STM32L476G_EVAL BSP User Manual
|
stm32l476g_eval_io.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l476g_eval_io.c 00004 * @author MCD Application Team 00005 * @version $VERSION$ 00006 * @date $DATE$ 00007 * @brief This file provides a set of functions needed to manage the IO pins 00008 * on STM32L476G-EVAL evaluation board. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* File Info : ----------------------------------------------------------------- 00040 User NOTES 00041 1. How To use this driver: 00042 -------------------------- 00043 - This driver is used to drive the IO module of the STM32L476G-EVAL evaluation 00044 board. 00045 - The STMPE811 and STMPE1600 IO expander device component driver must be included with this 00046 driver in order to run the IO functionalities commanded by the IO expander 00047 device mounted on the evaluation board. 00048 00049 2. Driver description: 00050 --------------------- 00051 + Initialization steps: 00052 o Initialize the IO module using the BSP_IO_Init() function. This 00053 function includes the MSP layer hardware resources initialization and the 00054 communication layer configuration to start the IO functionalities use. 00055 00056 + IO functionalities use 00057 o The IO pin mode is configured when calling the function BSP_IO_ConfigPin(), you 00058 must specify the desired IO mode by choosing the "IO_ModeTypedef" parameter 00059 predefined value. 00060 o If an IO pin is used in interrupt mode, the function BSP_IO_ITGetStatus() is 00061 needed to get the interrupt status. To clear the IT pending bits, you should 00062 call the function BSP_IO_ITClear() with specifying the IO pending bit to clear. 00063 o The IT is handled using the corresponding external interrupt IRQ handler, 00064 the user IT callback treatment is implemented on the same external interrupt 00065 callback. 00066 o To get/set an IO pin combination state you can use the functions 00067 BSP_IO_ReadPin()/BSP_IO_WritePin() or the function BSP_IO_TogglePin() to toggle the pin 00068 state. 00069 00070 ------------------------------------------------------------------------------*/ 00071 00072 /* Includes ------------------------------------------------------------------*/ 00073 #include "stm32l476g_eval_io.h" 00074 00075 /** @addtogroup BSP 00076 * @{ 00077 */ 00078 00079 /** @addtogroup STM32L476G_EVAL 00080 * @{ 00081 */ 00082 00083 /** @defgroup STM32L476G_EVAL_IO STM32L476G_EVAL IO 00084 * @{ 00085 */ 00086 00087 /* Private constants ---------------------------------------------------------*/ 00088 00089 /** @defgroup STM32L476G_EVAL_IO_Private_Constants Private Constants 00090 * @{ 00091 */ 00092 00093 /** 00094 * @} 00095 */ 00096 00097 /* Private macros -------------------------------------------------------------*/ 00098 00099 /** @defgroup STM32L476G_EVAL_IO_Private_Macros Private Macros 00100 * @{ 00101 */ 00102 00103 /** 00104 * @} 00105 */ 00106 00107 /* Private variables ---------------------------------------------------------*/ 00108 00109 /** @defgroup STM32L476G_EVAL_IO_Private_Variables Private Variables 00110 * @{ 00111 */ 00112 static IO_DrvTypeDef *io1_driver; 00113 static IO_DrvTypeDef *io2_driver; 00114 00115 /** 00116 * @} 00117 */ 00118 00119 /* Private function prototypes -----------------------------------------------*/ 00120 00121 /** @defgroup STM32L476G_EVAL_IO_Private_Functions Private Functions 00122 * @{ 00123 */ 00124 00125 /** 00126 * @} 00127 */ 00128 00129 /* Private functions ---------------------------------------------------------*/ 00130 00131 /** @addtogroup STM32L476G_EVAL_IO_Exported_Functions 00132 * @{ 00133 */ 00134 00135 /** 00136 * @brief Initializes and configures the IO functionalities and configures all 00137 * necessary hardware resources (GPIOs, clocks..). 