STM32L4R9I-Discovery BSP User Manual
|
stm32l4r9i_discovery_camera.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l4r9i_discovery_camera.c 00004 * @author MCD Application Team 00005 * @brief This file includes the driver for Camera modules mounted on 00006 * STM32L4R9I_DISCOVERY board. 00007 @verbatim 00008 How to use this driver: 00009 ------------------------ 00010 - This driver is used to drive the camera. 00011 - The OV9655 component driver MUST be included with this driver. 00012 00013 Driver description: 00014 ------------------- 00015 + Initialization steps: 00016 o Initialize the camera using the BSP_CAMERA_Init() function. 00017 o Start the camera capture/snapshot using the CAMERA_Start() function. 00018 o Suspend, resume or stop the camera capture using the following functions: 00019 - BSP_CAMERA_Suspend() 00020 - BSP_CAMERA_Resume() 00021 - BSP_CAMERA_Stop() 00022 00023 + Options 00024 o Increase or decrease on the fly the brightness and/or contrast 00025 using the following function: 00026 - BSP_CAMERA_ContrastBrightnessConfig 00027 o Add a special effect on the fly using the following functions: 00028 - BSP_CAMERA_BlackWhiteConfig() 00029 - BSP_CAMERA_ColorEffectConfig() 00030 @endverbatim 00031 ****************************************************************************** 00032 * @attention 00033 * 00034 * <h2><center>© COPYRIGHT(c) 2017 STMicroelectronics</center></h2> 00035 * 00036 * Redistribution and use in source and binary forms, with or without modification, 00037 * are permitted provided that the following conditions are met: 00038 * 1. Redistributions of source code must retain the above copyright notice, 00039 * this list of conditions and the following disclaimer. 00040 * 2. Redistributions in binary form must reproduce the above copyright notice, 00041 * this list of conditions and the following disclaimer in the documentation 00042 * and/or other materials provided with the distribution. 00043 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00044 * may be used to endorse or promote products derived from this software 00045 * without specific prior written permission. 00046 * 00047 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00048 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00049 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00050 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00051 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00052 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00053 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00054 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00055 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00056 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00057 * 00058 ****************************************************************************** 00059 */ 00060 00061 /* Includes ------------------------------------------------------------------*/ 00062 #include "stm32l4r9i_discovery_camera.h" 00063 #include "stm32l4r9i_discovery.h" 00064 #include "stm32l4r9i_discovery_io.h" 00065 00066 /** @addtogroup BSP 00067 * @{ 00068 */ 00069 00070 /** @addtogroup STM32L4R9I_DISCOVERY 00071 * @{ 00072 */ 00073 00074 /** @defgroup STM32L4R9I_DISCOVERY_CAMERA