Ap4RtpHint.h
Go to the documentation of this file.00001 /***************************************************************** 00002 | 00003 | AP4 - RTP Hint Objects 00004 | 00005 | Copyright 2002-2008 Axiomatic Systems, LLC 00006 | 00007 | 00008 | This file is part of Bento4/AP4 (MP4 Atom Processing Library). 00009 | 00010 | Unless you have obtained Bento4 under a difference license, 00011 | this version of Bento4 is Bento4|GPL. 00012 | Bento4|GPL is free software; you can redistribute it and/or modify 00013 | it under the terms of the GNU General Public License as published by 00014 | the Free Software Foundation; either version 2, or (at your option) 00015 | any later version. 00016 | 00017 | Bento4|GPL is distributed in the hope that it will be useful, 00018 | but WITHOUT ANY WARRANTY; without even the implied warranty of 00019 | MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00020 | GNU General Public License for more details. 00021 | 00022 | You should have received a copy of the GNU General Public License 00023 | along with Bento4|GPL; see the file COPYING. If not, write to the 00024 | Free Software Foundation, 59 Temple Place - Suite 330, Boston, MA 00025 | 02111-1307, USA. 00026 | 00027 ****************************************************************/ 00028 00029 #ifndef _AP4_RTP_HINT_H_ 00030 #define _AP4_RTP_HINT_H_ 00031 00032 /*---------------------------------------------------------------------- 00033 | includes 00034 +---------------------------------------------------------------------*/ 00035 #include "Ap4Types.h" 00036 #include "Ap4List.h" 00037 #include "Ap4DataBuffer.h" 00038 #include "Ap4Interfaces.h" 00039 00040 /*---------------------------------------------------------------------- 00041 | forward declarations 00042 +---------------------------------------------------------------------*/ 00043 class AP4_ByteStream; 00044 class AP4_RtpConstructor; 00045 class AP4_RtpPacket; 00046 00047 /*---------------------------------------------------------------------- 00048 | AP4_RtpSampleData 00049 +---------------------------------------------------------------------*/ 00050 class AP4_RtpSampleData 00051 { 00052 public: 00053 // constructors and destructor 00054 AP4_RtpSampleData(AP4_ByteStream& stream, AP4_UI32 size); 00055 AP4_RtpSampleData() {} 00056 virtual ~AP4_RtpSampleData(); 00057 00058 // methods 00059 virtual AP4_Result AddPacket(AP4_RtpPacket* packet); 00060 virtual AP4_Size GetSize(); 00061 virtual AP4_ByteStream* ToByteStream(); 00062 00063 // accessors 00064 AP4_List<AP4_RtpPacket>& GetPackets() { 00065 return m_Packets; 00066 } 00067 const AP4_DataBuffer& GetExtraData() const { 00068 return m_ExtraData; 00069 } 00070 00071 protected: 00072 // members 00073 AP4_List<AP4_RtpPacket> m_Packets; 00074 AP4_DataBuffer m_ExtraData; 00075 }; 00076 00077 /*---------------------------------------------------------------------- 00078 | AP4_RtpPacket 00079 +---------------------------------------------------------------------*/ 00080 class AP4_RtpPacket : public AP4_Referenceable 00081 { 00082 public: 00083 // constructor and destructor 00084 AP4_RtpPacket(AP4_ByteStream& stream); 00085 AP4_RtpPacket(int relative_time, 00086 bool p_bit, 00087 bool x_bit, 00088 bool m_bit, 00089 AP4_UI08 payload_type, 00090 AP4_UI16 sequence_seed, 00091 int time_stamp_offset = 0, 00092 bool bframe_flag = false, 00093 bool repeat_flag = false); 00094 ~AP4_RtpPacket(); 00095 00096 // methods 00097 AP4_Result Write(AP4_ByteStream& stream); 00098 AP4_Result