00138 * @note BSP_IO_Init() is using HAL_Delay() function to ensure that stmpe811 00139 * IO Expander is correctly reset. HAL_Delay() function provides accurate 00140 * delay (in milliseconds) based on variable incremented in SysTick ISR. 00141 * This implies that if BSP_IO_Init() is called from a peripheral ISR process, 00142 * then the SysTick interrupt must have higher priority (numerically lower) 00143 * than the peripheral interrupt. Otherwise the caller ISR process will be blocked. 00144 * @retval IO_OK: if all initializations are OK. Other value if error. 00145 */ 00146 uint8_t BSP_IO_Init(void) 00147 { 00148 uint8_t ret = IO_ERROR; 00149 00150 /* Initialize IO Expander 1*/ 00151 if(stmpe811_io_drv.ReadID(IO1_I2C_ADDRESS) == STMPE811_ID) 00152 { 00153 if(io1_driver != &stmpe811_io_drv) 00154 { 00155 /* Initialize the IO Expander 1 driver structure */ 00156 io1_driver = &stmpe811_io_drv; 00157 00158 io1_driver->Init(IO1_I2C_ADDRESS); 00159 io1_driver->Start(IO1_I2C_ADDRESS, IO1_PIN_ALL >> IO1_PIN_OFFSET); 00160 } 00161 ret = IO_OK; 00162 } 00163 00164 if(stmpe1600_io_drv.ReadID(IO2_I2C_ADDRESS) == STMPE1600_ID) 00165 { 00166 if(io2_driver != &stmpe1600_io_drv) 00167 { 00168 /* Initialize the IO Expander 2 driver structure */ 00169 io2_driver = &stmpe1600_io_drv; 00170 00171 io2_driver->Init(IO2_I2C_ADDRESS); 00172 io2_driver->Start(IO2_I2C_ADDRESS, IO2_PIN_ALL >> IO2_PIN_OFFSET); 00173 } 00174 ret = IO_OK; 00175 } 00176 00177 return ret; 00178 } 00179 00180 /** 00181 * @brief Gets the selected pins IT status. 00182 * @param IO_Pin: Selected pins to check the status. 00183 * This parameter can be any combination of the IO pins. 00184 * @retval Status of the checked IO pin(s). 00185 */ 00186 uint32_t BSP_IO_ITGetStatus(uint32_t IO_Pin) 00187 { 00188 uint32_t status = 0; 00189 uint32_t io1_pin = 0; 00190 uint32_t io2_pin = 0; 00191 00192 io1_pin = (IO_Pin & IO1_PIN_ALL) >> IO1_PIN_OFFSET; 00193 io2_pin = (IO_Pin & IO2_PIN_ALL) >> IO2_PIN_OFFSET; 00194 00195 if (io1_pin != 0) 00196 { 00197 /* Return the IO Expander 1 Pin IT status */ 00198 status |= (io1_driver->ITStatus(IO1_I2C_ADDRESS, io1_pin)) << IO1_PIN_OFFSET; 00199 } 00200 00201 if (io2_pin != 0) 00202 { 00203 /* Return the IO Expander 2 Pin IT status */ 00204 status |= (io2_driver->ITStatus(IO2_I2C_ADDRESS, io2_pin)) << IO2_PIN_OFFSET; 00205 } 00206 00207 return status; 00208 } 00209 00210 /** 00211 * @brief Clears the selected IO IT pending bit. 00212 * @param IO_Pin: Selected pins to check the status. 00213 * This parameter can be any combination of the IO pins. 00214 * @retval None 00215 */ 00216 void BSP_IO_ITClear(uint32_t IO_Pin) 00217 { 00218 uint32_t io1_pin = 0; 00219 uint32_t io2_pin = 0; 00220 00221 io1_pin = (IO_Pin & IO1_PIN_ALL) >> IO1_PIN_OFFSET; 00222 io2_pin = (IO_Pin & IO2_PIN_ALL) >> IO2_PIN_OFFSET; 00223 00224 if (io1_pin != 0) 00225 { 00226 /* Clears the selected IO Expander 1 pin(s) mode */ 00227 io1_driver->ClearIT(IO1_I2C_ADDRESS, io1_pin); 00228 } 00229 00230 if (io2_pin != 0) 00231 { 00232 /* Clears the selected IO Expander 2 pin(s) mode */ 00233 io2_driver->ClearIT(IO2_I2C_ADDRESS, io2_pin); 00234 } 00235 } 00236 00237 /** 00238 * @brief Configures the IO pin(s) according to IO mode structure value. 00239 * @param IO_Pin: Output pin to be set or reset. 00240 * This parameter can be any combination of the IO pins. 00241 * @param IO_Mode: IO pin mode to configure 00242 * This parameter can be one of the following values: 00243 * @arg IO_MODE_INPUT 00244 * @arg IO_MODE_OUTPUT 00245 * @arg IO_MODE_IT_RISING_EDGE 00246 * @arg IO_MODE_IT_FALLING_EDGE 00247 * @arg IO_MODE_IT_LOW_LEVEL 00248 * @arg IO_MODE_IT_HIGH_LEVEL 00249 * @retval IO_OK: if all initializations are OK. Other value if error. 