![]() |
BlueNRG-MS pack for STM32CubeMX
V4.4.0
The BlueNRG-MS pack is an additional software for STM32CubeMX.
|
ble_list.h
Go to the documentation of this file.
struct _tListNode tListNode
void list_remove_tail(tListNode *listHead, tListNode **node)
Definition: ble_list.c:109
void list_remove_head(tListNode *listHead, tListNode **node)
Definition: ble_list.c:95
void list_get_next_node(tListNode *ref_node, tListNode **node)
Definition: ble_list.c:172
Definition: ble_list.h:20
void list_get_prev_node(tListNode *ref_node, tListNode **node)
Definition: ble_list.c:183
void list_insert_node_before(tListNode *node, tListNode *ref_node)
Definition: ble_list.c:137
void list_insert_head(tListNode *listHead, tListNode *node)
Definition: ble_list.c:55
void list_insert_tail(tListNode *listHead, tListNode *node)
Definition: ble_list.c:69
struct _tListNode * pListNode
void list_insert_node_after(tListNode *node, tListNode *ref_node)
Definition: ble_list.c:123
Generated on Mon Apr 15 2019 18:10:39 for BlueNRG-MS pack for STM32CubeMX by
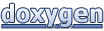