Ap4Piff.h
Go to the documentation of this file.00001 /***************************************************************** 00002 | 00003 | AP4 - PIFF support 00004 | 00005 | Copyright 2002-2009 Axiomatic Systems, LLC 00006 | 00007 | 00008 | This file is part of Bento4/AP4 (MP4 Atom Processing Library). 00009 | 00010 | Unless you have obtained Bento4 under a difference license, 00011 | this version of Bento4 is Bento4|GPL. 00012 | Bento4|GPL is free software; you can redistribute it and/or modify 00013 | it under the terms of the GNU General Public License as published by 00014 | the Free Software Foundation; either version 2, or (at your option) 00015 | any later version. 00016 | 00017 | Bento4|GPL is distributed in the hope that it will be useful, 00018 | but WITHOUT ANY WARRANTY; without even the implied warranty of 00019 | MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00020 | GNU General Public License for more details. 00021 | 00022 | You should have received a copy of the GNU General Public License 00023 | along with Bento4|GPL; see the file COPYING. If not, write to the 00024 | Free Software Foundation, 59 Temple Place - Suite 330, Boston, MA 00025 | 02111-1307, USA. 00026 | 00027 ****************************************************************/ 00028 00029 #ifndef _AP4_PIFF_H_ 00030 #define _AP4_PIFF_H_ 00031 00032 /*---------------------------------------------------------------------- 00033 | includes 00034 +---------------------------------------------------------------------*/ 00035 #include "Ap4Types.h" 00036 #include "Ap4SampleEntry.h" 00037 #include "Ap4Atom.h" 00038 #include "Ap4AtomFactory.h" 00039 #include "Ap4SampleDescription.h" 00040 #include "Ap4Processor.h" 00041 #include "Ap4Protection.h" 00042 #include "Ap4UuidAtom.h" 00043 00044 /*---------------------------------------------------------------------- 00045 | class references 00046 +---------------------------------------------------------------------*/ 00047 class AP4_StreamCipher; 00048 class AP4_CbcStreamCipher; 00049 class AP4_CtrStreamCipher; 00050 class AP4_PiffSampleEncryptionAtom; 00051 00052 /*---------------------------------------------------------------------- 00053 | constants 00054 +---------------------------------------------------------------------*/ 00055 const AP4_UI32 AP4_PROTECTION_SCHEME_TYPE_PIFF = AP4_ATOM_TYPE('p','i','f','f'); 00056 const AP4_UI32 AP4_PROTECTION_SCHEME_VERSION_PIFF_10 = 0x00010000; 00057 const AP4_UI32 AP4_PIFF_BRAND = AP4_ATOM_TYPE('p','i','f','f'); 00058 const AP4_UI32 AP4_PIFF_ALGORITHM_ID_NONE = 0; 00059 const AP4_UI32 AP4_PIFF_ALGORITHM_ID_CTR = 1; 00060 const AP4_UI32 AP4_PIFF_ALGORITHM_ID_CBC = 2; 00061 00062 extern AP4_UI08 const AP4_UUID_PIFF_TRACK_ENCRYPTION_ATOM[16]; 00063 extern AP4_UI08 const AP4_UUID_PIFF_SAMPLE_ENCRYPTION_ATOM[16]; 00064 00065 const unsigned int AP4_PIFF_SAMPLE_ENCRYPTION_FLAG_OVERRIDE_TRACK_ENCRYPTION_DEFAULTS = 1; 00066 00067 typedef enum { 00068 AP4_PIFF_CIPHER_MODE_CTR, 00069 AP4_PIFF_CIPHER_MODE_CBC 00070 } AP4_PiffCipherMode; 00071 00072 /*---------------------------------------------------------------------- 00073 | AP4_PiffSampleEncrypter 00074 +---------------------------------------------------------------------*/ 