00250 */ 00251 uint8_t BSP_IO_ConfigPin(uint32_t IO_Pin, IO_ModeTypedef IO_Mode) 00252 { 00253 uint32_t io1_pin = 0; 00254 uint32_t io2_pin = 0; 00255 00256 io1_pin = (IO_Pin & IO1_PIN_ALL) >> IO1_PIN_OFFSET; 00257 io2_pin = (IO_Pin & IO2_PIN_ALL) >> IO2_PIN_OFFSET; 00258 00259 if (io1_pin != 0) 00260 { 00261 /* Configure the selected IO Expander 1 pin(s) mode */ 00262 io1_driver->Config(IO1_I2C_ADDRESS, io1_pin, IO_Mode); 00263 } 00264 00265 if (io2_pin != 0) 00266 { 00267 /* Configure the selected IO Expander 2 pin(s) mode */ 00268 io2_driver->Config(IO2_I2C_ADDRESS, io2_pin, IO_Mode); 00269 } 00270 00271 return IO_OK; 00272 } 00273 00274 /** 00275 * @brief Sets the selected pins state. 00276 * @param IO_Pin: Selected pins to write. 00277 * This parameter can be any combination of the IO pins. 00278 * @param PinState: New pins state to write 00279 * @retval None 00280 */ 00281 void BSP_IO_WritePin(uint32_t IO_Pin, uint8_t PinState) 00282 { 00283 uint32_t io1_pin = 0; 00284 uint32_t io2_pin = 0; 00285 00286 io1_pin = (IO_Pin & IO1_PIN_ALL) >> IO1_PIN_OFFSET; 00287 io2_pin = (IO_Pin & IO2_PIN_ALL) >> IO2_PIN_OFFSET; 00288 00289 if (io1_pin != 0) 00290 { 00291 /* Sets the IO Expander 1 selected pins state */ 00292 io1_driver->WritePin(IO1_I2C_ADDRESS, io1_pin, PinState); 00293 } 00294 00295 if (io2_pin != 0) 00296 { 00297 /* Sets the IO Expander 2 selected pins state */ 00298 io2_driver->WritePin(IO2_I2C_ADDRESS, io2_pin, PinState); 00299 } 00300 } 00301 00302 /** 00303 * @brief Gets the selected pins current state. 00304 * @param IO_Pin: Selected pins to read. 00305 * This parameter can be any combination of the IO pins. 00306 * @retval The current pins state 00307 */ 00308 uint32_t BSP_IO_ReadPin(uint32_t IO_Pin) 00309 { 00310 uint32_t pin_state = 0; 00311 uint32_t io1_pin = 0; 00312 uint32_t io2_pin = 0; 00313 00314 io1_pin = (IO_Pin & IO1_PIN_ALL) >> IO1_PIN_OFFSET; 00315 io2_pin = (IO_Pin & IO2_PIN_ALL) >> IO2_PIN_OFFSET; 00316 00317 if (io1_pin != 0) 00318 { 00319 /* Gets the IO Expander 1 selected pins current state */ 00320 pin_state |= (io1_driver->ReadPin(IO1_I2C_ADDRESS, io1_pin)) << IO1_PIN_OFFSET; 00321 } 00322 00323 if (io2_pin != 0) 00324 { 00325 /* Gets the IO Expander 2 selected pins current state */ 00326 pin_state |= (io2_driver->ReadPin(IO2_I2C_ADDRESS, io2_pin)) << IO2_PIN_OFFSET; 00327 } 00328 00329 return pin_state; 00330 } 00331 00332 /** 00333 * @brief Toggles the selected pins state 00334 * @param IO_Pin: Selected pins to toggle. 00335 * This parameter can be any combination of the IO pins. 00336 * @retval None 00337 */ 00338 void BSP_IO_TogglePin(uint32_t IO_Pin) 00339 { 00340 uint32_t io1_pin = 0; 00341 uint32_t io2_pin = 0; 00342 00343 io1_pin = (IO_Pin & IO1_PIN_ALL) >> IO1_PIN_OFFSET; 00344 io2_pin = (IO_Pin & IO2_PIN_ALL) >> IO2_PIN_OFFSET; 00345 00346 if (io1_pin != 0) 00347 { 00348 /* Toggles the IO Expander 1 selected pins state */ 00349 if(io1_driver->ReadPin(IO1_I2C_ADDRESS, io1_pin) == RESET) /* Set */ 00350 { 00351 BSP_IO_WritePin(io1_pin, GPIO_PIN_SET); /* Reset */ 00352 } 00353 else 00354 { 00355 BSP_IO_WritePin(io1_pin, GPIO_PIN_RESET); /* Set */ 00356 } 00357 } 00358 00359 if (io2_pin != 0) 00360 { 00361 /* Toggles the IO Expander 2 selected pins state */ 00362 if(io2_driver->ReadPin(IO2_I2C_ADDRESS, io2_pin) == RESET) /* Set */ 00363 { 00364 BSP_IO_WritePin(io2_pin, GPIO_PIN_SET); /* Reset */ 00365 } 00366 else 00367 { 00368 BSP_IO_WritePin(io2_pin, GPIO_PIN_RESET); /* Set */ 00369 } 00370 } 00371 } 00372 00373 /** 00374 * @} 00375 */ 00376 00377 /** 00378 * @} 00379 */ 00380 00381 /** 00382 * @} 00383 */ 00384 00385 /** 00386 * @} 00387 */ 00388 00389 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Sun Jun 21 2015 23:46:41 for STM32L476G_EVAL BSP User Manual by
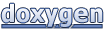