STM32L4R9I-Discovery BSP User Manual
|
stm32l4r9i_discovery.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l4r9i_discovery.c 00004 * @author MCD Application Team 00005 * @brief This file provides a set of firmware functions to manage Leds, 00006 * push-button and joystick of STM32L4R9I_DISCOVERY board (MB1311) 00007 ****************************************************************************** 00008 * @attention 00009 * 00010 * <h2><center>© COPYRIGHT(c) 2017 STMicroelectronics</center></h2> 00011 * 00012 * Redistribution and use in source and binary forms, with or without modification, 00013 * are permitted provided that the following conditions are met: 00014 * 1. Redistributions of source code must retain the above copyright notice, 00015 * this list of conditions and the following disclaimer. 00016 * 2. Redistributions in binary form must reproduce the above copyright notice, 00017 * this list of conditions and the following disclaimer in the documentation 00018 * and/or other materials provided with the distribution. 00019 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00020 * may be used to endorse or promote products derived from this software 00021 * without specific prior written permission. 00022 * 00023 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00024 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00025 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00026 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00027 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00028 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00029 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00030 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00031 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00032 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00033 * 00034 ****************************************************************************** 00035 */ 00036 00037 /* Includes ------------------------------------------------------------------*/ 00038 #include "stm32l4r9i_discovery.h" 00039 #include "stm32l4r9i_discovery_io.h" 00040 #include "stm32l4r9i_discovery_lcd.h" 00041 00042 /** @addtogroup BSP 00043 * @{ 00044 */ 00045 00046 /** @defgroup STM32L4R9I_DISCOVERY STM32L4R9I_DISCOVERY 00047 * @{ 00048 */ 00049 00050 /** @defgroup STM32L4R9I_DISCOVERY_Common STM32L4R9I_DISCOVERY Common 00051 * @{ 00052 */ 00053 00054 /** @defgroup STM32L4R9I_DISCOVERY_Private_Defines Private Defines 00055 * @{ 00056 */ 00057 00058 /** 00059 * @brief STM32L4R9I_DISCOVERY BSP Driver version number 00060 */ 00061 #define __STM32L4R9I_DISCOVERY_BSP_VERSION_MAIN (0x01) /*!< [31:24] main version */ 00062 #define __STM32L4R9I_DISCOVERY_BSP_VERSION_SUB1 (0x00) /*!< [23:16] sub1 version */ 00063 #define __STM32L4R9I_DISCOVERY_BSP_VERSION_SUB2 (0x00) /*!< [15:8] sub2 version */ 00064 #define __STM32L4R9I_DISCOVERY_BSP_VERSION_RC (0x00) /*!< [7:0] release candidate */ 00065 #define __STM32L4R9I_DISCOVERY_BSP_VERSION ((__STM32L4R9I_DISCOVERY_BSP_VERSION_MAIN << 24)\ 00066 |(__STM32L4R9I_DISCOVERY_BSP_VERSION_SUB1 << 16)\ 00067 |(__STM32L4R9I_DISCOVERY_BSP_VERSION_SUB2 << 8 )\ 00068 |(__STM32L4R9I_DISCOVERY_BSP_VERSION_RC)) 00069 00070 #ifdef HAL_I2C_MODULE_ENABLED 00071 /** 00072 * @brief BSP I2C users 00073 */ 00074 #define BSP_I2C_NO_USER 0x00000000U 00075 #define BSP_I2C_AUDIO_USER 0x00000001U 00076 #define BSP_I2C_TS_USER 0x00000002U 00077 #define BSP_I2C_MFX_USER 0x00000004U 00078 #define BSP_I2C_CAMERA_USER 0x00000008U 00079 #define BSP_I2C_ALL_USERS 0x0000000FU 00080 #endif 00081 00082 /** 00083 * @} 00084 */ 00085 00086 00087 /** @defgroup STM32L4R9I_DISCOVERY_Exported_Variables Exported Variables 00088 * @{ 00089 */ 00090 00091 /** 00092 * @brief LED variables 00093 */ 00094 const uint16_t LED_PIN[LEDn] = {LED1_PIN, 00095 LED2_PIN}; 00096 00097 /** 00098 * @brief COM port variables 00099 */ 00100 #if defined(HAL_UART_MODULE_ENABLED) 00101 USART_TypeDef* COM_USART[COMn] = {DISCOVERY_COM1}; 00102 GPIO_TypeDef* COM_TX_PORT[COMn] = {DISCOVERY_COM1_TX_GPIO_PORT}; 00103 GPIO_TypeDef* COM_RX_PORT[COMn] = {DISCOVERY_COM1_RX_GPIO_PORT}; 00104 const uint16_t COM_TX_PIN[COMn] = {DISCOVERY_COM1_TX_PIN}; 00105 const uint16_t COM_RX_PIN[COMn] = {DISCOVERY_COM1_RX_PIN}; 00106 const uint16_t COM_TX_AF[COMn] = {DISCOVERY_COM1_TX_AF}; 00107 const uint16_t COM_RX_AF[COMn] = {DISCOVERY_COM1_RX_AF}; 00108 #endif /* HAL_UART_MODULE_ENABLED */ 00109 00110 /** 00111 * @brief BUS variables 00112 */ 00113 #if defined(HAL_I2C_MODULE_ENABLED) 00114 uint32_t I2c1Timeout = DISCOVERY_I2C1_TIMEOUT_MAX; /*<! Value of Timeout when I2C1 communication fails */ 00115 static I2C_HandleTypeDef I2c1Handle = {0}; 00116 static uint32_t v_bspI2cUsers = BSP_I2C_NO_USER; 00117 #if defined(BSP_USE_CMSIS_OS) 00118 static osSemaphoreId BspI2cSemaphore; 00119 #endif 00120 #endif /* HAL_I2C_MODULE_ENABLED */ 00121 00122 /* LCD/PSRAM initialization status sharing the same power source */ 00123 uint32_t bsp_lcd_initialized = 0; 00124 uint32_t bsp_psram_initialized = 0; 00125 00126 /** 00127 * @} 00128 */ 00129 00130 /** @defgroup STM32L4R9I_DISCOVERY_Private_FunctionPrototypes Private Functions Prototypes 00131 * @{ 00132 */ 00133 /**************************** Bus functions ************************************/ 00134 /* I2C1 bus function */ 00135 #if defined(HAL_I2C_MODULE_ENABLED) 00136 static void I2C1_Init(uint32_t user); 00137 static void