserInterface.c
void guiDrawDestroy | ( | void | ) |
Destroy the drawing surface
Utilities.h UserInterface.c
unsigned long guiImageDisplay | ( | char * | title, |
char * | text, | ||
char * | buf, | ||
int | delay | ||
) |
Dialog to display image file
- Parameters
-
title (I) header to be displayed text (I) text to be displayed buf (I) location of the file to be displayed delay (I) Timeout delay
- Returns
- key entered
Utilities.h UserInterface.c
unsigned long guiProgressDisplay | ( | char * | title, |
char * | text, | ||
char * | loc, | ||
progressUpdate | sta, | ||
int | delay | ||
) |
Dialog to display progress bar
- Parameters
-
title (I) header to be displayed text (I) text to be displayed loc (I) location of the media file to be displayed above the progress bar sta (I) virtual function to update the status of the progress bar delay (I) Timeout delay
- Returns
- key entered
Utilities.h UserInterface.c
unsigned long guiMediaDisplay | ( | char * | title, |
char * | text, | ||
char * | loc, | ||
int | vol, | ||
int | delay | ||
) |
Dialog to display a multimedia file
- Parameters
-
title (I) header to be displayed text (I) text to be displayed loc (I) location of the media file to be played vol (I) volume of the media file to be played (0-255) delay (I) Timeout delay
- Returns
- key entered
Utilities.h UserInterface.c
unsigned long guiSliderDisplay | ( | char * | title, |
char * | text, | ||
char * | loc, | ||
short * | val, | ||
sliderUpdate | sta, | ||
int | delay | ||
) |
Dialog to display slider bar
- Parameters
-
title (I) header to be displayed text (I) text to be displayed loc (I) location of the media file to be displayed above the slider bar val (O) location of the slider sta (O) status of the slider delay (I) Timeout delay
- Returns
- key entered
Utilities.h UserInterface.c
unsigned long enterStr | ( | char * | title, |
char * | text, | ||
char * | buf, | ||
int | len | ||
) |
Dialog for string input If during waiting a key is pressed the function returns the code of the key pressed.
- Parameters
-
title (I) title ofthe screen text (I) text to be displayed on the screen buf (I) input buffer len (I) size of the input buffer
- Returns
- the key or button pressed Utilities.h UserInterface.c
unsigned long enterPwd | ( | char * | title, |
char * | text, | ||
char * | buf, | ||
int | len | ||
) |
Dialog for numeric password input If during waiting a key is pressed the function returns the code of the key pressed.
- Parameters
-
title (I) title ofthe screen text (I) text to be displayed on the screen buf (I) input buffer len (I) size of the input buffer
- Returns
- the key or button pressed Utilities.h UserInterface.c
unsigned long enterPwdTxt | ( | char * | title, |
char * | text, | ||
char * | buf, | ||
int | len | ||
) |
Dialog for alphanumeric password input If during waiting a key is pressed the function returns the code of the key pressed.
- Parameters
-
title (I) title ofthe screen text (I) text to be displayed on the screen buf (I) input buffer len (I) size of the input buffer
- Returns
- the key or button pressed Utilities.h UserInterface.c
unsigned long enterAmt | ( | char * | title, |
char * | text, | ||
char * | buf, | ||
int | len, | ||
int | exp | ||
) |
Dialog for amount input If during waiting a key is pressed the function returns the code of the key pressed.
- Parameters
-
title (I) title ofthe screen text (I) text to be displayed on the screen buf (I) input buffer len (I) size of the input buffer exp (I) number of decimal places
- Returns
- the key or button pressed Utilities.h UserInterface.c
unsigned long enterTcp | ( | char * | title, |
char * | text, | ||
char * | buf, | ||
int | len | ||
) |
Dialog for IP adddress input If during waiting a key is pressed the function returns the code of the key pressed.
- Parameters
-
title (I) title ofthe screen text (I) text to be displayed on the screen buf (I) input buffer len (I) size of the input buffer
- Returns
- the key or button pressed Utilities.h UserInterface.c
unsigned long enterTxt | ( | char * | title, |
char * | text, | ||
char * | buf, | ||
int | len | ||
) |
Dialog for text input If during waiting a key is pressed the function returns the code of the key pressed.
- Parameters
-
title (I) title ofthe screen text (I) text to be displayed on the screen buf (I) input buffer len (I) size of the input buffer
- Returns
- the key or button pressed Utilities.h UserInterface.c
unsigned long enterDate | ( | char * | title, |
char * | text, | ||
char * | buf, | ||
int | len | ||
) |
Date Input If during waiting a key is pressed the function returns the code of the key pressed.
- Parameters
-
title (I) title ofthe screen text (I) text to be displayed on the screen buf (I) input buffer len (I) size of the input buffer
- Returns
- the key or button pressed Utilities.h UserInterface.c
unsigned long enterTime | ( | char * | title, |
char * | text, | ||
char * | buf, | ||
int | len | ||
) |
Time Input If during waiting a key is pressed the function returns the code of the key pressed.
- Parameters
-
title (I) title ofthe screen text (I) text to be displayed on the screen buf (I) input buffer len (I) size of the input buffer
- Returns
- the key or button pressed Utilities.h UserInterface.c
Macro Definition Documentation
#define WORDHL | ( | H, | |
L | |||
) | ((unsigned short)((((unsigned short)H)<<8)|((unsigned short)L))) |
Combine two bytes into a word.
#define HBYTE | ( | W | ) | (unsigned char)(((unsigned short)W&0xFF00)>>8) |
Extract highest byte from a word.
#define LBYTE | ( | W | ) | (unsigned char)((unsigned short)W&0x00FF) |
Extract lowest byte from a word.
#define CARDHL | ( | H, | |
L | |||
) | ((unsigned long)((((unsigned long)H)<<16)|((unsigned long)L))) |
Combine two words into a card.
#define HWORD | ( | C | ) | (unsigned short)(((unsigned long)C&0xFFFF0000UL)>>16) |
Extract highest word from a card.
#define LWORD | ( | C | ) | (unsigned short)((unsigned long)C&0x0000FFFFUL) |
Extract lowest word from a card.
Typedef Documentation
typedef struct sGoalRange tGoalRange |
typedef short(* progressUpdate)(short val) |
virtual method function for progress bar
typedef void(* sliderUpdate)(tGoalRange range) |
virtual method function for slider
Enumeration Type Documentation
enum eMnuType |
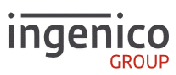