AP4_Atom Class Reference
Abstract base class for all atom types. More...
#include <Ap4Atom.h>

Public Types | |
typedef AP4_UI32 | Type |
Public Member Functions | |
AP4_Atom (Type type, AP4_UI32 size=AP4_ATOM_HEADER_SIZE) | |
Create a simple atom with a specified type and 32-bit size. | |
AP4_Atom (Type type, AP4_UI64 size, bool force_64=false) | |
Create a simple atom with a specified type and 64-bit size. | |
AP4_Atom (Type type, AP4_UI32 size, AP4_UI32 version, AP4_UI32 flags) | |
Create a full atom with a specified type, 32-bit size, version and flags. | |
AP4_Atom (Type type, AP4_UI64 size, bool force_64, AP4_UI32 version, AP4_UI32 flags) | |
Create a full atom with a specified type, 64-bit size, version and flags. | |
virtual | ~AP4_Atom () |
AP4_UI32 | GetFlags () const |
void | SetFlags (AP4_UI32 flags) |
Type | GetType () const |
void | SetType (Type type) |
virtual AP4_Size | GetHeaderSize () const |
AP4_UI64 | GetSize () const |
void | SetSize (AP4_UI64 size, bool force_64=false) |
AP4_UI32 | GetSize32 () const |
void | SetSize32 (AP4_UI32 size) |
AP4_UI64 | GetSize64 () const |
void | SetSize64 (AP4_UI64 size) |
virtual AP4_Result | Write (AP4_ByteStream &stream) |
virtual AP4_Result | WriteHeader (AP4_ByteStream &stream) |
virtual AP4_Result | WriteFields (AP4_ByteStream &stream)=0 |
virtual AP4_Result | Inspect (AP4_AtomInspector &inspector) |
virtual AP4_Result | InspectHeader (AP4_AtomInspector &inspector) |
virtual AP4_Result | InspectFields (AP4_AtomInspector &) |
virtual AP4_Result | SetParent (AP4_AtomParent *parent) |
virtual AP4_AtomParent * | GetParent () |
virtual AP4_Result | Detach () |
virtual AP4_Atom * | Clone () |
Create a clone of the object. | |
Static Public Member Functions | |
static Type | TypeFromString (const char *four_cc) |
static AP4_Result | ReadFullHeader (AP4_ByteStream &stream, AP4_UI32 &version, AP4_UI32 &flags) |
Protected Attributes | |
Type | m_Type |
AP4_UI32 | m_Size32 |
AP4_UI64 | m_Size64 |
bool | m_IsFull |
AP4_UI32 | m_Version |
AP4_UI32 | m_Flags |
AP4_AtomParent * | m_Parent |
Detailed Description
Abstract base class for all atom types.
Definition at line 152 of file Ap4Atom.h.
Member Typedef Documentation
typedef AP4_UI32 AP4_Atom::Type |
Constructor & Destructor Documentation
AP4_Atom::AP4_Atom | ( | Type | type, | |
AP4_UI32 | size = AP4_ATOM_HEADER_SIZE | |||
) | [explicit] |
Create a simple atom with a specified type and 32-bit size.
Create a simple atom with a specified type and 64-bit size.
Create a full atom with a specified type, 32-bit size, version and flags.
AP4_Atom::AP4_Atom | ( | Type | type, | |
AP4_UI64 | size, | |||
bool | force_64, | |||
AP4_UI32 | version, | |||
AP4_UI32 | flags | |||
) | [explicit] |
Create a full atom with a specified type, 64-bit size, version and flags.
Member Function Documentation
virtual AP4_Atom* AP4_Atom::Clone | ( | ) | [virtual] |
Create a clone of the object.
This method returns a clone of the atom, or NULL if the atom cannot be cloned. Override this if your want to make an atom cloneable in a more efficient way than the default implementation.
