STM32L476G_EVAL BSP User Manual
|
stm32l476g_eval_glass_lcd.h
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l476g_eval_glass_lcd.h 00004 * @author MCD Application Team 00005 * @version $VERSION$ 00006 * @date $DATE$ 00007 * @brief Header file for stm32l476g_eval_glass_lcd.c module. 00008 ****************************************************************************** 00009 * @attention 00010 * 00011 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00012 * 00013 * Redistribution and use in source and binary forms, with or without modification, 00014 * are permitted provided that the following conditions are met: 00015 * 1. Redistributions of source code must retain the above copyright notice, 00016 * this list of conditions and the following disclaimer. 00017 * 2. Redistributions in binary form must reproduce the above copyright notice, 00018 * this list of conditions and the following disclaimer in the documentation 00019 * and/or other materials provided with the distribution. 00020 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00021 * may be used to endorse or promote products derived from this software 00022 * without specific prior written permission. 00023 * 00024 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00025 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00026 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00027 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00028 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00029 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00030 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00031 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00032 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 * 00035 ****************************************************************************** 00036 */ 00037 00038 /* Define to prevent recursive inclusion -------------------------------------*/ 00039 #ifndef __STM32L476G_EVAL_GLASS_LCD_H 00040 #define __STM32L476G_EVAL_GLASS_LCD_H 00041 00042 #ifdef __cplusplus 00043 extern "C" { 00044 #endif 00045 00046 /* Includes ------------------------------------------------------------------*/ 00047 #include "stm32l476g_eval.h" 00048 00049 /** @addtogroup BSP 00050 * @{ 00051 */ 00052 00053 /** @addtogroup STM32L476G_EVAL 00054 * @{ 00055 */ 00056 00057 /** @addtogroup STM32L476G_EVAL_GLASS_LCD 00058 * @{ 00059 */ 00060 00061 /* Exported types ------------------------------------------------------------*/ 00062 00063 /** @defgroup STM32L476G_EVAL_GLASS_LCD_Exported_Types Exported Types 00064 * @{ 00065 */ 00066 /** 00067 * @brief LCD Glass point 00068 * Warning: element values correspond to LCD Glass point. 00069 */ 00070 00071 typedef enum 00072 { 00073 POINT_OFF = 0, 00074 POINT_ON = 1 00075 }Point_Typedef; 00076 00077 /** 00078 * @brief LCD Glass Double point 00079 * Warning: element values correspond to LCD Glass Double point. 00080 */ 00081 typedef enum 00082 { 00083 DOUBLEPOINT_OFF = 0, 00084 DOUBLEPOINT_ON = 1 00085 }DoublePoint_Typedef; 00086 00087 /** 00088 * @brief LCD Glass Battery Level 00089 * Warning: element values correspond to LCD Glass Battery Level. 00090 */ 00091 00092 typedef enum 00093 { 00094 BATTERYLEVEL_OFF = 0, 00095 BATTERYLEVEL_1_4 = 1, 00096 BATTERYLEVEL_1_2 = 2, 00097 BATTERYLEVEL_3_4 = 3, 00098 BATTERYLEVEL_FULL = 4 00099 }BatteryLevel_TypeDef; 00100 00101 /** 00102 * @brief LCD Glass Temperature Level 00103 * Warning: element values correspond to LCD Glass Temperature Level. 00104 */ 00105 00106 typedef enum 00107 { 00108 TEMPERATURELEVEL_OFF = 0, 00109 TEMPERATURELEVEL_1 = 1, 00110 TEMPERATURELEVEL_2 = 2, 00111 TEMPERATURELEVEL_3 = 3, 00112 TEMPERATURELEVEL_4 = 4, 00113 TEMPERATURELEVEL_5 = 5, 00114 TEMPERATURELEVEL_6 = 6 00115 }TemperatureLevel_TypeDef; 00116 00117 /** 00118 * @brief LCD Glass Arrow Direction 00119 * Warning: element values correspond to LCD Glass Arrow Direction. 