00075 class AP4_PiffSampleEncrypter 00076 { 00077 public: 00078 // constructor and destructor 00079 AP4_PiffSampleEncrypter() { AP4_SetMemory(m_Iv, 0, 16); }; 00080 virtual ~AP4_PiffSampleEncrypter() {} 00081 00082 // methods 00083 virtual AP4_Result EncryptSampleData(AP4_DataBuffer& data_in, 00084 AP4_DataBuffer& data_out) = 0; 00085 00086 void SetIv(const AP4_UI08* iv) { AP4_CopyMemory(m_Iv, iv, 16); } 00087 const AP4_UI08* GetIv() { return m_Iv; } 00088 00089 protected: 00090 AP4_UI08 m_Iv[16]; 00091 }; 00092 00093 /*---------------------------------------------------------------------- 00094 | AP4_PiffCtrSampleEncrypter 00095 +---------------------------------------------------------------------*/ 00096 class AP4_PiffCtrSampleEncrypter : public AP4_PiffSampleEncrypter 00097 { 00098 public: 00099 // constructor and destructor 00100 AP4_PiffCtrSampleEncrypter(AP4_BlockCipher* block_cipher); 00101 ~AP4_PiffCtrSampleEncrypter(); 00102 00103 // methods 00104 virtual AP4_Result EncryptSampleData(AP4_DataBuffer& data_in, 00105 AP4_DataBuffer& data_out); 00106 00107 protected: 00108 // members 00109 AP4_CtrStreamCipher* m_Cipher; 00110 }; 00111 00112 /*---------------------------------------------------------------------- 00113 | AP4_PiffAvcCtrSampleEncrypter 00114 +---------------------------------------------------------------------*/ 00115 class AP4_PiffAvcCtrSampleEncrypter : public AP4_PiffCtrSampleEncrypter 00116 { 00117 public: 00118 // constructor and destructor 00119 AP4_PiffAvcCtrSampleEncrypter(AP4_BlockCipher* block_cipher, 00120 AP4_Size nalu_length_size) : 00121 AP4_PiffCtrSampleEncrypter(block_cipher), 00122 m_NaluLengthSize(nalu_length_size) {} 00123 00124 // methods 00125 virtual AP4_Result EncryptSampleData(AP4_DataBuffer& data_in, 00126 AP4_DataBuffer& data_out); 00127 00128 private: 00129 // members 00130 AP4_Size m_NaluLengthSize; 00131 }; 00132 00133 /*---------------------------------------------------------------------- 00134 | AP4_PiffCbcSampleEncrypter 00135 +---------------------------------------------------------------------*/ 00136 class AP4_PiffCbcSampleEncrypter : public AP4_PiffSampleEncrypter 00137 { 00138 public: 00139 // constructor and destructor 00140 AP4_PiffCbcSampleEncrypter(AP4_BlockCipher* block_cipher); 00141 ~AP4_PiffCbcSampleEncrypter(); 00142 00143 // methods 00144 virtual AP4_Result EncryptSampleData(AP4_DataBuffer& data_in, 00145 AP4_DataBuffer& data_out); 00146 00147 protected: 00148 // members 00149 AP4_CbcStreamCipher* m_Cipher; 00150 }; 00151 00152 /*---------------------------------------------------------------------- 00153 | AP4_PiffAvcCbcSampleEncrypter 00154 +---------------------------------------------------------------------*/ 00155 class AP4_PiffAvcCbcSampleEncrypter : public AP4_PiffCbcSampleEncrypter 00156 { 00157 public: 00158 // constructor and destructor 00159 AP4_PiffAvcCbcSampleEncrypter(AP4_BlockCipher* block_cipher, 00160 AP4_Size nalu_length_size) : 00161 AP4_PiffCbcSampleEncrypter(block_cipher), 00162 m_NaluLengthSize(nalu_length_size) {} 00163 00164 // methods 00165 virtual AP4_Result EncryptSampleData(AP4_DataBuffer& data_in, 00166 