Reimplemented in AP4_UnknownAtom, AP4_ContainerAtom, AP4_GrpiAtom, AP4_IkmsAtom, AP4_IsfmAtom, and AP4_OhdrAtom.
virtual AP4_Result AP4_Atom::Detach | ( | ) | [virtual] |
AP4_UI32 AP4_Atom::GetFlags | ( | ) | const [inline] |
virtual AP4_Size AP4_Atom::GetHeaderSize | ( | ) | const [virtual] |
Reimplemented in AP4_UuidAtom.
virtual AP4_AtomParent* AP4_Atom::GetParent | ( | ) | [inline, virtual] |
AP4_UI64 AP4_Atom::GetSize | ( | ) | const [inline] |
AP4_UI32 AP4_Atom::GetSize32 | ( | ) | const [inline] |
AP4_UI64 AP4_Atom::GetSize64 | ( | ) | const [inline] |
Type AP4_Atom::GetType | ( | ) | const [inline] |
virtual AP4_Result AP4_Atom::Inspect | ( | AP4_AtomInspector & | inspector | ) | [virtual] |
Reimplemented in AP4_SampleEntry.
Referenced by AP4_AtomListInspector::Action().
virtual AP4_Result AP4_Atom::InspectFields | ( | AP4_AtomInspector & | ) | [inline, virtual] |
Reimplemented in AP4_8bdlAtom, AP4_NullTerminatedStringAtom, AP4_AvccAtom, AP4_Co64Atom, AP4_ContainerAtom, AP4_CttsAtom, AP4_ElstAtom, AP4_EsdsAtom, AP4_FrmaAtom, AP4_FtypAtom, AP4_GrpiAtom, AP4_HdlrAtom, AP4_HmhdAtom, AP4_IkmsAtom, AP4_IodsAtom, AP4_IproAtom, AP4_IsfmAtom, AP4_IsltAtom, AP4_MdhdAtom, AP4_MehdAtom, AP4_MfhdAtom, AP4_MfroAtom, AP4_MvhdAtom, AP4_OdafAtom, AP4_OddaAtom, AP4_OdheAtom, AP4_OhdrAtom, AP4_PiffTrackEncryptionAtom, AP4_PiffSampleEncryptionAtom, AP4_RtpAtom, AP4_SampleEntry, AP4_AudioSampleEntry, AP4_VisualSampleEntry, AP4_RtpHintSampleEntry, AP4_SchmAtom, AP4_SdpAtom, AP4_SmhdAtom, AP4_StcoAtom, AP4_StscAtom, AP4_StsdAtom, AP4_StssAtom, AP4_StszAtom, AP4_SttsAtom, AP4_TfhdAtom, AP4_TfraAtom, AP4_TimsAtom, AP4_TkhdAtom, AP4_TrefTypeAtom, AP4_TrexAtom, AP4_TrunAtom, AP4_UrlAtom, AP4_VmhdAtom, AP4_3GppLocalizedStringAtom, AP4_DcfStringAtom, AP4_DcfdAtom, AP4_MetaDataStringAtom, and AP4_DataAtom.
Definition at line 213 of file Ap4Atom.h.
References AP4_SUCCESS.
virtual AP4_Result AP4_Atom::InspectHeader | ( | AP4_AtomInspector & | inspector | ) | [virtual] |
Reimplemented in AP4_UuidAtom.
static AP4_Result AP4_Atom::ReadFullHeader | ( | AP4_ByteStream & | stream, | |
AP4_UI32 & | version, | |||
AP4_UI32 & | flags | |||
) | [static] |
void AP4_Atom::SetFlags | ( | AP4_UI32 | flags | ) | [inline] |
virtual AP4_Result AP4_Atom::SetParent | ( | AP4_AtomParent * | parent | ) | [inline, virtual] |
Definition at line 218 of file Ap4Atom.h.
