B-L475E-IOT01 BSP User Manual
|
stm32l475e_iot01_gyro.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l475e_iot01_gyro.c 00004 * @author MCD Application Team 00005 * @version V1.0.0 00006 * @date 17-March-2017 00007 * @brief This file provides a set of functions needed to manage the gyroscope sensor 00008 ****************************************************************************** 00009 * @attention 00010 * 00011 * <h2><center>© Copyright (c) 2017 STMicroelectronics International N.V. 00012 * All rights reserved.</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without 00015 * modification, are permitted, provided that the following conditions are met: 00016 * 00017 * 1. Redistribution of source code must retain the above copyright notice, 00018 * this list of conditions and the following disclaimer. 00019 * 2. Redistributions in binary form must reproduce the above copyright notice, 00020 * this list of conditions and the following disclaimer in the documentation 00021 * and/or other materials provided with the distribution. 00022 * 3. Neither the name of STMicroelectronics nor the names of other 00023 * contributors to this software may be used to endorse or promote products 00024 * derived from this software without specific written permission. 00025 * 4. This software, including modifications and/or derivative works of this 00026 * software, must execute solely and exclusively on microcontroller or 00027 * microprocessor devices manufactured by or for STMicroelectronics. 00028 * 5. Redistribution and use of this software other than as permitted under 00029 * this license is void and will automatically terminate your rights under 00030 * this license. 00031 * 00032 * THIS SOFTWARE IS PROVIDED BY STMICROELECTRONICS AND CONTRIBUTORS "AS IS" 00033 * AND ANY EXPRESS, IMPLIED OR STATUTORY WARRANTIES, INCLUDING, BUT NOT 00034 * LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY, FITNESS FOR A 00035 * PARTICULAR PURPOSE AND NON-INFRINGEMENT OF THIRD PARTY INTELLECTUAL PROPERTY 00036 * RIGHTS ARE DISCLAIMED TO THE FULLEST EXTENT PERMITTED BY LAW. IN NO EVENT 00037 * SHALL STMICROELECTRONICS OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, 00038 * INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT 00039 * LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, 00040 * OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF 00041 * LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING 00042 * NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, 00043 * EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00044 * 00045 ****************************************************************************** 00046 */ 00047 00048 /* Includes ------------------------------------------------------------------*/ 00049 #include "stm32l475e_iot01_gyro.h" 00050 00051 /** @addtogroup BSP 00052 * @{ 00053 */ 00054 00055 /** @addtogroup STM32L475E_IOT01 00056 * @{ 00057 */ 00058 00059 /** @defgroup STM32L475E_IOT01_GYROSCOPE GYROSCOPE 00060 * @{ 00061 */ 00062 00063 /** @defgroup STM32L475E_IOT01_GYROSCOPE_Private_Variables GYROSCOPE Private Variables 00064 * @{ 00065 */ 00066 static GYRO_DrvTypeDef *GyroscopeDrv; 00067 00068 /** 00069 * @} 00070 */ 00071 00072 00073 /** @defgroup STM32L475E_IOT01_GYROSCOPE_Private_Functions GYROSCOPE Private Functions 00074 * @{ 00075 */ 00076 /** 00077 * @brief Initialize Gyroscope. 00078 * @retval GYRO_OK or GYRO_ERROR 00079 */ 00080 uint8_t BSP_GYRO_Init(void) 00081 { 00082 uint8_t ret = GYRO_ERROR; 00083 uint16_t ctrl = 0x0000; 00084 GYRO_InitTypeDef LSM6DSL_InitStructure; 00085 00086 if(Lsm6dslGyroDrv.ReadID() != LSM6DSL_ACC_GYRO_WHO_AM_I) 00087 { 00088 ret = GYRO_ERROR; 00089 } 00090 else 00091 { 00092 /* Initialize the gyroscope driver structure */ 00093 GyroscopeDrv = &Lsm6dslGyroDrv; 00094 00095 /* Configure Mems : data rate, power mode, full scale and axes */ 00096 LSM6DSL_InitStructure.Power_Mode = 0; 00097 LSM6DSL_InitStructure.Output_DataRate = LSM6DSL_ODR_52Hz; 00098 LSM6DSL_InitStructure.Axes_Enable = 0; 00099 LSM6DSL_InitStructure.Band_Width = 0; 00100 LSM6DSL_InitStructure.BlockData_Update = LSM6DSL_BDU_BLOCK_UPDATE; 00101 LSM6DSL_InitStructure.Endianness = 0; 00102 LSM6DSL_InitStructure.Full_Scale = LSM6DSL_GYRO_FS_2000; 00103 00104 /* Configure MEMS: data rate, full scale */ 00105 ctrl = (LSM6DSL_InitStructure.Full_Scale | LSM6DSL_InitStructure.Output_DataRate); 00106 00107 /* Configure MEMS: BDU and Auto-increment for multi read/write */ 00108 ctrl |= ((LSM6DSL_InitStructure.BlockData_Update | LSM6DSL_ACC_GYRO_IF_INC_ENABLED) << 8); 00109 00110 /* Initialize component */ 00111 GyroscopeDrv->Init(ctrl); 00112 00113 ret = GYRO_OK; 00114 } 00115 00116 return ret; 00117 } 00118 00119 00120 /** 00121 * @brief DeInitialize Gyroscope. 00122 */ 00123 void BSP_GYRO_DeInit(void) 00124 { 00125 /* DeInitialize the Gyroscope IO interfaces */ 00126 if(GyroscopeDrv != NULL) 00127 { 00128 if(GyroscopeDrv->DeInit!= NULL) 00129 { 00130 GyroscopeDrv->DeInit(); 00131 } 00132 } 00133 } 00134 00135 00136 /** 00137 * @brief Set/Unset Gyroscope in low power mode. 00138 * @param status 0 means disable Low Power Mode, otherwise Low Power Mode is enabled 00139 */ 00140 void BSP_GYRO_LowPower(uint16_t status) 00141 { 00142 /* Set/Unset component in low-power mode */ 00143 if(GyroscopeDrv != NULL) 00144 { 00145 if(GyroscopeDrv->LowPower!= NULL) 00146 { 00147 GyroscopeDrv->LowPower(status); 00148 } 00149 } 00150 } 00151 00152 /** 00153 * @brief Get XYZ angular acceleration from the Gyroscope. 00154 * @param pfData: pointer on floating array 00155 */ 00156 void BSP_GYRO_GetXYZ(float* pfData) 00157 { 00158 if(GyroscopeDrv != NULL) 00159 { 00160 if(GyroscopeDrv->GetXYZ!= NULL) 00161 { 00162 GyroscopeDrv->GetXYZ(pfData); 00163 } 00164 } 00165 } 00166 00167 /** 00168 * @} 00169 */ 00170 00171 /** 00172 * @} 00173 */ 00174 00175 /** 00176 * @} 00177 */ 00178 00179 /** 00180 * @} 00181 */ 00182 00183 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Thu Mar 16 2017 10:38:32 for B-L475E-IOT01 BSP User Manual by
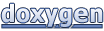