I2C1_MspInit(I2C_HandleTypeDef *hi2c); 00138 void I2C1_DeInit(uint32_t user); 00139 static void I2C1_MspDeInit(I2C_HandleTypeDef *hi2c); 00140 static void I2C1_WriteData(uint16_t Addr, uint16_t Reg, uint16_t RegSize, uint8_t Value); 00141 static HAL_StatusTypeDef I2C1_WriteBuffer(uint16_t Addr, uint16_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length); 00142 static uint8_t I2C1_ReadData(uint16_t Addr, uint16_t Reg, uint16_t RegSize); 00143 static HAL_StatusTypeDef I2C1_ReadBuffer(uint16_t Addr, uint16_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length); 00144 static void I2C1_Error(void); 00145 static HAL_StatusTypeDef I2C1_ReadMultiple(uint8_t Addr, uint16_t Reg, uint16_t MemAddSize, uint8_t *Buffer, uint16_t Length); 00146 static HAL_StatusTypeDef I2C1_WriteMultiple(uint8_t Addr, uint16_t Reg, uint16_t MemAddSize, uint8_t *Buffer, uint16_t Length); 00147 static uint8_t I2C1_isDeviceReady(uint16_t Addr, uint32_t Trials); 00148 #endif/* HAL_I2C_MODULE_ENABLED */ 00149 00150 /**************************** Link functions ***********************************/ 00151 #if defined(HAL_I2C_MODULE_ENABLED) 00152 00153 /* Link functions for Audio Codec peripheral */ 00154 void AUDIO_IO_Init(void); 00155 void AUDIO_IO_DeInit(void); 00156 void AUDIO_IO_Write(uint8_t Addr, uint8_t Reg, uint8_t Value); 00157 uint8_t AUDIO_IO_Read(uint8_t Addr, uint8_t Reg); 00158 void AUDIO_IO_Delay(uint32_t delay); 00159 00160 /* TouchScreen (TS) IO functions */ 00161 void TS_IO_Init(void); 00162 void TS_IO_Write(uint8_t Addr, uint8_t Reg, uint8_t Value); 00163 uint8_t TS_IO_Read(uint8_t Addr, uint8_t Reg); 00164 uint16_t TS_IO_ReadMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length); 00165 void TS_IO_WriteMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length); 00166 void TS_IO_Delay(uint32_t Delay); 00167 00168 /* CAMERA IO functions */ 00169 void CAMERA_IO_Init(void); 00170 void CAMERA_Delay(uint32_t Delay); 00171 void CAMERA_IO_Write(uint8_t Addr, uint8_t Reg, uint8_t Value); 00172 uint8_t CAMERA_IO_Read(uint8_t Addr, uint8_t Reg); 00173 00174 /* Link functions for IDD measurment */ 00175 void MFX_IO_Init(void); 00176 void MFX_IO_DeInit(void); 00177 void MFX_IO_ITConfig (void); 00178 void MFX_IO_EnableWakeupPin(void); 00179 void MFX_IO_Wakeup(void); 00180 void MFX_IO_Delay(uint32_t delay); 00181 void MFX_IO_Write(uint16_t addr, uint8_t reg, uint8_t value); 00182 uint8_t MFX_IO_Read(uint16_t addr, uint8_t reg); 00183 void MFX_IO_WriteMultiple(uint16_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length); 00184 uint16_t MFX_IO_ReadMultiple(uint16_t addr, uint8_t reg, uint8_t *buffer, uint16_t length); 00185 00186 #endif/* HAL_I2C_MODULE_ENABLED */ 00187 /** 00188 * @} 00189 */ 00190 00191 /** @defgroup STM32L4R9I_DISCOVERY_Exported_Functions Exported Functions 00192 * @{ 00193 */ 00194 00195 /** 00196 * @brief Error Handler 00197 * @note Defined as a weak function to be overwritten by the application. 00198 * @retval None 00199 */ 00200 __weak void BSP_ErrorHandler(void) 00201 { 00202 while(1); 00203 } 00204 00205 /** 00206 * @brief This method returns the STM32L4R9I DISCOVERY BSP Driver revision 00207 * @retval version : 0xXYZR (8bits for each decimal, R for RC) 00208 */ 00209 uint32_t BSP_GetVersion(void) 00210 { 00211 return __STM32L4R9I_DISCOVERY_BSP_VERSION; 00212 } 00213 00214 /** 00215 * @brief Configures LED GPIOs. 00216 * @param Led: Specifies the Led to be configured. 00217 * This parameter can be one of following parameters: 00218 * @arg LED1 00219 * @arg LED2 00220 * @retval None 00221 */ 00222 void BSP_LED_Init(Led_TypeDef Led) 00223 { 00224 GPIO_InitTypeDef GPIO_InitStructure; 00225 00226 if (Led == LED2) 00227 { 00228 /* Enable the GPIO_LED clock */ 00229 LED2_GPIO_CLK_ENABLE(); 00230 00231 /* Configure the GPIO_LED pin */ 00232 GPIO_InitStructure.Pin = LED_PIN[Led]; 00233 GPIO_InitStructure.Mode = GPIO_MODE_OUTPUT_PP; 00234 GPIO_InitStructure.Pull = GPIO_NOPULL; 00235 GPIO_InitStructure.Speed = GPIO_SPEED_FREQ_VERY_HIGH; 00236 00237 HAL_GPIO_Init(LED2_GPIO_PORT, &GPIO_InitStructure); 00238 /* By default, turn off LED */ 00239 HAL_GPIO_WritePin(LED2_GPIO_PORT, GPIO_InitStructure.Pin, GPIO_PIN_SET); 00240 } 00241 else 00242 { 00243 /* Initialize the IO functionalities */ 00244 if (BSP_IO_Init() == IO_ERROR) 00245 { 00246 BSP_ErrorHandler(); 00247 } 00248 00249 /* By default, turn off LED */ 00250 BSP_IO_WritePin(LED_PIN[Led], GPIO_PIN_SET); 00251 00252 BSP_IO_ConfigPin(LED_PIN[Led],IO_MODE_OUTPUT); 00253 } 00254 00255 } 00256 00257 00258 /** 00259 * @brief Unconfigures LED GPIOs. 00260 * @param Led: Specifies the Led to be unconfigured. 00261 * This parameter can be one of following parameters: 00262 * @arg LED1 00263 * @arg LED2 00264 * @retval None 00265 */ 00266 void BSP_LED_DeInit(Led_TypeDef Led) 00267 { 00268 if (Led == LED2) 00269 { 00270 /* Enable the GPIO_LED clock */ 00271 LED2_GPIO_CLK_ENABLE(); 00272 00273 HAL_GPIO_DeInit(LED2_GPIO_PORT, LED_PIN[Led]); 00274 } 00275 } 00276 00277 00278 /** 00279 * @brief Turns selected LED On. 00280 * @param Led: Specifies the Led to be set on. 00281 * This parameter can be one of following parameters: 00282 * @arg LED1 00283 * @arg LED2 00284 * @retval None 00285 */ 00286 void BSP_LED_On(Led_TypeDef Led) 00287 { 00288 if (Led == LED2) 00289 { 00290 HAL_GPIO_WritePin(LED2_GPIO_PORT, LED_PIN[Led], GPIO_PIN_RESET); 00291 } 00292 else 00293 { 00294 BSP_IO_WritePin(LED_PIN[Led], GPIO_PIN_RESET); 00295 } 00296 } 00297 00298 /** 00299 * @brief Turns selected LED Off. 00300 * @param Led: Specifies the Led to be set off. 00301 * This parameter can be one of following parameters: 00302 * @arg LED1 00303 * @arg LED2 00304 * @retval None 00305 */ 00306 void BSP_LED_Off(Led_TypeDef Led) 00307 { 00308 if (Led == LED2) 00309 { 00310 HAL_GPIO_WritePin(LED2_GPIO_PORT, LED_PIN[Led], GPIO_PIN_SET); 00311 } 00312 else 00313 { 00314 BSP_IO_WritePin(LED_PIN[Led], GPIO_PIN_SET); 00315 } 00316 } 00317 00318 /** 00319 * @brief Toggles the selected LED. 00320 * @param Led: Specifies the Led to be toggled. 