00120 */ 00121 00122 typedef enum 00123 { 00124 ARROWDIRECTION_OFF = 0, 00125 ARROWDIRECTION_UP = 1, 00126 ARROWDIRECTION_DOWN = 2, 00127 ARROWDIRECTION_LEFT = 3, 00128 ARROWDIRECTION_RIGHT = 4 00129 }ArrowDirection_TypeDef; 00130 00131 /** 00132 * @brief LCD Glass Value Unit 00133 * Warning: element values correspond to LCD Glass Value Unit. 00134 */ 00135 typedef enum 00136 { 00137 VALUEUNIT_OFF = 0, 00138 VALUEUNIT_MILLIAMPERE = 1, 00139 VALUEUNIT_MICROAMPERE = 2, 00140 VALUEUNIT_NANOAMPERE = 3 00141 }ValueUnit_TypeDef; 00142 00143 /** 00144 * @brief LCD Glass Sign 00145 * Warning: element values correspond to LCD Glass Sign. 00146 */ 00147 typedef enum 00148 { 00149 SIGN_POSITIVE = 0, 00150 SIGN_NEGATIVE = 1 00151 }Sign_TypeDef; 00152 00153 /** 00154 * @brief LCD Glass Pixel Row 00155 * Warning: element values correspond to LCD Glass Pixel Row. 00156 */ 00157 typedef enum 00158 { 00159 PIXELROW_1 = 1, 00160 PIXELROW_2 = 2, 00161 PIXELROW_3 = 3, 00162 PIXELROW_4 = 4, 00163 PIXELROW_5 = 5, 00164 PIXELROW_6 = 6, 00165 PIXELROW_7 = 7, 00166 PIXELROW_8 = 8, 00167 PIXELROW_9 = 9, 00168 PIXELROW_10 = 10 00169 }PixelRow_TypeDef; 00170 00171 /** 00172 * @brief LCD Glass Pixel Column 00173 * Warning: element values correspond to LCD Glass Pixel Column. 00174 */ 00175 typedef enum 00176 { 00177 PIXELCOLUMN_1 = 1, 00178 PIXELCOLUMN_2 = 2, 00179 PIXELCOLUMN_3 = 3, 00180 PIXELCOLUMN_4 = 4, 00181 PIXELCOLUMN_5 = 5, 00182 PIXELCOLUMN_6 = 6, 00183 PIXELCOLUMN_7 = 7, 00184 PIXELCOLUMN_8 = 8, 00185 PIXELCOLUMN_9 = 9, 00186 PIXELCOLUMN_10 = 10, 00187 PIXELCOLUMN_11 = 11, 00188 PIXELCOLUMN_12 = 12, 00189 PIXELCOLUMN_13 = 13, 00190 PIXELCOLUMN_14 = 14, 00191 PIXELCOLUMN_15 = 15, 00192 PIXELCOLUMN_16 = 16, 00193 PIXELCOLUMN_17 = 17, 00194 PIXELCOLUMN_18 = 18, 00195 PIXELCOLUMN_19 = 19 00196 }PixelColumn_TypeDef; 00197 00198 /** 00199 * @} 00200 */ 00201 00202 /** @defgroup STM32L476G_EVAL_GLASS_LCD_Exported_Constants Exported Constants 00203 * @{ 00204 */ 00205 00206 /* Define for scrolling sentences*/ 00207 #define SCROLL_SPEED 200 00208 #define SCROLL_SPEED_L 400 00209 #define SCROLL_NUM 1 00210 00211 #define DOT 0x8000 /* for add decimal point in string */ 00212 #define DOUBLE_DOT 0x4000 /* for add decimal point in string */ 00213 00214 /** 00215 * @} 00216 */ 00217 00218 /* Exported functions --------------------------------------------------------*/ 00219 00220 /** @defgroup STM32L476G_EVAL_GLASS_LCD_Exported_Functions Exported Functions 00221 * @{ 00222 */ 00223 void BSP_LCD_GLASS_Init(void); 00224 void BSP_LCD_GLASS_BlinkConfig(uint32_t BlinkMode, uint32_t BlinkFrequency); 00225 void BSP_LCD_GLASS_Contrast(uint32_t Contrast); 00226 void BSP_LCD_GLASS_DisplayChar(uint8_t* Ch, Point_Typedef Point, DoublePoint_Typedef DoublePoint, uint8_t Position); 00227 void BSP_LCD_GLASS_DisplayString(uint8_t* ptr); 00228 void BSP_LCD_GLASS_WriteChar(uint8_t* ch, uint8_t Point, uint8_t Column, uint8_t Position); 00229 void BSP_LCD_GLASS_ClearChar(uint8_t position); 00230 void BSP_LCD_GLASS_DisplayStrDeci(uint16_t* ptr); 00231 void BSP_LCD_GLASS_Clear(void); 00232 00233 void BSP_LCD_GLASS_ClearTextZone(void); 00234 void BSP_LCD_GLASS_DisplayLogo(FunctionalState NewState); 00235 void BSP_LCD_GLASS_ArrowConfig(ArrowDirection_TypeDef ArrowDirection); 00236 void BSP_LCD_GLASS_TemperatureConfig(TemperatureLevel_TypeDef TemperatureLevel); 00237 void BSP_LCD_GLASS_ValueUnitConfig(ValueUnit_TypeDef ValueUnit); 00238 void BSP_LCD_GLASS_SignCmd(Sign_TypeDef Sign, FunctionalState NewState); 00239 00240 void BSP_LCD_GLASS_WriteMatrixPixel(PixelRow_TypeDef PixelRow, PixelColumn_TypeDef PixelColumn); 00241 void BSP_LCD_GLASS_ClearMatrixPixel(PixelRow_TypeDef PixelRow, PixelColumn_TypeDef PixelColumn); 00242 00243 void BSP_LCD_GLASS_ScrollSentence(uint8_t* ptr, uint16_t nScroll, uint16_t ScrollSpeed); 00244 void BSP_LCD_GLASS_BarLevelConfig(uint8_t BarLevel); 00245 00246 /** 00247 * @} 00248 */ 00249 00250 /** 00251 * @} 00252 */ 00253 00254 /** 00255 * @} 00256 */ 00257 00258 /** 00259 * @} 00260 */ 00261 00262 #ifdef __cplusplus 00263 } 00264 #endif 00265 00266 #endif /* __STM32L476G_EVAL_GLASS_LCD_H */ 00267 00268 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Sun Jun 21 2015 23:46:41 for STM32L476G_EVAL BSP User Manual by
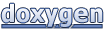