STM32L4R9I-Discovery BSP User Manual
|
stm32l4r9i_discovery_ts.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l4r9i_discovery_ts.c 00004 * @author MCD Application Team 00005 * @brief This file provides a set of functions needed to manage the Touch 00006 * Screen on DSI LCD of STM32L4R9I_DISCOVERY discovery board. 00007 ****************************************************************************** 00008 * @attention 00009 * 00010 * <h2><center>© COPYRIGHT(c) 2017 STMicroelectronics</center></h2> 00011 * 00012 * Redistribution and use in source and binary forms, with or without modification, 00013 * are permitted provided that the following conditions are met: 00014 * 1. Redistributions of source code must retain the above copyright notice, 00015 * this list of conditions and the following disclaimer. 00016 * 2. Redistributions in binary form must reproduce the above copyright notice, 00017 * this list of conditions and the following disclaimer in the documentation 00018 * and/or other materials provided with the distribution. 00019 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00020 * may be used to endorse or promote products derived from this software 00021 * without specific prior written permission. 00022 * 00023 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00024 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00025 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00026 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00027 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00028 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00029 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00030 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00031 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00032 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00033 * 00034 ****************************************************************************** 00035 */ 00036 00037 /* File Info : ----------------------------------------------------------------- 00038 User NOTES 00039 1. How To use this driver: 00040 -------------------------- 00041 - This driver is used to drive the touch screen module of the STM32L4R9I_DISCOVERY 00042 discovery board on the DSI LCD mounted on MB1314 daughter board. 00043 The touch screen driver IC is a FT3X67 type. 00044 00045 2. Driver description: 00046 --------------------- 00047 + Initialization steps: 00048 o Initialize the TS module using the BSP_TS_Init() function. This 00049 function includes the MSP layer hardware resources initialization and the 00050 communication layer configuration to start the TS use. The LCD size properties 00051 (x and y) are passed as parameters. 00052 o If TS interrupt mode is desired, you must configure the TS interrupt mode 00053 by calling the function BSP_TS_ITConfig(). The TS interrupt mode is generated 00054 as an external interrupt whenever a touch is detected. 00055 00056 + Touch screen use 00057 o The touch screen state is captured whenever the function BSP_TS_GetState() is 00058 used. This function returns information about the last LCD touch occurred 00059 in the TS_StateTypeDef structure. 00060 o FT3X67 doesn't support weight and area features (always 0 value returned). 00061 00062 ------------------------------------------------------------------------------*/ 00063 00064 /* Includes ------------------------------------------------------------------*/ 00065 #include "stm32l4r9i_discovery_ts.h" 00066 #include "math.h" 00067 00068 /** @addtogroup BSP 00069 * @{ 00070 */ 00071 00072 /** @addtogroup STM32L4R9I_DISCOVERY 00073 * @{ 00074 */ 00075 00076 /** @addtogroup STM32L4R9I_DISCOVERY_TS STM32L4R9I_DISCOVERY TS 00077 * @{ 00078 */ 00079 00080 /** @defgroup STM32L4R9I_DISCOVERY_TS_Private_Variables Private Variables 00081 * @{ 00082 */ 00083 00084 static TS_DrvTypeDef *tsDriver; 00085 static uint8_t I2C_Address = 0; 00086 static uint8_t HwRotation = 0; 00087 00088 /** 00089 * @} 00090 */ 00091 00092 /** @addtogroup STM32L4R9I_DISCOVERY_TS_Exported_Functions Exported Functions 00093 * @{ 00094 */ 00095 00096 /** 00097 * @brief Initialize and configures the touch screen functionalities and 00098 * configures all necessary hardware resources (GPIOs, I2C, clocks..). 