AddConstructor(AP4_RtpConstructor* constructor); 00099 AP4_Size GetSize(); 00100 AP4_Size GetConstructedDataSize(); 00101 00102 // Referenceable methods 00103 void AddReference(); 00104 void Release(); 00105 00106 // Accessors 00107 int GetRelativeTime() const { return m_RelativeTime; } 00108 bool GetPBit() const { return m_PBit; } 00109 bool GetXBit() const { return m_XBit; } 00110 bool GetMBit() const { return m_MBit; } 00111 AP4_UI08 GetPayloadType() const { return m_PayloadType; } 00112 AP4_UI16 GetSequenceSeed() const { return m_SequenceSeed; } 00113 int GetTimeStampOffset() const { return m_TimeStampOffset; } 00114 bool GetBFrameFlag() const { return m_BFrameFlag; } 00115 bool GetRepeatFlag() const { return m_RepeatFlag; } 00116 AP4_List<AP4_RtpConstructor>& GetConstructors() { 00117 return m_Constructors; 00118 } 00119 00120 private: 00121 // members 00122 AP4_Cardinal m_ReferenceCount; 00123 int m_RelativeTime; 00124 bool m_PBit; 00125 bool m_XBit; 00126 bool m_MBit; 00127 AP4_UI08 m_PayloadType; 00128 AP4_UI16 m_SequenceSeed; 00129 int m_TimeStampOffset; 00130 bool m_BFrameFlag; 00131 bool m_RepeatFlag; 00132 AP4_List<AP4_RtpConstructor> m_Constructors; 00133 }; 00134 00135 /*---------------------------------------------------------------------- 00136 | AP4_RtpContructor 00137 +---------------------------------------------------------------------*/ 00138 class AP4_RtpConstructor : public AP4_Referenceable 00139 { 00140 public: 00141 // types 00142 typedef AP4_UI08 Type; 00143 00144 // constructor & destructor 00145 AP4_RtpConstructor(Type type) : m_ReferenceCount(1), m_Type(type) {} 00146 00147 // methods 00148 Type GetType() const { return m_Type; } 00149 AP4_Result Write(AP4_ByteStream& stream); 00150 virtual AP4_Size GetConstructedDataSize() = 0; 00151 00152 // Referenceable methods 00153 void AddReference(); 00154 void Release(); 00155 00156 protected: 00157 // methods 00158 virtual ~AP4_RtpConstructor() {} 00159 virtual AP4_Result DoWrite(AP4_ByteStream& stream) = 0; 00160 00161 // members 00162 AP4_Cardinal m_ReferenceCount; 00163 Type m_Type; 00164 }; 00165 00166 /*---------------------------------------------------------------------- 00167 | constructor size 00168 +---------------------------------------------------------------------*/ 00169 const AP4_Size AP4_RTP_CONSTRUCTOR_SIZE = 16; 00170 00171 /*---------------------------------------------------------------------- 00172 | constructor types 00173 +---------------------------------------------------------------------*/ 00174 const AP4_RtpConstructor::Type AP4_RTP_CONSTRUCTOR_TYPE_NOOP = 0; 00175 const AP4_RtpConstructor::Type AP4_RTP_CONSTRUCTOR_TYPE_IMMEDIATE = 1; 00176 const AP4_RtpConstructor::Type AP4_RTP_CONSTRUCTOR_TYPE_SAMPLE = 2; 00177 const AP4_RtpConstructor::Type AP4_RTP_CONSTRUCTOR_TYPE_SAMPLE_DESC = 3; 00178 00179 /*---------------------------------------------------------------------- 00180 | AP4_NoopRtpConstructor 00181 +---------------------------------------------------------------------*/ 00182 class AP4_NoopRtpConstructor : public AP4_RtpConstructor 00183 { 00184 public: 00185 // constructor 00186 AP4_NoopRtpConstructor(AP4_ByteStream& stream); 00187 AP4_NoopRtpConstructor() : AP4_RtpConstructor(AP4_RTP_CONSTRUCTOR_TYPE_NOOP) {} 00188 00189 // methods 00190 virtual AP4_Size GetConstructedDataSize() { return 0; } 00191 00192 protected: 00193 // methods 00194 virtual AP4_Result DoWrite(AP4_ByteStream& stream); 00195 }; 00196 00197 /*---------------------------------------------------------------------- 