00321 * This parameter can be one of following parameters: 00322 * @arg LED1 00323 * @arg LED2 00324 * @retval None 00325 */ 00326 void BSP_LED_Toggle(Led_TypeDef Led) 00327 { 00328 if (Led == LED2) 00329 { 00330 HAL_GPIO_TogglePin(LED2_GPIO_PORT, LED_PIN[Led]); 00331 } 00332 else 00333 { 00334 BSP_IO_TogglePin(LED_PIN[Led]); 00335 } 00336 } 00337 00338 /** 00339 * @brief Configures all buttons of the joystick in GPIO or EXTI modes. 00340 * @param Joy_Mode: Joystick mode. 00341 * This parameter can be one of the following values: 00342 * @arg JOY_MODE_GPIO: Joystick pins will be used as simple IOs 00343 * @arg JOY_MODE_EXTI: Joystick pins will be connected to EXTI line 00344 * with interrupt generation capability 00345 * @retval HAL_OK: if all initializations are OK. Other value if error. 00346 */ 00347 uint8_t BSP_JOY_Init(JOYMode_TypeDef Joy_Mode) 00348 { 00349 uint8_t Status = HAL_OK; 00350 GPIO_InitTypeDef GPIO_InitStruct; 00351 00352 /* Initialized BSP IO */ 00353 BSP_IO_Init(); 00354 /* Common configuration for GPIO used for joystick SEL */ 00355 SEL_JOY_GPIO_CLK_ENABLE(); 00356 GPIO_InitStruct.Pin = SEL_JOY_PIN; 00357 GPIO_InitStruct.Pull = GPIO_PULLDOWN; 00358 GPIO_InitStruct.Speed = GPIO_SPEED_LOW; 00359 00360 if(Joy_Mode == JOY_MODE_GPIO) 00361 { 00362 /* Configure GPIO used for joystick SEL as input */ 00363 GPIO_InitStruct.Mode = GPIO_MODE_INPUT; 00364 HAL_GPIO_Init(SEL_JOY_GPIO_PORT, &GPIO_InitStruct); 00365 00366 /* Configure other joystick pins */ 00367 BSP_IO_ConfigPin((RIGHT_JOY_PIN | LEFT_JOY_PIN | UP_JOY_PIN | DOWN_JOY_PIN), IO_MODE_INPUT_PU); 00368 } 00369 else if(Joy_Mode == JOY_MODE_EXTI) 00370 { 00371 /* Configure GPIO used for joystick SEL as input with External interrupt */ 00372 GPIO_InitStruct.Mode = GPIO_MODE_IT_RISING; 00373 HAL_GPIO_Init(SEL_JOY_GPIO_PORT, &GPIO_InitStruct); 00374 /* Enable and set corresponding EXTI Interrupt to the lowest priority */ 00375 HAL_NVIC_SetPriority((IRQn_Type)(SEL_JOY_EXTI_IRQn), 0x0F, 0x00); 00376 HAL_NVIC_EnableIRQ((IRQn_Type)(SEL_JOY_EXTI_IRQn)); 00377 00378 /* Configure other joystick pins */ 00379 BSP_IO_ConfigPin((RIGHT_JOY_PIN | LEFT_JOY_PIN | UP_JOY_PIN | DOWN_JOY_PIN), IO_MODE_IT_HIGH_LEVEL_PD); 00380 /* Configure IO Expander interrupt */ 00381 MFX_IO_ITConfig(); 00382 } 00383 else 00384 { 00385 Status = HAL_ERROR; 00386 } 00387 return Status; 00388 } 00389 00390 /** 00391 * @brief Unonfigures all GPIOs used as buttons of the joystick. 00392 * @retval None. 00393 */ 00394 void BSP_JOY_DeInit(void) 00395 { 00396 /* Enable the JOY clock */ 00397 SEL_JOY_GPIO_CLK_ENABLE(); 00398 HAL_GPIO_DeInit(SEL_JOY_GPIO_PORT, SEL_JOY_PIN); 00399 00400 BSP_IO_ConfigPin((RIGHT_JOY_PIN | LEFT_JOY_PIN | UP_JOY_PIN | DOWN_JOY_PIN), IO_MODE_OFF); 00401 } 00402 00403 /** 00404 * @brief Returns the current joystick status. 00405 * @retval Code of the joystick key pressed 00406 * This code can be one of the following values: 00407 * @arg JOY_NONE 00408 * @arg JOY_SEL 00409 * @arg JOY_DOWN 00410 * @arg JOY_LEFT 00411 * @arg JOY_RIGHT 00412 * @arg JOY_UP 00413 */ 00414 JOYState_TypeDef BSP_JOY_GetState(void) 00415 { 00416 uint32_t tmp = 0; 00417 00418 if(HAL_GPIO_ReadPin(SEL_JOY_GPIO_PORT, SEL_JOY_PIN) != GPIO_PIN_RESET) 00419 { 00420 return JOY_SEL; 00421 } 00422 00423 tmp = BSP_IO_ReadPin((RIGHT_JOY_PIN | LEFT_JOY_PIN | UP_JOY_PIN | DOWN_JOY_PIN)); 00424 /* Check the pressed joystick button */ 00425 if(tmp & DOWN_JOY_PIN) 00426 { 00427 return JOY_DOWN; 00428 } 00429 else if(tmp & LEFT_JOY_PIN) 00430 { 00431 return JOY_LEFT; 00432 } 00433 else if(tmp & RIGHT_JOY_PIN) 00434 { 00435 return JOY_RIGHT; 00436 } 00437 else if(tmp & UP_JOY_PIN) 00438 { 00439 return JOY_UP; 00440 } 00441 else 00442 { 00443 return JOY_NONE; 00444 } 00445 } 00446 00447 #if defined(HAL_UART_MODULE_ENABLED) 00448 /** 00449 * @brief Configures COM port. 00450 * @param COM: COM port to be configured. 00451 * This parameter can be one of the following values: 00452 * @arg COM1 00453 * @param huart: Pointer to a UART_HandleTypeDef structure that contains the 00454 * configuration information for the specified USART peripheral. 00455 */ 00456 void BSP_COM_Init(COM_TypeDef COM, UART_HandleTypeDef *huart) 00457 { 00458 GPIO_InitTypeDef gpio_init_structure; 00459 00460 /* Enable GPIO clock */ 00461 DISCOVERY_COMx_TX_GPIO_CLK_ENABLE(COM); 00462 DISCOVERY_COMx_RX_GPIO_CLK_ENABLE(COM); 00463 00464 /* Enable USART clock */ 00465 DISCOVERY_COMx_CLK_ENABLE(COM); 00466 00467 /* Configure USART Tx as alternate function */ 00468 gpio_init_structure.Pin = COM_TX_PIN[COM]; 00469 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00470 gpio_init_structure.Speed = GPIO_SPEED_FREQ_HIGH; 00471 gpio_init_structure.Pull = GPIO_NOPULL; 00472 gpio_init_structure.Alternate = COM_TX_AF[COM]; 00473 HAL_GPIO_Init(COM_TX_PORT[COM], &gpio_init_structure); 00474 00475 /* Configure USART Rx as alternate function */ 00476 gpio_init_structure.Pin = COM_RX_PIN[COM]; 00477 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00478 gpio_init_structure.Alternate = COM_RX_AF[COM]; 00479 HAL_GPIO_Init(COM_RX_PORT[COM], &gpio_init_structure); 00480 00481 /* USART configuration */ 00482 huart->Instance = COM_USART[COM]; 00483 HAL_UART_Init(huart); 00484 } 00485 00486 /** 00487 * @brief DeInit COM port. 00488 * @param COM: COM port to be configured. 