AP4_DataBuffer& data_out); 00167 00168 private: 00169 // members 00170 AP4_Size m_NaluLengthSize; 00171 }; 00172 00173 /*---------------------------------------------------------------------- 00174 | AP4_PiffEncryptingProcessor 00175 +---------------------------------------------------------------------*/ 00176 class AP4_PiffEncryptingProcessor : public AP4_Processor 00177 { 00178 public: 00179 // types 00180 struct Encrypter { 00181 Encrypter(AP4_UI32 track_id, AP4_PiffSampleEncrypter* sample_encrypter) : 00182 m_TrackId(track_id), 00183 m_SampleEncrypter(sample_encrypter) {} 00184 Encrypter() { delete m_SampleEncrypter; } 00185 AP4_UI32 m_TrackId; 00186 AP4_PiffSampleEncrypter* m_SampleEncrypter; 00187 }; 00188 00189 // constructor 00190 AP4_PiffEncryptingProcessor(AP4_PiffCipherMode cipher_mode, 00191 AP4_BlockCipherFactory* block_cipher_factory = NULL); 00192 ~AP4_PiffEncryptingProcessor(); 00193 00194 // accessors 00195 AP4_ProtectionKeyMap& GetKeyMap() { return m_KeyMap; } 00196 AP4_TrackPropertyMap& GetPropertyMap() { return m_PropertyMap; } 00197 00198 // AP4_Processor methods 00199 virtual AP4_Result Initialize(AP4_AtomParent& top_level, 00200 AP4_ByteStream& stream, 00201 AP4_Processor::ProgressListener* listener = NULL); 00202 virtual AP4_Processor::TrackHandler* CreateTrackHandler(AP4_TrakAtom* trak); 00203 virtual AP4_Processor::FragmentHandler* CreateFragmentHandler(AP4_ContainerAtom* traf); 00204 00205 protected: 00206 // members 00207 AP4_PiffCipherMode m_CipherMode; 00208 AP4_BlockCipherFactory* m_BlockCipherFactory; 00209 AP4_ProtectionKeyMap m_KeyMap; 00210 AP4_TrackPropertyMap m_PropertyMap; 00211 AP4_List<Encrypter> m_Encrypters; 00212 }; 00213 00214 /*---------------------------------------------------------------------- 00215 | AP4_PiffSampleDecrypter 00216 +---------------------------------------------------------------------*/ 00217 class AP4_PiffSampleDecrypter : public AP4_SampleDecrypter 00218 { 00219 public: 00220 // factory 00221 static AP4_Result Create(AP4_ProtectedSampleDescription* sample_description, 00222 AP4_ContainerAtom* traf, 00223 const AP4_UI08* key, 00224 AP4_Size key_size, 00225 AP4_BlockCipherFactory* block_cipher_factory, 00226 AP4_PiffSampleDecrypter*& decrypter); 00227 00228 // methods 00229 virtual AP4_Result SetSampleIndex(AP4_Ordinal sample_index); 00230 00231 protected: 00232 AP4_PiffSampleDecrypter(AP4_PiffSampleEncryptionAtom* sample_encryption_atom) : 00233 m_SampleEncryptionAtom(sample_encryption_atom), 00234 m_SampleIndex(0) {} 00235 AP4_PiffSampleEncryptionAtom* m_SampleEncryptionAtom; 00236 AP4_Ordinal m_SampleIndex; 00237 }; 00238 00239 /*---------------------------------------------------------------------- 00240 | AP4_PiffNullSampleDecrypter 00241 +---------------------------------------------------------------------*/ 00242 class AP4_PiffNullSampleDecrypter : public AP4_PiffSampleDecrypter 00243 { 00244 public: 00245 // constructor 00246 AP4_PiffNullSampleDecrypter() : AP4_PiffSampleDecrypter(NULL) {} 00247 00248 // methods 00249 virtual AP4_Result DecryptSampleData(AP4_DataBuffer& data_in, 00250 AP4_DataBuffer& data_out, 00251 const AP4_UI08* /*iv = NULL*/) { 00252 