STM32L4xx_Nucleo BSP User Manual
|
Functions | |
static void | SPIx_Init (void) |
Initializes SPI HAL. | |
static void | SPIx_Write (uint8_t Value) |
SPI Write a byte to device. | |
static uint32_t | SPIx_Read (void) |
SPI Read 4 bytes from device. | |
static void | SPIx_Error (void) |
SPI error treatment function. | |
static void | SPIx_MspInit (void) |
Initializes SPI MSP. | |
static HAL_StatusTypeDef | ADCx_Init (void) |
Initializes ADC HAL. | |
static void | ADCx_MspInit (ADC_HandleTypeDef *hadc) |
Initializes ADC MSP. | |
void | SD_IO_Init (void) |
Initializes the SD Card and put it into StandBy State (Ready for data transfer). | |
HAL_StatusTypeDef | SD_IO_WriteCmd (uint8_t Cmd, uint32_t Arg, uint8_t Crc, uint8_t Response) |
Sends 5 bytes command to the SD card and get response. | |
HAL_StatusTypeDef | SD_IO_WaitResponse (uint8_t Response) |
Waits response from the SD card. | |
void | SD_IO_WriteDummy (void) |
Sends dummy byte with CS High. | |
void | SD_IO_WriteByte (uint8_t Data) |
Writes a byte on the SD. | |
uint8_t | SD_IO_ReadByte (void) |
Reads a byte from the SD. | |
void | LCD_IO_Init (void) |
Initializes the LCD. | |
void | LCD_IO_WriteMultipleData (uint8_t *pData, uint32_t Size) |
Write register value. | |
void | LCD_IO_WriteReg (uint8_t LCDReg) |
Writes command to select the LCD register. | |
void | LCD_Delay (uint32_t Delay) |
Wait for loop in ms. |
Function Documentation
static HAL_StatusTypeDef ADCx_Init | ( | void | ) | [static] |
Initializes ADC HAL.
- Return values:
-
None
Definition at line 809 of file stm32l4xx_nucleo.c.
References ADCx_MspInit(), hnucleo_Adc, and NUCLEO_ADCx.
Referenced by BSP_JOY_Init().
static void ADCx_MspInit | ( | ADC_HandleTypeDef * | hadc | ) | [static] |
Initializes ADC MSP.
- Return values:
-
None
Definition at line 779 of file stm32l4xx_nucleo.c.
References NUCLEO_ADCx_CLK_ENABLE, NUCLEO_ADCx_GPIO_CLK_ENABLE, NUCLEO_ADCx_GPIO_PIN, and NUCLEO_ADCx_GPIO_PORT.
Referenced by ADCx_Init().
void LCD_Delay | ( | uint32_t | Delay | ) |
Wait for loop in ms.
- Parameters:
-
Delay in ms.
- Return values:
-
None
Definition at line 766 of file stm32l4xx_nucleo.c.
void LCD_IO_Init | ( | void | ) |
Initializes the LCD.
- Return values:
-
None
Definition at line 664 of file stm32l4xx_nucleo.c.
References LCD_CS_GPIO_CLK_ENABLE, LCD_CS_HIGH, LCD_CS_PIN, LCD_DC_GPIO_CLK_ENABLE, LCD_DC_GPIO_PORT, LCD_DC_PIN, SD_CS_GPIO_PORT, and SPIx_Init().
void LCD_IO_WriteMultipleData | ( | uint8_t * | pData, |
uint32_t | Size | ||
) |
Write register value.
- Parameters:
-
pData Pointer on the register value Size Size of byte to transmit to the register
- Return values:
-
None
Definition at line 716 of file stm32l4xx_nucleo.c.
References hnucleo_Spi, LCD_CS_HIGH, LCD_CS_LOW, LCD_DC_HIGH, and SPIx_Write().
void LCD_IO_WriteReg | ( | uint8_t | LCDReg | ) |
Writes command to select the LCD register.
- Parameters:
-
LCDReg,: Address of the selected register.
- Return values:
-
None
Definition at line 695 of file stm32l4xx_nucleo.c.
References LCD_CS_HIGH, LCD_CS_LOW, LCD_DC_LOW, and SPIx_Write().
void SD_IO_Init | ( | void | ) |
Initializes the SD Card and put it into StandBy State (Ready for data transfer).
- Return values:
-
None
Definition at line 524 of file stm32l4xx_nucleo.c.
