Bluetooth® Sample Application Programmer's Reference
|
Utilities.h File Reference
#include <GL_GraphicLib.h>
Functions | |
int | magStart (void) |
start the magstripe perypheral More... | |
int | magStop (void) |
stop the magstripe perypheral More... | |
int | magGet (char *trk1, char *trk2, char *trk3) |
get magstripe tracks More... | |
int | getCard (unsigned char *buf, const char *ctl) |
process event card input More... | |
void | detectCard (void) |
detect if chip card is inserted More... | |
int | iccStart (unsigned char rdr) |
Open Integrated Circuit Card perypheral. More... | |
int | iccStop (unsigned char rdr) |
Close Integrated Circuit Card perypheral. More... | |
int | iccDetect (unsigned char rdr) |
Detect card. More... | |
int | iccCommand (unsigned char rdr, const unsigned char *cmd, const unsigned char *dat, unsigned char *rsp) |
Send a command and data to a smart card and retrieve the response. More... | |
int | prtStart (void) |
Start printer perypheral. More... | |
int | prtStop (void) |
Stop printer perypheral. More... | |
int | prtCtrl (void) |
Control paper presence. More... | |
int | dspStart (void) |
Start display perypheral. More... | |
int | dspStop (void) |
Stop display perypheral. More... | |
int | kbdStart (unsigned char fls) |
start waiting for a key More... | |
int | kbdStop (void) |
stop waiting for a key More... | |
char | kbdKey (void) |
get a key pressed More... | |
int | tftStart (void) |
int | tftStop (void) |
int | tmrStart (unsigned char tmr, int dly) |
start the timer tmr for dly centiseconds More... | |
int | tmrGet (unsigned char tmr) |
get the number of centiseconds waitng for timer tmr More... | |
void | tmrStop (unsigned char tmr) |
stop the timer tmr More... | |
int | printText (const char *str) |
int | printSizeSet (eTextSize size) |
int | printAlignSet (eTextAlign size) |
void | printDocument (void) |
void | stateNext (void) |
void | statePrev (void) |
void | stateSet (int nextState) |
int | stateGet (void) |
T_GL_HGRAPHIC_LIB | guiStart (void) |
void | guiStop (void) |
int | guiScreen (char *title, char *text, int icon, int delay) |
int | guiScreenSize (int *x, int *y) |
int | guiMenu (int type, int sta, const char *hdr, const char **mnu) |
void | guiWidgetText (const char *text, int delay) |
int | guiDrawCreate (void) |
void | guiDrawDisplay (void) |
void | guiDrawText (short x, short y, unsigned short width, unsigned short height, char *text) |
void | guiDrawImage (short x, short y, const char *filename) |
void | guiDrawDestroy (void) |
unsigned long | guiImageDisplay (char *title, char *text, char *buf, int delay) |
unsigned long | guiProgressDisplay (char *title, char *text, char *loc, progressUpdate sta, int delay) |
unsigned long | guiMediaDisplay (char *title, char *text, char *loc, int vol, int delay) |
unsigned long | guiSliderDisplay (char *title, char *text, char *loc, short *val, sliderUpdate sta, int delay) |
unsigned long | enterStr (char *title, char *text, char *buf, int len) |
unsigned long | enterPwd (char *title, char *text, char *buf, int len) |
unsigned long | enterPwdTxt (char *title, char *text, char *buf, int len) |
unsigned long | enterAmt (char *title, char *text, char *buf, int len, int exp) |
unsigned long | enterTcp (char *title, char *text, char *buf, int len) |
unsigned long | enterTxt (char *title, char *text, char *buf, int len) |
unsigned long | enterDate (char *title, char *text, char *buf, int len) |
unsigned long | enterTime (char *title, char *text, char *buf, int len) |
Macros | |
#define | CHECK(CND, LBL) {if(!(CND)){goto LBL;}} |
#define | VERIFY(CND) {if(!(CND)){}} |
#define | CHK(CND, LBL) {if(!(CND)) goto LBL;} |
#define | BIT(B) (0x01<<(B-1)) |
#define | trcS(s) {} |
#define | trcFL(f, l) {} |
#define | trcFS(f, s) {} |
#define | trcFN(f, n) {} |
#define | trcBuf(b, n) {} |
#define | trcBN(b, n) {} |
#define | trcAN(b, n) {} |
#define | trcBAN(b, n) {} |
#define | trcErr(n) {} |
#define | WORDHL(H, L) ((unsigned short)((((unsigned short)H)<<8)|((unsigned short)L))) |
Combine two bytes into a word. More... | |
#define | HBYTE(W) (unsigned char)(((unsigned short)W&0xFF00)>>8) |
Extract highest byte from a word. More... | |
#define | LBYTE(W) (unsigned char)((unsigned short)W&0x00FF) |
Extract lowest byte from a word. More... | |
#define | CARDHL(H, L) ((unsigned long)((((unsigned long)H)<<16)|((unsigned long)L))) |
Combine two words into a card. More... | |
#define | HWORD(C) (unsigned short)(((unsigned long)C&0xFFFF0000UL)>>16) |
Extract highest word from a card. More... | |
#define | LWORD(C) (unsigned short)((unsigned long)C&0x0000FFFFUL) |
Extract lowest word from a card. More... | |
Typedefs | |
typedef enum eTextSize | eTextSize |
typedef enum eTextAlign | eTextAlign |
typedef struct sGoalRange | tGoalRange |
typedef short(* | progressUpdate )(short val) |
virtual method function for progress bar More... | |
typedef void(* | sliderUpdate )(tGoalRange range) |
virtual method function for slider More... | |
Enumerations | |
enum | eIcc { iccBeg, iccCardRemoved, iccCardMute, iccCardPb, iccDriverPb, iccReadFailure, iccKO } |
enum | eTextSize { textSizeXXSmall = 0x3FF0, textSizeXSmall = 0x3FF1, textSizeSmall = 0x3FF2, textSizeMedium = 0x3FF3, textSizeLarge = 0x3FF4, textSizeXLarge = 0x3FF5, textSizeXXLarge =0x3FF6 } |
enum | eTextAlign { textAlignCenter = 0x00, textAlignLeft = 0x01, textAlignRight = 0x02, textAlignTop = 0x04, textAlignBottom = 0x08 } |
enum | { STATE_OK, STATE_NEXT, STATE_BACK, STATE_SET, STATE_ABORT, STATE_FAILED = -1 } |
enum | eMnuType { mnuTypeBeg, mnuTypeDialog, mnuTypeIcon, mnuTypeButton, mnuTypeEnd } |
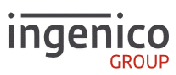