00489 * This parameter can be one of the following values: 00490 * @arg COM1 00491 * @param huart: Pointer to a UART_HandleTypeDef structure that contains the 00492 * configuration information for the specified USART peripheral. 00493 */ 00494 void BSP_COM_DeInit(COM_TypeDef COM, UART_HandleTypeDef *huart) 00495 { 00496 /* USART deinitialization */ 00497 huart->Instance = COM_USART[COM]; 00498 HAL_UART_DeInit(huart); 00499 00500 /* Disable USART clock */ 00501 DISCOVERY_COMx_CLK_DISABLE(COM); 00502 00503 /* USART TX/RX pins deinitializations */ 00504 HAL_GPIO_DeInit(COM_TX_PORT[COM], COM_TX_PIN[COM]); 00505 HAL_GPIO_DeInit(COM_RX_PORT[COM], COM_RX_PIN[COM]); 00506 00507 /* Disable GPIOs clock is left for application */ 00508 } 00509 #endif /* HAL_UART_MODULE_ENABLED */ 00510 00511 /** 00512 * @} 00513 */ 00514 00515 /** @defgroup STM32L4R9I_DISCOVERY_Private_Functions Private Functions 00516 * @{ 00517 */ 00518 00519 /******************************************************************************* 00520 BUS OPERATIONS 00521 *******************************************************************************/ 00522 00523 #if defined(HAL_I2C_MODULE_ENABLED) 00524 /******************************* I2C Routines**********************************/ 00525 /** 00526 * @brief Discovery I2C1 Bus initialization 00527 * @retval None 00528 */ 00529 static void I2C1_Init(uint32_t user) 00530 { 00531 if(HAL_I2C_GetState(&I2c1Handle) == HAL_I2C_STATE_RESET) 00532 { 00533 #if defined(BSP_USE_CMSIS_OS) 00534 /* Create semaphore to prevent multiple I2C access */ 00535 osSemaphoreDef(BSP_I2C_SEM); 00536 BspI2cSemaphore = osSemaphoreCreate(osSemaphore(BSP_I2C_SEM), 1); 00537 #endif 00538 00539 I2c1Handle.Instance = I2C1; 00540 I2c1Handle.Init.Timing = DISCOVERY_I2C1_TIMING; 00541 I2c1Handle.Init.OwnAddress1 = 0x70; 00542 I2c1Handle.Init.AddressingMode = I2C_ADDRESSINGMODE_7BIT; 00543 I2c1Handle.Init.DualAddressMode = I2C_DUALADDRESS_DISABLE; 00544 I2c1Handle.Init.OwnAddress2 = 0xFF; 00545 I2c1Handle.Init.GeneralCallMode = I2C_GENERALCALL_DISABLE; 00546 I2c1Handle.Init.NoStretchMode = I2C_NOSTRETCH_DISABLE; 00547 00548 /* Init the I2C */ 00549 I2C1_MspInit(&I2c1Handle); 00550 HAL_I2C_Init(&I2c1Handle); 00551 } 00552 /* Update BSP I2C users list */ 00553 v_bspI2cUsers |= user; 00554 } 00555 00556 /** 00557 * @brief Discovery I2C1 MSP Initialization 00558 * @param hi2c: I2C1 handle 00559 * @retval None 00560 */ 00561 static void I2C1_MspInit(I2C_HandleTypeDef *hi2c) 00562 { 00563 GPIO_InitTypeDef GPIO_InitStructure; 00564 RCC_PeriphCLKInitTypeDef RCC_PeriphCLKInitStruct; 00565 00566 if (hi2c->Instance == I2C1) 00567 { 00568 /*##-1- Configure the Discovery I2C1 clock source. The clock is derived from the SYSCLK #*/ 00569 RCC_PeriphCLKInitStruct.PeriphClockSelection = RCC_PERIPHCLK_I2C1; 00570 RCC_PeriphCLKInitStruct.I2c1ClockSelection = RCC_I2C1CLKSOURCE_SYSCLK; 00571 HAL_RCCEx_PeriphCLKConfig(&RCC_PeriphCLKInitStruct); 00572 00573 /*##-2- Configure the GPIOs ################################################*/ 00574 /* Enable GPIO clock */ 00575 __HAL_RCC_GPIOB_CLK_ENABLE(); 00576 /* VddIO2 must be enabled to access GPIO port G[2:15] */ 00577 __HAL_RCC_PWR_CLK_ENABLE(); 00578 HAL_PWREx_EnableVddIO2(); 00579 __HAL_RCC_GPIOG_CLK_ENABLE(); 00580 00581 /* Configure I2C pins as alternate function */ 00582 GPIO_InitStructure.Pin = GPIO_PIN_6; 00583 GPIO_InitStructure.Mode = GPIO_MODE_AF_OD; 00584 GPIO_InitStructure.Pull = GPIO_PULLUP; 00585 GPIO_InitStructure.Speed = GPIO_SPEED_FREQ_VERY_HIGH; 00586 GPIO_InitStructure.Alternate = GPIO_AF4_I2C1; 00587 HAL_GPIO_Init(GPIOB, &GPIO_InitStructure); /* I2C1_SCL on PB6 */ 00588 GPIO_InitStructure.Pin = GPIO_PIN_13; 00589 HAL_GPIO_Init(GPIOG, &GPIO_InitStructure); /* I2C1_SDA on PG13 */ 00590 00591 /*##-3- Configure the Discovery I2C1 peripheral #############################*/ 00592 /* Enable I2C1 clock */ 00593 __HAL_RCC_I2C1_CLK_ENABLE(); 00594 00595 /* Force and release the I2C1 Peripheral */ 00596 __HAL_RCC_I2C1_FORCE_RESET(); 00597 __HAL_RCC_I2C1_RELEASE_RESET(); 00598 00599 /* Enable and set I2C1 event interrupt to the highest priority */ 00600 HAL_NVIC_SetPriority(I2C1_EV_IRQn, 0x00, 0); 00601 HAL_NVIC_EnableIRQ(I2C1_EV_IRQn); 00602 00603 /* Enable and set Discovery I2C1 error interrupt to the highest priority */ 00604 HAL_NVIC_SetPriority(I2C1_ER_IRQn, 0x00, 0); 00605 HAL_NVIC_EnableIRQ(I2C1_ER_IRQn); 00606 } 00607 } 00608 00609 /** 00610 * @brief Discovery I2C1 Bus Deinitialization 00611 * @retval None 00612 */ 00613 void I2C1_DeInit(uint32_t user) 00614 { 00615 /* Update BSP I2C users list */ 00616 v_bspI2cUsers &= ~(user); 00617 00618 if((HAL_I2C_GetState(&I2c1Handle) != HAL_I2C_STATE_RESET) && (v_bspI2cUsers == BSP_I2C_NO_USER)) 00619 { 00620 /* DeInit the I2C */ 00621 HAL_I2C_DeInit(&I2c1Handle); 00622 I2C1_MspDeInit(&I2c1Handle); 00623 00624 #if defined(BSP_USE_CMSIS_OS) 00625 /* Delete semaphore to prevent multiple I2C access */ 00626 osSemaphoreDelete(BspI2cSemaphore); 00627 #endif 00628 } 00629 } 00630 00631 /** 00632 * @brief Discovery I2C1 MSP DeInitialization 00633 * @param hi2c: I2C1 handle 00634 * @retval None 00635 */ 00636 static void I2C1_MspDeInit(I2C_HandleTypeDef *hi2c) 00637 { 00638 if (hi2c->Instance == I2C1) 00639 { 00640 /*##-1- Unconfigure the GPIOs ################################################*/ 00641 /* Enable GPIO clock */ 00642 __HAL_RCC_GPIOB_CLK_ENABLE(); 00643 /* VddIO2 must be enabled to access GPIO port G[2:15] */ 00644 __HAL_RCC_PWR_CLK_ENABLE(); 00645 HAL_PWREx_EnableVddIO2(); 00646 __HAL_RCC_GPIOG_CLK_ENABLE(); 00647 00648 /* Reset configuration of I2C GPIOs as alternate function */ 00649 HAL_GPIO_DeInit(GPIOB, GPIO_PIN_6); /* I2C1_SCL */ 00650 HAL_GPIO_DeInit(GPIOG, GPIO_PIN_13); /* I2C1_SDA */ 00651 00652 /*##-2- Unconfigure the Discovery I2C1 peripheral ############################*/ 00653 /* Force and release I2C1 Peripheral */ 00654 __HAL_RCC_I2C1_FORCE_RESET(); 00655 __HAL_RCC_I2C1_RELEASE_RESET(); 00656 00657 /* Disable I2C1 clock */ 00658 __HAL_RCC_I2C1_CLK_DISABLE(); 00659 00660 /* Disable I2C1 interrupts */ 00661 HAL_NVIC_DisableIRQ(I2C1_EV_IRQn); 00662 HAL_NVIC_DisableIRQ(I2C1_ER_IRQn); 00663 } 00664 } 00665 00666 /** 00667 * @brief Write a value in a register of the device through BUS. 00668 * @param Addr: Device address on BUS Bus. 00669 * @param Reg: The target register address to write 00670 * @param RegSize: The target register size (can be 8BIT or 16BIT) 00671 * @param Value: The target register value to be written 00672 * @retval None 00673 */ 00674 static void I2C1_WriteData(uint16_t Addr, uint16_t Reg, uint16_t RegSize, uint8_t Value) 00675 { 00676 HAL_StatusTypeDef status = HAL_OK; 00677 00678 #if defined(BSP_USE_CMSIS_OS) 00679 /* Get semaphore to prevent multiple I2C access */ 00680 osSemaphoreWait(BspI2cSemaphore, osWaitForever); 00681 #endif 00682 00683 status = HAL_I2C_Mem_Write(&I2c1Handle, Addr, (uint16_t)Reg, RegSize, &Value, 1, I2c1Timeout); 00684 00685 #if defined(BSP_USE_CMSIS_OS) 00686 /* Release semaphore to prevent multiple I2C access */ 00687 osSemaphoreRelease(BspI2cSemaphore); 00688 #endif 00689 00690 /* Check the communication status */ 00691 if(status != HAL_OK) 00692 { 00693 /* Re-Initiaize the BUS */ 00694 I2C1_Error(); 00695 } 00696 } 00697 00698 /** 00699 * @brief Write a value in a register of the device through BUS. 00700 * @param Addr: Device address on BUS Bus. 00701 * @param Reg: The target register address to write 00702 * @param RegSize: The target register size (can be 8BIT or 16BIT) 00703 * @param pBuffer: The target register value to be written 00704 * @param Length: buffer size to be written 00705 * @retval None 00706 */ 00707 static HAL_StatusTypeDef I2C1_WriteBuffer(uint16_t Addr, uint16_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length) 00708 { 00709 HAL_StatusTypeDef status = HAL_OK; 00710 00711 #if defined(BSP_USE_CMSIS_OS) 00712 /* Get semaphore to prevent multiple I2C access */ 00713 osSemaphoreWait(BspI2cSemaphore, osWaitForever); 00714 #endif 00715 00716 status = HAL_I2C_Mem_Write(&I2c1Handle, Addr, (uint16_t)Reg, RegSize, pBuffer, Length, I2c1Timeout); 00717 00718 #if defined(BSP_USE_CMSIS_OS) 00719 /* Release semaphore to prevent multiple I2C access */ 00720 osSemaphoreRelease(BspI2cSemaphore); 00721 #endif 00722 00723 /* Check the communication status */ 00724 if(status != HAL_OK) 00725 { 00726 /* Re-Initiaize the BUS */ 00727 I2C1_Error(); 00728 } 00729 00730 return status; 00731 } 00732 00733 /** 00734 * @brief Read a register of the device through BUS 00735 * @param Addr: Device address on BUS 00736 * @param Reg: The target register address to read 00737 * @param RegSize: The target register size (can be 8BIT or 16BIT) 00738 * @retval read register value 00739 */ 00740 static uint8_t I2C1_ReadData(uint16_t Addr, uint16_t Reg, uint16_t RegSize) 00741 { 00742 HAL_StatusTypeDef status = HAL_OK; 00743 uint8_t value = 0x0; 00744 00745 #if defined(BSP_USE_CMSIS_OS) 00746 /* Get semaphore to prevent multiple I2C access */ 00747 osSemaphoreWait(BspI2cSemaphore, osWaitForever); 00748 #endif 00749 00750 status = HAL_I2C_Mem_Read(&I2c1Handle, Addr, Reg, RegSize, &value, 1, I2c1Timeout); 00751 00752 #if defined(BSP_USE_CMSIS_OS) 00753 /* Release semaphore to prevent multiple I2C access */ 00754 osSemaphoreRelease(BspI2cSemaphore); 00755 #endif 00756 00757 /* Check the communication status */ 00758 if(status != HAL_OK) 00759 { 00760 /* Re-Initiaize the BUS */ 00761 I2C1_Error(); 00762 } 00763 00764 return value; 00765 } 00766 00767 /** 00768 * @brief Checks if target device is ready for communication. 