00198 | AP4_ImmediateRtpConstructor 00199 +---------------------------------------------------------------------*/ 00200 class AP4_ImmediateRtpConstructor : public AP4_RtpConstructor 00201 { 00202 public: 00203 // constructor 00204 AP4_ImmediateRtpConstructor(AP4_ByteStream& stream); 00205 AP4_ImmediateRtpConstructor(const AP4_DataBuffer& data); 00206 00207 // accessors 00208 const AP4_DataBuffer& GetData() const { return m_Data; } 00209 00210 // methods 00211 virtual AP4_Size GetConstructedDataSize() { return m_Data.GetDataSize(); } 00212 00213 protected: 00214 // methods 00215 virtual AP4_Result DoWrite(AP4_ByteStream& stream); 00216 00217 // members 00218 AP4_DataBuffer m_Data; 00219 }; 00220 00221 /*---------------------------------------------------------------------- 00222 | AP4_SampleRtpConstructor 00223 +---------------------------------------------------------------------*/ 00224 class AP4_SampleRtpConstructor : public AP4_RtpConstructor 00225 { 00226 public: 00227 // constructor 00228 AP4_SampleRtpConstructor(AP4_ByteStream& stream); 00229 AP4_SampleRtpConstructor(AP4_UI08 track_ref_index, 00230 AP4_UI16 length, 00231 AP4_UI32 sample_num, 00232 AP4_UI32 sample_offset); 00233 00234 // accessors 00235 AP4_UI08 GetTrackRefIndex() const { return m_TrackRefIndex; } 00236 AP4_UI16 GetLength() const { return m_Length; } 00237 AP4_UI32 GetSampleNum() const { return m_SampleNum; } 00238 AP4_UI32 GetSampleOffset() const { return m_SampleOffset; } 00239 00240 // methods 00241 virtual AP4_Size GetConstructedDataSize() { return m_Length; } 00242 00243 protected: 00244 // methods 00245 virtual AP4_Result DoWrite(AP4_ByteStream& stream); 00246 00247 // members 00248 AP4_UI08 m_TrackRefIndex; 00249 AP4_UI16 m_Length; 00250 AP4_UI32 m_SampleNum; 00251 AP4_UI32 m_SampleOffset; 00252 }; 00253 00254 /*---------------------------------------------------------------------- 00255 | AP4_SampleDescRtpConstructor 00256 +---------------------------------------------------------------------*/ 00257 class AP4_SampleDescRtpConstructor : public AP4_RtpConstructor 00258 { 00259 public: 00260 // constructor 00261 AP4_SampleDescRtpConstructor(AP4_ByteStream& stream); 00262 AP4_SampleDescRtpConstructor(AP4_UI08 track_ref_index, 00263 AP4_UI16 length, 00264 AP4_UI32 sample_desc_index, 00265 AP4_UI32 sample_desc_offset); 00266 00267 // accessors 00268 AP4_UI08 GetTrackRefIndex() const { return m_TrackRefIndex; } 00269 AP4_UI16 GetLength() const { return m_Length; } 00270 AP4_UI32 GetSampleDescIndex() const { return m_SampleDescIndex; } 00271 AP4_UI32 GetSampleDescOffset() const { return m_SampleDescOffset; } 00272 00273 // methods 00274 virtual AP4_Size GetConstructedDataSize() { return m_Length; } 00275 00276 protected: 00277 // methods 00278 virtual AP4_Result DoWrite(AP4_ByteStream& stream); 00279 00280 // members 00281 AP4_UI08 m_TrackRefIndex; 00282 AP4_UI16 m_Length; 00283 AP4_UI32 m_SampleDescIndex; 00284 AP4_UI32 m_SampleDescOffset; 00285 }; 00286 00287 /*---------------------------------------------------------------------- 00288 | AP4_RtpConstructorFactory 00289 +---------------------------------------------------------------------*/ 00290 class AP4_RtpConstructorFactory 00291 { 00292 public: 00293 static AP4_Result CreateConstructorFromStream(AP4_ByteStream& stream, 00294 AP4_RtpConstructor*& constructor); 00295 }; 00296 00297 #endif // _AP4_RTP_HINT_H_
Generated on Thu May 13 16:36:33 2010 for Bento4 MP4 SDK by
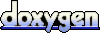