References SD_CS_GPIO_CLK_ENABLE, SD_CS_GPIO_PORT, SD_CS_HIGH, SD_CS_PIN, SD_DUMMY_BYTE, SD_IO_WriteByte(), and SPIx_Init().
uint8_t SD_IO_ReadByte | ( | void | ) |
Reads a byte from the SD.
- Return values:
-
The received byte.
Definition at line 570 of file stm32l4xx_nucleo.c.
References SPIx_Read().
Referenced by SD_IO_WaitResponse().
HAL_StatusTypeDef SD_IO_WaitResponse | ( | uint8_t | Response | ) |
Waits response from the SD card.
- Parameters:
-
Response,: Expected response from the SD card
- Return values:
-
HAL_StatusTypeDef HAL Status
Definition at line 624 of file stm32l4xx_nucleo.c.
References SD_IO_ReadByte().
Referenced by SD_IO_WriteCmd().
void SD_IO_WriteByte | ( | uint8_t | Data | ) |
Writes a byte on the SD.
- Parameters:
-
Data,: byte to send.
- Return values:
-
None
Definition at line 560 of file stm32l4xx_nucleo.c.
References SPIx_Write().
Referenced by SD_IO_Init(), SD_IO_WriteCmd(), and SD_IO_WriteDummy().
HAL_StatusTypeDef SD_IO_WriteCmd | ( | uint8_t | Cmd, |
uint32_t | Arg, | ||
uint8_t | Crc, | ||
uint8_t | Response | ||
) |
Sends 5 bytes command to the SD card and get response.
- Parameters:
-
Cmd,: The user expected command to send to SD card. Arg,: The command argument. Crc,: The CRC. Response,: Expected response from the SD card
- Return values:
-
HAL_StatusTypeDef HAL Status
Definition at line 589 of file stm32l4xx_nucleo.c.
References SD_CS_LOW, SD_IO_WaitResponse(), SD_IO_WriteByte(), and SD_NO_RESPONSE_EXPECTED.
void SD_IO_WriteDummy | ( | void | ) |
Sends dummy byte with CS High.
- Return values:
-
None
Definition at line 650 of file stm32l4xx_nucleo.c.
References SD_CS_HIGH, SD_DUMMY_BYTE, and SD_IO_WriteByte().
static void SPIx_Error | ( | void | ) | [static] |
SPI error treatment function.
- Return values:
-
None
Definition at line 505 of file stm32l4xx_nucleo.c.
References hnucleo_Spi, and SPIx_Init().
Referenced by SPIx_Read(), and SPIx_Write().
static void SPIx_Init | ( | void | ) | [static] |
Initializes SPI HAL.
- Return values:
-
None
Definition at line 427 of file stm32l4xx_nucleo.c.
References hnucleo_Spi, NUCLEO_SPIx, and SPIx_MspInit().
Referenced by LCD_IO_Init(), SD_IO_Init(), and SPIx_Error().
static void SPIx_MspInit | ( | void | ) | [static] |
Initializes SPI MSP.
- Return values:
-
None
Definition at line 392 of file stm32l4xx_nucleo.c.
References NUCLEO_SPIx_CLK_ENABLE, NUCLEO_SPIx_MISO_MOSI_AF, NUCLEO_SPIx_MISO_MOSI_GPIO_CLK_ENABLE, NUCLEO_SPIx_MISO_MOSI_GPIO_PORT, NUCLEO_SPIx_MISO_PIN, NUCLEO_SPIx_MOSI_PIN, NUCLEO_SPIx_SCK_AF, NUCLEO_SPIx_SCK_GPIO_CLK_ENABLE, NUCLEO_SPIx_SCK_GPIO_PORT, and NUCLEO_SPIx_SCK_PIN.
Referenced by SPIx_Init().
static uint32_t SPIx_Read | ( | void | ) | [static] |
SPI Read 4 bytes from device.
- Return values:
-
Read data
Definition at line 464 of file stm32l4xx_nucleo.c.
References hnucleo_Spi, hnucleo_SpixTimeout, and SPIx_Error().
Referenced by SD_IO_ReadByte().
static void SPIx_Write | ( | uint8_t | Value | ) | [static] |
SPI Write a byte to device.
- Parameters:
-
Value,: value to be written
- Return values:
-
None
Definition at line 487 of file stm32l4xx_nucleo.c.
References hnucleo_Spi, hnucleo_SpixTimeout, and SPIx_Error().
Referenced by LCD_IO_WriteMultipleData(), LCD_IO_WriteReg(), and SD_IO_WriteByte().
Generated on Fri Jun 19 2015 16:52:00 for STM32L4xx_Nucleo BSP User Manual by
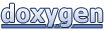