00769 * @note This function is used with Memory devices 00770 * @param Addr: Target device address 00771 * @param Trials: Number of trials 00772 * @retval HAL status 00773 */ 00774 static uint8_t I2C1_isDeviceReady(uint16_t Addr, uint32_t Trials) 00775 { 00776 HAL_StatusTypeDef status = HAL_OK; 00777 00778 #if defined(BSP_USE_CMSIS_OS) 00779 /* Get semaphore to prevent multiple I2C access */ 00780 osSemaphoreWait(BspI2cSemaphore, osWaitForever); 00781 #endif 00782 00783 status = HAL_I2C_IsDeviceReady(&I2c1Handle, Addr, Trials, 50); 00784 00785 #if defined(BSP_USE_CMSIS_OS) 00786 /* Release semaphore to prevent multiple I2C access */ 00787 osSemaphoreRelease(BspI2cSemaphore); 00788 #endif 00789 00790 /* Check the communication status */ 00791 if(status != HAL_OK) 00792 { 00793 /* Re-Initiaize the BUS */ 00794 I2C1_Error(); 00795 } 00796 00797 return status; 00798 } 00799 00800 /** 00801 * @brief Reads multiple data on the BUS. 00802 * @param Addr: I2C Address 00803 * @param Reg: Reg Address 00804 * @param RegSize : The target register size (can be 8BIT or 16BIT) 00805 * @param pBuffer: pointer to read data buffer 00806 * @param Length: length of the data 00807 * @retval 0 if no problems to read multiple data 00808 */ 00809 static HAL_StatusTypeDef I2C1_ReadBuffer(uint16_t Addr, uint16_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length) 00810 { 00811 HAL_StatusTypeDef status = HAL_OK; 00812 00813 #if defined(BSP_USE_CMSIS_OS) 00814 /* Get semaphore to prevent multiple I2C access */ 00815 osSemaphoreWait(BspI2cSemaphore, osWaitForever); 00816 #endif 00817 00818 status = HAL_I2C_Mem_Read(&I2c1Handle, Addr, (uint16_t)Reg, RegSize, pBuffer, Length, I2c1Timeout); 00819 00820 #if defined(BSP_USE_CMSIS_OS) 00821 /* Release semaphore to prevent multiple I2C access */ 00822 osSemaphoreRelease(BspI2cSemaphore); 00823 #endif 00824 00825 /* Check the communication status */ 00826 if(status != HAL_OK) 00827 { 00828 /* Re-Initiaize the BUS */ 00829 I2C1_Error(); 00830 } 00831 00832 return status; 00833 } 00834 00835 /** 00836 * @brief Discovery I2C1 error treatment function 00837 * @retval None 00838 */ 00839 static void I2C1_Error(void) 00840 { 00841 uint32_t tmpI2cUsers; 00842 00843 BSP_ErrorHandler(); 00844 00845 /* De-initialize the I2C communication BUS */ 00846 tmpI2cUsers = v_bspI2cUsers; 00847 I2C1_DeInit(BSP_I2C_ALL_USERS); 00848 00849 /* Re- Initiaize the I2C communication BUS */ 00850 I2C1_Init(tmpI2cUsers); 00851 } 00852 00853 /** 00854 * @brief Reads multiple data. 00855 * @param Addr: I2C address 00856 * @param Reg: Reg address 00857 * @param MemAddress: Memory address 00858 * @param Buffer: Pointer to data buffer 00859 * @param Length: Length of the data 00860 * @retval HAL status 00861 */ 00862 static HAL_StatusTypeDef I2C1_ReadMultiple(uint8_t Addr, 00863 uint16_t Reg, 00864 uint16_t MemAddress, 00865 uint8_t *Buffer, 00866 uint16_t Length) 00867 { 00868 HAL_StatusTypeDef status = HAL_OK; 00869 00870 #if defined(BSP_USE_CMSIS_OS) 00871 /* Get semaphore to prevent multiple I2C access */ 00872 osSemaphoreWait(BspI2cSemaphore, osWaitForever); 00873 #endif 00874 00875 status = HAL_I2C_Mem_Read(&I2c1Handle, Addr, (uint16_t)Reg, MemAddress, Buffer, Length, I2c1Timeout); 00876 00877 #if defined(BSP_USE_CMSIS_OS) 00878 /* Release semaphore to prevent multiple I2C access */ 00879 osSemaphoreRelease(BspI2cSemaphore); 00880 #endif 00881 00882 /* Check the communication status */ 00883 if(status != HAL_OK) 00884 { 00885 /* I2C error occurred */ 00886 I2C1_Error(); 00887 } 00888 return status; 00889 } 00890 00891 /** 00892 * @brief Writes a value in a register of the device through BUS. 00893 * @param Addr: Device address on BUS Bus. 00894 * @param Reg: The target register address to write 00895 * @param MemAddress: Memory address 00896 * @param Buffer: The target register value to be written 00897 * @param Length: buffer size to be written 00898 * @retval HAL status 00899 */ 00900 static HAL_StatusTypeDef I2C1_WriteMultiple(uint8_t Addr, 00901 uint16_t Reg, 00902 uint16_t MemAddress, 00903 uint8_t *Buffer, 00904 uint16_t Length) 00905 { 00906 HAL_StatusTypeDef status = HAL_OK; 00907 00908 #if defined(BSP_USE_CMSIS_OS) 00909 /* Get semaphore to prevent multiple I2C access */ 00910 osSemaphoreWait(BspI2cSemaphore, osWaitForever); 00911 #endif 00912 00913 status = HAL_I2C_Mem_Write(&I2c1Handle, Addr, (uint16_t)Reg, MemAddress, Buffer, Length, I2c1Timeout); 00914 00915 #if defined(BSP_USE_CMSIS_OS) 00916 /* Release semaphore to prevent multiple I2C access */ 00917 osSemaphoreRelease(BspI2cSemaphore); 00918 #endif 00919 00920 /* Check the communication status */ 00921 if(status != HAL_OK) 00922 { 00923 /* Re-Initialize the I2C Bus */ 00924 I2C1_Error(); 00925 } 00926 return status; 00927 } 00928 #endif /*HAL_I2C_MODULE_ENABLED*/ 00929 00930 /******************************************************************************* 00931 LINK OPERATIONS 00932 *******************************************************************************/ 00933 00934 #if defined(HAL_I2C_MODULE_ENABLED) 00935 /********************************* LINK MFX ***********************************/ 00936 /** 00937 * @brief Initializes MFX low level. 00938 * @retval None 00939 */ 00940 void MFX_IO_Init(void) 00941 { 00942 /* I2C1 init */ 00943 I2C1_Init(BSP_I2C_MFX_USER); 00944 00945 /* Wait for device ready */ 00946 if(I2C1_isDeviceReady(IO_I2C_ADDRESS, 4) != HAL_OK) 00947 { 00948 BSP_ErrorHandler(); 00949 } 00950 } 00951 /** 00952 * @brief Deinitializes MFX low level. 00953 * @retval None 00954 */ 00955 void MFX_IO_DeInit(void) 00956 { 00957 GPIO_InitTypeDef GPIO_InitStruct; 00958 00959 /* Enable wakeup gpio clock */ 00960 MFX_WAKEUP_GPIO_CLK_ENABLE(); 00961 00962 /* MFX wakeup pin configuration */ 00963 GPIO_InitStruct.Pin = MFX_WAKEUP_PIN; 00964 GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP; 00965 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_LOW; 00966 GPIO_InitStruct.Pull = GPIO_PULLDOWN; 00967 HAL_GPIO_Init(MFX_WAKEUP_GPIO_PORT, &GPIO_InitStruct); 00968 00969 /* DeInit interrupt pin : disable IRQ before to avoid spurious interrupt */ 00970 HAL_NVIC_DisableIRQ((IRQn_Type)(MFX_INT_EXTI_IRQn)); 00971 MFX_INT_GPIO_CLK_ENABLE(); 00972 HAL_GPIO_DeInit(MFX_INT_GPIO_PORT, MFX_INT_PIN); 00973 00974 /* I2C1 Deinit */ 00975 I2C1_DeInit(BSP_I2C_MFX_USER); 00976 } 00977 00978 /** 00979 * @brief Configures MFX low level interrupt. 