STM32L4R9I_DISCOVERY CAMERA 00075 * @{ 00076 */ 00077 00078 /** @defgroup STM32L4R9I_DISCOVERY_CAMERA_Private_Defines Private Defines 00079 * @{ 00080 */ 00081 #define CAMERA_VGA_RES_X 640 00082 #define CAMERA_VGA_RES_Y 480 00083 #define CAMERA_480x272_RES_X 480 00084 #define CAMERA_480x272_RES_Y 272 00085 #define CAMERA_QVGA_RES_X 320 00086 #define CAMERA_QVGA_RES_Y 240 00087 #define CAMERA_QQVGA_RES_X 160 00088 #define CAMERA_QQVGA_RES_Y 120 00089 /** 00090 * @} 00091 */ 00092 00093 /** @defgroup STM32L4R9I_DISCOVERY_CAMERA_Private_Variables Private Variables 00094 * @{ 00095 */ 00096 DCMI_HandleTypeDef hDcmiHandler; 00097 CAMERA_DrvTypeDef *camera_drv; 00098 /* Camera current resolution naming (QQVGA, VGA, ...) */ 00099 static uint32_t CameraCurrentResolution; 00100 00101 /* Camera module I2C HW address */ 00102 static uint32_t CameraHwAddress; 00103 00104 /** 00105 * @} 00106 */ 00107 00108 /** @defgroup STM32L4R9I_DISCOVERY_CAMERA_Private_FunctionPrototypes Private Function Prototypes 00109 * @{ 00110 */ 00111 static uint32_t GetSize(uint32_t resolution); 00112 /** 00113 * @} 00114 */ 00115 00116 /** @defgroup STM32L4R9I_DISCOVERY_CAMERA_Exported_Functions Exported Functions 00117 * @{ 00118 */ 00119 00120 /** 00121 * @brief Initializes the camera. 00122 * @param Resolution : camera sensor requested resolution (x, y) : standard resolution 00123 * naming QQVGA, QVGA, VGA ... 00124 * @retval Camera status 00125 */ 00126 uint8_t BSP_CAMERA_Init(uint32_t Resolution) 00127 { 00128 DCMI_HandleTypeDef *phdcmi; 00129 uint8_t status = CAMERA_ERROR; 00130 00131 /* Get the DCMI handle structure */ 00132 phdcmi = &hDcmiHandler; 00133 00134 00135 /* Initialize the IO functionalities */ 00136 BSP_IO_Init(); 00137 00138 /* Set up the Camera */ 00139 BSP_CAMERA_PwrUp(); 00140 00141 /*** Configures the DCMI to interface with the camera module ***/ 00142 /* DCMI configuration */ 00143 phdcmi->Init.CaptureRate = DCMI_CR_ALL_FRAME; 00144 phdcmi->Init.HSPolarity = DCMI_HSPOLARITY_HIGH; 00145 phdcmi->Init.SynchroMode = DCMI_SYNCHRO_HARDWARE; 00146 phdcmi->Init.VSPolarity = DCMI_VSPOLARITY_HIGH; 00147 phdcmi->Init.ExtendedDataMode = DCMI_EXTEND_DATA_8B; 00148 phdcmi->Init.PCKPolarity = DCMI_PCKPOLARITY_RISING; 00149 phdcmi->Init.ByteSelectMode = DCMI_BSM_ALL; 00150 phdcmi->Init.ByteSelectStart = DCMI_OEBS_ODD; 00151 phdcmi->Init.LineSelectMode = DCMI_LSM_ALL; 00152 phdcmi->Instance = DCMI; 00153 00154 /* Camera initialization */ 00155 BSP_CAMERA_MspInit(&hDcmiHandler, NULL); 00156 00157 /* Read ID of Camera module via I2C */ 00158 if(ov9655_ReadID(CAMERA_I2C_ADDRESS) == OV9655_ID) 00159 { 00160 /* Initialize the camera driver structure */ 00161 camera_drv = &ov9655_drv; 00162 CameraHwAddress = CAMERA_I2C_ADDRESS; 00163 00164 /* DCMI Initialization */ 00165 HAL_DCMI_Init(phdcmi); 00166 00167 camera_drv->Init(CameraHwAddress, Resolution); 00168 00169 /* Set the RGB565 mode */ 00170 MFX_IO_Write(CameraHwAddress, 0x12 /*OV9655_COM7*/, 0x63); 00171 MFX_IO_Write(CameraHwAddress, 0x40 /*OV9655_COM15*/, 0x10); 00172 /* Invert the HRef signal */ 00173 MFX_IO_Write(CameraHwAddress, 0x15 /*OV9655_COM10*/, 0x08); 00174 00175 00176 CameraCurrentResolution = Resolution; 00177 00178 /* Return CAMERA_OK status */ 00179 status = CAMERA_OK; 00180 } 00181 else 00182 { 00183 /* Return CAMERA_NOT_SUPPORTED status */ 00184 status = CAMERA_NOT_SUPPORTED; 00185 } 00186 00187 return status; 00188 } 00189 00190 /** 00191 * @brief DeInitializes the camera. 