AP4_List< T > Class Template Reference
#include <Ap4List.h>
Classes | |
class | Item |
Public Member Functions | |
AP4_List () | |
virtual | ~AP4_List () |
AP4_Result | Clear () |
AP4_Result | Add (T *data) |
AP4_Result | Add (Item *item) |
AP4_Result | Remove (T *data) |
AP4_Result | Insert (Item *where, T *data) |
AP4_Result | Get (AP4_Ordinal idx, T *&data) const |
AP4_Result | PopHead (T *&data) |
AP4_Result | Apply (const typename Item::Operator &op) const |
AP4_Result | ApplyUntilFailure (const typename Item::Operator &op) const |
AP4_Result | ApplyUntilSuccess (const typename Item::Operator &op) const |
AP4_Result | ReverseApply (const typename Item::Operator &op) const |
AP4_Result | Find (const typename Item::Finder &finder, T *&data) const |
AP4_Result | ReverseFind (const typename Item::Finder &finder, T *&data) const |
AP4_Result | DeleteReferences () |
AP4_Cardinal | ItemCount () const |
Item * | FirstItem () const |
Item * | LastItem () const |
Protected Attributes | |
AP4_Cardinal | m_ItemCount |
Item * | m_Head |
Item * | m_Tail |
Detailed Description
template<typename T>
class AP4_List< T >
Definition at line 47 of file Ap4List.h.
Constructor & Destructor Documentation
Definition at line 125 of file Ap4List.h.
References AP4_List< T >::Clear().
Member Function Documentation
AP4_Result AP4_List< T >::Add | ( | Item * | item | ) | [inline] |
Definition at line 167 of file Ap4List.h.
References AP4_SUCCESS, AP4_List< T >::m_Head, AP4_List< T >::m_ItemCount, and AP4_List< T >::m_Tail.
AP4_Result AP4_List< T >::Add | ( | T * | data | ) | [inline] |
Definition at line 157 of file Ap4List.h.
Referenced by AP4_List< T >::Insert().
AP4_Result AP4_List< T >::Apply | ( | const typename Item::Operator & | op | ) | const [inline] |
Definition at line 337 of file Ap4List.h.
References AP4_List< T >::Item::Operator::Action(), AP4_SUCCESS, and AP4_List< T >::m_Head.
AP4_Result AP4_List< T >::ApplyUntilFailure | ( | const typename Item::Operator & | op | ) | const [inline] |
Definition at line 355 of file Ap4List.h.
References AP4_List< T >::Item::Operator::Action(), AP4_SUCCESS, and AP4_List< T >::m_Head.
AP4_Result AP4_List< T >::ApplyUntilSuccess | ( | const typename Item::Operator & | op | ) | const [inline] |
Definition at line 375 of file Ap4List.h.
References AP4_List< T >::Item::Operator::Action(), AP4_FAILURE, AP4_SUCCESS, and AP4_List< T >::m_Head.
AP4_Result AP4_List< T >::Clear | ( | ) | [inline] |
Definition at line 136 of file Ap4List.h.
References AP4_SUCCESS, AP4_List< T >::m_Head, AP4_List< T >::m_ItemCount, and AP4_List< T >::m_Tail.
Referenced by AP4_List< T >::~AP4_List().
AP4_Result AP4_List< T >::DeleteReferences | ( | ) | [inline] |
Definition at line 459 of file Ap4List.h.
References AP4_SUCCESS, AP4_List< T >::m_Head, AP4_List< T >::m_ItemCount, and AP4_List< T >::m_Tail.
Referenced by AP4_Processor::~AP4_Processor().
AP4_Result AP4_List< T >::Find | ( | const typename Item::Finder & | finder, | |
T *& | data | |||
) | const [inline] |
Definition at line 415 of file Ap4List.h.
References AP4_ERROR_NO_SUCH_ITEM, AP4_SUCCESS, AP4_List< T >::m_Head, and AP4_List< T >::Item::Finder::Test().