00099 * @param ts_SizeX : Maximum X size of the TS area on LCD 00100 * @param ts_SizeY : Maximum Y size of the TS area on LCD 00101 * @retval TS_OK if all initializations are OK. Other value if error. 00102 */ 00103 uint8_t BSP_TS_Init(uint16_t ts_SizeX, uint16_t ts_SizeY) 00104 { 00105 uint8_t status = TS_OK; 00106 00107 /* Initialize the communication channel to sensor (I2C) if necessary */ 00108 /* that is initialization is done only once after a power up */ 00109 ft3x67_ts_drv.Init(TS_I2C_ADDRESS); 00110 00111 /* Scan FT3X67 TouchScreen IC controller ID register by I2C Read */ 00112 /* Verify this is a FT3X67, otherwise this is an error case */ 00113 if(ft3x67_ts_drv.ReadID(TS_I2C_ADDRESS) == FT3X67_ID_VALUE) 00114 { 00115 /* Found FT3X67 : Initialize the TS driver structure */ 00116 tsDriver = &ft3x67_ts_drv; 00117 I2C_Address = TS_I2C_ADDRESS; 00118 00119 /* Calibrate, Configure and Start the TouchScreen driver */ 00120 tsDriver->Start(I2C_Address); 00121 00122 /* Read firmware register to know if HW rotation is implemented */ 00123 if(TS_IO_Read(I2C_Address, FT3X67_FIRMID_REG) != 0x01) 00124 { 00125 HwRotation = 1; 00126 } 00127 } 00128 else 00129 { 00130 status = TS_DEVICE_NOT_FOUND; 00131 } 00132 return status; 00133 } 00134 00135 /** 00136 * @brief Deinitialize the TouchScreen. 00137 * @retval TS state 00138 */ 00139 uint8_t BSP_TS_DeInit(void) 00140 { 00141 /* Actually ts_driver does not provide a DeInit function */ 00142 return TS_OK; 00143 } 00144 00145 /** 00146 * @brief Return status and positions of the touch screen. 00147 * @param TS_State: Pointer to touch screen current state structure 00148 * @retval TS_OK if OK. Other value if error. 00149 * @note FT3X67 doesn't support weight and area features (always 0 value returned). 00150 */ 00151 uint8_t BSP_TS_GetState(TS_StateTypeDef *TS_State) 00152 { 00153 static uint32_t xf[TS_MAX_NB_TOUCH] = {0, 0}; /* Final x value */ 00154 static uint32_t yf[TS_MAX_NB_TOUCH] = {0, 0}; /* Final y value */ 00155 uint8_t ts_status = TS_OK; 00156 uint16_t x[TS_MAX_NB_TOUCH]; /* Initial x value */ 00157 uint16_t y[TS_MAX_NB_TOUCH]; /* Initial y value */ 00158 int16_t xc, yc; /* Coordinates transfered to center screen */ 00159 int16_t xr, yr; /* Coordinates after rotation */ 00160 uint32_t index; 00161 uint32_t weight = 0; 00162 uint32_t area = 0; 00163 uint32_t event = 0; 00164 00165 /* Check and update the number of touches active detected */ 00166 TS_State->touchDetected = tsDriver->DetectTouch(I2C_Address); 00167 if(TS_State->touchDetected) 00168 { 00169 for(index = 0; index < TS_State->touchDetected; index++) 00170 { 00171 /* Get each touch coordinates */ 00172 tsDriver->GetXY(I2C_Address, &(x[index]), &(y[index])); 00173 00174 if(HwRotation == 0) 00175 { 00176 /* First translate coordonates to center screen */ 00177 xc = x[index] - 195; 00178 yc = y[index] - 195; 00179 00180 /* Apply rotation of 45� */ 00181 xr = (int16_t) (sqrt(2) * (xc - yc) / 2); 00182 yr = (int16_t) (sqrt(2) * (xc + yc) / 2); 00183 00184 /* Revert the initial translation */ 00185 xf[index] = xr + 195; 00186 yf[index] = yr + 195; 00187 00188 TS_State->touchX[index] = xf[index]; 00189 TS_State->touchY[index] = yf[index]; 00190 } 00191 else 00192 { 00193 TS_State->touchX[index] = x[index]; 00194 TS_State->touchY[index] = y[index]; 00195 } 00196 00197 /* Get touch info related to the current touch */ 00198 ft3x67_TS_GetTouchInfo(I2C_Address, index, &weight, &area, &event); 00199 00200 /* Update TS_State structure */ 00201 TS_State->touchWeight[index] = weight; 00202 TS_State->touchArea[index] = area; 00203 00204 /* Remap touch event */ 00205 switch(event) 00206 { 00207 case FT3X67_TOUCH_EVT_FLAG_PRESS_DOWN : 00208 TS_State->touchEventId[index] = TOUCH_EVENT_PRESS_DOWN; 00209 break; 00210 case FT3X67_TOUCH_EVT_FLAG_LIFT_UP : 00211 TS_State->touchEventId[index] = TOUCH_EVENT_LIFT_UP; 00212 break; 00213 case FT3X67_TOUCH_EVT_FLAG_CONTACT : 00214 TS_State->touchEventId[index] = TOUCH_EVENT_CONTACT; 00215 break; 00216 case FT3X67_TOUCH_EVT_FLAG_NO_EVENT : 00217 TS_State->touchEventId[index] = TOUCH_EVENT_NO_EVT; 00218 break; 00219 default : 00220 ts_status = TS_ERROR; 00221 break; 00222 } /* of switch(event) */ 00223 00224 } /* of for(index=0; index < TS_State->touchDetected; index++) */ 00225 00226 } /* end of if(TS_State->touchDetected != 0) */ 00227 00228 return (ts_status); 00229 } 00230 00231 /** 00232 * @brief Configure gesture feature. 