00192 * @retval Camera status 00193 */ 00194 uint8_t BSP_CAMERA_DeInit(void) 00195 { 00196 hDcmiHandler.Instance = DCMI; 00197 00198 HAL_DCMI_DeInit(&hDcmiHandler); 00199 BSP_CAMERA_MspDeInit(&hDcmiHandler, NULL); 00200 00201 /* Set Camera in Power Down */ 00202 BSP_CAMERA_PwrDown(); 00203 00204 return CAMERA_OK; 00205 } 00206 00207 /** 00208 * @brief Starts the camera capture in continuous mode. 00209 * @param buff: pointer to the camera output buffer 00210 * @retval None 00211 */ 00212 void BSP_CAMERA_ContinuousStart(uint8_t *buff) 00213 { 00214 /* Start the camera capture */ 00215 HAL_DCMI_Start_DMA(&hDcmiHandler, DCMI_MODE_CONTINUOUS, (uint32_t)buff, GetSize(CameraCurrentResolution)); 00216 } 00217 00218 /** 00219 * @brief Starts the camera capture in snapshot mode. 00220 * @param buff: pointer to the camera output buffer 00221 * @retval None 00222 */ 00223 void BSP_CAMERA_SnapshotStart(uint8_t *buff) 00224 { 00225 /* Start the camera capture */ 00226 HAL_DCMI_Start_DMA(&hDcmiHandler, DCMI_MODE_SNAPSHOT, (uint32_t)buff, GetSize(CameraCurrentResolution)); 00227 } 00228 00229 /** 00230 * @brief Suspend the CAMERA capture 00231 * @retval None 00232 */ 00233 void BSP_CAMERA_Suspend(void) 00234 { 00235 /* Suspend the Camera Capture */ 00236 HAL_DCMI_Suspend(&hDcmiHandler); 00237 } 00238 00239 /** 00240 * @brief Resume the CAMERA capture 00241 * @retval None 00242 */ 00243 void BSP_CAMERA_Resume(void) 00244 { 00245 /* Start the Camera Capture */ 00246 HAL_DCMI_Resume(&hDcmiHandler); 00247 } 00248 00249 /** 00250 * @brief Stop the CAMERA capture 00251 * @retval Camera status 00252 */ 00253 uint8_t BSP_CAMERA_Stop(void) 00254 { 00255 uint8_t status = CAMERA_ERROR; 00256 00257 if(HAL_DCMI_Stop(&hDcmiHandler) == HAL_OK) 00258 { 00259 status = CAMERA_OK; 00260 } 00261 00262 return status; 00263 } 00264 00265 /** 00266 * @brief CANERA power up 00267 * @retval None 00268 */ 00269 void BSP_CAMERA_PwrUp(void) 00270 { 00271 /* De-assert the camera POWER_DOWN pin (active high) */ 00272 BSP_IO_WritePin(CAMERA_PWR_EN_PIN, GPIO_PIN_RESET); 00273 00274 HAL_Delay(3); /* POWER_DOWN de-asserted during 3ms */ 00275 } 00276 00277 /** 00278 * @brief CAMERA power down 00279 * @retval None 00280 */ 00281 void BSP_CAMERA_PwrDown(void) 00282 { 00283 /* Assert the camera POWER_DOWN pin (active high) */ 00284 BSP_IO_WritePin(CAMERA_PWR_EN_PIN, GPIO_PIN_SET); 00285 } 00286 00287 /** 00288 * @brief Configures the camera contrast and brightness. 00289 * @param contrast_level: Contrast level 00290 * This parameter can be one of the following values: 00291 * @arg CAMERA_CONTRAST_LEVEL4: for contrast +2 00292 * @arg CAMERA_CONTRAST_LEVEL3: for contrast +1 00293 * @arg CAMERA_CONTRAST_LEVEL2: for contrast 0 00294 * @arg CAMERA_CONTRAST_LEVEL1: for contrast -1 00295 * @arg CAMERA_CONTRAST_LEVEL0: for contrast -2 00296 * @param brightness_level: Contrast level 00297 * This parameter can be one of the following values: 00298 * @arg CAMERA_BRIGHTNESS_LEVEL4: for brightness +2 00299 * @arg CAMERA_BRIGHTNESS_LEVEL3: for brightness +1 00300 * @arg CAMERA_BRIGHTNESS_LEVEL2: for brightness 0 00301 * @arg CAMERA_BRIGHTNESS_LEVEL1: for brightness -1 00302 * @arg CAMERA_BRIGHTNESS_LEVEL0: for brightness -2 00303 * @retval None 00304 */ 00305 void BSP_CAMERA_ContrastBrightnessConfig(uint32_t contrast_level, uint32_t brightness_level) 00306 { 00307 if(camera_drv->Config != NULL) 00308 { 00309 camera_drv->Config(CameraHwAddress, CAMERA_CONTRAST_BRIGHTNESS, contrast_level, brightness_level); 00310 } 00311 } 00312 00313 /** 00314 * @brief Configures the camera white balance. 00315 * @param Mode: black_white mode 00316 * This parameter can be one of the following values: 00317 * @arg CAMERA_BLACK_WHITE_BW 00318 * @arg CAMERA_BLACK_WHITE_NEGATIVE 00319 * @arg CAMERA_BLACK_WHITE_BW_NEGATIVE 00320 * @arg CAMERA_BLACK_WHITE_NORMAL 00321 * @retval None 00322 */ 00323 void BSP_CAMERA_BlackWhiteConfig(uint32_t Mode) 00324 { 00325 if(camera_drv->Config != NULL) 00326 { 00327 camera_drv->Config(CameraHwAddress, CAMERA_BLACK_WHITE, Mode, 0); 00328 } 00329 } 00330 00331 /** 00332 * @brief Configures the camera color effect. 00333 * @param Effect: Color effect 00334 * This parameter can be one of the following values: 00335 * @arg CAMERA_COLOR_EFFECT_ANTIQUE 00336 * @arg CAMERA_COLOR_EFFECT_BLUE 00337 * @arg CAMERA_COLOR_EFFECT_GREEN 00338 * @arg CAMERA_COLOR_EFFECT_RED 00339 * @retval None 00340 */ 00341 void BSP_CAMERA_ColorEffectConfig(uint32_t Effect) 00342 { 00343 if(camera_drv->Config != NULL) 00344 { 00345 camera_drv->Config(CameraHwAddress, CAMERA_COLOR_EFFECT, Effect, 0); 00346 } 00347 } 00348 00349 /** 00350 * @brief Get the capture size in pixels unit. 00351 * @param resolution: the current resolution. 00352 * @retval capture size in pixels unit. 00353 */ 00354 static uint32_t GetSize(uint32_t resolution) 00355 { 00356 uint32_t size = 0; 00357 00358 /* Get capture size */ 00359 switch (resolution) 00360 { 00361 case CAMERA_R160x120: 00362 { 00363 size = 0x2580; 00364 } 00365 break; 00366 case CAMERA_R320x240: 00367 { 00368 size = 0x9600; 00369 } 00370 break; 00371 case CAMERA_R480x272: 00372 { 00373 size = 0xFF00; 00374 } 00375 break; 00376 case CAMERA_R640x480: 00377 { 00378 size = 0x25800; 00379 } 00380 break; 00381 default: 00382 { 00383 break; 00384 } 00385 } 00386 00387 return size; 00388 } 00389 00390 /** 00391 * @brief Initializes the DCMI MSP. 00392 * @param hdcmi: HDMI handle 00393 * @param Params 00394 * @retval None 00395 */ 00396 __weak void BSP_CAMERA_MspInit(DCMI_HandleTypeDef *hdcmi, void *Params) 00397 { 00398 static DMA_HandleTypeDef hdma_handler; 00399 GPIO_InitTypeDef gpio_init_structure; 00400 00401 /*** Enable peripherals and GPIO clocks ***/ 00402 /* Enable DCMI clock */ 00403 __HAL_RCC_DCMI_CLK_ENABLE(); 00404 00405 /* Enable DMA2 clock */ 00406 __HAL_RCC_DMA2_CLK_ENABLE(); 00407 /* Enable DMAmux1 clock to be able to use DMA_REQUEST_DCMI */ 00408 __HAL_RCC_DMAMUX1_CLK_ENABLE(); 00409 00410 /* Enable GPIO clocks */ 00411 __HAL_RCC_GPIOC_CLK_ENABLE(); 00412 __HAL_RCC_GPIOH_CLK_ENABLE(); 00413 __HAL_RCC_GPIOE_CLK_ENABLE(); 00414 __HAL_RCC_GPIOI_CLK_ENABLE(); 00415 __HAL_RCC_GPIOA_CLK_ENABLE(); 00416 00417 