00980 * @retval None 00981 */ 00982 void MFX_IO_ITConfig(void) 00983 { 00984 GPIO_InitTypeDef GPIO_InitStruct; 00985 00986 /* Enable the GPIO clock */ 00987 MFX_INT_GPIO_CLK_ENABLE(); 00988 00989 /* MFX_OUT_IRQ (normally used for EXTI_WKUP) */ 00990 GPIO_InitStruct.Pin = MFX_INT_PIN; 00991 GPIO_InitStruct.Pull = GPIO_NOPULL; 00992 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_LOW; 00993 GPIO_InitStruct.Mode = GPIO_MODE_IT_RISING; 00994 HAL_GPIO_Init(MFX_INT_GPIO_PORT, &GPIO_InitStruct); 00995 00996 /* Enable and set GPIO EXTI Interrupt to the lowest priority */ 00997 HAL_NVIC_SetPriority((IRQn_Type)(MFX_INT_EXTI_IRQn), 0x0F, 0x0F); 00998 HAL_NVIC_EnableIRQ((IRQn_Type)(MFX_INT_EXTI_IRQn)); 00999 } 01000 01001 /** 01002 * @brief Configures MFX wke up pin. 01003 * @retval None 01004 */ 01005 void MFX_IO_EnableWakeupPin(void) 01006 { 01007 GPIO_InitTypeDef GPIO_InitStruct; 01008 01009 /* Enable wakeup gpio clock */ 01010 MFX_WAKEUP_GPIO_CLK_ENABLE(); 01011 01012 /* MFX wakeup pin configuration */ 01013 GPIO_InitStruct.Pin = MFX_WAKEUP_PIN; 01014 GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP; 01015 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_HIGH; 01016 GPIO_InitStruct.Pull = GPIO_NOPULL; 01017 HAL_GPIO_Init(MFX_WAKEUP_GPIO_PORT, &GPIO_InitStruct); 01018 } 01019 01020 /** 01021 * @brief Wakeup MFX. 01022 * @retval None 01023 */ 01024 void MFX_IO_Wakeup(void) 01025 { 01026 /* Set Wakeup pin to high to wakeup Idd measurement component from standby mode */ 01027 HAL_GPIO_WritePin(MFX_WAKEUP_GPIO_PORT, MFX_WAKEUP_PIN, GPIO_PIN_SET); 01028 01029 /* Wait */ 01030 HAL_Delay(1); 01031 01032 /* Set gpio pin basck to low */ 01033 HAL_GPIO_WritePin(MFX_WAKEUP_GPIO_PORT, MFX_WAKEUP_PIN, GPIO_PIN_RESET); 01034 } 01035 01036 /** 01037 * @brief MFX writes single data. 01038 * @param Addr: I2C address 01039 * @param Reg: Register address 01040 * @param Value: Data to be written 01041 * @retval None 01042 */ 01043 void MFX_IO_Write(uint16_t Addr, uint8_t Reg, uint8_t Value) 01044 { 01045 I2C1_WriteData(Addr, Reg, I2C_MEMADD_SIZE_8BIT, Value); 01046 } 01047 01048 /** 01049 * @brief MFX reads single data. 01050 * @param Addr: I2C address 01051 * @param Reg: Register address 01052 * @retval Read data 01053 */ 01054 uint8_t MFX_IO_Read(uint16_t Addr, uint8_t Reg) 01055 { 01056 return I2C1_ReadData(Addr, Reg, I2C_MEMADD_SIZE_8BIT); 01057 } 01058 01059 /** 01060 * @brief MFX reads multiple data. 01061 * @param Addr: I2C address 01062 * @param Reg: Register address 01063 * @param Buffer: Pointer to data buffer 01064 * @param Length: Length of the data 01065 * @retval Number of read data 01066 */ 01067 uint16_t MFX_IO_ReadMultiple(uint16_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length) 01068 { 01069 return I2C1_ReadBuffer(Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT, Buffer, Length); 01070 } 01071 01072 /** 01073 * @brief MFX writes multiple data. 01074 * @param Addr: I2C address 01075 * @param Reg: Register address 01076 * @param Buffer: Pointer to data buffer 01077 * @param Length: Length of the data 01078 * @retval None 01079 */ 01080 void MFX_IO_WriteMultiple(uint16_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length) 01081 { 01082 I2C1_WriteBuffer(Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT, Buffer, Length); 01083 } 01084 01085 /** 01086 * @brief MFX delay 01087 * @param Delay: Delay in ms 01088 * @retval None 01089 */ 01090 void MFX_IO_Delay(uint32_t Delay) 01091 { 01092 HAL_Delay(Delay); 01093 } 01094 01095 01096 /********************************* LINK AUDIO *********************************/ 01097 /** 01098 * @brief Initializes Audio low level. 01099 * @retval None 01100 */ 01101 void AUDIO_IO_Init(void) 01102 { 01103 uint8_t Value; 01104 01105 /* Initialized BSP IO */ 01106 BSP_IO_Init(); 01107 01108 /* Audio reset pin configuration */ 01109 BSP_IO_ConfigPin(AUDIO_RESET_PIN, IO_MODE_OUTPUT_PP_PD); 01110 01111 /* I2C bus init */ 01112 I2C1_Init(BSP_I2C_AUDIO_USER); 01113 01114 /* Power off the codec */ 01115 CODEC_AUDIO_POWER_OFF(); 01116 01117 /* wait until power supplies are stable */ 01118 HAL_Delay(10); 01119 01120 /* Power on the codec */ 01121 CODEC_AUDIO_POWER_ON(); 01122 01123 /* Wait at least 500ns after reset */ 01124 HAL_Delay(1); 01125 01126 /* Set the device in standby mode */ 01127 Value = AUDIO_IO_Read(AUDIO_I2C_ADDRESS, 0x02); 01128 AUDIO_IO_Write(AUDIO_I2C_ADDRESS, 0x02, (Value | 0x01)); 01129 01130 /* Set all power down bits to 1 */ 01131 AUDIO_IO_Write(AUDIO_I2C_ADDRESS, 0x02, 0x7F); 01132 Value = AUDIO_IO_Read(AUDIO_I2C_ADDRESS, 0x03); 01133 AUDIO_IO_Write(AUDIO_I2C_ADDRESS, 0x03, (Value | 0x0E)); 01134 } 01135 01136 /** 01137 * @brief Deinitializes Audio low level. 