STM32L4xx_Nucleo BSP User Manual
|
stm32l4xx_nucleo.h
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l4xx_nucleo.h 00004 * @author MCD Application Team 00005 * @version $VERSION$ 00006 * @date $DATE$ 00007 * @brief This file contains definitions for: 00008 * - LEDs and push-button available on STM32L4XX-Nucleo Kit 00009 * from STMicroelectronics 00010 * - LCD, joystick and microSD available on Adafruit 1.8" TFT LCD 00011 * shield (reference ID 802) 00012 ****************************************************************************** 00013 * @attention 00014 * 00015 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00016 * 00017 * Redistribution and use in source and binary forms, with or without modification, 00018 * are permitted provided that the following conditions are met: 00019 * 1. Redistributions of source code must retain the above copyright notice, 00020 * this list of conditions and the following disclaimer. 00021 * 2. Redistributions in binary form must reproduce the above copyright notice, 00022 * this list of conditions and the following disclaimer in the documentation 00023 * and/or other materials provided with the distribution. 00024 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00025 * may be used to endorse or promote products derived from this software 00026 * without specific prior written permission. 00027 * 00028 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00029 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00030 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00031 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00032 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00033 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00034 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00035 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00036 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00037 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00038 * 00039 ****************************************************************************** 00040 */ 00041 00042 /* Define to prevent recursive inclusion -------------------------------------*/ 00043 #ifndef __STM32L4XX_NUCLEO_H 00044 #define __STM32L4XX_NUCLEO_H 00045 00046 #ifdef __cplusplus 00047 extern "C" { 00048 #endif 00049 00050 /** @addtogroup BSP 00051 * @{ 00052 */ 00053 00054 /** @addtogroup STM32L4XX_NUCLEO 00055 * @{ 00056 */ 00057 00058 /* Includes ------------------------------------------------------------------*/ 00059 #include "stm32l4xx_hal.h" 00060 00061 00062 /** @defgroup STM32L4XX_NUCLEO_Exported_Types Exported Types 00063 * @{ 00064 */ 00065 typedef enum 00066 { 00067 LED2 = 0, 00068 00069 LED_GREEN = LED2 00070 } Led_TypeDef; 00071 00072 typedef enum 00073 { 00074 BUTTON_USER = 0, 00075 /* Alias */ 00076 BUTTON_KEY = BUTTON_USER 00077 } Button_TypeDef; 00078 00079 typedef enum 00080 { 00081 BUTTON_MODE_GPIO = 0, 00082 BUTTON_MODE_EXTI = 1 00083 } ButtonMode_TypeDef; 00084 00085 typedef enum 00086 { 00087 JOY_NONE = 0, 00088 JOY_SEL = 1, 00089 JOY_DOWN = 2, 00090 JOY_LEFT = 3, 00091 JOY_RIGHT = 4, 00092 JOY_UP = 5 00093 } JOYState_TypeDef; 00094 00095 /** 00096 * @} 00097 */ 00098 00099 /** @defgroup STM32L4XX_NUCLEO_Exported_Constants Exported Constants 00100 * @{ 00101 */ 00102 00103 /** 00104 * @brief Define for STM32L4XX_NUCLEO board 00105 */ 00106 #if !defined (USE_STM32L4XX_NUCLEO) 00107 #define USE_STM32L4XX_NUCLEO 00108 #endif 00109 00110 /** @defgroup STM32L4XX_NUCLEO_LED LED Constants 00111 * @{ 00112 */ 00113 #define LEDn 1 00114 00115 #define LED2_PIN GPIO_PIN_5 00116 #define LED2_GPIO_PORT GPIOA 00117 #define LED2_GPIO_CLK_ENABLE() __HAL_RCC_GPIOA_CLK_ENABLE() 00118 #define LED2_GPIO_CLK_DISABLE() __HAL_RCC_GPIOA_CLK_DISABLE() 00119 00120 #define LEDx_GPIO_CLK_ENABLE(__LED__) do { if((__LED__) == LED2) { LED2_GPIO_CLK_ENABLE(); } } while(0) 00121 00122 #define LEDx_GPIO_CLK_DISABLE(__LED__) do { if((__LED__) == LED2) { LED2_GPIO_CLK_DISABLE(); } } while(0) 00123 /** 00124 * @} 00125 */ 00126 00127 /** @defgroup STM32L4XX_NUCLEO_BUTTON BUTTON Constants 00128 * @{ 00129 */ 00130 #define BUTTONn 1 00131 00132 /** 00133 * @brief Key push-button 00134 */ 00135 #define USER_BUTTON_PIN GPIO_PIN_13 00136 #define USER_BUTTON_GPIO_PORT GPIOC 00137 #define USER_BUTTON_GPIO_CLK_ENABLE() __HAL_RCC_GPIOC_CLK_ENABLE() 00138 #define USER_BUTTON_GPIO_CLK_DISABLE() __HAL_RCC_GPIOC_CLK_DISABLE() 00139 #define USER_BUTTON_EXTI_LINE GPIO_PIN_13 00140 #define USER_BUTTON_EXTI_IRQn EXTI15_10_IRQn 00141 /* Aliases */ 00142 #define KEY_BUTTON_PIN USER_BUTTON_PIN 00143 #define KEY_BUTTON_GPIO_PORT USER_BUTTON_GPIO_PORT 00144 #define KEY_BUTTON_GPIO_CLK_ENABLE() USER_BUTTON_GPIO_CLK_ENABLE() 00145 #define KEY_BUTTON_GPIO_CLK_DISABLE() USER_BUTTON_GPIO_CLK_DISABLE() 00146 #define KEY_BUTTON_EXTI_LINE USER_BUTTON_EXTI_LINE 00147 #define KEY_BUTTON_EXTI_IRQn USER_BUTTON_EXTI_IRQn 00148 00149 00150 #define BUTTONx_GPIO_CLK_ENABLE(__BUTTON__) do { if((__BUTTON__) == BUTTON_USER) { USER_BUTTON_GPIO_CLK_ENABLE(); } } while(0) 00151 00152 #define BUTTONx_GPIO_CLK_DISABLE(__BUTTON__) do { if((__BUTTON__) == BUTTON_USER) { USER_BUTTON_GPIO_CLK_DISABLE(); } } while(0) 00153 /** 00154 * @} 00155 */ 00156 00157 /** @addtogroup STM32L4XX_NUCLEO_BUS BUS Constants 00158 * @{ 00159 */ 00160 /*###################### SPI1 ###################################*/ 00161 #define NUCLEO_SPIx SPI1 00162 #define NUCLEO_SPIx_CLK_ENABLE() __HAL_RCC_SPI1_CLK_ENABLE() 00163 00164 #define NUCLEO_SPIx_SCK_AF GPIO_AF5_SPI1 00165 #define NUCLEO_SPIx_SCK_GPIO_PORT GPIOA 00166 #define NUCLEO_SPIx_SCK_PIN GPIO_PIN_5 00167 #define NUCLEO_SPIx_SCK_GPIO_CLK_ENABLE() __HAL_RCC_GPIOA_CLK_ENABLE() 00168 #define NUCLEO_SPIx_SCK_GPIO_CLK_DISABLE() __HAL_RCC_GPIOA_CLK_DISABLE() 00169 00170 #define NUCLEO_SPIx_MISO_MOSI_AF GPIO_AF5_SPI1 00171 #define NUCLEO_SPIx_MISO_MOSI_GPIO_PORT GPIOA 00172 #define NUCLEO_SPIx_MISO_MOSI_GPIO_CLK_ENABLE() __HAL_RCC_GPIOA_CLK_ENABLE() 00173 #define NUCLEO_SPIx_MISO_MOSI_GPIO_CLK_DISABLE() __HAL_RCC_GPIOA_CLK_DISABLE() 00174 #define NUCLEO_SPIx_MISO_PIN GPIO_PIN_6 00175 #define NUCLEO_SPIx_MOSI_PIN GPIO_PIN_7 00176 /* Maximum Timeout values for flags waiting loops. These timeouts are not based 00177 on accurate values, they just guarantee that the application will not remain 00178 stuck if the SPI communication is corrupted. 