HAL_RCC_MCOConfig(RCC_MCO1, RCC_MCO1SOURCE_SYSCLK, RCC_MCODIV_4); /* PA8 Camera modul input clock 20 MHz */ 00418 __HAL_RCC_HSI48_ENABLE(); 00419 HAL_Delay(10); // HSI48 should start in 10ms 00420 00421 /*** Configure the GPIO ***/ 00422 /* Configure DCMI GPIO as alternate function */ 00423 gpio_init_structure.Pin = GPIO_PIN_6 | GPIO_PIN_7; 00424 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00425 gpio_init_structure.Pull = GPIO_NOPULL; 00426 gpio_init_structure.Speed = GPIO_SPEED_HIGH; 00427 gpio_init_structure.Alternate = GPIO_AF10_DCMI; 00428 HAL_GPIO_Init(GPIOC, &gpio_init_structure); 00429 00430 gpio_init_structure.Pin = GPIO_PIN_11 | GPIO_PIN_12 | GPIO_PIN_5; 00431 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00432 gpio_init_structure.Pull = GPIO_NOPULL; 00433 gpio_init_structure.Speed = GPIO_SPEED_HIGH; 00434 gpio_init_structure.Alternate = GPIO_AF10_DCMI; 00435 HAL_GPIO_Init(GPIOH, &gpio_init_structure); 00436 00437 gpio_init_structure.Pin = GPIO_PIN_4; 00438 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00439 gpio_init_structure.Pull = GPIO_NOPULL; 00440 gpio_init_structure.Speed = GPIO_SPEED_HIGH; 00441 gpio_init_structure.Alternate = GPIO_AF10_DCMI; 00442 HAL_GPIO_Init(GPIOE, &gpio_init_structure); 00443 00444 gpio_init_structure.Pin = GPIO_PIN_4 | GPIO_PIN_7 | GPIO_PIN_5; 00445 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00446 gpio_init_structure.Pull = GPIO_NOPULL; 00447 gpio_init_structure.Speed = GPIO_SPEED_HIGH; 00448 gpio_init_structure.Alternate = GPIO_AF10_DCMI; 00449 HAL_GPIO_Init(GPIOI, &gpio_init_structure); 00450 00451 gpio_init_structure.Pin = GPIO_PIN_4; 00452 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00453 gpio_init_structure.Pull = GPIO_NOPULL; 00454 gpio_init_structure.Speed = GPIO_SPEED_HIGH; 00455 gpio_init_structure.Alternate = GPIO_AF10_DCMI; 00456 HAL_GPIO_Init(GPIOA, &gpio_init_structure); 00457 00458 /*** Configure the DMA ***/ 00459 /* Set the parameters to be configured */ 00460 hdma_handler.Instance = BSP_CAMERA_DMA_INSTANCE; 00461 00462 hdma_handler.Init.Request = DMA_REQUEST_DCMI; 00463 hdma_handler.Init.Direction = DMA_PERIPH_TO_MEMORY; 00464 hdma_handler.Init.PeriphInc = DMA_PINC_DISABLE; 00465 hdma_handler.Init.MemInc = DMA_MINC_ENABLE; /* Image captured by the DCMI is stored in memory */ 00466 hdma_handler.Init.PeriphDataAlignment = DMA_PDATAALIGN_WORD; 00467 hdma_handler.Init.MemDataAlignment = DMA_MDATAALIGN_WORD; 00468 hdma_handler.Init.Mode = DMA_CIRCULAR; 00469 hdma_handler.Init.Priority = DMA_PRIORITY_HIGH; 00470 00471 /* Associate the initialized DMA handle to the DCMI handle */ 00472 __HAL_LINKDMA(hdcmi, DMA_Handle, hdma_handler); 00473 00474 /*** Configure the NVIC for DCMI and DMA ***/ 00475 /* NVIC configuration for DCMI transfer complete interrupt */ 00476 HAL_NVIC_SetPriority(DCMI_IRQn, 0x0F, 0); 00477 HAL_NVIC_EnableIRQ(DCMI_IRQn); 00478 00479 /* NVIC configuration for DMA2D transfer complete interrupt */ 00480 HAL_NVIC_SetPriority(DMA2_Channel6_IRQn, 0x0F, 0); 00481 HAL_NVIC_EnableIRQ(DMA2_Channel6_IRQn); 00482 00483 /* Configure the DMA stream */ 00484 HAL_DMA_Init(hdcmi->DMA_Handle); 00485 } 00486 00487 00488 /** 00489 * @brief DeInitializes the DCMI MSP. 00490 * @param hdcmi: HDMI