AP4_Result AP4_List< T >::Get | ( | AP4_Ordinal | idx, | |
T *& | data | |||
) | const [inline] |
Definition at line 286 of file Ap4List.h.
References AP4_ERROR_NO_SUCH_ITEM, AP4_SUCCESS, AP4_List< T >::m_Head, and AP4_List< T >::m_ItemCount.
AP4_Result AP4_List< T >::Insert | ( | Item * | where, | |
T * | data | |||
) | [inline] |
Definition at line 242 of file Ap4List.h.
References AP4_List< T >::Add(), AP4_SUCCESS, AP4_List< T >::m_Head, AP4_List< T >::m_ItemCount, and AP4_List< T >::m_Tail.
AP4_Cardinal AP4_List< T >::ItemCount | ( | ) | const [inline] |
AP4_Result AP4_List< T >::PopHead | ( | T *& | data | ) | [inline] |
Definition at line 305 of file Ap4List.h.
References AP4_ERROR_LIST_EMPTY, AP4_SUCCESS, AP4_List< T >::m_Head, AP4_List< T >::m_ItemCount, and AP4_List< T >::m_Tail.
AP4_Result AP4_List< T >::Remove | ( | T * | data | ) | [inline] |
Definition at line 193 of file Ap4List.h.
References AP4_ERROR_NO_SUCH_ITEM, AP4_SUCCESS, AP4_List< T >::m_Head, AP4_List< T >::m_ItemCount, and AP4_List< T >::m_Tail.
AP4_Result AP4_List< T >::ReverseApply | ( | const typename Item::Operator & | op | ) | const [inline] |
Definition at line 395 of file Ap4List.h.
References AP4_List< T >::Item::Operator::Action(), AP4_ERROR_LIST_OPERATION_ABORTED, AP4_SUCCESS, and AP4_List< T >::m_Tail.
AP4_Result AP4_List< T >::ReverseFind | ( | const typename Item::Finder & | finder, | |
T *& | data | |||
) | const [inline] |
Definition at line 437 of file Ap4List.h.
References AP4_ERROR_NO_SUCH_ITEM, AP4_SUCCESS, AP4_List< T >::m_Tail, and AP4_List< T >::Item::Finder::Test().
Member Data Documentation
Definition at line 112 of file Ap4List.h.
Referenced by AP4_List< T >::Add(), AP4_List< T >::Apply(), AP4_List< T >::ApplyUntilFailure(), AP4_List< T >::ApplyUntilSuccess(), AP4_List< T >::Clear(), AP4_List< T >::DeleteReferences(), AP4_List< T >::Find(), AP4_List< AP4_RtpPacket >::FirstItem(), AP4_List< T >::Get(), AP4_List< T >::Insert(), AP4_List< T >::PopHead(), and AP4_List< T >::Remove().
AP4_Cardinal AP4_List< T >::m_ItemCount [protected] |
Definition at line 111 of file Ap4List.h.
Referenced by AP4_List< T >::Add(), AP4_List< T >::Clear(), AP4_List< T >::DeleteReferences(), AP4_List< T >::Get(), AP4_List< T >::Insert(), AP4_List< AP4_RtpPacket >::ItemCount(), AP4_List< T >::PopHead(), and AP4_List< T >::Remove().
Definition at line 113 of file Ap4List.h.
Referenced by AP4_List< T >::Add(), AP4_List< T >::Clear(), AP4_List< T >::DeleteReferences(), AP4_List< T >::Insert(), AP4_List< AP4_RtpPacket >::LastItem(), AP4_List< T >::PopHead(), AP4_List< T >::Remove(), AP4_List< T >::ReverseApply(), and AP4_List< T >::ReverseFind().
The documentation for this class was generated from the following file:
Generated on Thu May 13 16:37:21 2010 for Bento4 MP4 SDK by
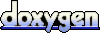