00179 You may modify these timeout values depending on CPU frequency and application 00180 conditions (interrupts routines ...). */ 00181 #define NUCLEO_SPIx_TIMEOUT_MAX 1000 00182 00183 00184 /** 00185 * @brief SD Control Lines management 00186 */ 00187 #define SD_CS_LOW() HAL_GPIO_WritePin(SD_CS_GPIO_PORT, SD_CS_PIN, GPIO_PIN_RESET) 00188 #define SD_CS_HIGH() HAL_GPIO_WritePin(SD_CS_GPIO_PORT, SD_CS_PIN, GPIO_PIN_SET) 00189 00190 /** 00191 * @brief LCD Control Lines management 00192 */ 00193 #define LCD_CS_LOW() HAL_GPIO_WritePin(LCD_CS_GPIO_PORT, LCD_CS_PIN, GPIO_PIN_RESET) 00194 #define LCD_CS_HIGH() HAL_GPIO_WritePin(LCD_CS_GPIO_PORT, LCD_CS_PIN, GPIO_PIN_SET) 00195 #define LCD_DC_LOW() HAL_GPIO_WritePin(LCD_DC_GPIO_PORT, LCD_DC_PIN, GPIO_PIN_RESET) 00196 #define LCD_DC_HIGH() HAL_GPIO_WritePin(LCD_DC_GPIO_PORT, LCD_DC_PIN, GPIO_PIN_SET) 00197 00198 /** 00199 * @brief SD Control Interface pins 00200 */ 00201 #define SD_CS_PIN GPIO_PIN_5 00202 #define SD_CS_GPIO_PORT GPIOB 00203 #define SD_CS_GPIO_CLK_ENABLE() __HAL_RCC_GPIOB_CLK_ENABLE() 00204 #define SD_CS_GPIO_CLK_DISABLE() __HAL_RCC_GPIOB_CLK_DISABLE() 00205 00206 /** 00207 * @brief LCD Control Interface pins 00208 */ 00209 #define LCD_CS_PIN GPIO_PIN_6 00210 #define LCD_CS_GPIO_PORT GPIOB 00211 #define LCD_CS_GPIO_CLK_ENABLE() __HAL_RCC_GPIOB_CLK_ENABLE() 00212 #define LCD_CS_GPIO_CLK_DISABLE() __HAL_RCC_GPIOB_CLK_DISABLE() 00213 00214 /** 00215 * @brief LCD Data/Command Interface pins 00216 */ 00217 #define LCD_DC_PIN GPIO_PIN_9 00218 #define LCD_DC_GPIO_PORT GPIOA 00219 #define LCD_DC_GPIO_CLK_ENABLE() __HAL_RCC_GPIOA_CLK_ENABLE() 00220 #define LCD_DC_GPIO_CLK_DISABLE() __HAL_RCC_GPIOA_CLK_DISABLE() 00221 00222 /*##################### ADC1 ###################################*/ 00223 /** 00224 * @brief ADC Interface pins 00225 * used to detect motion of Joystick available on Adafruit 1.8" TFT shield 00226 */ 00227 #define NUCLEO_ADCx ADC1 00228 #define NUCLEO_ADCx_CLK_ENABLE() __HAL_RCC_ADC_CLK_ENABLE() 00229 00230 #define NUCLEO_ADCx_GPIO_PORT GPIOB 00231 #define NUCLEO_ADCx_GPIO_PIN GPIO_PIN_0 00232 #define NUCLEO_ADCx_GPIO_CLK_ENABLE() __HAL_RCC_GPIOB_CLK_ENABLE() 00233 #define NUCLEO_ADCx_GPIO_CLK_DISABLE() __HAL_RCC_GPIOB_CLK_DISABLE() 00234 00235 /** 00236 * @} 00237 */ 00238 00239 /** 00240 * @} 00241 */ 00242 00243 /** @addtogroup STM32L4XX_NUCLEO_Exported_Functions 00244 * @{ 00245 */ 00246 uint32_t BSP_GetVersion(void); 00247 00248 /** @addtogroup STM32L4XX_NUCLEO_LED_Functions 00249 * @{ 00250 */ 00251 void BSP_LED_Init(Led_TypeDef Led); 00252 void BSP_LED_On(Led_TypeDef Led); 00253 void BSP_LED_Off(Led_TypeDef Led); 00254 void BSP_LED_Toggle(Led_TypeDef Led); 00255 /** 00256 * @} 00257 */ 00258 00259 /** @addtogroup STM32L4XX_NUCLEO_BUTTON_Functions 00260 * @{ 00261 */ 00262 void BSP_PB_Init(Button_TypeDef Button, ButtonMode_TypeDef ButtonMode); 00263 uint32_t BSP_PB_GetState(Button_TypeDef Button); 00264 #ifdef HAL_ADC_MODULE_ENABLED 00265 uint8_t BSP_JOY_Init(void); 00266 JOYState_TypeDef BSP_JOY_GetState(void); 00267 #endif /* HAL_ADC_MODULE_ENABLED */ 00268 /** 00269 * @} 00270 */ 00271 00272 /** 00273 * @} 00274 */ 00275 00276 /** 00277 * @} 00278 */ 00279 00280 /** 00281 * @} 00282 */ 00283 00284 #ifdef __cplusplus 00285 } 00286 #endif 00287 00288 #endif /* __STM32L4XX_NUCLEO_H */ 00289 00290 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/ 00291
Generated on Fri Jun 19 2015 16:52:00 for STM32L4xx_Nucleo BSP User Manual by
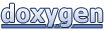