data_out.SetData(data_in.GetData(), data_in.GetDataSize()); 00253 return AP4_SUCCESS; 00254 } 00255 }; 00256 00257 /*---------------------------------------------------------------------- 00258 | AP4_PiffCtrSampleDecrypter 00259 +---------------------------------------------------------------------*/ 00260 class AP4_PiffCtrSampleDecrypter : public AP4_PiffSampleDecrypter 00261 { 00262 public: 00263 // constructor and destructor 00264 AP4_PiffCtrSampleDecrypter(AP4_BlockCipher* block_cipher, 00265 AP4_Size iv_size, 00266 AP4_PiffSampleEncryptionAtom* sample_encryption_atom); 00267 ~AP4_PiffCtrSampleDecrypter(); 00268 00269 // methods 00270 virtual AP4_Result DecryptSampleData(AP4_DataBuffer& data_in, 00271 AP4_DataBuffer& data_out, 00272 const AP4_UI08* iv = NULL); 00273 00274 private: 00275 // members 00276 AP4_CtrStreamCipher* m_Cipher; 00277 AP4_Size m_IvSize; 00278 }; 00279 00280 /*---------------------------------------------------------------------- 00281 | AP4_PiffAvcCtrSampleDecrypter 00282 +---------------------------------------------------------------------*/ 00283 class AP4_PiffAvcCtrSampleDecrypter : public AP4_PiffCtrSampleDecrypter 00284 { 00285 public: 00286 // constructor and destructor 00287 AP4_PiffAvcCtrSampleDecrypter(AP4_BlockCipher* block_cipher, 00288 AP4_Size iv_size, 00289 AP4_PiffSampleEncryptionAtom* sample_encryption_atom, 00290 AP4_Size nalu_length_size) : 00291 AP4_PiffCtrSampleDecrypter(block_cipher, iv_size, sample_encryption_atom), 00292 m_NaluLengthSize(nalu_length_size) {} 00293 00294 // methods 00295 virtual AP4_Result DecryptSampleData(AP4_DataBuffer& data_in, 00296 AP4_DataBuffer& data_out, 00297 const AP4_UI08* iv = NULL); 00298 00299 private: 00300 // members 00301 AP4_Size m_NaluLengthSize; 00302 }; 00303 00304 /*---------------------------------------------------------------------- 00305 | AP4_PiffCbcSampleDecrypter 00306 +---------------------------------------------------------------------*/ 00307 class AP4_PiffCbcSampleDecrypter : public AP4_PiffSampleDecrypter 00308 { 00309 public: 00310 // constructor and destructor 00311 AP4_PiffCbcSampleDecrypter(AP4_BlockCipher* block_cipher, 00312 AP4_PiffSampleEncryptionAtom* sample_encryption_atom); 00313 ~AP4_PiffCbcSampleDecrypter(); 00314 00315 // methods 00316 virtual AP4_Result DecryptSampleData(AP4_DataBuffer& data_in, 00317 AP4_DataBuffer& data_out, 00318 const AP4_UI08* iv = NULL); 00319 00320 protected: 00321 // members 00322 AP4_CbcStreamCipher* m_Cipher; 00323 }; 00324 00325 /*---------------------------------------------------------------------- 00326 | AP4_PiffAvcCbcSampleDecrypter 00327 +---------------------------------------------------------------------*/ 00328 class AP4_PiffAvcCbcSampleDecrypter : public AP4_PiffCbcSampleDecrypter 00329 { 00330 public: 00331 // constructor and destructor 00332 AP4_PiffAvcCbcSampleDecrypter(AP4_BlockCipher* block_cipher, 00333 AP4_PiffSampleEncryptionAtom* sample_encryption_atom, 00334 AP4_Size nalu_length_size) : 00335 AP4_PiffCbcSampleDecrypter(block_cipher, sample_encryption_atom), 00336 m_NaluLengthSize(nalu_length_size) {} 00337 00338 // methods 00339 virtual AP4_Result DecryptSampleData(AP4_DataBuffer& data_in, 00340 