01138 * @retval None 01139 */ 01140 void AUDIO_IO_DeInit(void) 01141 { 01142 uint8_t Value; 01143 01144 /* Mute DAC and ADC */ 01145 Value = AUDIO_IO_Read(AUDIO_I2C_ADDRESS, 0x08); 01146 AUDIO_IO_Write(AUDIO_I2C_ADDRESS, 0x08, (Value | 0x03)); 01147 Value = AUDIO_IO_Read(AUDIO_I2C_ADDRESS, 0x07); 01148 AUDIO_IO_Write(AUDIO_I2C_ADDRESS, 0x07, (Value | 0x03)); 01149 01150 /* Disable soft ramp and zero cross */ 01151 Value = AUDIO_IO_Read(AUDIO_I2C_ADDRESS, 0x06); 01152 AUDIO_IO_Write(AUDIO_I2C_ADDRESS, 0x06, (Value & 0xF0)); 01153 01154 /* Set PDN to 1 */ 01155 Value = AUDIO_IO_Read(AUDIO_I2C_ADDRESS, 0x02); 01156 AUDIO_IO_Write(AUDIO_I2C_ADDRESS, 0x02, (Value | 0x01)); 01157 01158 /* Set all power down bits to 1 */ 01159 AUDIO_IO_Write(AUDIO_I2C_ADDRESS, 0x02, 0x7F); 01160 Value = AUDIO_IO_Read(AUDIO_I2C_ADDRESS, 0x03); 01161 AUDIO_IO_Write(AUDIO_I2C_ADDRESS, 0x03, (Value | 0x0E)); 01162 01163 /* Power off the codec */ 01164 CODEC_AUDIO_POWER_OFF(); 01165 01166 /* I2C bus Deinit */ 01167 I2C1_DeInit(BSP_I2C_AUDIO_USER); 01168 } 01169 01170 /** 01171 * @brief Writes a single data. 01172 * @param Addr: I2C address 01173 * @param Reg: Reg address 01174 * @param Value: Data to be written 01175 * @retval None 01176 */ 01177 void AUDIO_IO_Write(uint8_t Addr, uint8_t Reg, uint8_t Value) 01178 { 01179 I2C1_WriteBuffer(Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT, &Value, 1); 01180 } 01181 01182 /** 01183 * @brief Reads a single data. 01184 * @param Addr: I2C address 01185 * @param Reg: Reg address 01186 * @retval Data to be read 01187 */ 01188 uint8_t AUDIO_IO_Read(uint8_t Addr, uint8_t Reg) 01189 { 01190 uint8_t Read_Value = 0; 01191 01192 I2C1_ReadBuffer((uint16_t) Addr, (uint16_t) Reg, I2C_MEMADD_SIZE_8BIT, &Read_Value, 1); 01193 01194 return Read_Value; 01195 } 01196 01197 /** 01198 * @brief AUDIO Codec delay 01199 * @param Delay: Delay in ms 01200 * @retval None 01201 */ 01202 void AUDIO_IO_Delay(uint32_t Delay) 01203 { 01204 HAL_Delay(Delay); 01205 } 01206 01207 /******************************************************************************* 01208 LINK OPERATIONS 01209 *******************************************************************************/ 01210 01211 /************************** LINK TS (TouchScreen) *****************************/ 01212 /** 01213 * @brief Initializes Touchscreen low level. 01214 * @retval None 01215 */ 01216 void TS_IO_Init(void) 01217 { 01218 I2C1_Init(BSP_I2C_TS_USER); 01219 } 01220 01221 /** 01222 * @brief Writes a single data. 01223 * @param Addr: I2C address 01224 * @param Reg: Reg address 01225 * @param Value: Data to be written 01226 * @retval None 01227 */ 01228 void TS_IO_Write(uint8_t Addr, uint8_t Reg, uint8_t Value) 01229 { 01230 I2C1_WriteMultiple(Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT,(uint8_t*)&Value, 1); 01231 } 01232 01233 /** 01234 * @brief Reads a single data. 01235 * @param Addr: I2C address 01236 * @param Reg: Reg address 01237 * @retval Data to be read 01238 */ 01239 uint8_t TS_IO_Read(uint8_t Addr, uint8_t Reg) 01240 { 01241 return I2C1_ReadData(Addr, Reg, I2C_MEMADD_SIZE_8BIT); 01242 } 01243 01244 /** 01245 * @brief Reads multiple data with I2C communication 01246 * channel from TouchScreen. 01247 * @param Addr: I2C address 01248 * @param Reg: Register address 01249 * @param Buffer: Pointer to data buffer 01250 * @param Length: Length of the data 01251 * @retval Number of read data 01252 */ 01253 uint16_t TS_IO_ReadMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length) 01254 { 01255 return I2C1_ReadMultiple(Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT, Buffer, Length); 01256 } 01257 01258 /** 01259 * @brief Writes multiple data with I2C communication 01260 * channel from MCU to TouchScreen. 01261 * @param Addr: I2C address 01262 * @param Reg: Register address 01263 * @param Buffer: Pointer to data buffer 01264 * @param Length: Length of the data 01265 * @retval None 01266 */ 01267 void TS_IO_WriteMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length) 01268 { 01269 I2C1_WriteMultiple(Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT, Buffer, Length); 01270 } 01271 01272 /** 01273 * @brief Delay function used in TouchScreen low level driver. 01274 * @param Delay: Delay in ms 01275 * @retval None 01276 */ 01277 void TS_IO_Delay(uint32_t Delay) 01278 { 01279 HAL_Delay(Delay); 01280 } 01281 01282 01283 /************************** Camera *****************************/ 01284 /** 01285 * @brief Initializes Camera low level. 01286 * @retval None 01287 */ 01288 void CAMERA_IO_Init(void) 01289 { 01290 I2C1_Init(BSP_I2C_CAMERA_USER); 01291 } 01292 01293 /** 01294 * @brief Camera writes single data. 01295 * @param Addr: I2C address 01296 * @param Reg: Register address 01297 * @param Value: Data to be written 01298 * @retval None 01299 */ 01300 void CAMERA_IO_Write(uint8_t Addr, uint8_t Reg, uint8_t Value) 01301 { 01302 I2C1_WriteMultiple(Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT,(uint8_t*)&Value, 1); 01303 } 01304 01305 /** 01306 * @brief Camera reads single data. 01307 * @param Addr: I2C address 01308 * @param Reg: Register address 01309 * @retval Read data 01310 */ 01311 uint8_t CAMERA_IO_Read(uint8_t Addr, uint8_t Reg) 01312 { 01313 uint8_t read_value = 0; 01314 01315 I2C1_ReadMultiple(Addr, Reg, I2C_MEMADD_SIZE_8BIT, (uint8_t*)&read_value, 1); 01316 01317 return read_value; 01318 } 01319 01320 /** 01321 * @brief Camera delay 01322 * @param Delay: Delay in ms 01323 * @retval None 01324 */ 01325 void CAMERA_Delay(uint32_t Delay) 01326 { 01327 HAL_Delay(Delay); 01328 } 01329 01330 #endif /* HAL_I2C_MODULE_ENABLED */ 01331 01332 /** 01333 * @} 01334 */ 01335 01336 /** 01337 * @} 01338 */ 01339 01340 /** 01341 * @} 01342 */ 01343 01344 /** 01345 * @} 01346 */ 01347 01348 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Fri Oct 13 2017 02:37:42 for STM32L4R9I-Discovery BSP User Manual by
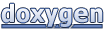