00233 * @param State: Enable/Disable gesture feature. 00234 * @retval TS_OK if OK. Other value if error. 00235 */ 00236 uint8_t BSP_TS_GestureConfig(FunctionalState State) 00237 { 00238 uint8_t ts_status = TS_OK; 00239 uint32_t Activation; 00240 00241 /* Configure gesture feature */ 00242 Activation = (State == ENABLE) ? FT3X67_GESTURE_ENABLE : FT3X67_GESTURE_DISABLE; 00243 ft3x67_TS_GestureConfig(I2C_Address, Activation); 00244 00245 return(ts_status); 00246 } 00247 00248 /** 00249 * @brief Update gesture Id following a touch detected. 00250 * @param TS_State: Pointer to touch screen current state structure 00251 * @retval TS_OK if OK. Other value if error. 00252 */ 00253 uint8_t BSP_TS_Get_GestureId(TS_StateTypeDef *TS_State) 00254 { 00255 uint32_t gestureId = 0; 00256 uint8_t ts_status = TS_OK; 00257 00258 /* Get gesture Id */ 00259 ft3x67_TS_GetGestureID(I2C_Address, &gestureId); 00260 00261 /* Remap gesture Id to a TS_GestureIdTypeDef value */ 00262 switch(gestureId) 00263 { 00264 case FT3X67_GEST_ID_NO_GESTURE : 00265 TS_State->gestureId = GEST_ID_NO_GESTURE; 00266 break; 00267 case FT3X67_GEST_ID_MOVE_UP : 00268 TS_State->gestureId = GEST_ID_MOVE_UP; 00269 break; 00270 case FT3X67_GEST_ID_MOVE_RIGHT : 00271 TS_State->gestureId = GEST_ID_MOVE_RIGHT; 00272 break; 00273 case FT3X67_GEST_ID_MOVE_DOWN : 00274 TS_State->gestureId = GEST_ID_MOVE_DOWN; 00275 break; 00276 case FT3X67_GEST_ID_MOVE_LEFT : 00277 TS_State->gestureId = GEST_ID_MOVE_LEFT; 00278 break; 00279 case FT3X67_GEST_ID_DOUBLE_CLICK : 00280 TS_State->gestureId = GEST_ID_DOUBLE_CLICK; 00281 break; 00282 default : 00283 ts_status = TS_ERROR; 00284 break; 00285 } /* of switch(gestureId) */ 00286 00287 return(ts_status); 00288 } 00289 00290 /** 00291 * @brief Reset all touch data before a new acquisition 00292 * of touch information. 00293 * @param TS_State: Pointer to touch screen current state structure 00294 * @retval TS_OK if OK, TS_ERROR if problem found. 00295 */ 00296 uint8_t BSP_TS_ResetTouchData(TS_StateTypeDef *TS_State) 00297 { 00298 uint8_t ts_status = TS_ERROR; 00299 uint32_t index; 00300 00301 if (TS_State != (TS_StateTypeDef *)NULL) 00302 { 00303 TS_State->gestureId = GEST_ID_NO_GESTURE; 00304 TS_State->touchDetected = 0; 00305 00306 for(index = 0; index < TS_MAX_NB_TOUCH; index++) 00307 { 00308 TS_State->touchX[index] = 0; 00309 TS_State->touchY[index] = 0; 00310 TS_State->touchArea[index] = 0; 00311 TS_State->touchEventId[index] = TOUCH_EVENT_NO_EVT; 00312 TS_State->touchWeight[index] = 0; 00313 } 00314 00315 ts_status = TS_OK; 00316 } /* of if (TS_State != (TS_StateTypeDef *)NULL) */ 00317 00318 return (ts_status); 00319 } 00320 00321 /** 00322 * @brief Configure and enable the touch screen interrupts. 00323 * @retval TS_OK if OK. Other value if error. 00324 */ 00325 uint8_t BSP_TS_ITConfig(void) 00326 { 00327 /* Enable the TS ITs */ 00328 tsDriver->EnableIT(I2C_Address); 00329 00330 /* Configure touchscreen interrupt pin */ 00331 BSP_IO_ConfigPin(TS_INT_PIN, IO_MODE_IT_FALLING_EDGE_PU); 00332 /* Configure IO Expander interrupt */ 00333 MFX_IO_ITConfig(); 00334 00335 return TS_OK; 00336 } 00337 00338 /** 00339 * @brief Deconfigure and disable the touch screen interrupts. 00340 * @retval TS_OK if OK. Other value if error. 00341 */ 00342 uint8_t BSP_TS_ITDisable(void) 00343 { 00344 /* Configure touchscreen interrupt pin */ 00345 BSP_IO_ConfigPin(TS_INT_PIN, IO_MODE_ANALOG); 00346 00347 /* Disable the TS ITs */ 00348 tsDriver->DisableIT(I2C_Address); 00349 00350 return TS_OK; 00351 } 00352 00353 /** 00354 * @brief Clear all touch screen interrupts. 00355 */ 00356 void BSP_TS_ITClear(void) 00357 { 00358 /* Empty function on component FT3X67 */ 00359 } 00360 00361 /** 00362 * @brief Get the touch screen interrupt status. 00363 * @retval TS_OK if OK. Other value if error. 00364 */ 00365 uint8_t BSP_TS_ITGetStatus(void) 00366 { 00367 /* Return the TS_OK because feature not available on FT3X67 */ 00368 return TS_OK; 00369 } 00370 00371 /** 00372 * @} 00373 */ 00374 00375 /** 00376 * @} 00377 */ 00378 00379 /** 00380 * @} 00381 */ 00382 00383 /** 00384 * @} 00385 */ 00386 00387 /** 00388 * @} 00389 */ 00390 00391 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Fri Oct 13 2017 02:37:42 for STM32L4R9I-Discovery BSP User Manual by
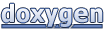