AP4_DataBuffer& data_out, 00341 const AP4_UI08* iv = NULL); 00342 00343 private: 00344 // members 00345 AP4_Size m_NaluLengthSize; 00346 }; 00347 00348 /*---------------------------------------------------------------------- 00349 | AP4_PiffTrackEncryptionAtom 00350 +---------------------------------------------------------------------*/ 00351 class AP4_PiffTrackEncryptionAtom : public AP4_UuidAtom 00352 { 00353 public: 00354 AP4_IMPLEMENT_DYNAMIC_CAST_D(AP4_PiffTrackEncryptionAtom, AP4_UuidAtom) 00355 00356 // class methods 00357 static AP4_PiffTrackEncryptionAtom* Create(AP4_Size size, 00358 AP4_ByteStream& stream); 00359 00360 // constructors 00361 AP4_PiffTrackEncryptionAtom(AP4_UI32 default_algorithm_id, 00362 AP4_UI08 default_iv_size, 00363 const AP4_UI08* default_kid); 00364 00365 // methods 00366 virtual AP4_Result InspectFields(AP4_AtomInspector& inspector); 00367 virtual AP4_Result WriteFields(AP4_ByteStream& stream); 00368 00369 // accessors 00370 AP4_UI32 GetDefaultAlgorithmId() { return m_DefaultAlgorithmId; } 00371 AP4_UI08 GetDefaultIvSize() { return m_DefaultIvSize; } 00372 const AP4_UI08* GetDefaultKid() { return m_DefaultKid; } 00373 00374 private: 00375 // methods 00376 AP4_PiffTrackEncryptionAtom(AP4_UI32 size, 00377 AP4_UI32 version, 00378 AP4_UI32 flags, 00379 AP4_ByteStream& stream); 00380 00381 // members 00382 AP4_UI32 m_DefaultAlgorithmId; 00383 AP4_UI08 m_DefaultIvSize; 00384 AP4_UI08 m_DefaultKid[16]; 00385 }; 00386 00387 /*---------------------------------------------------------------------- 00388 | AP4_PiffSampleEncryptionAtom 00389 +---------------------------------------------------------------------*/ 00390 class AP4_PiffSampleEncryptionAtom : public AP4_UuidAtom 00391 { 00392 public: 00393 AP4_IMPLEMENT_DYNAMIC_CAST_D(AP4_PiffSampleEncryptionAtom, AP4_UuidAtom) 00394 00395 // class methods 00396 static AP4_PiffSampleEncryptionAtom* Create(AP4_Size size, 00397 AP4_ByteStream& stream); 00398 00399 // constructors 00400 AP4_PiffSampleEncryptionAtom(AP4_Cardinal sample_count); 00401 AP4_PiffSampleEncryptionAtom(AP4_UI32 algorithm_id, 00402 AP4_UI08 iv_size, 00403 const AP4_UI08* kid, 00404 AP4_Cardinal sample_count); 00405 00406 // methods 00407 virtual AP4_Result InspectFields(AP4_AtomInspector& inspector); 00408 virtual AP4_Result WriteFields(AP4_ByteStream& stream); 00409 00410 // accessors 00411 AP4_UI32 GetAlgorithmId() { return m_AlgorithmId; } 00412 AP4_UI08 GetIvSize() { return m_IvSize; } 00413 AP4_Result SetIvSize(AP4_UI08 iv_size); 00414 const AP4_UI08* GetKid() { return m_Kid; } 00415 AP4_Cardinal GetIvCount() { return m_IvCount; } 00416 const AP4_UI08* GetIv(AP4_Ordinal indx); 00417 AP4_Result SetIv(AP4_Ordinal indx, const AP4_UI08* iv); 00418 00419 private: 00420 // methods 00421 AP4_PiffSampleEncryptionAtom(AP4_UI32 size, 00422 AP4_UI32 version, 00423 AP4_UI32 flags, 00424 AP4_ByteStream& stream); 00425 00426 // members 00427 AP4_UI32 m_AlgorithmId; 00428 AP4_UI08 m_IvSize; 00429 AP4_UI08 m_Kid[16]; 00430 AP4_Cardinal m_IvCount; 00431 AP4_DataBuffer m_Ivs; 00432 }; 00433 00434 #endif // _AP4_PIFF_H_
Generated on Thu May 13 16:36:33 2010 for Bento4 MP4 SDK by
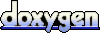