References AP4_SUCCESS, and m_Parent.
void AP4_Atom::SetSize | ( | AP4_UI64 | size, | |
bool | force_64 = false | |||
) |
void AP4_Atom::SetSize32 | ( | AP4_UI32 | size | ) | [inline] |
void AP4_Atom::SetSize64 | ( | AP4_UI64 | size | ) | [inline] |
void AP4_Atom::SetType | ( | Type | type | ) | [inline] |
static Type AP4_Atom::TypeFromString | ( | const char * | four_cc | ) | [static] |
virtual AP4_Result AP4_Atom::Write | ( | AP4_ByteStream & | stream | ) | [virtual] |
Reimplemented in AP4_SampleEntry.
virtual AP4_Result AP4_Atom::WriteFields | ( | AP4_ByteStream & | stream | ) | [pure virtual] |
Implemented in AP4_8bdlAtom, AP4_UnknownAtom, AP4_NullTerminatedStringAtom, AP4_AvccAtom, AP4_Co64Atom, AP4_ContainerAtom, AP4_CttsAtom, AP4_DrefAtom, AP4_ElstAtom, AP4_EsdsAtom, AP4_FrmaAtom, AP4_FtypAtom, AP4_GrpiAtom, AP4_HdlrAtom, AP4_HmhdAtom, AP4_IkmsAtom, AP4_IodsAtom, AP4_IproAtom, AP4_IsfmAtom, AP4_IsltAtom, AP4_MdhdAtom, AP4_MehdAtom, AP4_MfhdAtom, AP4_MfroAtom, AP4_MvhdAtom, AP4_NmhdAtom, AP4_OdafAtom, AP4_OddaAtom, AP4_OdheAtom, AP4_OhdrAtom, AP4_PiffTrackEncryptionAtom, AP4_PiffSampleEncryptionAtom, AP4_RtpAtom, AP4_SampleEntry, AP4_UnknownSampleEntry, AP4_AudioSampleEntry, AP4_VisualSampleEntry, AP4_RtpHintSampleEntry, AP4_SchmAtom, AP4_SdpAtom, AP4_SmhdAtom, AP4_StcoAtom, AP4_StscAtom, AP4_StsdAtom, AP4_StssAtom, AP4_StszAtom, AP4_SttsAtom, AP4_TfhdAtom, AP4_TfraAtom, AP4_TimsAtom, AP4_TkhdAtom, AP4_TrefTypeAtom, AP4_TrexAtom, AP4_TrunAtom, AP4_UrlAtom, AP4_UnknownUuidAtom, AP4_VmhdAtom, AP4_3GppLocalizedStringAtom, AP4_DcfStringAtom, AP4_DcfdAtom, AP4_MetaDataStringAtom, and AP4_DataAtom.
virtual AP4_Result AP4_Atom::WriteHeader | ( | AP4_ByteStream & | stream | ) | [virtual] |
Reimplemented in AP4_UuidAtom.
Member Data Documentation
AP4_UI32 AP4_Atom::m_Flags [protected] |
Definition at line 242 of file Ap4Atom.h.
Referenced by GetFlags(), and SetFlags().
bool AP4_Atom::m_IsFull [protected] |
AP4_AtomParent* AP4_Atom::m_Parent [protected] |
Definition at line 243 of file Ap4Atom.h.
Referenced by GetParent(), and SetParent().
AP4_UI32 AP4_Atom::m_Size32 [protected] |
Definition at line 237 of file Ap4Atom.h.
Referenced by GetSize(), GetSize32(), and SetSize32().
AP4_UI64 AP4_Atom::m_Size64 [protected] |
Definition at line 238 of file Ap4Atom.h.
Referenced by GetSize(), GetSize64(), and SetSize64().
Type AP4_Atom::m_Type [protected] |
AP4_UI32 AP4_Atom::m_Version [protected] |
The documentation for this class was generated from the following file:
Generated on Thu May 13 16:36:44 2010 for Bento4 MP4 SDK by
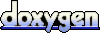