handle 00491 * @param Params 00492 * @retval None 00493 */ 00494 __weak void BSP_CAMERA_MspDeInit(DCMI_HandleTypeDef *hdcmi, void *Params) 00495 { 00496 /* Disable NVIC for DCMI transfer complete interrupt */ 00497 HAL_NVIC_DisableIRQ(DCMI_IRQn); 00498 00499 /* Disable NVIC for DMA2 transfer complete interrupt */ 00500 HAL_NVIC_DisableIRQ(DMA2_Channel6_IRQn); 00501 00502 /* Configure the DMA stream */ 00503 HAL_DMA_DeInit(hdcmi->DMA_Handle); 00504 00505 /* Disable DCMI clock */ 00506 __HAL_RCC_DCMI_CLK_DISABLE(); 00507 00508 /* GPIO pins clock and DMA clock can be shut down in the application 00509 by surcharging this __weak function */ 00510 } 00511 00512 /** 00513 * @brief Line event callback 00514 * @param hdcmi: pointer to the DCMI handle 00515 * @retval None 00516 */ 00517 void HAL_DCMI_LineEventCallback(DCMI_HandleTypeDef *hdcmi) 00518 { 00519 BSP_CAMERA_LineEventCallback(); 00520 } 00521 00522 /** 00523 * @brief Line Event callback. 00524 * @retval None 00525 */ 00526 __weak void BSP_CAMERA_LineEventCallback(void) 00527 { 00528 /* NOTE : This function should not be modified; when the callback is needed, 00529 the BSP_CAMERA_LineEventCallback can be implemented in the user file 00530 */ 00531 } 00532 00533 /** 00534 * @brief VSYNC event callback 00535 * @param hdcmi: pointer to the DCMI handle 00536 * @retval None 00537 */ 00538 void HAL_DCMI_VsyncEventCallback(DCMI_HandleTypeDef *hdcmi) 00539 { 00540 BSP_CAMERA_VsyncEventCallback(); 00541 } 00542 00543 /** 00544 * @brief VSYNC Event callback. 00545 * @retval None 00546 */ 00547 __weak void BSP_CAMERA_VsyncEventCallback(void) 00548 { 00549 /* NOTE : This function should not be modified; when the callback is needed, 00550 the BSP_CAMERA_VsyncEventCallback can be implemented in the user file 00551 */ 00552 } 00553 00554 /** 00555 * @brief Frame event callback 00556 * @param hdcmi: pointer to the DCMI handle 00557 * @retval None 00558 */ 00559 void HAL_DCMI_FrameEventCallback(DCMI_HandleTypeDef *hdcmi) 00560 { 00561 BSP_CAMERA_FrameEventCallback(); 00562 } 00563 00564 /** 00565 * @brief Frame Event callback. 00566 * @retval None 00567 */ 00568 __weak void BSP_CAMERA_FrameEventCallback(void) 00569 { 00570 /* NOTE : This function should not be modified; when the callback is needed, 00571 the BSP_CAMERA_FrameEventCallback can be implemented in the user file 00572 */ 00573 } 00574 00575 /** 00576 * @brief Error callback 00577 * @param hdcmi: pointer to the DCMI handle 00578 * @retval None 00579 */ 00580 void HAL_DCMI_ErrorCallback(DCMI_HandleTypeDef *hdcmi) 00581 { 00582 BSP_CAMERA_ErrorCallback(); 00583 } 00584 00585 /** 00586 * @brief Error callback. 00587 * @retval None 00588 */ 00589 __weak void BSP_CAMERA_ErrorCallback(void) 00590 { 00591 /* NOTE : This function should not be modified; when the callback is needed, 00592 the BSP_CAMERA_ErrorCallback can be implemented in the user file 00593 */ 00594 } 00595 00596 00597 /** 00598 * @} 00599 */ 00600 00601 /** 00602 * @} 00603 */ 00604 00605 /** 00606 * @} 00607 */ 00608 00609 /** 00610 * @} 00611 */ 00612 00613 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Fri Oct 13 2017 02:37:42 for STM32L4R9I-Discovery BSP User Manual by
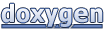